Solve Java Pagination Challenges with Effective Slider Strategies
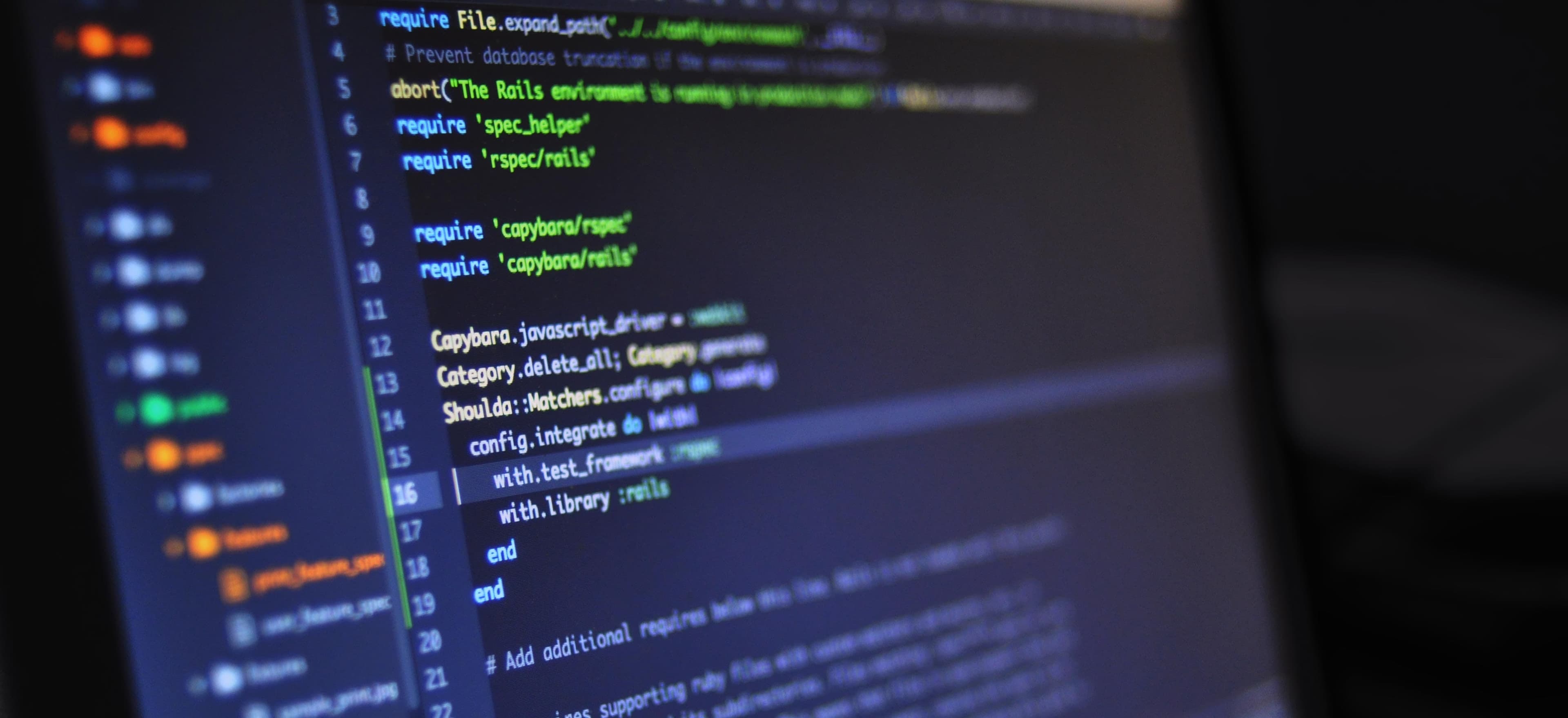
- Published on
Solve Java Pagination Challenges with Effective Slider Strategies
Pagination is a vital aspect of both web and mobile development, ensuring that content is delivered smoothly and efficiently. For Java developers, understanding how to implement pagination effectively can vastly improve user experiences and enhance application performance. In this post, we will explore various techniques to tackle pagination challenges in Java, focusing specifically on slider strategies.
What is Pagination?
Pagination refers to the process of dividing a large set of data into smaller, manageable chunks or pages. In a typical web application, users interact with data—such as lists of items, posts, or products—often requiring only a subset of the information at any given time. Effective pagination can not only improve load times but also the overall usability of an application.
Why Use Sliders for Pagination?
Sliders offer a unique way to navigate through data, providing a visual and interactive experience. Unlike traditional pagination methods that use numbered links, sliders allow users to smoothly scroll through items without losing track of their position.
Here are some key benefits of slider-based pagination:
- Enhanced User Experience: Users can quickly slide through items, making it easier to find what they need.
- Reduced Clutter: Sliders can display many items while keeping the interface clean and organized.
- Improved Load Times: Sliders often load data dynamically as users navigate, improving performance.
Getting Started: Java Pagination Basics
Before diving into slider implementation, let’s understand a few Java basics surrounding pagination.
Sample Data Model
Consider a scenario where we manage a collection of books. A simple Java class can represent our book model:
public class Book {
private String title;
private String author;
// Constructor
public Book(String title, String author) {
this.title = title;
this.author = author;
}
// Getters
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
}
This Book
class has basic attributes: title
and author
, along with the necessary constructors and getters.
Creating a Sample Data Repository
Next, we'll create a sample repository to hold a list of books. This data structure will simulate a database.
import java.util.ArrayList;
import java.util.List;
public class BookRepository {
private List<Book> books;
public BookRepository() {
books = new ArrayList<>();
// Adding sample books
books.add(new Book("Java Concurrency in Practice", "Brian Goetz"));
books.add(new Book("Effective Java", "Joshua Bloch"));
books.add(new Book("Spring in Action", "Craig Walls"));
// Add more books as needed
}
public List<Book> getBooks() {
return books;
}
}
In this example, we encapsulate a list of book objects within the BookRepository
. This provides a simple way to manage book data that we will use when implementing pagination.
Implementing Pagination
The next step is to implement the actual pagination logic. We will create a method that allows users to retrieve a slice of the book list based on the requested page number and size.
Pagination Logic
import java.util.List;
public class PaginationService {
private BookRepository repository;
public PaginationService(BookRepository repository) {
this.repository = repository;
}
public List<Book> getPaginatedBooks(int pageNo, int pageSize) {
List<Book> allBooks = repository.getBooks();
int start = pageNo * pageSize;
if (start >= allBooks.size()) {
return new ArrayList<>(); // Return empty if page number exceeds max
}
int end = Math.min(start + pageSize, allBooks.size());
return allBooks.subList(start, end);
}
}
Explanation
In this PaginationService
class, we have implemented a method called getPaginatedBooks
. This method takes two parameters: pageNo
(the desired page number) and pageSize
(the number of items per page).
start
is calculated by multiplying thepageNo
bypageSize
.- We check if
start
exceeds the total books available. - The
end
index is determined usingMath.min
to ensure we don’t go out of bounds.
The method returns a sublist of books based on the parameters provided.
Example Usage
Here's how you would use the PaginationService
in your application:
public static void main(String[] args) {
BookRepository repository = new BookRepository();
PaginationService paginationService = new PaginationService(repository);
int pageNo = 0; // First page
int pageSize = 2; // Two books per page
List<Book> booksOnPage = paginationService.getPaginatedBooks(pageNo, pageSize);
booksOnPage.forEach(book -> System.out.println(book.getTitle()));
}
Slider Implementation in Java
Now that we have created our pagination logic, the next step is to incorporate sliders for navigating through pages. While the actual implementation may vary based on the frontend framework you are using, here’s a basic conceptual outline using Java Swing as an example.
Java Swing Example
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class SliderPagination extends JFrame {
private JSlider slider;
private JLabel bookLabel;
private PaginationService paginationService;
private int pageCount;
public SliderPagination(PaginationService paginationService) {
this.paginationService = paginationService;
setupUI();
}
private void setupUI() {
setTitle("Book Slider Pagination");
setSize(400, 200);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLayout(new FlowLayout());
bookLabel = new JLabel();
add(bookLabel);
slider = new JSlider(0, pageCount - 1);
slider.addChangeListener(e -> updateBookDisplay());
add(slider);
updateBookDisplay();
}
private void updateBookDisplay() {
int pageIndex = slider.getValue();
List<Book> booksOnPage = paginationService.getPaginatedBooks(pageIndex, 2);
StringBuilder bookTitles = new StringBuilder("<html>");
for (Book book : booksOnPage) {
bookTitles.append(book.getTitle()).append("<br>");
}
bookTitles.append("</html>");
bookLabel.setText(bookTitles.toString());
}
public void setPageCount(int pageCount) {
this.pageCount = pageCount;
slider.setMaximum(pageCount - 1);
}
public static void main(String[] args) {
BookRepository repo = new BookRepository();
PaginationService service = new PaginationService(repo);
int totalBooks = repo.getBooks().size();
int pageSize = 2;
SliderPagination sliderPagination = new SliderPagination(service);
sliderPagination.setPageCount((int) Math.ceil((double) totalBooks / pageSize));
sliderPagination.setVisible(true);
}
}
Explanation
In this Swing example, we create a user interface featuring a slider that allows for navigation through book listings. The key components are:
- JSlider: Represents the slider component that users can move to select different pages.
- JLabel: Used for displaying the titles of books.
- updateBookDisplay(): This method fetches the books for the current page and updates the label accordingly.
The setPageCount
method allows setting the maximum page index based on the number of books divided by the page size.
Dynamic Page Count
Remember to update your slider's maximum value based on the total number of pages. You can calculate this when initializing the UI by creating an instance of SliderPagination
that evaluates the total number of books available.
Final Considerations
Effective pagination in Java, especially through the use of sliders, can significantly improve the end user's experience while interacting with applications.
By using the outlined pagination logic coupled with interactive sliders, developers can streamline data presentation and allow for smoother navigation.
To further your pagination knowledge, check out the article Mastering Pagination in PHP: Slider Implementation Tips.
This comprehensive look at Java pagination should give you the tools needed to build intuitive and responsive applications that handle data elegantly, ensuring your users remain engaged and satisfied. Happy coding!
Checkout our other articles