Overcoming Dynamic Content Challenges in Java Frameworks
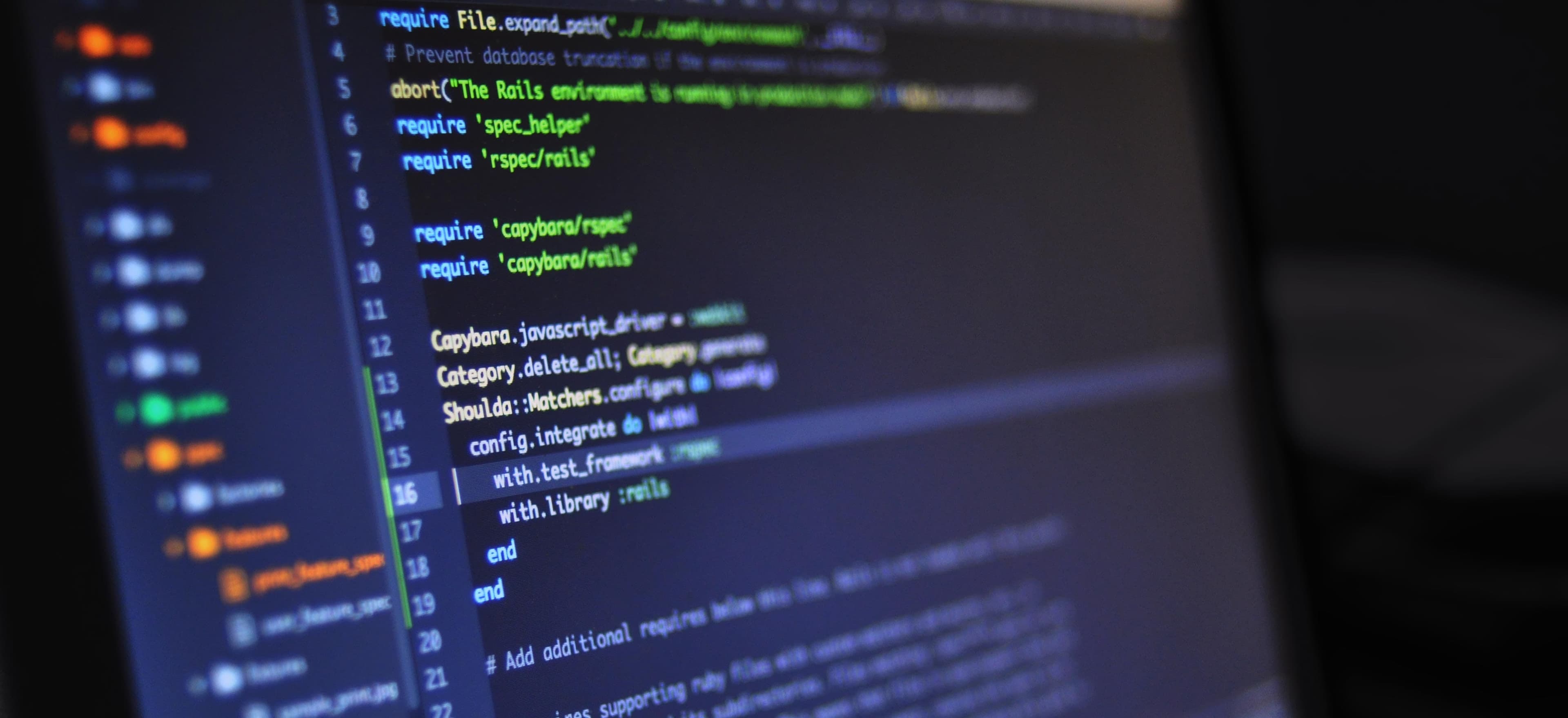
- Published on
Overcoming Dynamic Content Challenges in Java Frameworks
Java frameworks are pivotal in developing robust applications, especially when dealing with dynamic content. As we dive into the world of Java, we’ll explore various strategies for overcoming challenges associated with dynamic content presentation and management.
Dynamic content refers to information on a webpage that changes based on user interactions, preferences, or other factors. The ability to create dynamic content can enhance user experiences tremendously. However, it also brings along several challenges that developers must navigate.
In this guide, we will discuss various Java frameworks suitable for dynamic content management, common problems developers face, and effective solutions. Let's dive deeper.
Key Java Frameworks for Dynamic Content
Java has a plethora of frameworks. Here are some prominent ones you should consider for managing dynamic content:
-
Spring MVC: Ideal for building web applications. Its powerful Model-View-Controller architecture separates application logic from content, making it easier to update and maintain.
-
JSF (JavaServer Faces): A component-based framework that simplifies the development of user interfaces for Java web applications.
-
Hibernate: While primarily an Object-Relational Mapping (ORM) tool, it can also help manage the dynamic data that flows in and out of your web applications.
Why Choose These Frameworks?
Choosing a Java framework boils down to the project requirements. However, frameworks like Spring MVC and JSF provide more than just flexibility. They facilitate rapid development through features like dependency injection, aspect-oriented programming, and comprehensive convention over configuration principles.
Common Dynamic Content Challenges
Working with dynamic content often brings about specific challenges:
- State Management: Keeping track of user interactions and updates without reloading the state every time.
- Performance Issues: Loading dynamic content efficiently, especially with heavy data sets.
- Security Concerns: Ensuring that dynamic content is safe from SQL injections and other vulnerabilities.
- Data Binding: Connecting models to views seamlessly while maintaining responsiveness.
Let’s discuss these challenges in detail and provide strategies to overcome them.
1. State Management
State management is imperative when developing dynamic applications. Here’s how you can tackle state management:
Use Sessions
Java’s built-in session management can help you keep track of user data. For instance, in a Spring application, you can use the following approach:
@Controller
public class UserController {
@PostMapping("/login")
public String login(@RequestParam String username, HttpSession session) {
session.setAttribute("username", username);
return "redirect:/home";
}
}
Why this works: By storing the username in the session, you can retain information across multiple requests without needing a database call each time.
Store State in Databases
For long-lived state management, using a database to store user states can be effective. Combined with an ORM like Hibernate, this becomes more straightforward.
@Entity
public class UserSession {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private LocalDateTime created;
// Getters, setters, and constructors
}
Why this works: Storing user sessions allows for persistence across server restarts, ensuring user data is not lost.
2. Performance Issues
Dynamic content can bog down your application. Here are effective strategies to boost performance:
Lazy Loading
Use lazy loading to load data only when required. In Hibernate, you can annotate collections to achieve this:
@Entity
public class User {
@OneToMany(mappedBy = "user", fetch = FetchType.LAZY)
private List<Post> posts;
}
Why this works: Fetching data on-demand prevents unnecessary database calls, speeding up the initial loading time.
Caching
Implement caching to reduce the number of database hits:
@Cacheable("userData")
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
Why this works: Caching common requests dramatically speeds up data access and improves application responsiveness.
3. Security Concerns
Dynamic applications often face security vulnerabilities. Here are some tips to mitigate risks:
Input Validation
Always validate user inputs to thwart SQL injections.
@Query("SELECT u FROM User u WHERE u.username = :username")
User findByUsername(@Param("username") String username);
Why this works: Using parameterized queries, like the one above, ensures that user input does not compromise your SQL commands.
Use Secure Protocols
To protect data in transit, utilize HTTPS as opposed to HTTP, securing the connection between the client and server.
4. Data Binding
Developing a seamless model-view binding is vital in dynamic applications. Frameworks like Spring provide simple annotations for this purpose.
Model-View Binding in Spring
By employing @ModelAttribute
, the data from the form is automatically mapped to your Java model.
@PostMapping("/register")
public String register(@ModelAttribute User user) {
userService.save(user);
return "redirect:/success";
}
Why this works: This approach reduces boilerplate code and increases maintainability by relying on framework capabilities.
Additional Resources
To delve deeper into dynamic content management, understanding the nuances of handling data can be greatly beneficial. A resource worth exploring is the article titled Mastering Dynamic Content: Variable GridView Challenges, which addresses data manipulation frameworks like GridViews in Java applications. You can read more about it here.
My Closing Thoughts on the Matter
In conclusion, mastering dynamic content in Java is essential for modern web development. By selecting the right framework and employing strategies such as session management, lazy loading, effective security measures, and seamless data binding, you can build applications that are efficient and user-friendly.
As you progress, remember to stay updated with the latest practices in both Java and web development. Every challenge can be transformed into an opportunity with the right approach and knowledge.
By embracing these principles, you will effectively overcome the hurdles of dynamic content and build responsive, secure, and high-performing applications. Happy coding!
Checkout our other articles