Tackling Java's Date and Time API for Accurate Time Zones
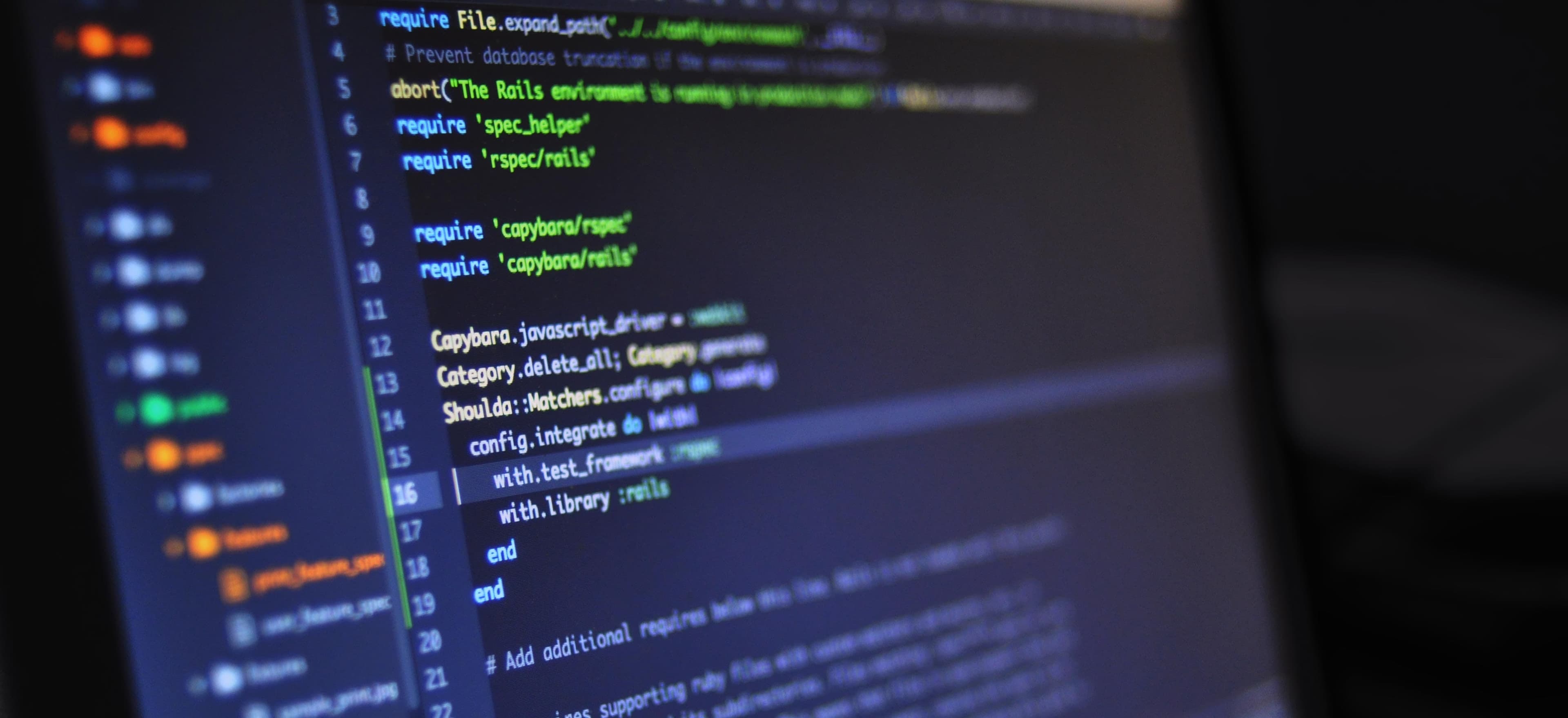
- Published on
Tackling Java's Date and Time API for Accurate Time Zones
When it comes to programming, managing date and time can be one of the trickiest tasks. Much like JavaScript, which has its own set of complexities around date handling, Java has its unique challenges. Therefore, understanding Java's Date and Time API is essential for any developer aiming to create applications that are globally-aware, considering different time zones.
Java's Date and Time API, introduced in Java 8 as part of the java.time
package, provides a robust framework for handling dates, times, and their associated complexities. In this post, we will explore the key components of the API, how to manage time zones effectively, and why it matters in your applications.
Why Time Zone Awareness Is Important
Time zones can significantly influence how users interact with applications. For example, if your application displays a meeting time in UTC but has users all over the world, you risk confusing your users. If you're interested in mastering date handling in JavaScript, check out this article that sheds light on similar complexities present in JavaScript.
The Basics of Java's Date and Time API
Java's Date and Time API is built around the following key classes:
- LocalDate: Represents a date without a time zone.
- LocalTime: Represents a time without a date or time zone.
- LocalDateTime: Combines both a date and a time but still does not include time zone information.
- ZonedDateTime: Represents a date and time with time zone information.
- Instant: Represents a moment on the timeline in UTC.
The Power of ZonedDateTime
One of the most essential classes when dealing with time zones is ZonedDateTime
. This class not only keeps track of local date and time but also includes time zone information, enabling accurate date-time conversions.
Here’s a simple code example that demonstrates how to create and manipulate a ZonedDateTime
object:
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class TimeZoneExample {
public static void main(String[] args) {
// Create a ZonedDateTime object for New York
ZonedDateTime newYorkTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
System.out.println("Current Time in New York: " + newYorkTime);
// Convert to Tokyo time
ZonedDateTime tokyoTime = newYorkTime.withZoneSameInstant(ZoneId.of("Asia/Tokyo"));
System.out.println("Corresponding Time in Tokyo: " + tokyoTime);
}
}
Commentary on the Code Snippet
-
Creating a ZonedDateTime Object: The method
ZonedDateTime.now(ZoneId.of("America/New_York"))
fetches the current date and time for New York. -
Converting Time Zones: By calling the
withZoneSameInstant
method, we can convert the New York date and time to Tokyo time without losing the exact moment in time.
This piece of code highlights how to quickly manipulate date and time through the API to cater to international use cases.
Local vs. UTC Time
When developing applications that have users across multiple time zones, deciding whether to store time in local time or UTC can have long-term implications. While local time is user-friendly, UTC is often used in backend systems due to its consistency and lack of daylight saving time changes.
To manage a UTC DateTime, you can use the Instant
class. Here's how you can convert a ZonedDateTime
to UTC:
import java.time.ZonedDateTime;
import java.time.Instant;
public class UTCExample {
public static void main(String[] args) {
// Create a ZonedDateTime object for Los Angeles
ZonedDateTime laTime = ZonedDateTime.now(ZoneId.of("America/Los_Angeles"));
System.out.println("Current Time in Los Angeles: " + laTime);
// Convert to an Instant (UTC)
Instant utcTime = laTime.toInstant();
System.out.println("Equivalent UTC Time: " + utcTime);
}
}
Why Use Instant?
-
Maintain Consistency: Using
Instant
ensures that you capture the exact moment in time without any ambiguity due to local time nuances. -
Easy Format Conversion: The flexibility of converting between
ZonedDateTime
andInstant
allows various systems interacting in different locales to be synchronized.
Handling Daylight Saving Time
Daylight saving time (DST) can complicate date-time calculations. Java’s Date and Time API takes care of DST transitions automatically, but it's essential to test and verify if your application's logic can handle these changes. Here is how you can work with DST:
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class DSTExample {
public static void main(String[] args) {
// Create a ZonedDateTime object for London
ZonedDateTime londonTime = ZonedDateTime.now(ZoneId.of("Europe/London"));
System.out.println("Current Time in London: " + londonTime);
// Show the time one day later
ZonedDateTime londonNextDay = londonTime.plusDays(1);
System.out.println("London Time Tomorrow: " + londonNextDay);
}
}
Key Takeaways
-
Automatic Adjustments: The API adjusts for DST when you manipulate dates, which mitigates common errors.
-
Planning Ahead: Understanding how to work with time zones and DST will reduce the chances of errors in critical date-time calculations.
Testing and Validation
Regardless of how robust the API is, testing your code is paramount. Tools like JUnit can be used to make sure your date and time calculations are correct. Here’s a brief example of how you might test a time zone conversion.
import static org.junit.jupiter.api.Assertions.assertEquals;
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class TimeZoneConversionTest {
@org.junit.jupiter.api.Test
public void testTimeZoneConversion() {
ZonedDateTime laTime = ZonedDateTime.now(ZoneId.of("America/Los_Angeles"));
ZonedDateTime utcTime = laTime.withZoneSameInstant(ZoneId.of("UTC"));
assertEquals(utcTime.getZone(), ZoneId.of("UTC"));
}
}
Final Considerations
Mastering Java's Date and Time API allows developers to create applications that can interactively deal with multiple time zones. This not only enhances user experience but also improves the reliability of applications in a global context.
For developers familiar with JavaScript's challenges around handling dates and times, understanding Java's API can smooth out similar curves. If you're interested in expanding your knowledge base in this area, consider checking out this resource for JavaScript as well.
In summary, Java’s Date and Time API provides a powerful toolkit for tackling the complexities of date and time management across different zones. Arm yourself with this knowledge, and you'll be one step closer to building globally-aware applications.
Checkout our other articles