Conquering Time Zone Challenges in Java with Joda-Time
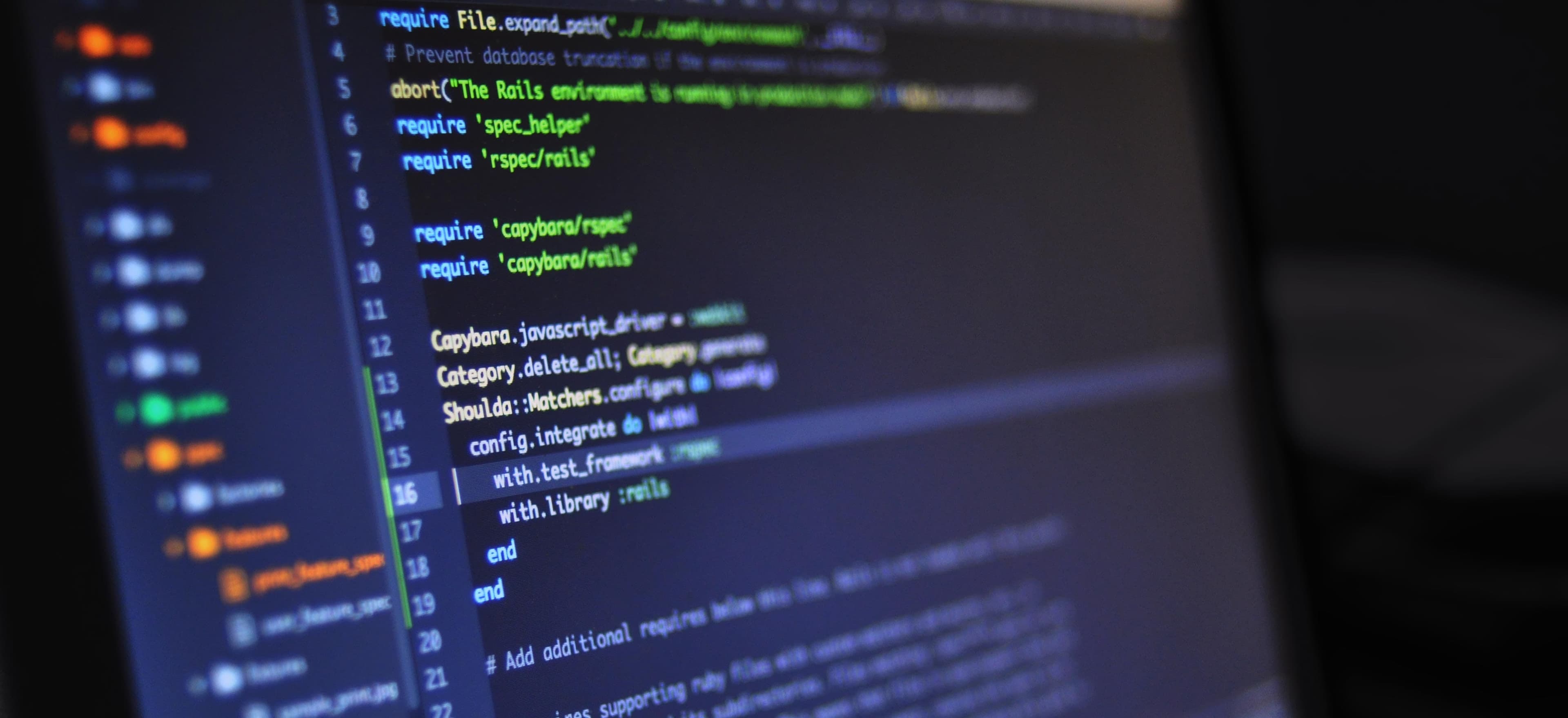
- Published on
Conquering Time Zone Challenges in Java with Joda-Time
When dealing with date and time in programming, one of the most formidable challenges is managing time zones. Handling time accurately is crucial in applications that rely on data from different geographic regions. This blog post explores how to effectively manage time zones in Java using the Joda-Time library, a powerful tool that simplifies date and time manipulation beyond the default capabilities of Java’s built-in libraries.
Understanding Time Zones in Java
Java's native date and time handling mechanics—specifically the java.util.Date
and java.util.Calendar
classes—have undergone criticism. These classes can be convoluted and lacking in intuitive support for time zones, which can lead to common pitfalls.
The Complexity of Time Zone Management
Why do time zones matter? Practically, if a user in New York schedules an event intended for a participant in Tokyo, the application must accurately calculate the time difference. Failing to do so can result in missed meetings or confusion about availability.
The Joda-Time library was created to address these shortcomings, letting developers effectively manage dates, times, and time zones.
Introducing Joda-Time
Joda-Time provides a cleaner API for date and time operations. As of Java 8, many of its features were incorporated into the new java.time
package. However, Joda-Time remains a robust option and still sees use in many projects.
Setting Up Joda-Time
To get started with Joda-Time, you need to add it as a dependency in your project. If you are using Maven, include the following snippet in your pom.xml
:
<dependency>
<groupId>joda-time</groupId>
<artifactId>joda-time</artifactId>
<version>2.10.10</version>
</dependency>
Basic Usage of Joda-Time
Joda-Time is intuitive and easy to use. Here's a simple example illustrating how to create instances of date and time and apply a time zone.
Creating DateTime Instances
Here’s how to create a DateTime
instance:
import org.joda.time.DateTime;
import org.joda.time.DateTimeZone;
public class JodaTimeExample {
public static void main(String[] args) {
// Get the current time in UTC
DateTime utcTime = new DateTime(DateTimeZone.UTC);
System.out.println("Current time in UTC: " + utcTime);
// Specify a time zone (e.g., Asia/Tokyo)
DateTime tokyoTime = utcTime.withZone(DateTimeZone.forID("Asia/Tokyo"));
System.out.println("Current time in Tokyo: " + tokyoTime);
}
}
Why Use Joda-Time?
In the above example:
- We initialize the current time in UTC, which provides a universal reference point.
- Next, we convert that time to Tokyo's local time using
.withZone()
.
This method ensures that daylight savings and other local time adjustments are accounted for automatically.
Handling Daylight Saving Time (DST)
Daylight Saving Time adds complexity to time calculations. With Joda-Time, you can seamlessly handle these transitions.
Example of DST Resolution
The following code snippet demonstrates how Joda-Time manages transitions:
import org.joda.time.DateTime;
import org.joda.time.DateTimeZone;
public class DaylightSavingTimeExample {
public static void main(String[] args) {
DateTime newYorkTime = new DateTime(DateTimeZone.forID("America/New_York"));
// Moving to a date during DST transition
DateTime dstStart = newYorkTime.withDate(2023, 3, 12).withTime(2, 0, 0, 0);
System.out.println("Time before DST starts: " + dstStart);
DateTime afterDstStart = dstStart.plusHours(1);
System.out.println("Time after adding 1 hour: " + afterDstStart);
}
}
Comments on DST Example
In this snippet:
- We create a
DateTime
object for March 12, 2023, at 2:00 AM in New York—a time just before DST starts. - When we add one hour, instead of moving to 3:00 AM, Joda-Time automatically jumps to 3:00 AM in the local time zone because DST initiates at 2:00 AM.
Joda-Time abstracts this complexity seamlessly, demonstrating its value in real-world applications.
Converting Between Time Zones
Joda-Time makes it straightforward to convert dates and times between different time zones.
Example of Converting Time Zones
import org.joda.time.DateTime;
import org.joda.time.DateTimeZone;
public class TimeZoneConversionExample {
public static void main(String[] args) {
DateTime newYorkTime = new DateTime(DateTimeZone.forID("America/New_York"));
System.out.println("New York time: " + newYorkTime);
// Convert to London time
DateTime londonTime = newYorkTime.withZone(DateTimeZone.forID("Europe/London"));
System.out.println("London time: " + londonTime);
}
}
Breakdown of Time Zone Conversion
In this example:
- A
DateTime
instance is created for New York. - We then convert this instance to London time using
.withZone()
, which again handles all necessary calculations, including any DST adjustments.
Additional Resources
For more intricate details on handling time zones and time-related operations, refer to the article titled Mastering Time Zone Issues in JavaScript Date Handling.
Best Practices When Dealing with Time Zones
-
Always Use UTC: Store dates and times in UTC to prevent confusion. Convert to local times only when displaying to users.
-
Leverage Libraries: Use Joda-Time or Java 8's
java.time
for their robust features and easier manipulation. -
Be Mindful of DST: Test time-related code during DST transitions.
-
Document Time Zones: Clearly document the time zones used in your applications to ease troubleshooting and user experience.
-
Validate User Input: Always validate and convert user input timestamps based on their provided time zones.
Closing the Chapter
Managing time zone challenges in Java doesn’t have to be daunting. The Joda-Time library empowers developers to handle dates and times with ease, accounting for the multifaceted intricacies of various time zones and DST. As you embed time functionalities into your applications, remember to use UTC as your foundation and transition thoughtfully to local times.
With powerful tools at your disposal, you can eliminate the headaches that often accompany date and time management. As always, keep testing, and if you ever find yourself stuck, consult resources like the one at Mastering Time Zone Issues in JavaScript Date Handling.
Happy coding!
Checkout our other articles