Debugging Java Frameworks: Enhancing UX in Menu Navigation
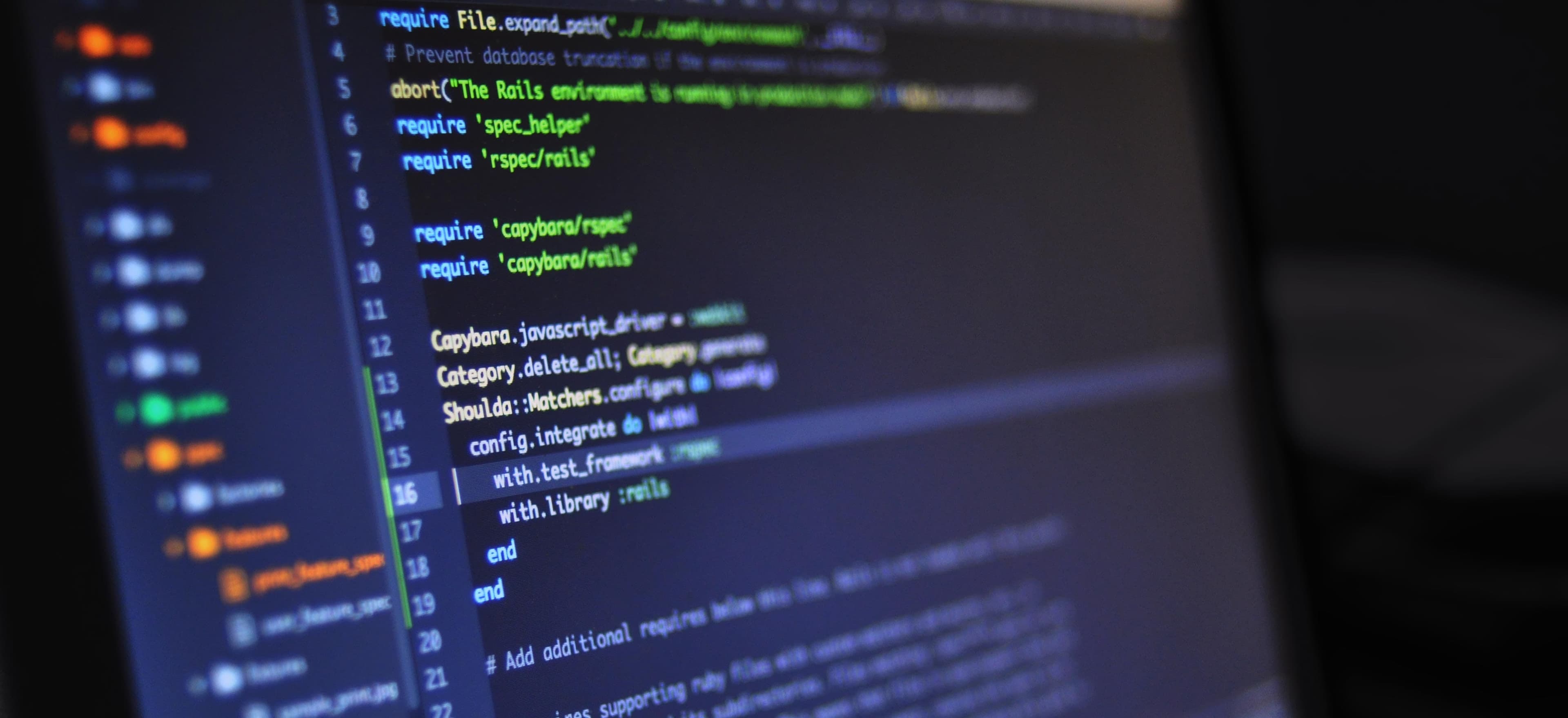
- Published on
Debugging Java Frameworks: Enhancing UX in Menu Navigation
In the fast-paced world of web development, User Experience (UX) can make or break a project. A seamless navigation system, particularly in web applications built on Java frameworks, is essential. This blog post will explore how debugging Java frameworks can enhance UX in menu navigation systems. Additionally, we will delve into the Suckerfish Menu Navigation, referencing the article "Fixing Suckerfish Menu Navigation Bugs for Better UX" for further insights.
Understanding Menu Navigation
Menu navigation serves as the backbone of any web application. It leads users to various functionalities and content offered by the application. A well-designed menu provides an intuitive user experience, allowing users to find what they need quickly. However, poorly constructed navigation can lead to frustration and abandonment.
Common Issues in Menu Navigation
Before diving into Java frameworks, let's examine some common issues that plague menu navigation:
- Responsive Design Flaws: Menus that don't adjust to different screen sizes create an inconsistent user experience.
- Submenu Bugs: Items that fail to open or close properly can confuse users.
- Accessibility Problems: Not adhering to accessibility standards can alienate users with disabilities.
- Performance Issues: Slow-loading menus can lead to an unresponsive feel.
Debugging Techniques in Java Frameworks
When dealing with Java frameworks, debugging is essential for enhancing UX. Here are some techniques that can help:
1. Logging Frameworks
Utilizing logging frameworks, like Log4j or SLF4J, can aid in tracking down where issues occur within your menu navigation code.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MenuNavigation {
private static final Logger logger = LoggerFactory.getLogger(MenuNavigation.class);
public void navigate(String selection) {
logger.info("Navigation attempt to: {}", selection);
// Code to handle navigation
}
}
In this snippet, a logger records navigation attempts. This way, you can trace user interactions and identify where problems might arise.
2. Debugging Tools
Use Integrated Development Environment (IDE) tools to step through code. For example, IntelliJ IDEA or Eclipse provides built-in debugging capabilities. Set breakpoints to pause execution and inspect variable states in real-time.
Why: This hands-on approach allows you to understand how data flows through your application and how menus respond at various state changes.
3. Unit Testing
Implement unit tests to ensure that each menu function works independently. Java frameworks like JUnit make this easy.
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MenuNavigationTest {
@Test
public void testMenuSelection() {
MenuNavigation menu = new MenuNavigation();
String result = menu.navigate("Home");
assertEquals("Expected Home page navigation", expectedResult, result);
}
}
Why is unit testing important? It ensures each component performs as intended. Bugs discovered at this early stage are easier and cheaper to fix.
4. Performance Profiling
Use profiling tools like Java VisualVM to identify performance bottlenecks in menu rendering. Slow performance can make the entire application feel sluggish.
Tip: Look at rendering times and responsiveness when hovering over menu items or clicking links.
Addressing Suckerfish Menu Navigation Bugs
The Suckerfish menu is a popular dropdown navigation style that works well, but it is not without its flaws. Bugs in the Suckerfish menu can lead to a poor user experience, facilitating issues such as problems with submenu visibility and responsiveness.
As noted in the article "Fixing Suckerfish Menu Navigation Bugs for Better UX" (see it here: tech-snags.com/articles/fixing-suckerfish-menu-bugs-ux), addressing these bugs can enhance overall navigation experience remarkably.
Example: Fixing Suckerfish Menu Bugs in Java
Let’s consider a potential issue where a submenu does not show when hovered over. Here's a basic structure showing how to implement logic in a Java web application back end:
public class Menu {
private Map<String, List<String>> menuItems = new HashMap<>();
public Menu() {
// Initializing menu items
menuItems.put("Main", Arrays.asList("Home", "About", "Services"));
menuItems.put("Services", Arrays.asList("Consulting", "Development", "Support"));
}
public List<String> getSubMenuItems(String menuName) {
return menuItems.getOrDefault(menuName, Collections.emptyList());
}
}
Commentary
In this example, we create a simple mapping of main menu items to their respective submenus. The method getSubMenuItems
returns a list of submenu items based on the input.
Why: Utilizing a Java Map
structure, we can easily retrieve submenu items dynamically, which adds flexibility to the menu navigation system and helps to resolve visibility issues as long as the front-end is designed to take advantage of this structure.
Best Practices for Menu Navigation
Now that we have discussed debugging methods and identified potential bugs, let’s look at some best practices:
- Ensure Responsiveness: Use CSS flexbox or grid to create a mobile-responsive design.
- Test Across Devices: Manually test your navigation system on various devices and screen sizes.
- Utilize WAI-ARIA: Ensure your menus are accessible to assistive technologies by using WAI-ARIA roles and properties.
- Keep It Simple: Avoid cluttering menus with too many options. Simplicity enhances usability.
Lessons Learned
Debugging Java frameworks is critical to enhancing User Experience, particularly in menu navigation systems. By employing logging techniques, unit testing, and performance profiling, developers can identify and rectify issues effectively. Moreover, by learning from existing resources such as "Fixing Suckerfish Menu Navigation Bugs for Better UX", developers can gain valuable insights into improving navigation and ensuring it meets user needs.
By applying these best practices and leveraging Java’s robust features, you can create a user-friendly menu system that enhances overall application functionality. Prioritize debugging strategies to offer a smoother, more intuitive user experience. Happy coding!
For further reading, take a look at the article we referenced earlier: Fixing Suckerfish Menu Navigation Bugs for Better UX.