Clarifying Java String Concatenation: "a-b" vs "a-a-b"
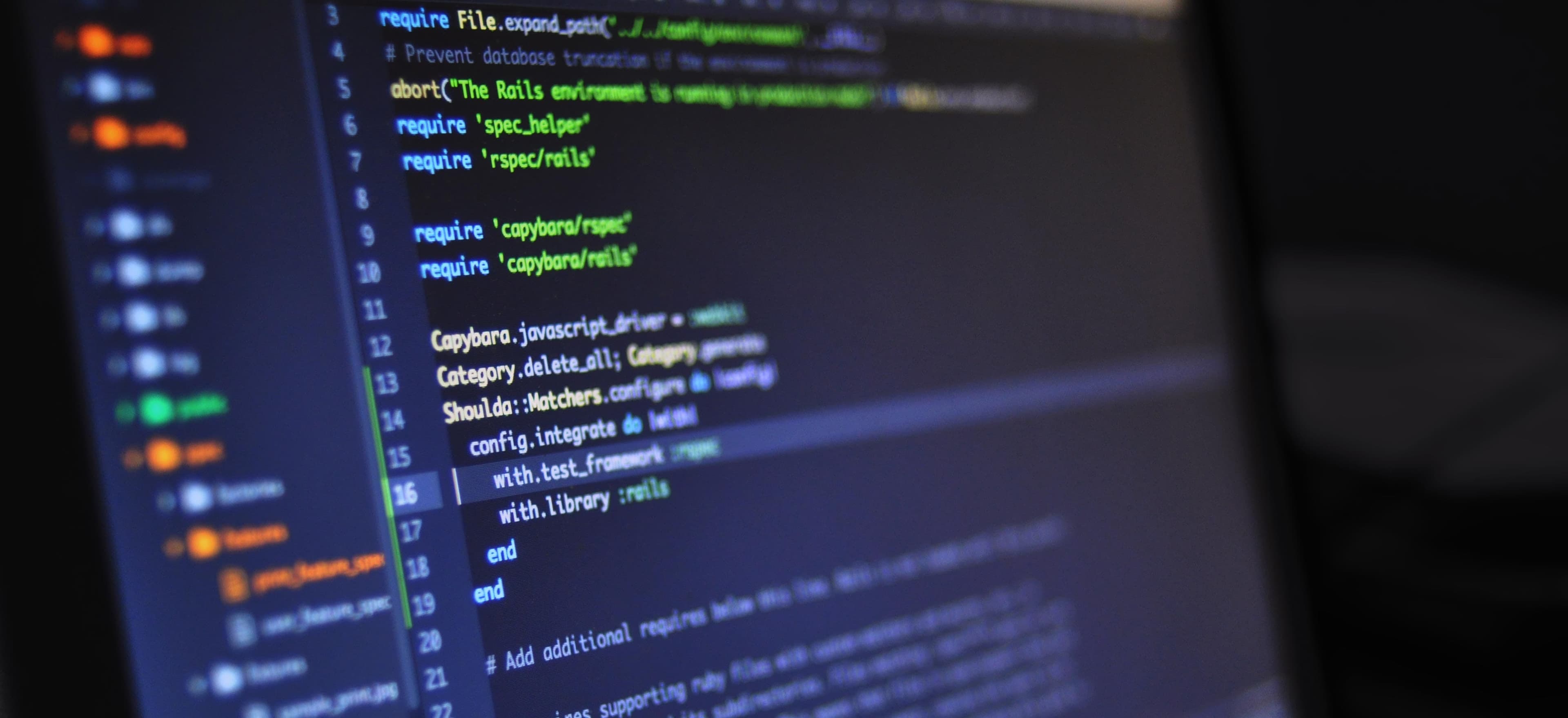
- Published on
Clarifying Java String Concatenation: "a-b" vs "a-a-b"
In the world of Java programming, string manipulation is a fundamental concept. Strings form the backbone of many applications, and understanding their concatenation is essential for effective coding. One area that often leads to confusion is the distinction between concatenated strings, particularly when we note that "a-b" is not equivalent to "a-a-b". This blog post will explore the intricacies of string concatenation in Java, elaborate on how it works, and clarify why these two expressions yield different results.
Understanding String Concatenation
String concatenation is the process of joining two or more strings together. In Java, this can be achieved using the +
operator or the String.concat()
method. It's helpful to understand both methods so that you can choose the appropriate one based on your code context.
Using the +
Operator
The +
operator is the most common way to concatenate strings in Java. Here’s a simple example:
public class StringConcatenation {
public static void main(String[] args) {
String part1 = "Hello";
String part2 = "World";
String result = part1 + " " + part2;
System.out.println(result); // Outputs: Hello World
}
}
Why Use the +
Operator?
The +
operator is both intuitive and easy to use. It makes the code cleaner and easier to understand at a glance. However, for extensive concatenations, it can create multiple String objects, which is something to consider for performance. For such scenarios, using StringBuilder
is recommended.
Using the String.concat()
Method
Another way to concatenate strings is with the String.concat()
method:
public class StringConcatenation {
public static void main(String[] args) {
String part1 = "Java";
String part2 = "Rules";
String result = part1.concat(" ").concat(part2);
System.out.println(result); // Outputs: Java Rules
}
}
Why Use String.concat()
?
Using String.concat()
can be more explicit about your intent of concatenation, yet it is often seen as less readable than the +
operator for simple operations. It does exactly what it says, but it may not always be the preferred choice when readability is paramount.
The Case of "a-b" vs "a-a-b"
Now, let’s focus on the variation between "a-b" and "a-a-b". Essentially, we're dealing with two concatenated string operations here.
- "a-b": This is a straightforward concatenation—combining the strings "a" and "b" with a hyphen in between.
- "a-a-b": This combines "a" with itself and adds "b" after the second "a".
Let’s look at this with code examples to make the distinction clearer.
Example Code Snippet
public class StringComparison {
public static void main(String[] args) {
String str1 = "a-b";
String str2 = "a" + "-" + "b";
String str3 = "a-a-b";
System.out.println("Is str1 equals to str2? : " + str1.equals(str2)); // true
System.out.println("Is str1 equals to str3? : " + str1.equals(str3)); // false
}
}
Output Explanation:
- The comparison
str1.equals(str2)
evaluates totrue
because both strings are identical. - However,
str1.equals(str3)
evaluates tofalse
because "a-a-b" contains an additional "a" and thus does not match "a-b".
This distinction highlights an essential programming principle: what you think you are concatenating may not represent the actual outcome.
Pitfalls to Avoid when Concatenating Strings
Even experienced developers can fall victim to common pitfalls when it comes to string concatenation. Below are a few tips to help you stay on track:
- Avoid Overusing
+
in Loops: If you are concatenating strings within a loop, consider usingStringBuilder
instead. It's more efficient in terms of memory and processing.
public class EfficientConcat {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("Iteration ").append(i).append("\n");
}
System.out.println(sb.toString());
}
}
-
Watch for Null Values: When concatenating, ensure none of the strings are null, as they will cause a
NullPointerException
. -
Understand Immutability: Remember that in Java, strings are immutable. Each time you concatenate strings, a new string is created, which may lead to performance issues.
-
String Interpolation: Java doesn’t have built-in string interpolation like some other languages. Always utilize concatenation or
String.format()
for inserting variables efficiently.
Additional Resources
For those who wish to delve deeper into understanding string concatenation and related concepts, I recommend reading the article titled Understanding the Confusion: Why "a-b" Isn't "a-a-b" on InfiniteJS. It provides valuable insights on nuances often overlooked by Java developers.
Final Considerations
String concatenation in Java is a basic yet essential skill for any developer. By understanding the difference between expressions like "a-b" and "a-a-b", you’ll avoid confusion and code errors in your programs. Focus on how you concatenate strings, be mindful of your choices, and utilize the methods best suited for your needs. Whether you prefer the +
operator for simplicity or StringBuilder
for performance, knowing when to use each can make a significant difference in your application's efficiency.
Remember, coding is as much about being precise as it is about being creative. Happy coding!
Checkout our other articles