Java Pagination Unleashed: Solve Your Data Display Issues
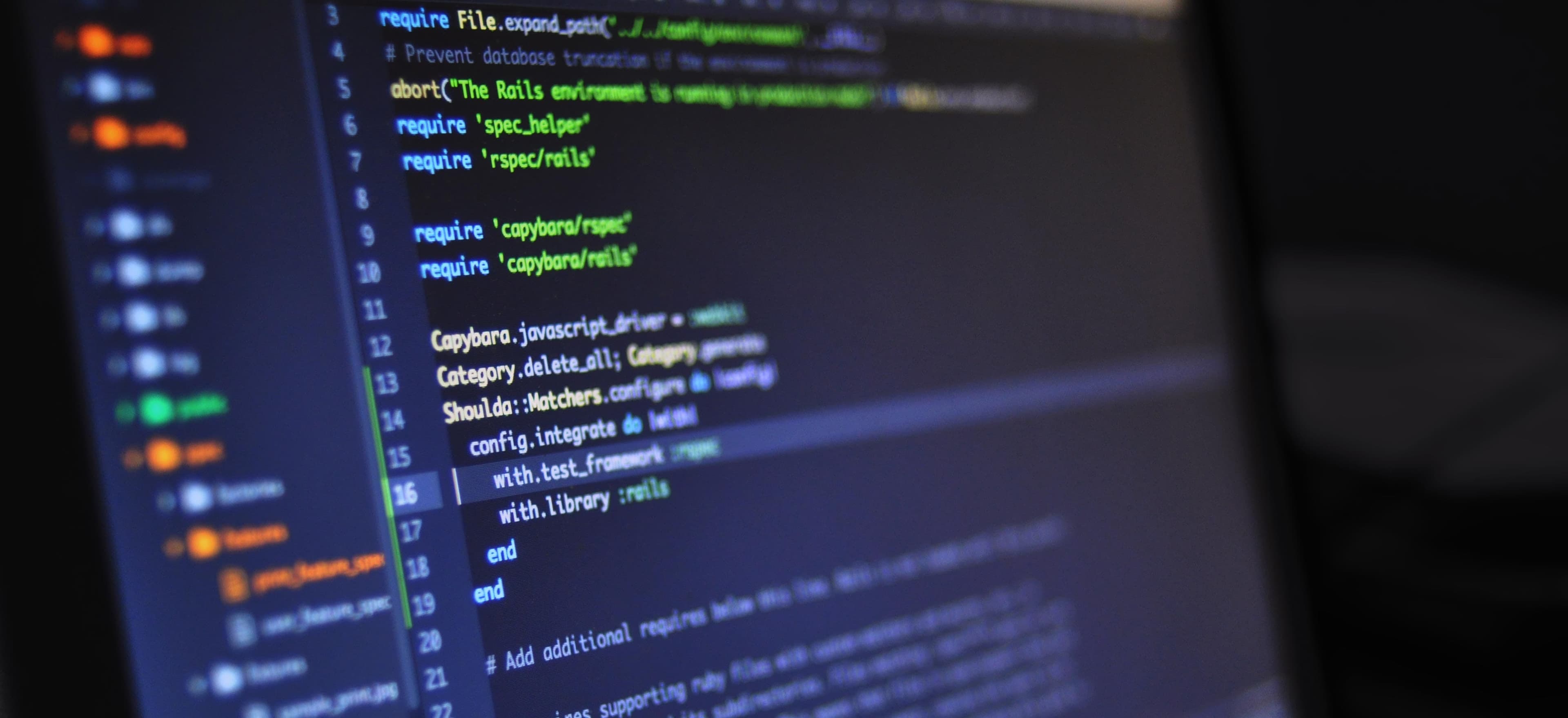
- Published on
Java Pagination Unleashed: Solve Your Data Display Issues
Pagination is an essential concept in web development, especially when dealing with large datasets. Whether you're displaying user profiles, product listings, or extensive blog articles, effective pagination can enhance user experience significantly. In this article, we will delve into effective strategies for implementing pagination in Java applications and explore practical examples, guiding you through the entire process.
Why Pagination Matters
When data grows, displaying it all at once can overwhelm users and degrade performance. Pagination breaks data into manageable sections. Effective pagination can:
- Improve Load Times: Loading fewer data entries reduces initial loading times.
- Enhance User Experience: Users can navigate through data without feeling inundated.
- Facilitate Better Data Management: Easier to display and manipulate smaller subsets of data.
Given these advantages, let's explore how to implement pagination in Java.
Setting Up the Environment
Before diving into code, ensure you've got your Java environment set up with a servlet container like Apache Tomcat or any other Java EE-compliant server. Also, familiarize yourself with frontend basics, as you'll need it for presenting paginated results.
Pagination Logic in Java
The pagination logic generally revolves around the following concepts:
- Current Page Number: The page the user is currently on.
- Total Number of Items: The total count of data entries.
- Items Per Page: The number of entries displayed on each page.
- Calculating Total Pages: Total pages can be calculated as
Math.ceil(totalItems / itemsPerPage)
.
Let’s see how this works in practice with sample code.
Sample Code for Pagination
We will create a simple Java servlet that demonstrates this pagination concept. Assume we have a list of items (e.g., products), and we want to paginate through them.
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
import java.util.List;
public class PaginationServlet extends HttpServlet {
private static final int ITEM_PER_PAGE = 10; // Set page size
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Fetching the current page number from request
int currentPage = Integer.parseInt(request.getParameter("page", "1"));
List<Item> items = fetchData(); // Assume this function fetches data from database
// Calculating total pages
int totalItems = items.size();
int totalPages = (int) Math.ceil(totalItems / (double) ITEM_PER_PAGE);
// Calculate the start and end index for the current page
int startIndex = (currentPage - 1) * ITEM_PER_PAGE;
int endIndex = Math.min(startIndex + ITEM_PER_PAGE, totalItems);
// Get sublist for current page
List<Item> paginatedItems = items.subList(startIndex, endIndex);
// Attaching data to request
request.setAttribute("items", paginatedItems);
request.setAttribute("currentPage", currentPage);
request.setAttribute("totalPages", totalPages);
// Forwarding to JSP page to display items
RequestDispatcher dispatcher = request.getRequestDispatcher("items.jsp");
dispatcher.forward(request, response);
}
}
Commentary on the Code
-
Current Page: The current page is retrieved from the request. This is essential for determining which subset of data to display.
-
Total Items and Calculation of Pages: We calculate the total pages using a straightforward formula. This ensures that users know how many pages of data exist.
-
Sublist for Current Page: By using the
subList
method, we create a slice of the original list. This approach is efficient as it allows us to avoid unnecessary data processing. -
Request Attributes: Finally, we attach the paginated list and page information to the request. This makes it accessible within our JSP for displaying.
Frontend Implementation
Once we have our servlet set up, we need to display the data on our frontend. Let's create a simple JSP page called items.jsp
that utilizes the paginated data.
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Paginated Items</title>
</head>
<body>
<h1>Items List</h1>
<table border="1">
<tr>
<th>ID</th>
<th>Name</th>
</tr>
<c:forEach var="item" items="${items}">
<tr>
<td>${item.id}</td>
<td>${item.name}</td>
</tr>
</c:forEach>
</table>
<div>
<c:if test="${currentPage > 1}">
<a href="?page=${currentPage - 1}">Previous</a>
</c:if>
<c:if test="${currentPage < totalPages}">
<a href="?page=${currentPage + 1}">Next</a>
</c:if>
</div>
<p>Page ${currentPage} of ${totalPages}</p>
</body>
</html>
Explanation of the JSP
-
Table Display: We utilize JSTL (Java Server Pages Standard Tag Library) to iterate over
items
and present them in a tabular format. -
Pagination Controls: Conditional links allow navigation between pages. If you're on the first page, the "Previous" link won't appear, and similarly, the "Next" link won't be displayed on the last page.
Enhancements to Consider
While the above code provides a fundamental pagination implementation, consider the following enhancements for a more robust solution:
-
Sorting: Allow users to sort data based on various fields.
-
Filtering: Implement options to filter the dataset, which can lead to more targeted pagination.
-
Styling: A good UI enhances the user experience. Utilize frameworks like Bootstrap for better aesthetics.
-
Asynchronous Pagination: Utilize AJAX to load new data without refreshing the page.
For advanced pagination techniques in a related language, you can refer to Mastering Pagination in PHP: Slider Implementation Tips.
A Final Look
Pagination is crucial for managing large datasets effectively in Java applications. This guide covered a simple yet effective way to implement pagination using servlets and JSP. Always remember to evaluate user experience through effective navigation, and consider implementing enhancements to provide an even better experience.
By grasping these fundamentals, you’ll be well-equipped to handle pagination in your Java applications, significantly improving how users interact with data. Happy coding!