Streamlining Java UI with Design Tokens for Theming
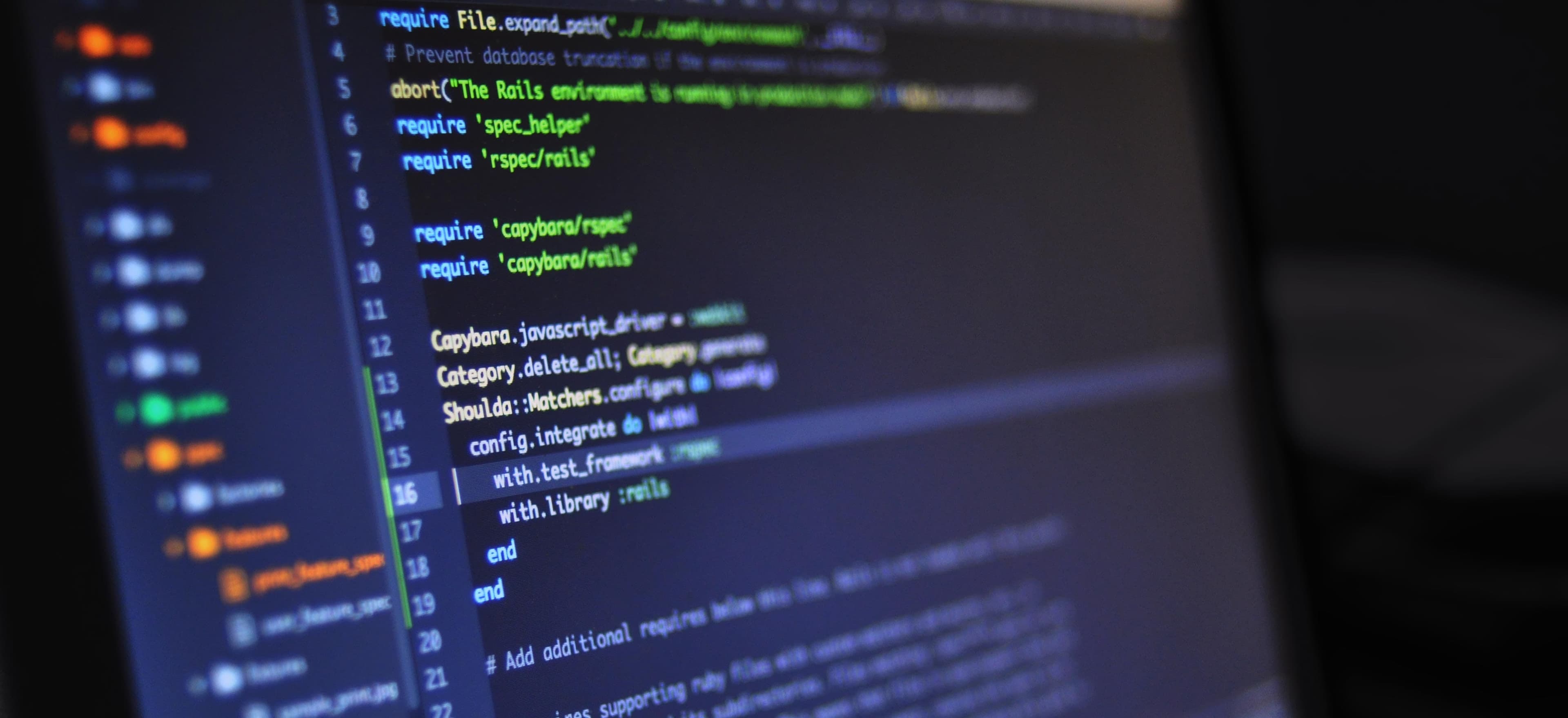
- Published on
Streamlining Java UI with Design Tokens for Theming
In the world of Java UI development, maintaining a consistent design across different components of an application can be challenging. The introduction of design tokens simplifies this task by providing a systematic approach to theming. In this blog post, we will explore how to implement design tokens in your Java application for a seamless and cohesive user interface. If you are unfamiliar with the concept of design tokens, I recommend checking out the article titled Mastering Design Tokens for Seamless Theming for a foundational understanding.
What are Design Tokens?
Design tokens are essentially a set of key-value pairs that store design decisions. They encapsulate various properties such as color, typography, spacing, and more into reusable units. Instead of hardcoding values across your UI components, you can reference these tokens, making it easier to maintain and update your design system.
For example, instead of using specific color codes like #FF5733
, you can define a design token color-primary
and utilize that throughout your application. This approach not only promotes consistency but also helps developers and designers collaborate more efficiently.
Benefits of Using Design Tokens in Java UI
- Consistency: With design tokens, you ensure uniformity in your application's UI, which contributes to a better user experience.
- Maintainability: Updating a token’s value reflects changes across the entire application, minimizing the need for extensive code modifications.
- Scalability: As your application grows, organizing styles with design tokens makes it easier to manage and scale your design system.
- Collaboration: Developers and designers can work from the same set of tokens, reducing discrepancies and enhancing teamwork.
Implementing Design Tokens in Java
To illustrate how design tokens can be integrated into a Java UI application, we'll create a simple desktop application using JavaFX. This approach highlights how to define design tokens and apply them effectively across GUI components.
Step 1: Defining Design Tokens
The first step is to define your design tokens. In a Java application, these can be organized in a properties file or directly within your Java code. For this example, we will define them as constants in a separate class.
public class DesignTokens {
public static final String COLOR_PRIMARY = "#6200EA";
public static final String COLOR_SECONDARY = "#03DAC5";
public static final String FONT_SIZE_LARGE = "16px";
public static final String FONT_SIZE_MEDIUM = "14px";
public static final String FONT_SIZE_SMALL = "12px";
public static final double PADDING_DEFAULT = 10.0;
}
In this code snippet, we declare several design tokens for colors and font sizes. This approach makes it easy to modify these values in one central location, underscoring the maintainability aspect of design tokens.
Step 2: Creating the JavaFX Application
Now, let's create a simple JavaFX application that uses these design tokens:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class DesignTokensApp extends Application {
@Override
public void start(Stage stage) {
Label titleLabel = new Label("Welcome to the JavaFX App");
titleLabel.setStyle("-fx-font-size: " + DesignTokens.FONT_SIZE_LARGE + "; " +
"-fx-text-fill: " + DesignTokens.COLOR_PRIMARY + ";");
Button actionButton = new Button("Click Me");
actionButton.setStyle("-fx-background-color: " + DesignTokens.COLOR_SECONDARY + "; " +
"-fx-padding: " + DesignTokens.PADDING_DEFAULT + "px;");
VBox vbox = new VBox(titleLabel, actionButton);
vbox.setSpacing(DesignTokens.PADDING_DEFAULT);
Scene scene = new Scene(vbox, 300, 200);
stage.setTitle("JavaFX with Design Tokens");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Discussion
In this example, we create a basic JavaFX application consisting of a Label and a Button. Both components utilize design tokens to set styles such as font size and color.
- Why Use Tokens? Using tokens (like
DesignTokens.FONT_SIZE_LARGE
) ensures that if you need to change the primary font size across the application, you only modify the value in one location. This can significantly reduce technical debt and streamline the update process.
Step 3: Extending Style with CSS
While using inline styles is valid, JavaFX also supports external CSS files for styling, which can further enhance the maintainability of your application. You can define CSS styles that refer to your design tokens.
Create a file named styles.css
:
.label-header {
-fx-font-size: 16px; /* FONT_SIZE_LARGE */
-fx-text-fill: #6200EA; /* COLOR_PRIMARY */
}
.button-main {
-fx-background-color: #03DAC5; /* COLOR_SECONDARY */
-fx-padding: 10px; /* PADDING_DEFAULT */
}
Then, apply these styles in your Java code:
titleLabel.getStyleClass().add("label-header");
actionButton.getStyleClass().add("button-main");
Benefits of Using CSS for Design Tokens
- Separation of Concerns: By using CSS, you separate stylistic elements from your Java logic, leading to cleaner code.
- Reusability: CSS classes can be reused across multiple components, promoting consistency and reducing duplication.
Tracking Changes with Version Control
Using design tokens not only simplifies styling but also plays nicely with version control systems. Changes to any design token can be tracked, making it easier to revert or analyze design decisions over time. This is particularly beneficial in collaborative environments where multiple developers might be working on the UI simultaneously.
Best Practices for Using Design Tokens
- Consistent Naming: Use clear and consistent naming conventions for your tokens to avoid confusion.
- Document Tokens: Maintain documentation for your design tokens to ensure everyone on the team understands their respective meanings and uses.
- Regular Updates: Periodically review and update your design tokens as necessary to reflect the changing needs of your application and design trends.
- Leverage Tools: Explore design token management tools and libraries that automate the generation and distribution of tokens across platforms.
Bringing It All Together
Integrating design tokens into your Java UI development not only streamlines your theming process but also enhances consistency, maintainability, and collaboration. By following the strategies outlined in this blog post, you can create a robust design system that evolves with your application.
For a deeper understanding of design tokens and their applications in theming, don’t forget to check out the insightful article Mastering Design Tokens for Seamless Theming.
By embracing design tokens in your Java applications, you can take a significant step towards achieving a more polished and coherent user experience, which is crucial in today's competitive landscape. Start implementing design tokens in your projects today and witness the transformation in your development workflow!
Checkout our other articles