Unpacking the Java Framework: Lessons from Historical Impacts
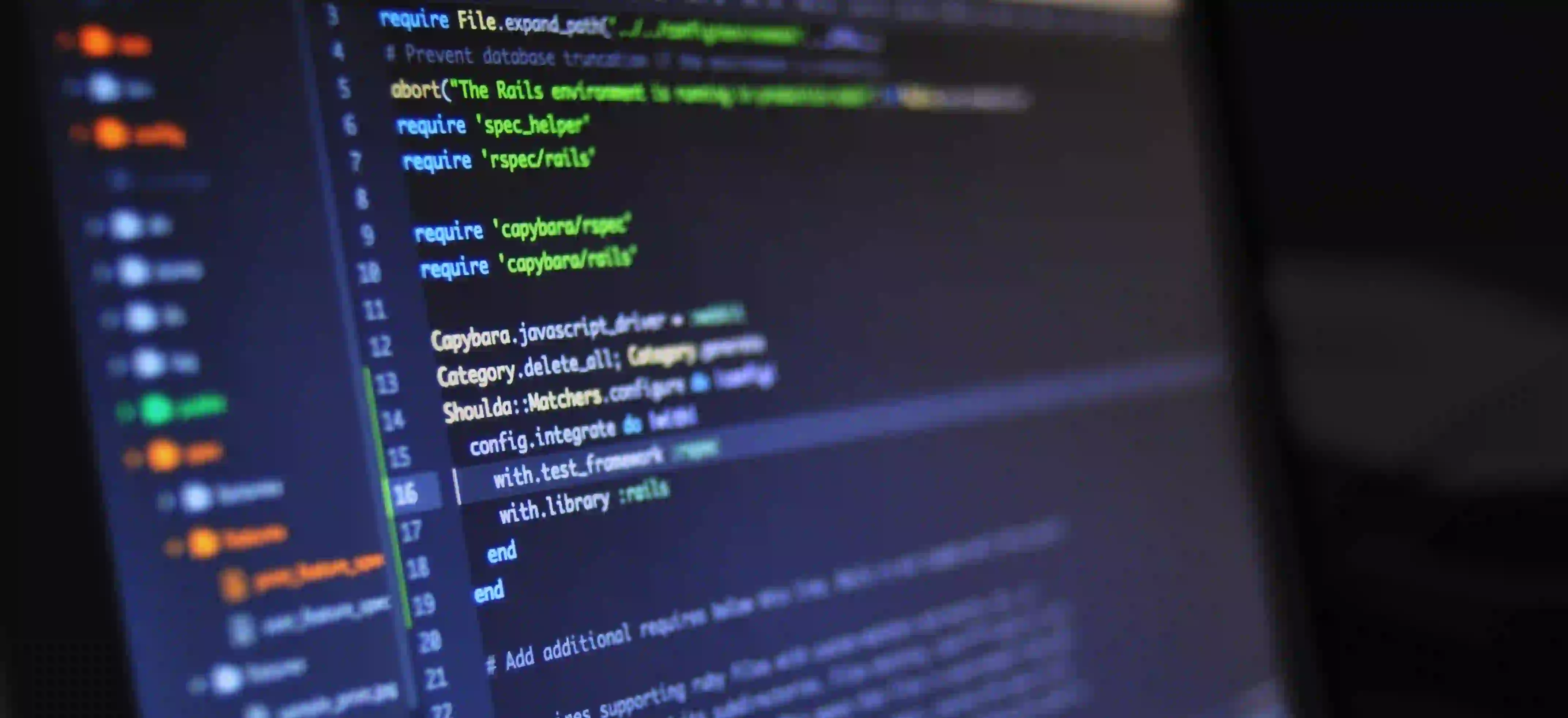
Unpacking the Java Framework: Lessons from Historical Impacts
In a world where technology evolves at breakneck speed, understanding programming languages like Java is crucial for developers. Not only is Java a versatile and powerful language, but its historical context can also provide valuable insights into its design and impact on the programming world. Let’s explore the nuances of Java, its framework, and draw intriguing parallels from historical events such as the Vertrag von Versailles. For a deeper insight into global controversies shaped by historical decisions, you can check out the article Vertrag von Versailles: Ursachen der globalen Kontroverse.
The Java Ecosystem
Java, created in 1995, is a language that embraces the principles of object-oriented programming. Its design emphasizes reusability and simplicity, appealing to developers looking to create scalable applications. Within Java's ecosystem, numerous frameworks have emerged, each with its own specialties and advantages. For example:
- Spring: A comprehensive framework that facilitates enterprise-level applications.
- Hibernate: A tool for simplifying database interactions.
- JavaServer Faces (JSF): A framework for building user interfaces for web applications.
These frameworks allow developers to leverage existing solutions, expediting the development process and enhancing code maintainability.
The Foundations of Java: Object-Oriented Design
The core tenets of Java revolve around object-oriented design (OOD). OOD is essential in programming as it helps in structuring complex applications into manageable components. Here is a basic example in Java showcasing the principles of OOD:
class Animal {
String name;
public Animal(String name) {
this.name = name;
}
public void makeSound() {
System.out.println(name + " makes a sound");
}
}
class Dog extends Animal {
public Dog(String name) {
super(name);
}
@Override
public void makeSound() {
System.out.println(name + " barks");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog("Buddy");
myDog.makeSound();
}
}
Why Objects Matter
This code snippet demonstrates the importance of creating a base class (Animal
) from which other classes (Dog
) can inherit. This promotes code reusability and organization — crucial for large-scale applications. In a sense, the approach reflects how historical frameworks or policies can have cascading effects, much like how decisions taken post-World War I, such as the Vertrag von Versailles, affected subsequent global dynamics.
Frameworks: Boosting Productivity
Frameworks not only speed up development but also standardize coding practices. By using Spring Boot, for instance, developers can simplify configuration and create stand-alone applications. Below is an example of a simple Spring Boot application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
The Power of Convention Over Configuration
Spring Boot uses a philosophy known as "convention over configuration", allowing developers to focus on building features rather than managing boilerplate code. The philosophy resonates similarly with the aftermath of Vertrag von Versailles, where certain agreements shaped future strategies aimed at preventing conflict.
Understanding Dependency Injection in Spring
A critical feature of the Spring framework is dependency injection (DI). DI enhances modularity and makes testing easier by decoupling components. The following shows dependency injection in action:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
class Service {
public void serve() {
System.out.println("Service is serving!");
}
}
@Component
class Controller {
private final Service service;
@Autowired
public Controller(Service service) {
this.service = service;
}
public void controlAction() {
service.serve();
}
}
Why Dependency Matters
By allowing the framework to inject dependencies, the code remains flexible and maintainable. This approach parallels how interdisciplinary cooperation (or lack thereof) among nations post-WWI led to varying outcomes — demonstrating the interconnectedness of systems and their implications.
Lessons from History: Effective Practices in Programming
When we explore the historical impacts, we note the importance of adapting to changes and learning from the past. For Java developers, this means understanding common pitfalls and employing best practices.
1. Embrace Version Control
Just as historical discussions often reconsider earlier analyses, programmers should utilize version control systems like Git. It gives a snapshot of project states and facilitates team collaboration, much like how historical evidence provides a clearer understanding of past events.
2. Write Clean, Maintainable Code
Akin to good governance, which requires transparency and pragmatism, writing clean code ensures that other developers (or your future self) can easily follow your logic and the purpose of sections within the code.
3. Test Thoroughly
The importance of thorough testing cannot be overstated. Just as historical narratives often require validation through extensive sources, software development demands rigorous testing to ensure reliability and trustworthiness.
Final Thoughts
Java and its frameworks provide developers with essential tools for building robust applications and navigating complexities inherent in software development. Lessons drawn from history, such as those surrounding the Vertrag von Versailles, underscore the importance of foresight, structure, and collaboration. As we build software, let us remember the legacies of past decisions — both good and bad — and aim for coherent, sustainable practices.
For further reading on the historical impacts and how they shape modern perspectives, refer to the insights drawn in Vertrag von Versailles: Ursachen der globalen Kontroverse. As technology continuously pivots, understanding the past will light the way for future innovations in Java and beyond.