Enhancing Java Web Apps with Solid Navigation Patterns
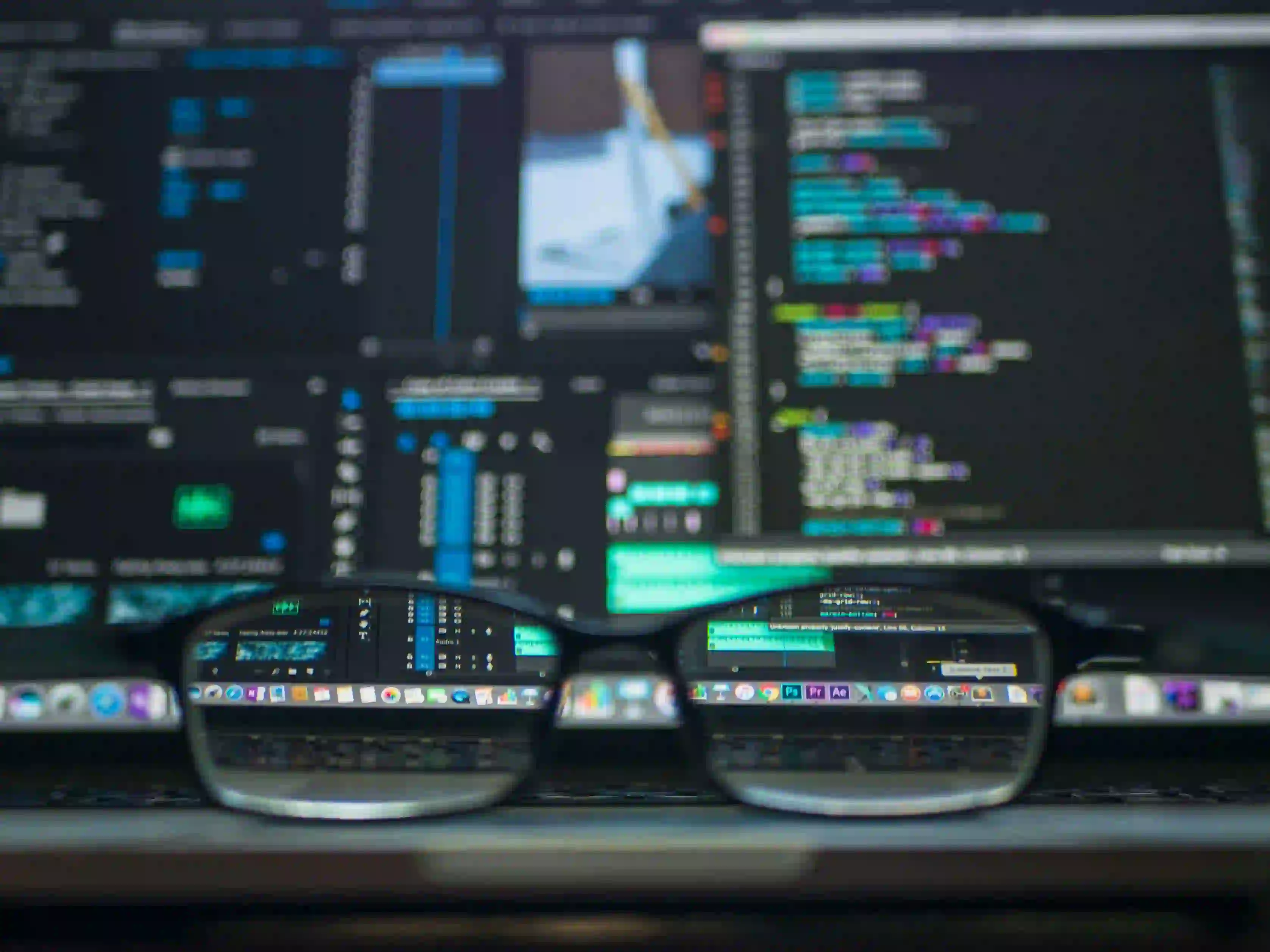
Enhancing Java Web Apps with Solid Navigation Patterns
When it comes to creating Java web applications, proper navigation is key to ensuring a seamless user experience. As outlined in the article “Fixing Suckerfish Menu Navigation Bugs for Better UX,” users often face frustration when they encounter navigation bugs. In this blog post, we will explore solid navigation patterns to improve usability in your Java web applications.
Why Navigation Matters
Navigational architecture is crucial because it directly influences how users interact with your application. Effective navigation allows users to find the content they need quickly, reducing frustration and improving engagement. If users can't navigate easily, they’re likely to abandon your site. Studies show that up to 70% of users leave a website due to poor navigation.
1. Consistent Navigation Layout
The Importance of Consistency
Having a consistent navigation layout creates familiarity and predictiveness for users. Layouts that shift drastically from page to page are disorienting. In Java web applications, you can implement a consistent layout using centralized header files.
Example:
<!-- header.jsp -->
<nav>
<ul>
<li><a href="home.jsp">Home</a></li>
<li><a href="about.jsp">About</a></li>
<li><a href="services.jsp">Services</a></li>
<li><a href="contact.jsp">Contact</a></li>
</ul>
</nav>
In the above snippet, header.jsp
defines a simple navigation menu. By including this file in every JSP page, you ensure consistent navigation.
<!-- anyPage.jsp -->
<%@ include file="header.jsp" %>
<h1>Welcome to My Page</h1>
<p>Your content here...</p>
Commentary
By incorporating a consistent navigation header across various pages, end-users become accustomed to the layout. User experience (UX) improves as they understand where to find key functionality across all parts of the application.
2. User-Centric Design
Knowing Your Audience
Understanding your users is pivotal. Their needs and expected pathways should guide your navigation design. Utilize user personas or conduct surveys to gather this information before development begins.
Tip: Utilize These Tools
- Google Analytics: Track visitor behavior and see what areas are most frequently accessed.
- Surveys and Feedback Forms: Create opportunities for users to share their experiences navigating your app.
Example of a User-Centric Menu
<!-- userProfile.jsp -->
<%@ include file="header.jsp" %>
<nav>
<ul>
<li><a href="settings.jsp">Settings</a></li>
<li><a href="logout.jsp">Logout</a></li>
</ul>
</nav>
<h1>User Profile</h1>
<p>Your user-specific content here...</p>
Commentary
User-centric navigation isn’t just about adding links but showing relevant options based on user roles. By providing specific links for users to manage their profiles effectively, you make navigation more intuitive.
3. Responsive Navigation
Think Mobile First
Given that many users access web applications on mobile devices, responsive navigation is essential. Using JavaScript frameworks like Bootstrap can make your navigation dynamic and adaptive to various device sizes.
Example:
<!-- Include Bootstrap CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<!-- Responsive Navbar -->
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">MyApp</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item active"><a class="nav-link" href="home.jsp">Home</a></li>
<li class="nav-item"><a class="nav-link" href="about.jsp">About</a></li>
<li class="nav-item"><a class="nav-link" href="services.jsp">Services</a></li>
<li class="nav-item"><a class="nav-link" href="contact.jsp">Contact</a></li>
</ul>
</div>
</nav>
Commentary
The above code snippet demonstrates how to build a responsive navigation bar using Bootstrap. As the screen size changes, the menu adapts accordingly, ensuring users maintain easy access regardless of their device.
4. Hierarchical Navigation Structure
Organizing Content
Establishing a clear hierarchical structure aids users in understanding how your content is organized. This can be accomplished by employing a breadcrumb navigation to allow users to retrace their steps easily.
Example:
<!-- breadcrumb.jsp -->
<nav aria-label="breadcrumb">
<ol class="breadcrumb">
<li class="breadcrumb-item"><a href="home.jsp">Home</a></li>
<li class="breadcrumb-item"><a href="services.jsp">Services</a></li>
<li class="breadcrumb-item active" aria-current="page">Detail</li>
</ol>
</nav>
Commentary
Breadcrumbs give users context about their current location on the site. This structure is not only user-friendly but also benefits SEO by allowing search engines to crawl your website more effectively.
5. Accessibility in Navigation
Keeping It Inclusive
Accessibility should never be an afterthought. Ensuring your navigation is usable for everyone, including people with disabilities, is crucial. Utilize semantic HTML and ARIA attributes to enhance accessibility.
<nav aria-label="Main navigation">
<ul>
<li><a href="home.jsp">Home</a></li>
<li><a href="about.jsp">About</a></li>
<li><a href="services.jsp">Services</a></li>
<li><a href="contact.jsp">Contact</a></li>
</ul>
</nav>
Commentary
Using aria-label
adds another layer of context that screen readers can use, making the navigation intuitive for users with visual impairments.
Final Considerations: Navigation is an Ongoing Process
Creating a seamless navigation experience in Java web applications does not stop after the initial phases of development. Continue to test your navigation under various conditions and collect user feedback. Regular updates and iterative improvements can vastly enhance the navigation experience over time.
By implementing these solid navigation patterns, you can ensure a smooth user journey through your application. Remember, an excellent user experience and effective navigation are key to retaining users and driving success for your web applications.
For further reading about enhancing usability in web applications, take a look at “Fixing Suckerfish Menu Navigation Bugs for Better UX.” Happy coding!