Decoding Java's Method Overloading Confusion: "a-b" vs "a-a-b"
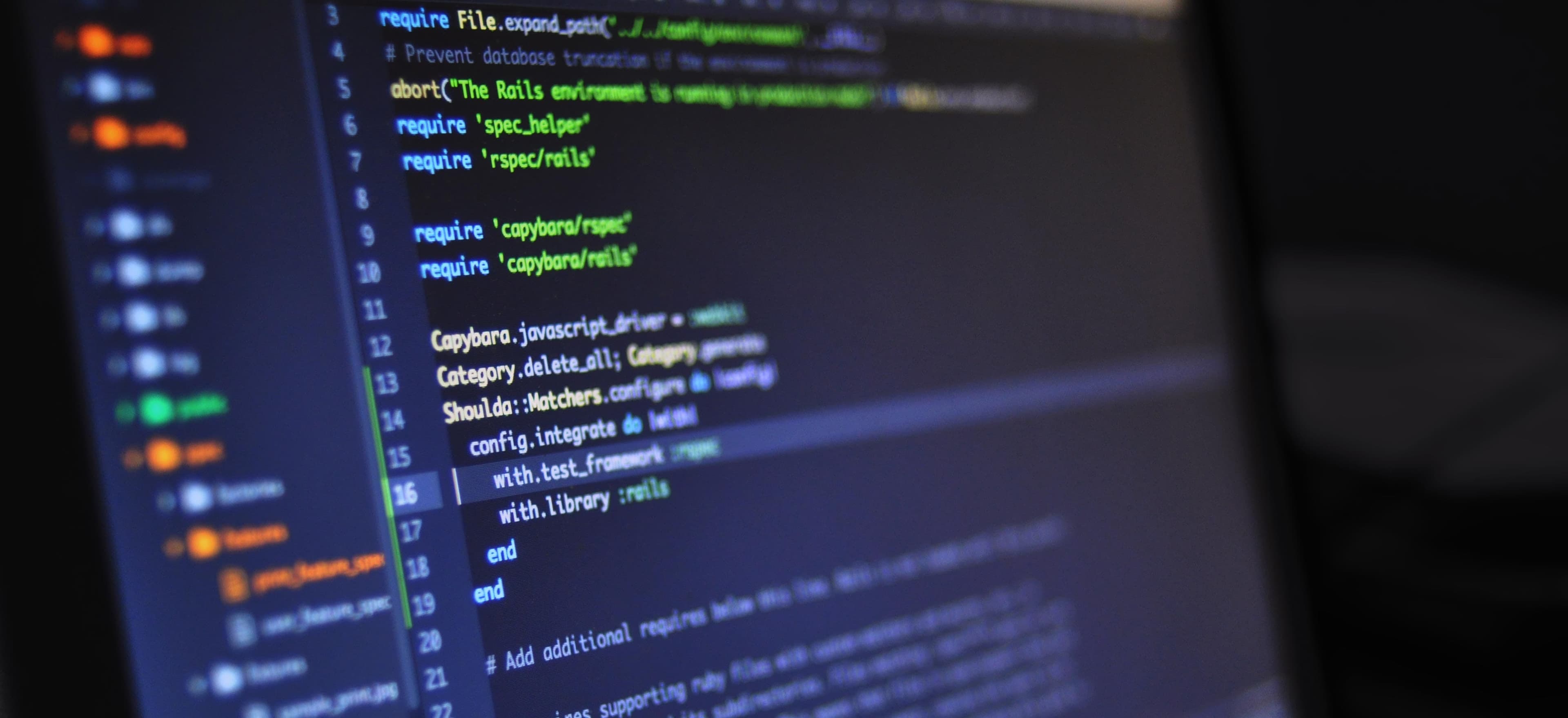
- Published on
Decoding Java's Method Overloading Confusion: "a-b" vs "a-a-b"
Java is a powerful programming language that offers developers a plethora of features to enhance code efficiency and readability. One of its standout capabilities is method overloading, which allows multiple methods to coexist with the same name, as long as they have different parameters. But seemingly simple concepts can lead to great confusion, especially when terms akin to "a-b" and "a-a-b" are tossed around. Today, we'll crack this code while guiding you through the ins and outs of method overloading in Java.
What is Method Overloading?
Method overloading in Java allows you to declare multiple methods with the same name but different parameters (the number or the type of parameters). This feature enhances the readability of the code and can lead to a more straightforward API design. For example, you might have a method add
that operates differently depending on whether you pass it integers, doubles, or strings.
Why Use Method Overloading?
- Enhanced Readability: When methods have the same purpose but handle different data types or parameter counts, it makes the codebase easier to understand.
- Improved Code Reusability: You can provide multiple implementations without creating new method names.
- Flexibility: Developers can provide the capability to call a method with different parameters, facilitating versatile interactions within the code.
Example of Method Overloading in Java
Let's look at an example to clarify the concept.
public class MathOperations {
// Method to add two integers
public int add(int a, int b) {
return a + b;
}
// Method to add three integers
public int add(int a, int b, int c) {
return a + b + c;
}
// Method to add two doubles
public double add(double a, double b) {
return a + b;
}
// Method to concatenate strings
public String add(String a, String b) {
return a + b;
}
}
In this MathOperations
class, the add
method is overloaded with four versions, each tailored to different parameter types or counts.
Commentary on the Example
- Flexibility in Operations: By offering various
add
implementations, the method caters to the needs of both numerical and string manipulations. - Readability and Maintainability: Despite the varied functionalities, the naming convention remains consistent, which intuitively communicates the methods' purpose.
Understanding the "a-b" vs "a-a-b" Confusion
In the world of Java, method overloading can sometimes cause confusion, especially when method signatures appear similar. Let's delve into the specifics of "a-b" vs "a-a-b" and how they relate to method overloading.
Breaking Down the Confusion
To clarify, let's use a relatable analogy: think of "a-b" as a representation of two parameters, and "a-a-b" as three parameters where one type is repeated. While the intuition might suggest they belong to the same category, they distinctly represent different method overloads.
Example Scenario
Consider a scenario where you have two classes:
class Employee {
String name;
int age;
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
}
class Manager extends Employee {
int teamSize;
public Manager(String name, int age, int teamSize) {
super(name, age);
this.teamSize = teamSize;
}
// Overloaded constructor for Manager
public Manager(String name, int age) {
this(name, age, 5); // Default team size
}
}
In this case, the Manager
class has two constructors. The first includes three parameters, while the second only requires two, effectively embodying the "a-b" and "a-a-b" paradigms.
- "a-b" (two parameters): When a
Manager
is created with just a name and age. - "a-a-b" (three parameters): When a
Manager
is instantiated with a name, age, and specific team size.
This subtlety highlights the mechanism of constructor overloading, which operates under the same principle as method overloading.
Code Example: Method Overloading with "a-b" or "a-a-b"
Let's create a method that demonstrates method overloading:
public class ShapeAreaCalculator {
// Method to calculate area of a rectangle
public double calculateArea(double length, double breadth) {
return length * breadth;
}
// Method to calculate area of a square
public double calculateArea(double side) {
return side * side;
}
// Overloaded method to calculate area of a triangle
public double calculateArea(double base, double height, String type) {
return 0.5 * base * height; // Triangle Area
}
}
Commentary on the Overloaded Methods
- Different Parameter Types: Here, we can see "a-b" in
calculateArea(double length, double breadth)
, which takes two parameters for a rectangle. - Distinct Functional Roles: While
calculateArea(double side)
represents a square, the triangle area method incorporates a string type parameter, showcasing further flexibility.
Wrapping Up
With Java's method overloading capabilities, you can create robust applications that are easy to read and maintain. The distinction between "a-b" and "a-a-b" serves as a reminder of the importance of method signatures in managing overloading effectively.
To unpack this further about overloaded methods and alleviate some of the confusion surrounding the topic, I highly recommend checking out "Understanding the Confusion: Why 'a-b' Isn't 'a-a-b'" at infinitejs.com/posts/understanding-a-b-not-aab.
Key Takeaways
- Consistency in Naming: Employ consistent naming conventions to ascertain clarity in overloaded methods.
- Parameter Types Matter: Different parameter types or counts lead to the creation of different overloaded methods.
- Keep Learning: Java is a complex language, and delving deeper into concepts like overloading will enhance your programming proficiency.
It’s time to harness the full potential of method overloading in your Java projects! Happy coding!
Checkout our other articles