Mastering Java Development with Node.js Version Compatibility
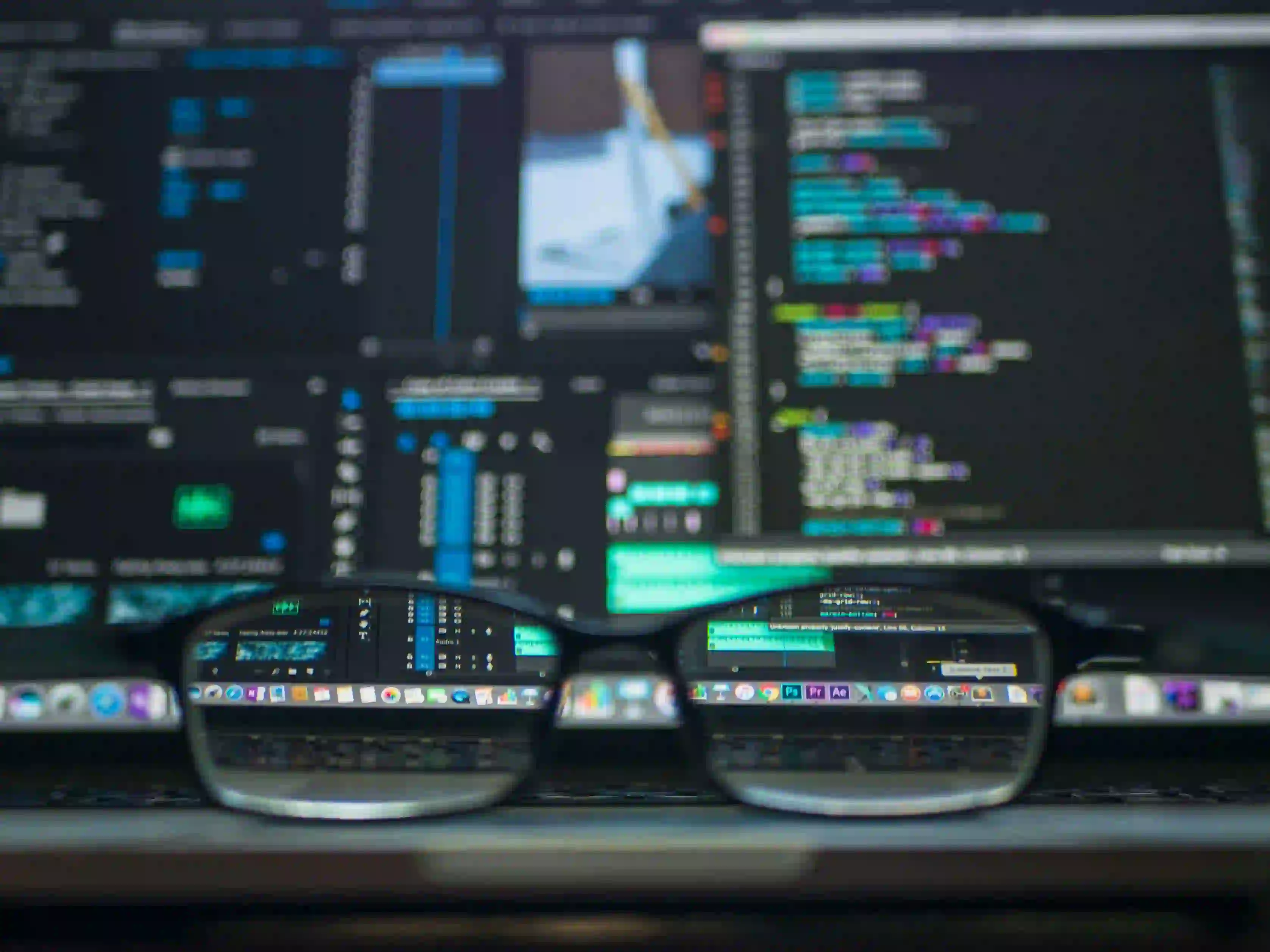
Mastering Java Development with Node.js Version Compatibility
In today's fast-paced world of software development, combining different technologies to build powerful applications is commonplace. Java, known for its portability, speed, and robust features, often aligns with Node.js, which excels in handling asynchronous operations and server-side scripting. The intertwining of these two languages raises a significant question: how do we ensure version compatibility?
In this blog post, we're diving into Java development and Node.js compatibility, exploring best practices, pitfalls, and solutions to maintain efficiency and performance. We'll draw insights from the existing article, "Taming Node.js Versions: Fish Shell's Common Pitfalls", which sheds light on the challenges of managing Node.js versions using Fish Shell.
The Need for Version Compatibility
When developing a Java application that relies on Node.js services, there are various factors at play. Different versions of Node.js may have varying features, bug fixes, or deprecated functions that could impact your Java application.
For example, consider that you've built a Java service that communicates with a Node.js REST API. If the Node.js service gets updated to a new version that removes a previously available endpoint, your Java application will start encountering errors like HTTP 404 Not Found.
The Purpose of Semantic Versioning
To mitigate such issues, understanding semantic versioning (semver) is crucial. Semantic versioning consists of three segments: MAJOR, MINOR, and PATCH.
- MAJOR: Incremented when there are incompatible API changes.
- MINOR: Incremented when functionality is added in a backward-compatible manner.
- PATCH: Incremented when backward-compatible bug fixes are introduced.
Hereβs a small diagram illustrating semantic versioning:
MAJOR.MINOR.PATCH
Ensuring Compatibility
1. Configuration management
The foremost step in ensuring version compatibility is effective configuration management. For Java developers using Node.js, managing configurations ensures that both environments operate within compatible constraints.
With the use of Docker, you can encapsulate your Node.js application in a container. This approach reduces inconsistencies caused by varying environments.
Here's a simple Dockerfile setup:
# Base Image
FROM node:14
# Set the working directory
WORKDIR /usr/src/app
# Copy package.json and install dependencies
COPY package*.json ./
RUN npm install
# Copy the application source code
COPY . .
# Expose application port
EXPOSE 3000
# Run the application
CMD ["node", "app.js"]
Using a specified version of Node in the base image (in this case, version 14) prevents discrepancies when deploying your application.
2. Use nvm for Node.js Management
When working with multiple projects, it's common to have different Node.js versions across those projects. Using nvm
(Node Version Manager) allows you to switch between Node.js versions effortlessly.
To install nvm
, follow the instructions on nvm's GitHub page. Once installed, run the following command to install a particular version:
nvm install 14.17.0
You can switch to your desired version with:
nvm use 14.17.0
By managing versions effectively, you lessen the chance of compatibility issues across different components of your application.
3. Update Dependencies Periodically
It's often tempting to defer dependency updates, especially in large applications. However, this can lead to major version incompatibilities down the line. Regularly updating your dependencies minimizes the risk of breaking changes.
When using a Java application with Node.js components, employing a Dependency Management Tool like Maven for Java and npm for Node.js will help you track and update dependencies efficiently.
Example Maven configuration to manage dependencies:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.4</version>
</dependency>
For the Node.js package.json:
{
"dependencies": {
"express": "^4.17.1"
}
}
Always refer to the official documentation for the latest updates.
Handling Errors and Fixes
Even with these measures, you may still encounter errors stemming from incompatible versions. In these situations, clear error handling in your Java application can shield users from crumbling experiences.
Consider the following Java code snippet to handle exceptions when interfacing with a Node.js API:
public void callNodeApi() {
try {
// Code to make a call to Node.js API
} catch (HttpClientErrorException e) {
// Handle 404 Not Found
System.out.println("Node.js service not found: " + e.getMessage());
}
// Additional error handling
}
This snippet captures errors like 404 Not Found, offering an opportunity to manage the application flow effectively.
Integration Testing
Automated integration testing is another key to ensuring compatibility among Java and Node.js versions. By running automated tests, you can quickly identify any potential issues arising from version changes.
Consider using tools such as JUnit for Java and Mocha for Node.js. Below is an example of a simplistic integration test in JUnit:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class NodeApiIntegrationTest {
@Test
public void testNodeApiResponse() {
// Your test invocation and assertions here
int responseStatus = getApiResponseStatus("http://localhost:3000/api/test");
assertEquals(200, responseStatus);
}
}
By ensuring that both systems can communicate effectively through comprehensive tests, you build a robust application resistant to versioning issues.
Lessons Learned
Combining Java with Node.js can lead to powerful applications, but version compatibility must be handled with utmost care. Through the use of semantic versioning, effective dependency management, and integration testing, we can mitigate compatibility issues and reduce downtime or broken functionalities.
As a developer, keeping abreast of Node.js changes and potential pitfalls is essential. For more detailed discussions on managing Node.js versions using the Fish shell, consider checking out the article titled "Taming Node.js Versions: Fish Shell's Common Pitfalls". Happy coding, and may your Java and Node.js projects flourish with seamless integration!