Optimizing Java Algorithms for Economic Data Analysis
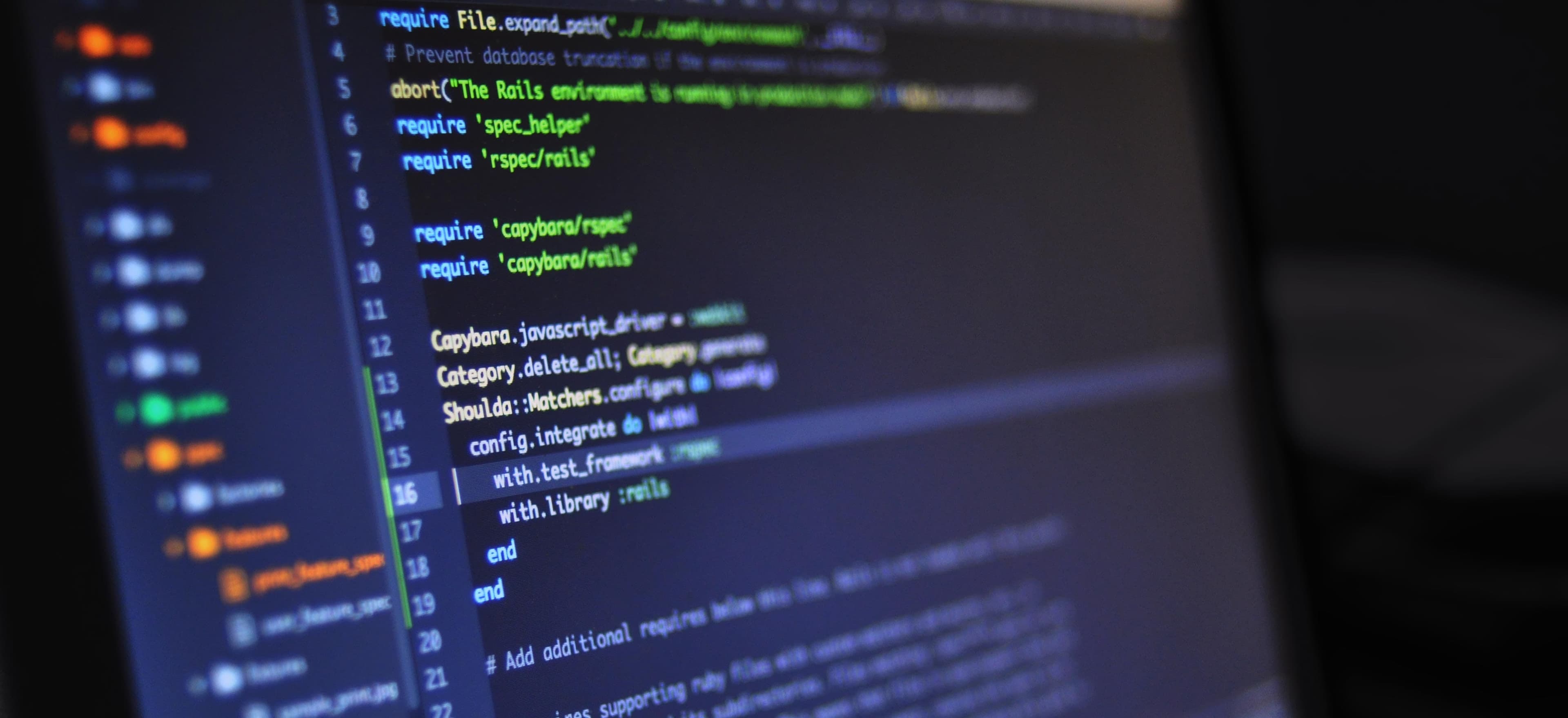
- Published on
Optimizing Java Algorithms for Economic Data Analysis
In today's data-driven world, economic data analysis is becoming increasingly important. Businesses rely heavily on data to make informed decisions. Java, a robust programming language, provides excellent capabilities for handling large datasets and performing complex calculations. This blog post aims to discuss how to optimize Java algorithms for economic data analysis while adhering to programming best practices.
Understanding Economic Data Analysis
Before diving into optimization strategies, it's essential to understand what economic data analysis entails. It involves interpreting and analyzing data to extract meaningful insights related to economic activities. This could be anything from analyzing consumer behavior to predicting market trends. For a deeper dive into the mathematical foundation behind economic models, refer to the article "Mathematik in der Wirtschaft: Ein Schlüssel zum Erfolg" at lernflux.com/post/mathematik-wirtschaft-erfolg.
The Role of Java in Economic Data Analysis
Java is favored in data analysis for its performance, portability, and ease of use. Libraries such as Apache Commons Math and JFreeChart provide additional functionality for mathematical operations and data visualization respectively.
Key Optimization Techniques
Tuning Java algorithms requires a solid understanding of both the Java language and the economic concepts involved. Here we explore several key optimization techniques.
1. Efficient Data Structures
Choosing the right data structure is vital. In economic data analysis, you often deal with time-series data, financial transactions, or user behavior logs.
Using HashMaps for Lookups:
import java.util.HashMap;
public class EconomicData {
private HashMap<String, Double> consumerSpending;
public EconomicData() {
consumerSpending = new HashMap<>();
}
public void addData(String category, Double amount) {
consumerSpending.put(category, amount);
}
public Double getData(String category) {
return consumerSpending.get(category);
}
}
Why: HashMaps provide O(1) time complexity for lookups, which is much faster than using an ArrayList with O(n) complexity for searches.
2. Parallel Processing
Java's concurrency utilities can be leveraged to perform data analysis tasks in parallel, making your application more efficient, especially for large datasets.
Using Java's Streams for Parallel Processing:
import java.util.Arrays;
import java.util.List;
public class EconomicAnalysis {
public double analyze(List<Double> data) {
return data.parallelStream()
.mapToDouble(Double::doubleValue)
.average()
.orElse(0);
}
}
Why: Utilizing parallel streams allows multiple threads to process data concurrently, significantly reducing computation time.
3. Lazy Loading
If you're dealing with massive datasets, consider implementing lazy loading to avoid unnecessary resource consumption. Only load the data when required.
Example:
public class LazyLoader {
private List<String> data;
public List<String> getData() {
if (data == null) {
loadData();
}
return data;
}
private void loadData() {
// Simulate data loading
data = Arrays.asList("Data1", "Data2", "Data3");
}
}
Why: This approach minimizes memory usage while keeping the performance intact by delaying resource-intensive operations.
4. Avoiding Unnecessary Computations
Optimize your algorithms by avoiding repetitive calculations. You can use memoization or caching techniques to store already computed results.
Example of Memoization:
import java.util.HashMap;
public class FibonacciCalculator {
private HashMap<Integer, Long> memo = new HashMap<>();
public long fibonacci(int n) {
if (n <= 1) return n;
return memo.computeIfAbsent(n, key -> fibonacci(key - 1) + fibonacci(key - 2));
}
}
Why: By storing previously computed results, you can drastically reduce the number of calculations needed, especially for recursive algorithms.
5. Utilizing Effective Libraries
Java has a wealth of libraries that can boost efficiency. Libraries like Apache Commons Math provide optimized algorithms for mathematical computations.
Example Usage:
import org.apache.commons.math3.stat.descriptive.DescriptiveStatistics;
public class StatisticsCalculator {
public double[] computeStatistics(double[] data) {
DescriptiveStatistics stats = new DescriptiveStatistics();
for (double d : data) {
stats.addValue(d);
}
return new double[]{stats.getMean(), stats.getStandardDeviation()};
}
}
Why: Using well-tested libraries can save you time and often offer more optimized performance compared to home-grown solutions.
Best Practices for Writing Optimized Java Code
Code Readability
Make your code readable with meaningful variable names, consistent naming conventions, and properly structured code blocks. This practice aids collaboration and maintenance.
Performance Profiling
Always profile your code before optimization. Use tools like VisualVM or JProfiler to identify bottlenecks and assess where changes will have the most significant impact.
Regular Updates
Stay updated with the latest versions of Java. New releases often come with performance improvements and enhanced features.
Documentation
Document your code thoroughly. This not only helps others understand your work but also allows you to revisit your code in the future without confusion.
Key Takeaways
Optimizing Java algorithms for economic data analysis is a multifaceted process that requires a blend of strategy, technique, and an understanding of both Java and economic principles. By selecting the right data structures, leveraging parallel processing, caching results, and using effective libraries, you can build powerful applications that perform efficiently and provide significant insights into economic data.
Remember, continual improvement is key. As datasets grow and evolve, so should your optimization techniques. For a more profound understanding of mathematical concepts that underpin economic data analysis, consider reading "Mathematik in der Wirtschaft: Ein Schlüssel zum Erfolg" at lernflux.com/post/mathematik-wirtschaft-erfolg.
By applying these best practices and techniques, you can ensure that your Java applications are not only efficient but also scalable and maintainable. Happy coding!