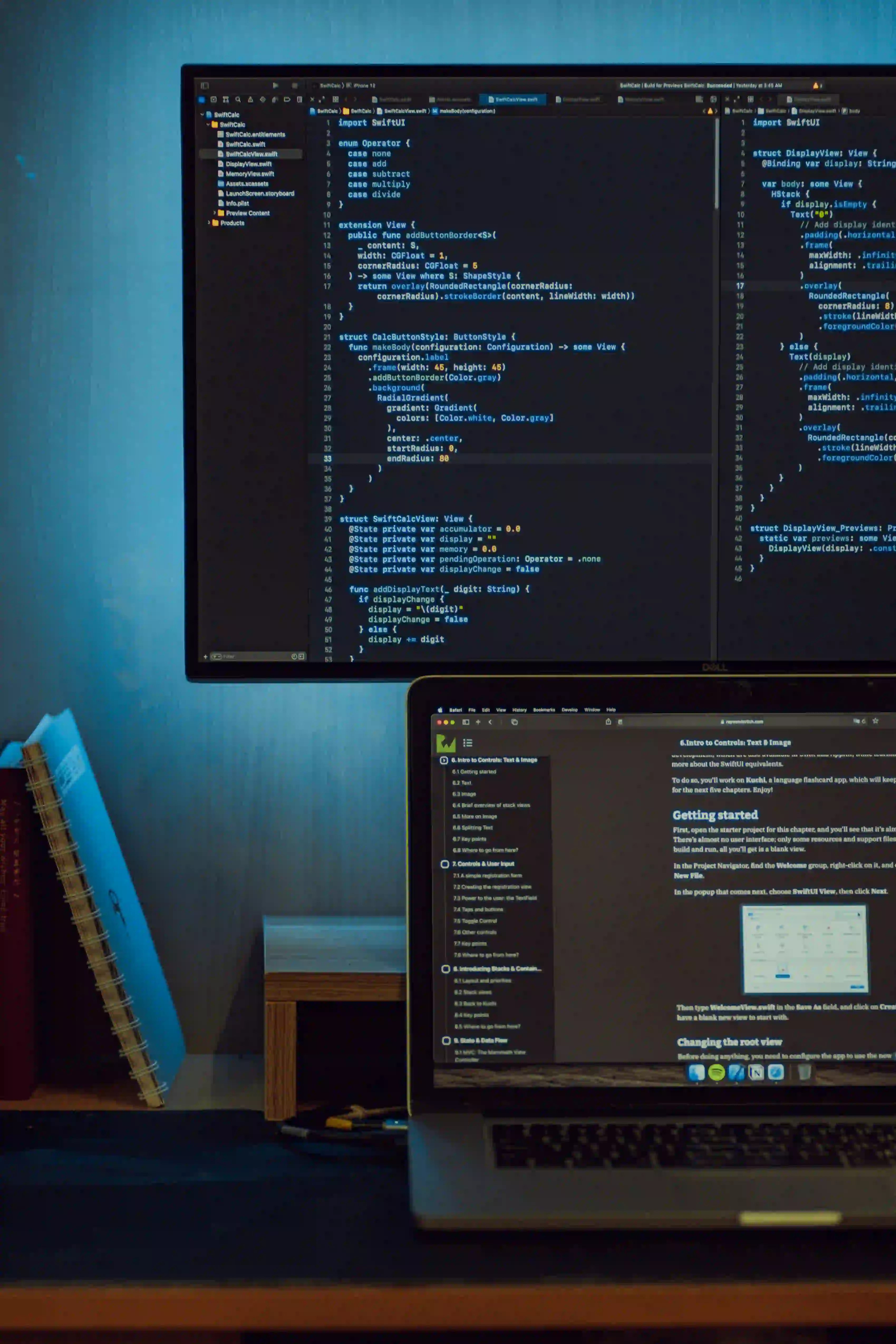
Effortless Integration of CSS Variables in Java Web Apps
In the era of modern web development, the importance of seamless integration between front-end and back-end frameworks is paramount. When building Java-based web applications, one often encounters challenges with styling. The use of CSS variables (also known as CSS custom properties) provides a robust solution to these challenges, allowing for easier maintenance, flexibility, and enhanced styling capabilities. In this blog post, we'll explore how to effectively integrate CSS variables into your Java web applications, enhancing your development process and ultimately improving the user experience.
What are CSS Variables?
CSS variables allow you to define reusable values that can be referenced throughout your CSS stylesheets. By declaring a variable in the :root
scope, you can ensure it's accessible throughout your application. Here’s a simple example:
:root {
--main-bg-color: #3498db;
--main-text-color: #ffffff;
}
body {
background-color: var(--main-bg-color);
color: var(--main-text-color);
}
Why Use CSS Variables?
- Reusability: They promote a more DRY (Don't Repeat Yourself) coding style.
- Dynamic Updates: You can change the value of CSS variables with JavaScript, allowing for dynamic styling based on user interactions.
- Maintenance: If you wish to change a color scheme or layout, you can update the variable in one place rather than hunting through multiple stylesheets.
Integrating CSS Variables in Java Web Apps
Integrating CSS variables into your Java web applications requires a few considerations for both your back-end logic and front-end layouts. Let’s break it down step-by-step.
Step 1: Define CSS Variables in Your Styles
Begin by defining your CSS variables in your main stylesheet. Here's an initial setup for a Java web app:
/* styles.css */
:root {
--primary-color: #4CAF50;
--secondary-color: #FF5722;
--font-family: 'Arial, sans-serif';
}
body {
font-family: var(--font-family);
background-color: var(--primary-color);
color: var(--secondary-color);
}
Putting these variables in a central file makes it easier to manage and tweak your styling as needed.
Step 2: Dynamically Change CSS Variables using JavaScript
You may also want to provide users with the ability to change themes or styles dynamically. Here’s how:
// script.js
function changeTheme(primary, secondary) {
document.documentElement.style.setProperty('--primary-color', primary);
document.documentElement.style.setProperty('--secondary-color', secondary);
}
// Example usage: Change to dark theme
changeTheme('#333', '#FFF');
This JavaScript function allows on-the-fly changes to your CSS variables, enhancing user experience without the need for a full page refresh.
Step 3: Delivering CSS Variables from Java to the Front-End
To effectively use CSS variables, you might want to configure some values dynamically from the Java side. For instance, you can pass values through objects that are rendered on the JSP or Thymeleaf templates.
For example, you might have a Theme
model in Java:
public class Theme {
private String primaryColor;
private String secondaryColor;
// Constructors, getters, setters
}
And in your controller, you populate this theme:
@Controller
public class ThemeController {
@GetMapping("/theme")
public String getTheme(Model model) {
Theme theme = new Theme("#4CAF50", "#FF5722");
model.addAttribute("theme", theme);
return "index";
}
}
And on the JSP side, you can integrate the theme’s colors directly into your CSS variables:
<style>
:root {
--primary-color: ${theme.primaryColor};
--secondary-color: ${theme.secondaryColor};
}
</style>
This method binds your Java back-end values directly into your front-end styles, providing flexibility based on user or application settings.
Enhancing User Interaction with CSS Variables
Incorporating CSS variables not only streamlines development but also enhances user engagement. With tools such as sliders or dropdowns, users can modify the application’s appearance. This is where integrating your variables directly into Java web applications becomes advantageous.
Here’s an example of a simple color picker to adjust the theme:
<input type="color" id="primaryColor" onchange="changeTheme(this.value, '#FFF')">
As users select colors, your JavaScript will call the changeTheme
method with the new primary color, coupled with a predefined secondary color.
Case Study: Streamlining Your Web Development
For real-world applications, it’s beneficial to gain insights from existing articles covering common challenges. A relevant piece titled Streamlining Web Dev: How to Effortlessly Generate CSS & JS Variables discusses generating variables across your project effectively. While the article provides a different perspective, it can be beneficial for integrating CSS and JS comprehensively.
In Conclusion, Here is What Matters
Integrating CSS variables within Java web applications opens new avenues for customization, maintainability, and user interface responsiveness. By defining your styles centrally, introducing dynamic updates via JavaScript, and managing theme settings through your back-end logic, you can significantly alleviate common constraints in web development.
To summarize:
- Define CSS Variables: Centralize style management.
- Dynamic Updates: Enhance interactivity for users.
- Back-End Integration: Leverage your Java logic for styling.
As you continue to develop your Java web applications, consider adopting CSS variables as a standard practice. This will promote cleaner code, a better user experience, and ultimately make your web application a standout in today’s competitive landscape.
Recommended Next Steps
- Explore more about CSS variables and their properties by visiting MDN Web Docs.
- Experiment with dynamic theming in your projects, and don’t hesitate to iterate.
Happy coding!