Mastering Java Environment Management: Avoiding Common Pitfalls
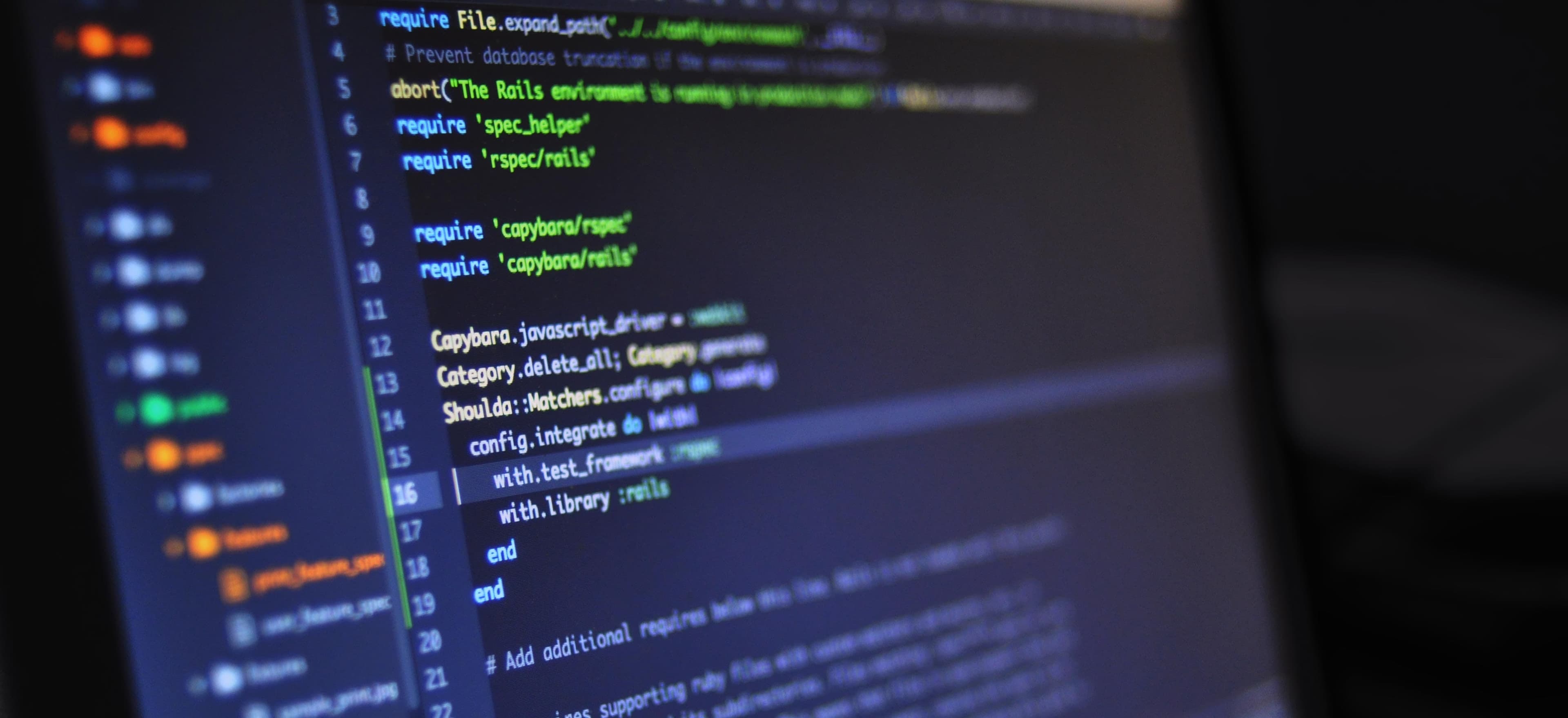
- Published on
Mastering Java Environment Management: Avoiding Common Pitfalls
Managing your Java environment effectively is crucial for any developer who wants to maintain productivity and avoid the frustration of incorrect configurations. In this blog post, we’ll dive into best practices for managing Java environments, discuss common pitfalls, and provide actionable tips to avoid them.
Understanding Java Environment Management
Java is a platform-independent programming language that runs on various operating systems. However, different projects may rely on different Java Development Kit (JDK) versions, libraries, and configurations. This is where Java environment management comes into play.
Using the correct environment setup ensures that your applications behave as expected and allows team members to collaborate without discrepancies arising from different configurations.
Key Components of Java Environment Management
-
Java Development Kit (JDK): The JDK includes the Java Runtime Environment (JRE) along with development tools. It’s crucial to use the correct JDK version for your project.
-
Build Tools: Tools like Maven and Gradle help manage dependencies, build processes, and project setups.
-
Environment Variables: Properly configured environment variables, such as
JAVA_HOME
, dictate which JDK version your projects will utilize.
Common Pitfalls in Java Environment Management
1. Inconsistent JDK Versions
Using different JDK versions across different projects can lead to compatibility issues. For instance, certain features in newer JDKs are not available in their older counterparts, leading to unexpected errors.
-
How to Avoid:
- Use a version manager like SDKMAN! to manage multiple JDK installations easily.
- Ensure that your project’s documentation specifies the required JDK version.
# Install a specific JDK version using SDKMAN! sdk install java 11.0.3-open
2. Not Setting JAVA_HOME
Failing to set the JAVA_HOME
environment variable can lead to issues when running Java applications or build tools that rely on it. The JAVA_HOME
variable tells your system where the JDK is installed.
- How to Set JAVA_HOME:
For Windows:
setx JAVA_HOME "C:\Program Files\Java\jdk-11.0.3"
setx PATH "%PATH%;%JAVA_HOME%\bin"
For Unix-like systems:
export JAVA_HOME=/usr/lib/jvm/java-11-openjdk-amd64
export PATH=$JAVA_HOME/bin:$PATH
3. Ignoring Dependency Management
One of the most significant challenges in Java development is managing dependencies efficiently. Ignoring versions or not specifying them correctly can lead to "Dependency Hell."
-
How to Avoid:
- Use Maven or Gradle for explicit dependency declarations. Let’s take a look at a simple example using Maven.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>example-app</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> <version>2.5.4</version> </dependency> </dependencies> </project>
4. Poorly Documented Environment Configuration
It's essential to have good documentation to ensure team members can set up their environments without pain.
- How to Improve Documentation:
- Create a README file in your project repository that includes setup instructions, required JDK versions, and any environment variables that need setting.
5. Not Testing Across Environments
Oftentimes, developers get caught up in their local configuration and forget to test deployments in different environments (staging, production).
- How to Mitigate Risks:
- Use Continuous Integration/Continuous Deployment (CI/CD) tools like Jenkins or GitHub Actions to test builds across various JDK versions and environments automatically.
Leveraging Java Build Tools
Utilizing build tools effectively can drastically reduce the headaches associated with environment management. Below is a quick overview of two popular tools:
Maven
Maven is primarily used for project management, compiling, and packaging Java applications. One key feature of Maven is the pom.xml
file, where you define dependencies, plugins, and various settings.
Gradle
Gradle is a flexible build tool that can handle projects of any scale. It combines the advantages of Maven with an easier scripting language (Groovy or Kotlin).
Example: Simple Gradle Build Script
plugins {
id 'java'
}
group 'com.example'
version '1.0-SNAPSHOT'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework:spring-core:5.3.10'
testImplementation 'junit:junit:4.12'
}
In Conclusion, Here is What Matters
In conclusion, managing Java environments does not have to be an arduous task. By following best practices like using version managers for JDK installations, maintaining a well-documented process, leveraging build tools, and ensuring consistent environments across different setups, you can significantly reduce pitfalls.
If you want to further enhance your understanding of environment management in programming contexts, check out this insightful article titled Taming Node.js Versions: Fish Shell's Common Pitfalls. While it focuses on Node.js, the concepts around version management and pitfalls are universal.
Implement these strategies to streamline your Java development process, and keep your focus on writing code instead of managing environments. Happy coding!
Checkout our other articles