Streamlining Java UI Development with Design Tokens
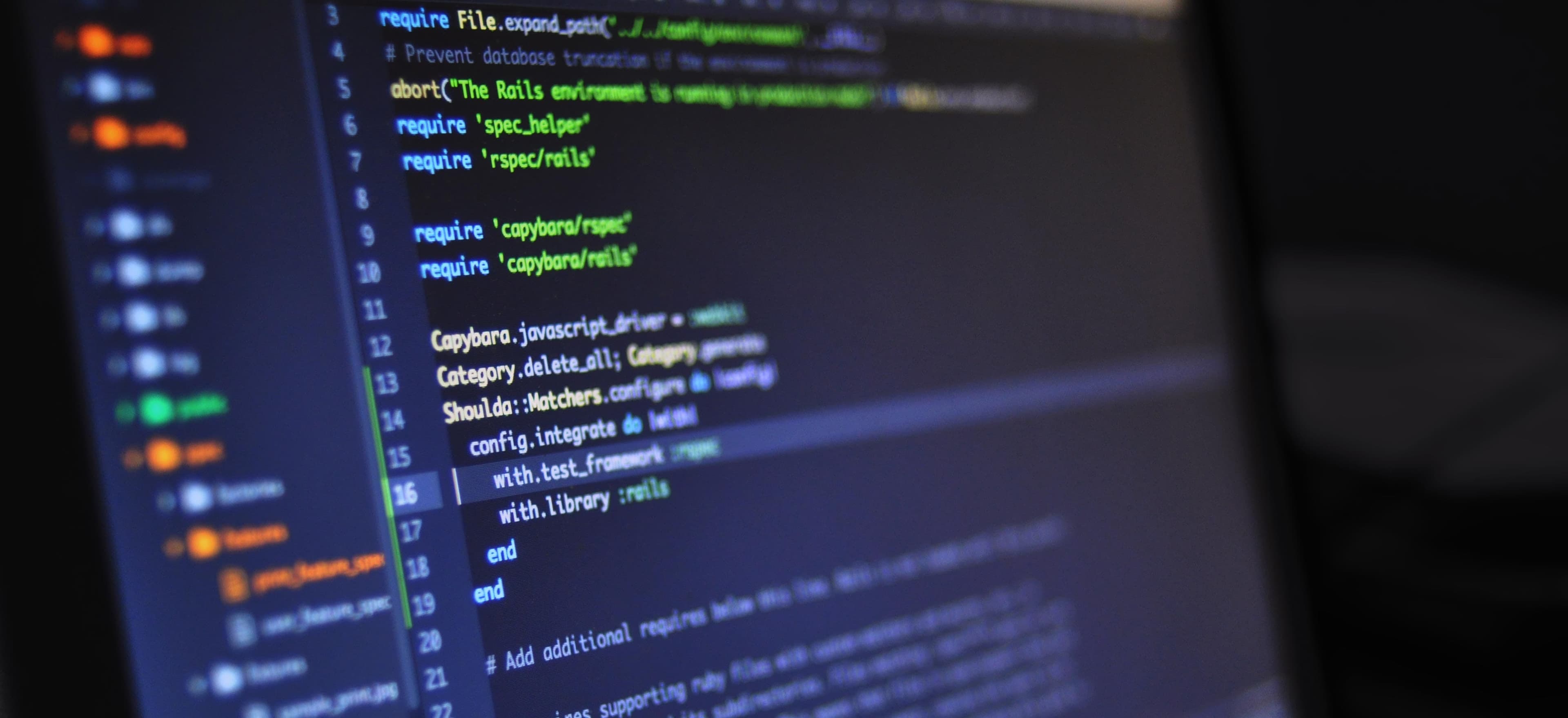
- Published on
Streamlining Java UI Development with Design Tokens
In the evolving world of software development, the need for consistent and maintainable user interfaces has led to the adoption of various design methodologies. One of the standout concepts that have been gaining significant traction is the idea of design tokens. This article explores how Java developers can leverage design tokens to streamline UI development and ensure a more cohesive design across their applications.
Understanding Design Tokens
Design tokens are essentially a set of variables that represent the visual properties of a design system. Think of them as the backbone of a user interface, encompassing everything from colors and typography to spacing and shadows. By defining these attributes as tokens, developers can create a system that is not only flexible but also inherently maintainable.
For a deeper understanding of design tokens, refer to Mastering Design Tokens for Seamless Theming, which explains the concept with a broader perspective.
Benefits of Design Tokens in Java UI Development
-
Consistency Across Platforms: When working in a multi-platform environment, design tokens ensure that your Java application's look and feel are uniform, irrespective of where it is deployed.
-
Easy Theme Changes: Want to change the entire color palette of your application? With design tokens, you can modify a single value, and the changes will propagate throughout your app.
-
Improved Collaboration: Designers and developers can work more coherently when design tokens are defined. Designers can focus on the visual aspects, while developers can translate those into code.
-
Scalability: As applications grow, maintaining UI consistency can become a challenge. Design tokens simplify this process, allowing for easy updates and scaling.
Implementing Design Tokens in Java
Now, let's dive into the practical aspect of using design tokens in a Java application. Below are steps and corresponding code examples to help you integrate design tokens effectively.
Step 1: Define Your Design Tokens
Start by defining the various visual properties of your application. In Java, this can be done through an Enum or a class with static variables.
public class DesignTokens {
public static final String PRIMARY_COLOR = "#3498db";
public static final String SECONDARY_COLOR = "#2ecc71";
public static final String FONT_FAMILY = "Arial, sans-serif";
public static final int BASE_FONT_SIZE = 16;
public static final int SPACING_UNIT = 8;
}
Why this approach? By keeping these properties centralized, you can easily access and modify them throughout your application. This reduces the possibility of inconsistent design elements.
Step 2: Use Design Tokens in Your UI Components
Once you've defined your design tokens, the next step is to implement them across your UI components. Here’s how you can do it using Java’s Swing framework.
import javax.swing.*;
import java.awt.*;
public class DesignTokenButton extends JButton {
public DesignTokenButton(String text) {
super(text);
// Apply design tokens
setBackground(Color.decode(DesignTokens.PRIMARY_COLOR));
setForeground(Color.white);
setFont(new Font(DesignTokens.FONT_FAMILY, Font.PLAIN, DesignTokens.BASE_FONT_SIZE));
setMargin(new Insets(DesignTokens.SPACING_UNIT, DesignTokens.SPACING_UNIT,
DesignTokens.SPACING_UNIT, DesignTokens.SPACING_UNIT));
}
public static void main(String[] args) {
JFrame frame = new JFrame("Design Token Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new DesignTokenButton("Click Me"));
frame.setSize(300, 200);
frame.setVisible(true);
}
}
Why use this method? By applying design tokens directly within your components, you achieve a cohesive style without the need to repeatedly define styles. This not only makes your code cleaner but also makes changing designs a breeze.
Step 3: Utilizing Design Tokens for Theming
One of the strongest advantages of design tokens is their capability to facilitate theming. Suppose you want to toggle between a light and dark theme. You can define separate sets of design tokens.
public class LightThemeTokens {
public static final String BACKGROUND_COLOR = "#ffffff";
public static final String TEXT_COLOR = "#000000";
}
public class DarkThemeTokens {
public static final String BACKGROUND_COLOR = "#000000";
public static final String TEXT_COLOR = "#ffffff";
}
You can create a method to switch themes dynamically:
public class ThemedButton extends JButton {
public ThemedButton(String text, boolean isDarkTheme) {
super(text);
// Apply respective design tokens based on theme
if (isDarkTheme) {
setBackground(Color.decode(DarkThemeTokens.BACKGROUND_COLOR));
setForeground(Color.decode(DarkThemeTokens.TEXT_COLOR));
} else {
setBackground(Color.decode(LightThemeTokens.BACKGROUND_COLOR));
setForeground(Color.decode(LightThemeTokens.TEXT_COLOR));
}
}
}
Why this is beneficial? By structuring your tokens to accommodate themes, switching themes becomes as simple as changing the class used to retrieve the color values. This approach encourages scalability as your application grows.
Best Practices for Using Design Tokens in Java UI Development
-
Keep Tokens Consistent: Ensure that your tokens maintain a consistent naming convention and structure. This improves readability and maintainability.
-
Version Control Your Tokens: If multiple developers are working on the UI, using version control systems helps track changes in design tokens, preventing conflicts.
-
Regularly Review and Refine: As your application evolves, so should your design tokens. Regularly revisiting them ensures they still meet the overall design requirements.
-
Document Everything: Providing documentation for tokens can greatly help other developers in your team understand and utilize them better.
A Final Look
The adoption of design tokens can significantly enhance your Java UI development process. By streamlining design consistency and enabling efficient theming, they pave the way for more maintainable and scalable applications. As you implement these tokens in your Java projects, keep in mind the best practices outlined for optimal effectiveness.
For further insights into the power of design tokens and their application in UI development, don’t forget to check out Mastering Design Tokens for Seamless Theming.
By embracing this flexible approach to design and development, you not only improve your codebase but also create a more engaging user experience. Happy coding!
Checkout our other articles