Mastering Java Integration for Dynamic CSS & JS Variable Generation
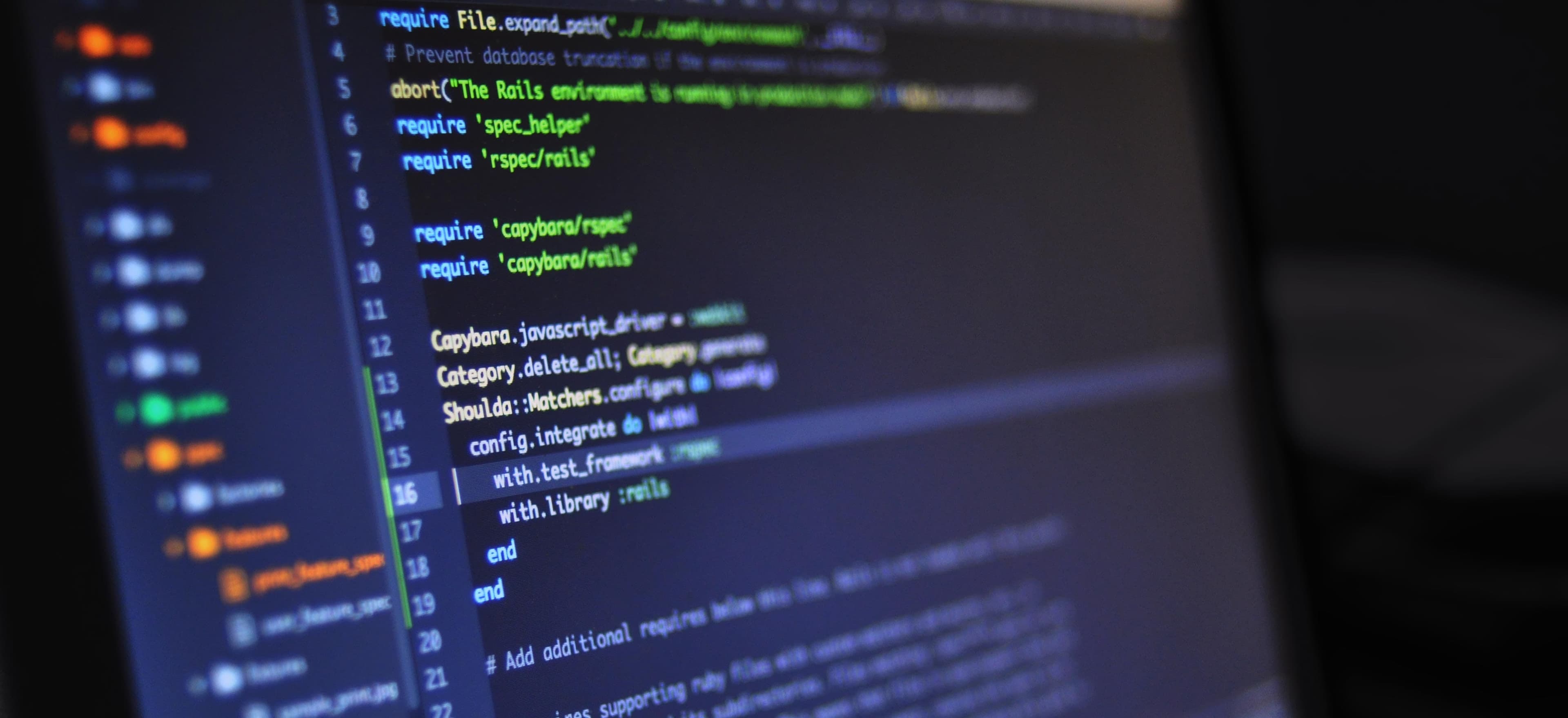
- Published on
Mastering Java Integration for Dynamic CSS & JS Variable Generation
In today's technologically advanced landscape, the intersection between Java and web development has become increasingly vital. With the growing emphasis on responsive and dynamic web applications, the ability to generate CSS and JavaScript variables dynamically—using Java—has become a sought-after skill. In this blog post, we will explore the nuances of integrating Java for generating CSS and JS variables effectively, ensuring that your web applications are not only functional but aesthetically pleasing and adaptable.
Table of Contents
- Understanding Dynamic CSS and JS Variables
- Benefits of Using Java for Variable Generation
- Java Basics for Web Development
- Generating CSS Variables with Java
- Creating Dynamic JS Variables with Java
- Case Study: A Practical Implementation
- Conclusion
Understanding Dynamic CSS and JS Variables
Dynamic CSS and JS variables allow developers to adjust styles and behaviors without refreshing the web page. This flexibility enhances user experiences and optimizes performance.
CSS variables (also known as custom properties) are defined within the CSS and can be manipulated using JavaScript. Using Java to generate these variables adds an additional layer of flexibility, allowing for real-time updates based on user interactions or other conditions.
Benefits of Using Java for Variable Generation
Using Java for generating CSS and JavaScript variables has several advantages:
- Robustness: Java is known for its strong typing and structure, resulting in fewer runtime errors.
- Scalability: Java applications can handle larger datasets and user loads more efficiently.
- Integration: Smooth integration with various frameworks, enabling CSS and JS variable generation from server-side operations.
Java Basics for Web Development
Before diving into variable generation, it's crucial to have a solid foundation in Java and its web technologies. Java-based web frameworks, such as Spring and Hibernate, are widely used for building dynamic web applications. Understanding the core concepts like Servlets and JSP (JavaServer Pages) can be beneficial, as they are often utilized in rendering HTML and manipulating CSS/JS content.
// Example of a simple Java Servlet
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
public class DynamicVariablesServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Set response content type
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h2>Dynamic CSS and JS Variables</h2>");
// Closing the HTML response
out.println("</body></html>");
}
}
In this snippet, we define a simple Java Servlet that responds to GET
requests by rendering a basic HTML structure. This forms the foundation for adding dynamic capabilities later.
Generating CSS Variables with Java
To generate CSS variables dynamically, we can store styles in a Java class and write them as inline CSS in the response:
public class CssVariableGenerator {
public String getDynamicCss() {
String primaryColor = "#3498db"; // Example dynamic value.
return ":root { --primary-color: " + primaryColor + "; }";
}
}
The getDynamicCss
method constructs a CSS variable and returns it as a string. It can be included directly in the HTML response of our servlet.
Including CSS in Servlet Response
Combine the generated CSS with your servlet response:
out.println("<style>" + new CssVariableGenerator().getDynamicCss() + "</style>");
This line inserts our generated CSS variable directly into the HTML, making it available on the client-side to use in styles.
Creating Dynamic JS Variables with Java
Similar to CSS, Java can also be used to generate JavaScript variables dynamically. The objective is to create variables that can be manipulated on the front end.
Consider the following method that generates a JavaScript variable:
public class JsVariableGenerator {
public String getDynamicJs() {
String apiEndpoint = "/api/data"; // Dynamic endpoint
return "const apiEndpoint = '" + apiEndpoint + "';";
}
}
Incorporating the JS into Servlet Output
Just like we incorporated CSS, we can include the generated JavaScript in our servlet's response:
out.println("<script>" + new JsVariableGenerator().getDynamicJs() + "</script>");
Case Study: A Practical Implementation
To showcase the benefits of Java integration in generating CSS and JS variables, consider a simple web application that updates styles based on user preferences, such as changing themes.
Step 1: User Input
Collect user input through a form using an HTML structure:
<form action="/updateStyles" method="POST">
<label for="color">Choose a primary color:</label>
<input type="color" id="color" name="color">
<input type="submit" value="Apply">
</form>
Step 2: Handling Input in Java Servlet
In the servlet handling the form submission, we can extract the user-defined color and use it to generate new CSS and JS variables dynamically:
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String userColor = request.getParameter("color");
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
// Generate dynamic CSS using user-defined color
CssVariableGenerator generator = new CssVariableGenerator();
out.println("<style>:root { --primary-color: " + userColor + "; }</style>");
// Example of using generated JS variable
String apiEndpoint = "/api/update?color=" + userColor;
out.println("<script>const apiEndpoint = '" + apiEndpoint + "';</script>");
out.println("<h2>Styles Updated</h2>");
out.println("</body></html>");
}
Step 3: Updating Page with New Styles
You can utilize the generated variables to dynamically change styles or behaviors on the web page:
document.body.style.backgroundColor = getComputedStyle(document.documentElement)
.getPropertyValue('--primary-color');
In Conclusion, Here is What Matters
Integrating Java for generating dynamic CSS and JS variables presents a powerful approach to modern web development. This method facilitates real-time customization, enhances user experiences, and bridges server-side logic with client-side dynamic behavior.
Incorporating Java into your web development toolkit, along with best practices outlined in Streamlining Web Dev: How to Effortlessly Generate CSS & JS Variables, allows you to build responsive, interactive applications that adapt to user interactions seamlessly.
By mastering these integrations, you will elevate your development skills and create web applications that meet and exceed user expectations in our dynamic digital world. Happy coding!
Checkout our other articles