Java Techniques for Handling HTML Form Element Styles
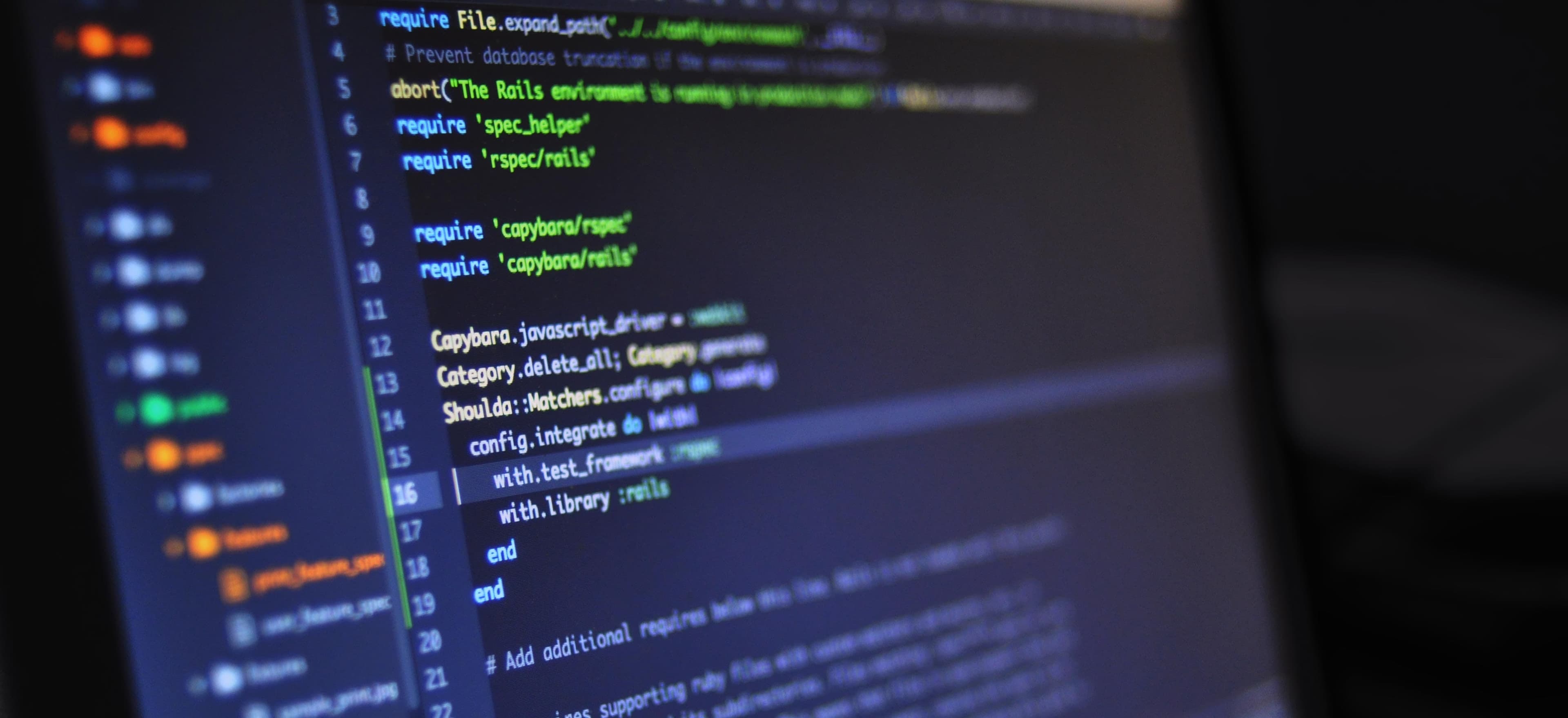
- Published on
Java Techniques for Handling HTML Form Element Styles
In the rapidly evolving world of web development, the integration of Java with HTML technologies remains indispensable. Specifically, leveraging Java to control the styles of HTML form elements can significantly enhance user experience while maintaining aesthetic appeal. In this blog post, we will explore various techniques to manage the styles of HTML form elements using Java, as well as how you can utilize existing resources effectively, such as How to Change Text Color in HTML Form Elements.
Understanding the Role of Styles in Web Forms
Styles dictate how elements appear on your page. They can enhance usability, convey brand identity, and provide visual feedback to users. When dealing with forms, styles can control aspects like:
- Text color
- Background color
- Border styles
- Padding and margins
Using Java to manipulate these styles dynamically, we can make forms interactive and visually appealing, encouraging user engagement.
Setting Up Your Environment
Before diving into code, let's ensure you have the necessary setup. Typically, you will need:
- A Java web server like Apache Tomcat
- JDK installed on your machine
- A good editor such as IntelliJ IDEA or Eclipse
Once your environment is ready, let’s explore the different Java techniques for handling HTML form element styles.
1. Using Servlet API for Dynamic Styles
Java Servlets provide an ideal backend solution to handle requests from HTML pages. You can manipulate style attributes before sending HTML content back to the client. Here is an example:
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/formStyle")
public class FormStyleServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
StringBuilder htmlResponse = new StringBuilder();
htmlResponse.append("<html>")
.append("<head><style>")
.append("input { color: blue; }") // Set text color to blue
.append("</style></head>")
.append("<body>")
.append("<form>")
.append("<input type='text' placeholder='Enter name'/>")
.append("</form>")
.append("</body></html>");
response.getWriter().write(htmlResponse.toString());
}
}
Why This Code?
In this example, we explore how to dynamically generate HTML with embedded CSS by utilizing a Java Servlet. The input
elements' text color is set to blue, creating a visually appealing form.
Key Learning Points:
- Flexibility: With Java Servlets, you can alter styles based on user input or other criteria.
- Readability: Using a StringBuilder allows for efficient concatenation of HTML strings.
2. Utilizing JSP (JavaServer Pages)
JSP offers a way to create dynamic web content with Java code embedded directly into HTML. This technique is particularly useful for smaller applications. Here’s an example of styling a text box:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<style>
.styled-input {
color: red; /* Text color */
background-color: yellow; /* Background color */
padding: 5px; /* Padding */
border: 2px solid green; /* Border with color */
}
</style>
</head>
<body>
<form>
<input type="text" class="styled-input" placeholder="Enter text here..." />
</form>
</body>
</html>
Why This Code?
Using JSP lets you seamlessly integrate Java code with HTML. The style for the input field includes different attributes, which makes it visually distinct and user-friendly.
Key Learning Points:
- Separation of Concerns: This approach keeps your styles organized and your HTML clean.
- Reusability: CSS classes can be reused across multiple elements or pages.
3. AJAX for Dynamic Style Change
AJAX can be an effective technique for changing styles on the fly without requiring page reloads. Here’s a simple example using Java with a bit of JavaScript:
<!DOCTYPE html>
<html>
<head>
<script>
function changeStyle() {
var textInput = document.getElementById("dynamicInput");
textInput.style.color = "purple"; // Change text color on button click
}
</script>
</head>
<body>
<form>
<input type="text" id="dynamicInput" placeholder="Click the button to change color" />
<button type="button" onclick="changeStyle()">Change Color</button>
</form>
</body>
</html>
Why This Code?
This example dynamically alters the text color of an input field using JavaScript. The integration of Java for backend processing coupled with JavaScript on the client side creates a seamless user experience.
Key Learning Points:
- Interactivity: Users can interact with the form elements without reloading the page.
- Instant Feedback: The visual change is immediate, improving user engagement.
4. Frameworks like Spring Boot
For larger applications, using frameworks like Spring Boot can encapsulate styling logic into a more robust architecture. Here’s a brief overview of using Thymeleaf (a popular templating engine) with Spring Boot.
@Controller
public class FormController {
@GetMapping("/form")
public String showForm(Model model) {
model.addAttribute("textColor", "blue");
return "formView"; // Refers to formView.html
}
}
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<style>
input {
color: [[${textColor}]]; /* Injected from the model */
}
</style>
</head>
<body>
<form>
<input type="text" placeholder="Dynamic text color" />
</form>
</body>
</html>
Why This Code?
Spring Boot allows you to manage styles effectively through model attributes, separating concerns and enhancing maintainability.
Key Learning Points:
- Clear Structure: Using frameworks enforces a clear MVC structure.
- Dynamic Content Injection: This allows styling to adjust based on user interaction or server-side conditions.
My Closing Thoughts on the Matter
Integrating Java with HTML form elements for style management is both efficient and enriching for user experience. The techniques discussed — from Servlets to JSP, AJAX, and advanced frameworks like Spring Boot — provide various avenues to achieve this.
As you explore these methods, consider reading external guides, such as the aforementioned article on How to Change Text Color in HTML Form Elements to deepen your understanding of related concepts.
By mastering these Java techniques, you become equipped to craft visually appealing and user-friendly forms. Happy coding!