Optimizing Java for Chemical Computations: Tips You Need
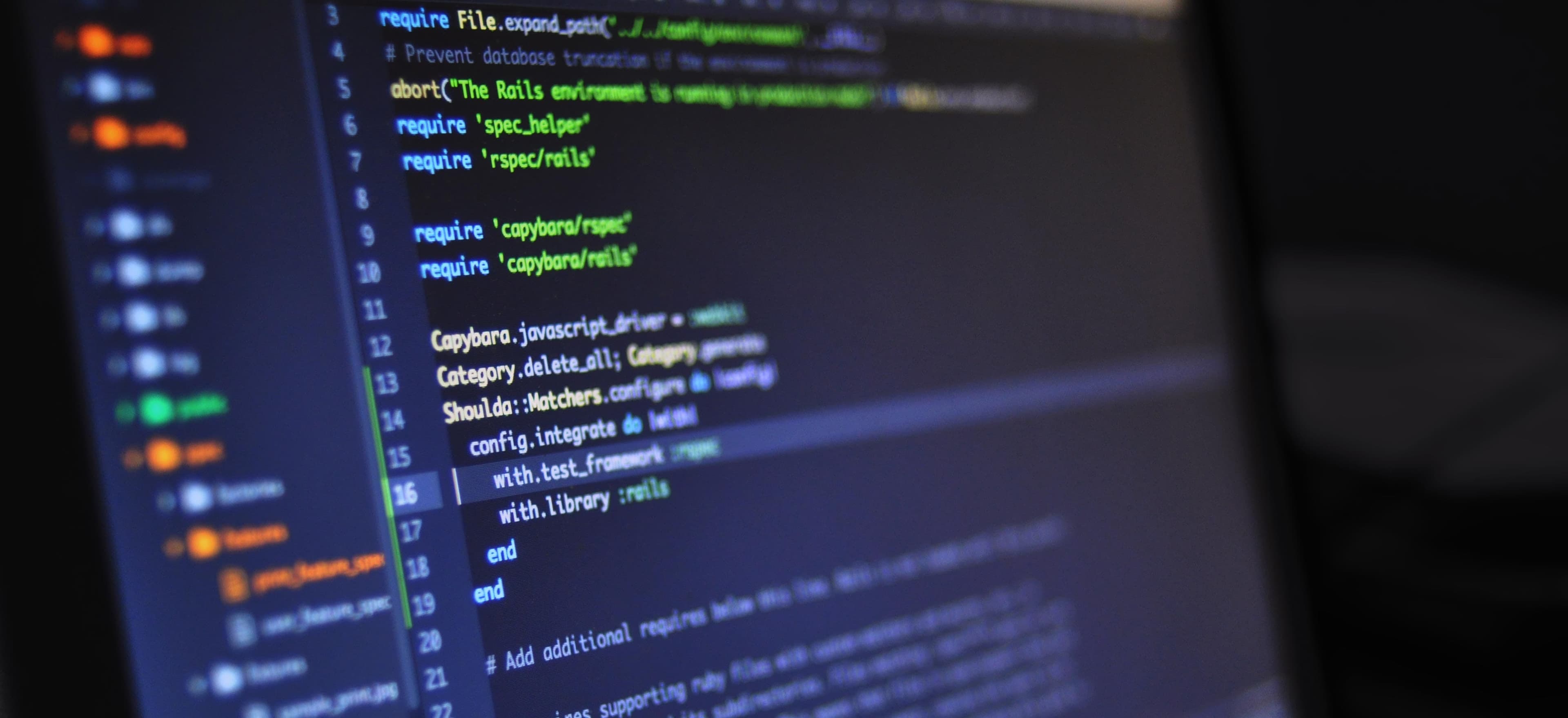
- Published on
Optimizing Java for Chemical Computations: Tips You Need
Java is a popular programming language that offers a rich set of features for building applications in various domains, including scientific computing. When it comes to chemical computations, precise algorithms and efficient data handling are crucial. In this post, we will explore how to optimize Java for handling chemical calculations effectively.
Understanding the Basics
To set the stage, let's clarify what we mean by chemical computations. These calculations often involve complex mathematical models such as molecular dynamics, quantum mechanics, and statistical mechanics. Optimizing your Java code for these tasks is essential for performance, especially when dealing with large datasets or intricate simulations.
For a more general approach to chemical calculations, you might want to explore the article titled "Chemische Berechnungen leicht gemacht: Tipps & Tricks", which covers various strategies to facilitate these calculations.
Efficient Data Structures
One of the first ways to optimize your Java application for chemical computations is by choosing the right data structures. Let's consider the data we typically deal with in this domain.
Choosing the Right Collections
Java Collections Framework offers a variety of data structures. For example, if you need to frequently retrieve and insert elements based on specific criteria, using a HashMap
can be beneficial due to its O(1) time complexity for get and put operations.
Here’s a simple illustration:
import java.util.HashMap;
public class ChemicalElements {
private HashMap<String, Integer> elements;
public ChemicalElements() {
elements = new HashMap<>();
elements.put("H", 1);
elements.put("He", 2);
elements.put("Li", 3);
// Add more elements
}
public Integer getAtomicNumber(String symbol) {
return elements.get(symbol);
}
}
Explanation
In this code snippet, we define a ChemicalElements
class that encapsulates the atomic numbers of chemical elements. Using a HashMap
allows for quick retrieval of atomic numbers based on element symbols, which can be frequently needed in various computations.
Multithreading
Chemical computations can benefit significantly from parallel processing. Java's built-in multithreading capabilities allow you to divide computational tasks across multiple threads, taking full advantage of modern multi-core processors.
Using Executors
Here's an example of how to use Java's Executor framework for parallelizing calculations:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ParallelCalculation {
private static final int THREADS = 4;
public void calculate() {
ExecutorService executor = Executors.newFixedThreadPool(THREADS);
for (int i = 0; i < 10; i++) {
final int taskNumber = i;
executor.submit(() -> performCalculation(taskNumber));
}
executor.shutdown();
}
private void performCalculation(int taskNumber) {
// Simulate heavy computation
System.out.println("Calculating for task: " + taskNumber);
try {
Thread.sleep(1000); // Simulate time-consuming task
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Explanation
In this code, we create a pool of threads to handle multiple tasks concurrently. The calculate
method submits tasks that perform potentially heavy computations. This parallelization can significantly reduce the total execution time, making your chemical calculations run faster.
Optimization Techniques
Profiling Your Code
Before optimizing, it’s essential to identify bottlenecks in your code. Java provides tools like VisualVM and Java Mission Control that allow you to profile your application. By identifying methods that consume the most CPU time, you can focus your optimization efforts where they will have the most impact.
Effective Memory Management
Memory management is another critical aspect when optimizing Java for heavy computations. Take special care with object creation—over-creating objects can lead to garbage collection overhead.
Example: Using Static Methods
Often, it is advantageous to use static methods for utility functions that don’t need object state. This reduces the need for object creation.
public class MathUtilities {
public static double calculateMolarMass(double[] atomicWeights) {
double total = 0.0;
for (double weight : atomicWeights) {
total += weight;
}
return total;
}
}
Explanation
In this example, the calculateMolarMass
method takes an array of atomic weights and calculates the total without creating an instance of MathUtilities
. Such simple utility functions enhance performance by minimizing memory overhead.
Using Appropriate Libraries
Leveraging well-designed libraries can drastically improve your efficiency. Libraries such as Apache Commons Math and JScience provide robust implementations of scientific algorithms.
Example Using JScience
import org.jscience.physics.amount.Amount;
import javax.measure.unit.SI;
public class PressureCalculator {
public Amount<Pressure> calculatePressure(double force, double area) {
return Amount.valueOf(force / area, SI.PASCAL);
}
}
Explanation
Here, we perform pressure calculations using the JScience library, which provides unit support and avoids potential pitfalls of unit conversions in manual calculations. Using established libraries not only saves time but can also enhance code reliability.
Final Considerations
Optimizing Java for chemical computations involves strategic decisions regarding data structures, multithreading practices, code profiling, and library usage. By understanding these elements and applying them effectively, you can significantly enhance the performance of your Java applications in the realm of chemical sciences.
For further reading, consider checking out the article "Chemische Berechnungen leicht gemacht: Tipps & Tricks" for additional insights into chemical computations.
Remember, optimization is often a balance. Strive to maintain code readability while pursuing performance improvements. By applying these tips, you will be well on your way to creating efficient, robust Java applications for chemical computations.