Optimizing Java Applications for Chemical Calculation Efficiency
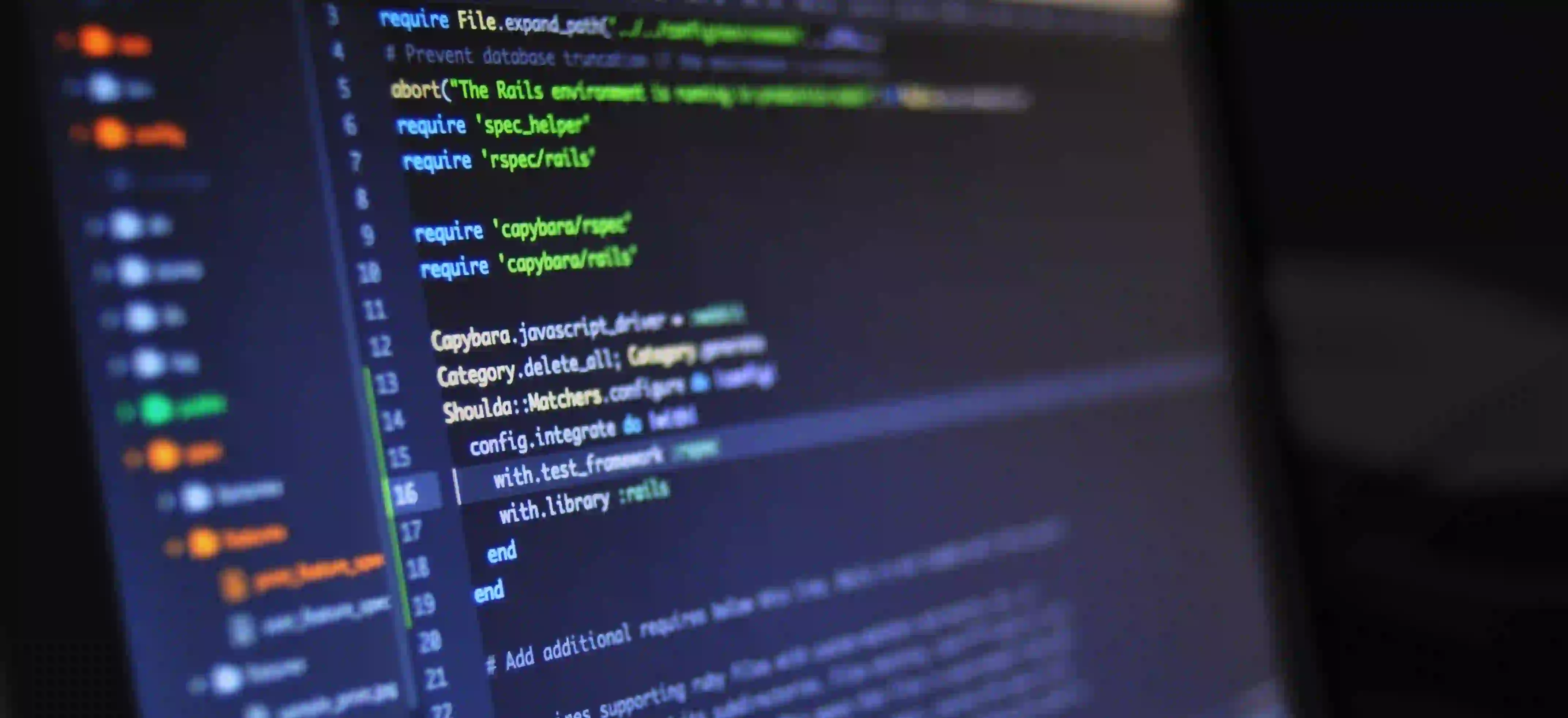
Optimizing Java Applications for Chemical Calculation Efficiency
In today's fast-paced world of scientific research and development, the efficiency of software applications plays a crucial role in expediting processes and enhancing overall productivity. This is particularly true in fields like chemistry, where complex calculations and simulations are frequent. In this blog post, we will explore techniques for optimizing Java applications that handle chemical calculations, ensuring not just functionality but also efficiency.
Why Optimize?
Before delving into the specifics, it is vital to understand why optimization matters. Larger datasets, intricate calculations, and the need for rapid results render optimization a necessity. An optimized application can significantly reduce execution times, leading to faster iterations and improved user experience.
For example, if you're working on chemical calculations, an optimized application means less time waiting for results and more time analyzing data. This aligns with practices outlined in the article Chemische Berechnungen leicht gemacht: Tipps & Tricks.
1. Choosing the Right Data Structures
Data structures are the foundation of any application. In the realm of chemical computations, selecting appropriate data types can lead to performance improvements.
Example Code Snippet
import java.util.HashMap;
public class ChemicalCompound {
private String name;
private HashMap<String, Integer> elements;
public ChemicalCompound(String name) {
this.name = name;
this.elements = new HashMap<>();
}
public void addElement(String element, int count) {
elements.put(element, count);
}
// Additional methods for calculations...
}
Commentary
Here, we utilize a HashMap
to store elements and their respective quantities in a chemical compound. This structure allows for quick access and modification, optimizing performance when dealing with multiple elements in compounds.
When to Use Different Structures
- ArrayList for ordered collections when direct access is needed.
- HashMap for key-value pairs where fast lookups are essential.
- LinkedList when frequent modifications (insertions/deletions) are required.
The choice of data structure directly impacts the efficiency of our calculations.
2. Implementing Multithreading
Java supports multithreading, which is instrumental in optimizing applications that require extensive calculations. By running multiple threads, we can perform calculations simultaneously, thereby reducing overall execution time.
Example Code Snippet
class CalculationTask implements Runnable {
private String formula;
public CalculationTask(String formula) {
this.formula = formula;
}
@Override
public void run() {
// Perform chemical calculations here
System.out.println("Calculating: " + formula);
}
}
// In the main application
public void executeCalculations(List<String> formulas) {
List<Thread> threads = new ArrayList<>();
for (String formula : formulas) {
Thread thread = new Thread(new CalculationTask(formula));
threads.add(thread);
thread.start();
}
// Join threads
for (Thread thread : threads) {
try {
thread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Commentary
In this code, we define a CalculationTask
class that implements Runnable
. Each task can be executed on a separate thread, allowing calculations to run concurrently. This approach is particularly beneficial when handling large datasets or multiple formulas, significantly speeding up the process.
3. Utilizing Libraries and Frameworks
While building applications from scratch is educative, leveraging existing libraries can save time and harness optimized algorithms. Libraries like JScience or Apache Commons Math offer robust tools for scientific computations.
Example Code Snippet with JScience
import org.jscience.mathematics.function.Complex;
public class ComplexCalculation {
public static void main(String[] args) {
Complex a = new Complex(2, 3); // 2 + 3i
Complex b = new Complex(1, 4); // 1 + 4i
Complex result = a.add(b);
System.out.println("The result is: " + result);
}
}
Commentary
Using libraries such as JScience allows developers to perform complex mathematical calculations simply and effectively. It streamlines the development process and reduces the likelihood of errors compared to writing complex algorithms from scratch.
4. Profiling and Benchmarking Your Application
Once you've implemented optimizations, profiling your application helps determine where you stand. Tools such as VisualVM, YourKit, and JProfiler allow developers to monitor memory usage, CPU utilization, and thread activity.
Example Benchmarking Code
import java.util.ArrayList;
public class Benchmark {
public static void main(String[] args) {
long startTime = System.nanoTime();
executeCalculations(generateTestFormulas());
long endTime = System.nanoTime();
System.out.println("Execution Time: " + (endTime - startTime) + " nanoseconds");
}
private static List<String> generateTestFormulas() {
List<String> formulas = new ArrayList<>();
// Generating dummy formulas for testing
for (int i = 0; i < 1000; i++) {
formulas.add("H2O" + i);
}
return formulas;
}
}
Commentary
In this example, time taken for execution is measured. Benchmarking is essential. By profiling, you'll identify bottlenecks and focus on sections of your code that require further optimization.
5. Consideration of JVM Optimizations
Java is known for its ability to optimize code on the fly. Nonetheless, understanding JVM tuning options can lead to significant improvements in application performance. Tweaking the heap size, garbage collection settings, and other parameters can optimize how Java handles memory and processing.
Example JVM Options
To specify heap size, you can use the following options when starting your Java application:
java -Xms512m -Xmx2048m -jar YourApp.jar
Commentary
Setting initial and maximum heap sizes can prevent frequent garbage collections that can slow down your application. Understanding these settings provides an added layer of optimization.
To Wrap Things Up
Optimizing Java applications for chemical calculation efficiency is not a one-size-fits-all approach. It requires a combination of appropriate data structures, processes like multithreading, reliance on powerful libraries, thorough profiling, and JVM optimizations.
Incorporating these techniques will nudge your applications toward improved performance. As outlined in the article Chemische Berechnungen leicht gemacht: Tipps & Tricks, practical approaches can lead to tangible enhancements in efficiency.
By continuously monitoring, profiling, and refining your application, you'll not only improve its performance but also vastly enhance the user experience. In the ever-evolving field of chemistry and computational analysis, efficiency is your ally — so use it wisely!