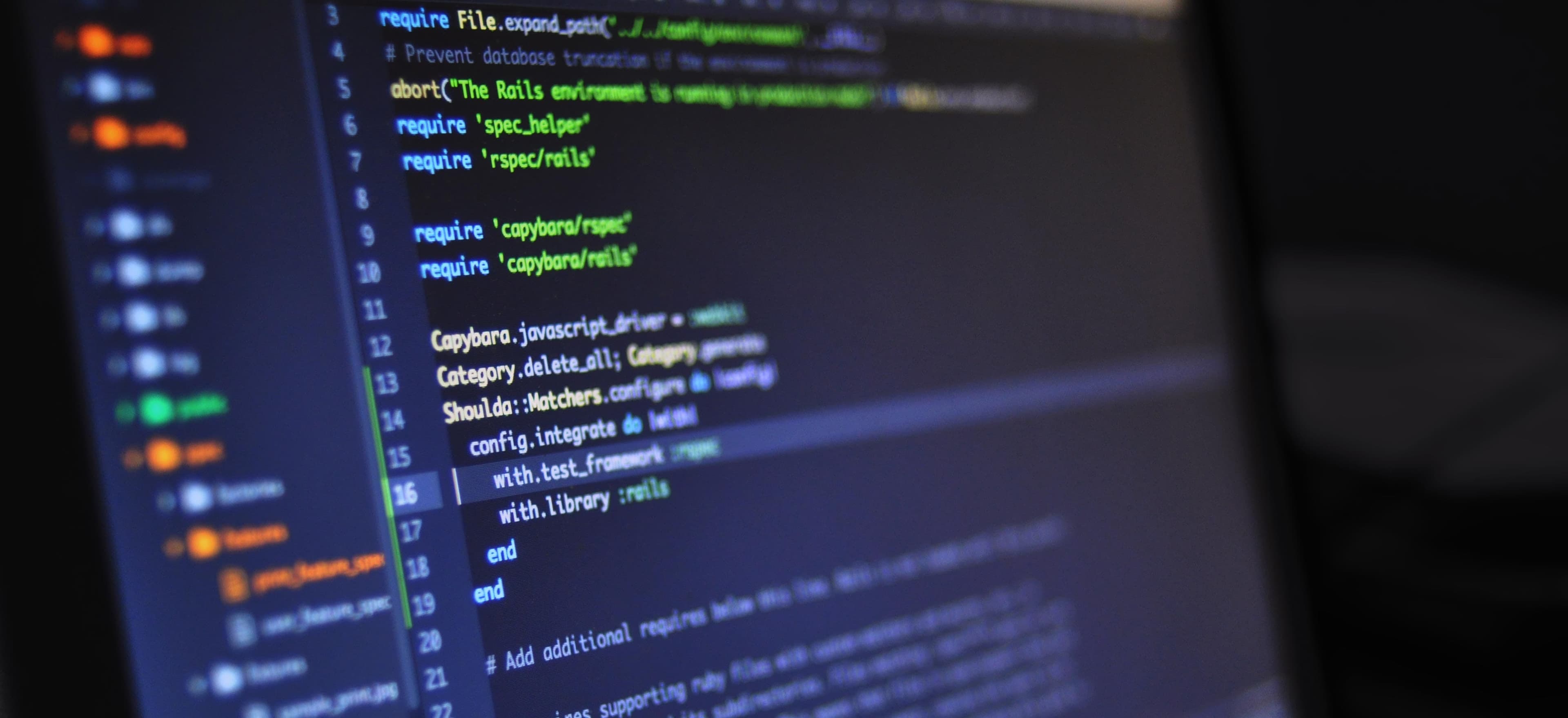
- Published on
Bridging Java Frameworks with Design Tokens for Theming
In the realm of user interface design and web development, the importance of cohesive theming cannot be overstated. The evolution of software design has introduced several paradigms and tools that aim to streamline the process of creating visually appealing and user-friendly applications. One such innovation is the concept of design tokens — a standardized approach to managing and applying design style values. This blog post will explore how Java frameworks can effectively leverage design tokens for seamless theming, while also drawing insights from the article titled "Mastering Design Tokens for Seamless Theming".
Understanding Design Tokens
Before diving into Java frameworks, let’s clarify what design tokens are. Design tokens are small, reusable entities that represent design decisions. These can involve colors, typography, spacing, and other UI-related properties. By defining these tokens in a centralized manner, developers can ensure consistency across various platforms and frameworks.
For instance:
{
"color": {
"primary": "#007bff",
"secondary": "#6c757d"
},
"font": {
"baseSize": "16px",
"headingSize": "24px"
},
"spacing": {
"small": "8px",
"medium": "16px",
"large": "32px"
}
}
This JSON structure illustrates how design tokens can encapsulate basic design values. The real power of design tokens lies in their ability to unify design language, irrespective of where they’re implemented — whether in a Java-based application or any other platform.
The Role of Java Frameworks
Java is a powerful programming language supported by a variety of frameworks, such as Spring, JavaFX, and Hibernate, each catering to different aspects of application development. Integrating design tokens within these frameworks can significantly enhance the theming and styling processes.
Leveraging Design Tokens in JavaFX
JavaFX is a popular framework for building desktop applications in Java. It allows developers to create rich UIs that are both attractive and interactive. To illustrate how design tokens can be used in JavaFX:
- Create a Token Configuration Class: This class will encapsulate design token values.
public class DesignTokens {
private static final String PRIMARY_COLOR = "#007bff";
private static final String SECONDARY_COLOR = "#6c757d";
public static String getPrimaryColor() {
return PRIMARY_COLOR;
}
public static String getSecondaryColor() {
return SECONDARY_COLOR;
}
}
- Apply Tokens in your JavaFX UI:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ThemedApp extends Application {
@Override
public void start(Stage primaryStage) {
Button primaryButton = new Button("Primary Action");
primaryButton.setStyle("-fx-background-color: " + DesignTokens.getPrimaryColor());
StackPane root = new StackPane();
root.getChildren().add(primaryButton);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Design Tokens in JavaFX");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why This Matters: By centralizing design tokens in a dedicated configuration class, any alterations to the design values can be managed efficiently. If a change is required — for example, updating the primary color — developers need only to edit the value in one location.
Utilizing Design Tokens in Spring Boot
Spring Boot is another significant Java framework, primarily used for building web applications. The integration of design tokens here can take advantage of frontend frameworks like Thymeleaf or React when paired with RESTful APIs.
Example: Injecting Design Tokens into Thymeleaf
- Create a Properties File: Define your design tokens in an application properties file.
design.token.primary.color=#007bff
design.token.secondary.color=#6c757d
- Access Tokens in Your Controller:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class ThemeController {
@Value("${design.token.primary.color}")
private String primaryColor;
@Value("${design.token.secondary.color}")
private String secondaryColor;
@GetMapping("/themed-page")
public String getThemedPage(Model model) {
model.addAttribute("primaryColor", primaryColor);
model.addAttribute("secondaryColor", secondaryColor);
return "themed";
}
}
- Integrate Tokens in Thymeleaf Template:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Themed Page</title>
<style>
.primary-button {
background-color: [[${primaryColor}]];
color: white;
}
</style>
</head>
<body>
<button class="primary-button">Primary Action</button>
</body>
</html>
Why This Approach Works: By utilizing @Value
, developers can inject design token values directly into their web application's templates. This creates a flexible theming solution wherein changes to design tokens are automatically reflected across the UI without extensive rework.
The Need for Flexibility and Scalability
In both the desktop and web application contexts, the incorporation of design tokens allows for streamlined theming. The separation of design values from implementation logic not only enhances maintainability but also facilitates collaboration between designers and developers. This practice is particularly beneficial in larger applications where a uniform design consistency is paramount.
Design tokens provide a foundation for scaling and updating themes as the project evolves. As discussed in the article on Mastering Design Tokens for Seamless Theming, the core principle of design tokens lies in their adaptability across multiple platforms, reinforcing the idea of a unified approach to design.
Final Considerations
In summary, bridging Java frameworks with design tokens creates a more efficient workflow for implementing themes. Whether you're working with JavaFX for desktop applications or Spring Boot for web applications, design tokens allow for easily modifiable and coherent styling practices, resulting in improved user experiences.
By understanding and leveraging design tokens, Java developers can ensure design consistency, facilitate scaling, and maintain flexibility. As we move forward in an increasingly design-driven world, adopting such methodologies will only serve to enhance our applications further.
For a deeper dive into design tokens and their benefits, I highly recommend reading the full article on Mastering Design Tokens for Seamless Theming.
Keep your designs consistent, maintainable, and scalable. Happy coding!
Checkout our other articles