Tackling Dynamic Grids in Java: Best Practices for JavaFX
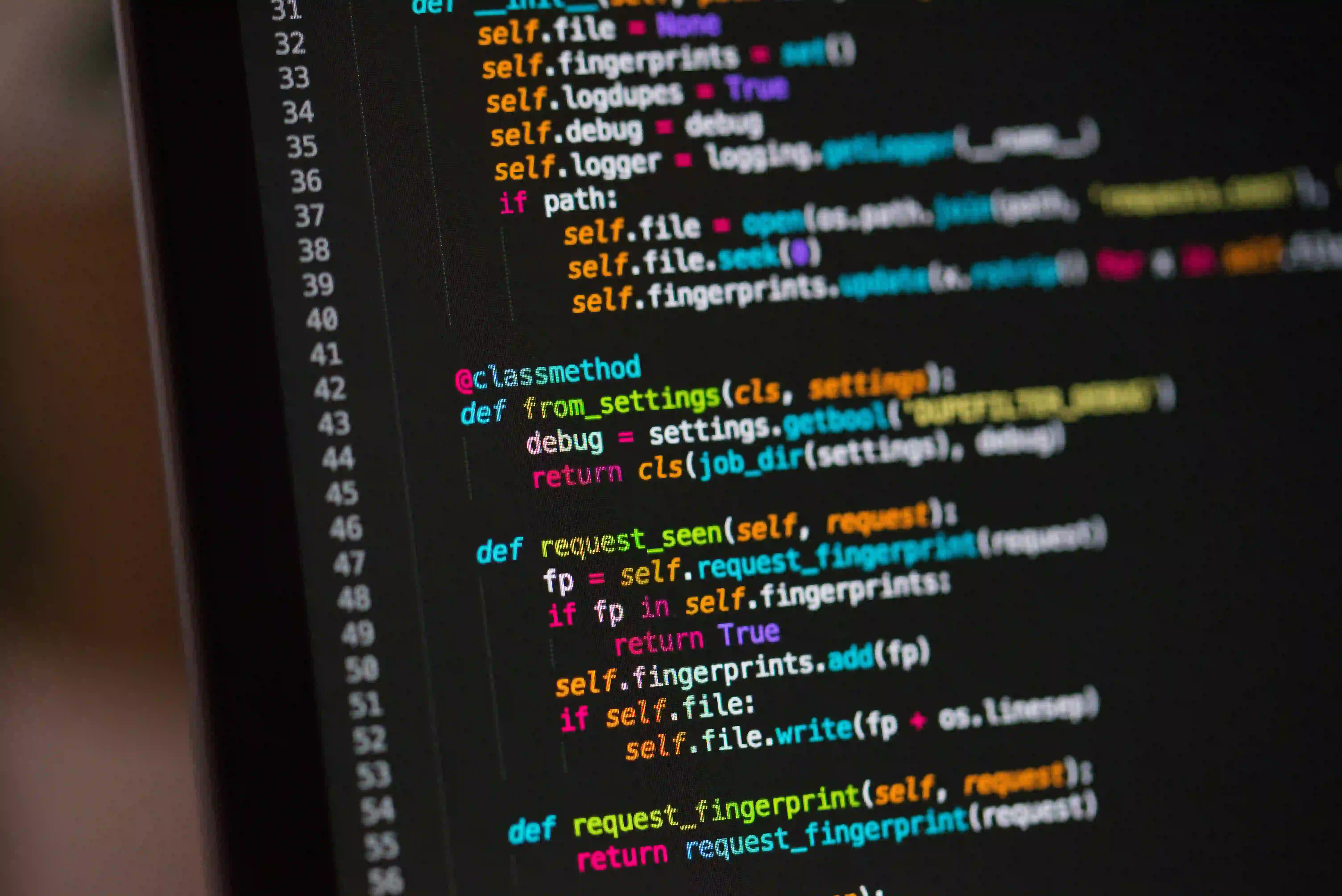
Tackling Dynamic Grids in Java: Best Practices for JavaFX
Dynamic content management is one of the essential features in modern application development, and JavaFX offers robust tools to deal with it effectively. In this post, we will explore best practices for managing dynamic grids in JavaFX while discussing techniques that enhance user experience and maintain performance.
Understanding Dynamic Grids in JavaFX
Dynamic grids are layouts that can change based on user actions or data input. They can be tricky to handle without the appropriate design and structure, especially when elements need to be added, removed, or updated regularly. In JavaFX, GridPane
is commonly used for creating such dynamic layouts.
To understand how to manage dynamic content effectively, you might want to check out Mastering Dynamic Content: Variable GridView Challenges, which discusses grid challenges and caching strategies that can apply to JavaFX as well.
Setting Up Your JavaFX Application
Before diving into dynamic grids, let’s ensure your JavaFX environment is correctly set up. You will need the Java Development Kit (JDK) and a JavaFX library. Here’s how to include JavaFX in your project if you are using Maven:
<dependencies>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>17.0.0</version>
</dependency>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-fxml</artifactId>
<version>17.0.0</version>
</dependency>
</dependencies>
This snippet configures your project to use JavaFX controls and FXML, laying the foundation for creating dynamic user interfaces.
Creating a Simple Dynamic Grid
Now, let’s create a basic dynamic grid. The following code snippet demonstrates a simple GridPane
setup where you can dynamically add buttons to the grid.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class DynamicGridExample extends Application {
private int row = 0;
private int col = 0;
@Override
public void start(Stage primaryStage) {
GridPane gridPane = new GridPane();
Button addButton = new Button("Add Button");
addButton.setOnAction(e -> addButtonToGrid(gridPane));
gridPane.add(addButton, 0, 0);
Scene scene = new Scene(gridPane, 400, 400);
primaryStage.setScene(scene);
primaryStage.setTitle("Dynamic Grid Example");
primaryStage.show();
}
private void addButtonToGrid(GridPane gridPane) {
Button newButton = new Button("Button " + (row * 2 + col + 1));
gridPane.add(newButton, col, row + 1);
col++;
if (col >= 2) { // Reset column after a certain number of columns
col = 0;
row++;
}
}
public static void main(String[] args) {
launch(args);
}
}
Explanation
-
Threading with JavaFX: The
start
method initializes the JavaFX application. It's important that any UI updates are handled on the JavaFX Application Thread. -
Dynamic Addition of Buttons: The
addButtonToGrid
method lets users add buttons as needed. When the button is clicked, it checks column bounds. If too many buttons are in one row, it resets the column to0
and increments the row counter. -
Grid Layout: GridPane is fully customizable. You can control the placement and size of each node, enabling responsive designs.
Efficient Handling of Dynamic Data
Now that your grid is set up, you want to manage data more efficiently. Understanding how to handle dynamic data input is crucial in developing responsive applications.
Using ObservableList
JavaFX provides several properties and collections tailored for the UI. The ObservableList
interface automatically updates the UI when items are added or removed. Below is an example of using ObservableList
with a dynamic grid.
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ListView;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class DynamicGridWithListView extends Application {
private ObservableList<String> items = FXCollections.observableArrayList();
@Override
public void start(Stage primaryStage) {
GridPane gridPane = new GridPane();
ListView<String> listView = new ListView<>(items);
Button addButton = new Button("Add Item");
addButton.setOnAction(e -> {
items.add("Item " + (items.size() + 1));
listView.setItems(items);
});
gridPane.add(listView, 0, 0);
gridPane.add(addButton, 1, 0);
Scene scene = new Scene(gridPane, 400, 400);
primaryStage.setScene(scene);
primaryStage.setTitle("Dynamic Grid with ObservableList");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Explanation
-
ObservableList: This collection allows the GridPane's ListView to automatically update whenever items are added. It's particularly useful in dynamic grids.
-
User Interaction: The add button allows users to interact and modify the content. Each click adds a new item to the list, showcasing how data binding works dynamically within JavaFX components.
Best Practices for Performance
When working with dynamic grids in JavaFX, consider these best practices to ensure optimal performance:
-
Limit Redraws: Only update what needs to change; avoid full redraws as they can be computationally expensive.
-
Use Virtualization: For large data sets, consider using
ListView
orTableView
, which supports virtualization. This means only the elements currently in the viewport are rendered, significantly improving performance. -
Control Layout Updates: When modifying your grid, batch updates to reduce the number of layout passes. This can be achieved by disabling layout updates while making multiple changes.
-
Lazy Loading for Large Datasets: Implement lazy loading techniques to display data in chunks. This approach improves initial load times and enhances user experience.
Final Thoughts
Dynamic grids can significantly enhance how users interact with your JavaFX applications. By implementing best practices like using the GridPane
, effectively managing data with ObservableList
, and ensuring optimal performance, you can create responsive and dynamic interfaces.
If you are interested in a deeper dive into handling dynamic content, don’t forget to check out Mastering Dynamic Content: Variable GridView Challenges for additional frameworks and caching strategies that might be beneficial for your projects.
With these insights into JavaFX dynamic grids, you are well-equipped to tackle user interface challenges with confidence. Explore, implement, and watch your applications thrive!
Additional Resources
Happy coding!