Mastering Java Frameworks: Tackling Change Detection Issues
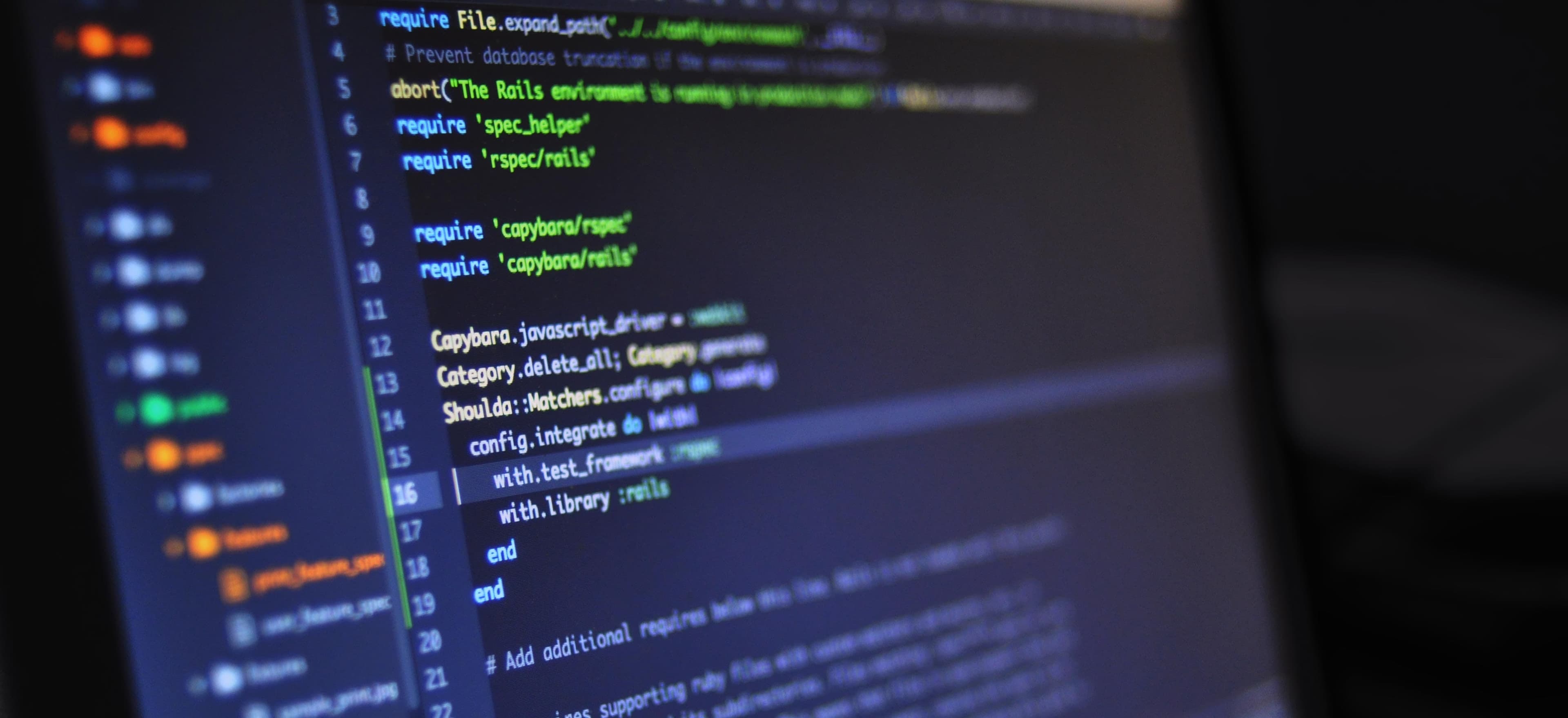
- Published on
Mastering Java Frameworks: Tackling Change Detection Issues
In the realm of software development, change detection is a crucial aspect, particularly when working with reactive frameworks. In the Java ecosystem, frameworks like Spring and JavaFX present unique challenges in managing application state and ensuring updates propagate correctly. In this article, we will explore how to tackle change detection issues in these frameworks, drawing parallels to some insights gained from the article titled Demystifying Angular: Solving Change Detection Mysteries.
Understanding Change Detection
Change detection refers to the mechanism by which a framework determines when an observable state has changed and subsequently reflects these changes within the user interface (UI). In modern applications, efficient change detection is essential for performance and a smooth user experience.
Why is this important? When developing a responsive application, ensuring that UI elements reflect the current state without unnecessary re-renders is vital. Poor change detection can lead to various problems such as performance degradation, stale data presentation, or even application crashes.
Change Detection in Java: The Spring Framework
The Spring Framework provides a robust environment for building enterprise applications. However, it comes with its own nuances when it comes to changes in state. Understanding how to manage change detection effectively is key.
1. The Observer Design Pattern
Spring utilizes the Observer design pattern, allowing components to observe changes in state. This means that when one component changes, other components that have registered to listen to these changes can react accordingly. However, developers need to ensure loose coupling between components to avoid an overly interdependent system.
Example Code Snippet
Here is a simple implementation showing how to observe changes within a Spring application:
import org.springframework.context.ApplicationListener;
import org.springframework.stereotype.Component;
// Event to signal changes
class ChangeEvent {
private String message;
public ChangeEvent(String message) {
this.message = message;
}
public String getMessage() {
return message;
}
}
// Listener for handling change events
@Component
class ChangeEventListener implements ApplicationListener<ChangeEvent> {
@Override
public void onApplicationEvent(ChangeEvent event) {
System.out.println("Received change event - message: " + event.getMessage());
}
}
// Triggering an event
@Component
class ChangeEventPublisher {
private final ApplicationEventPublisher publisher;
public ChangeEventPublisher(ApplicationEventPublisher publisher) {
this.publisher = publisher;
}
public void publish(String message) {
ChangeEvent event = new ChangeEvent(message);
publisher.publishEvent(event);
}
}
Why this code matters: This code demonstrates how to decouple event listeners from event publishers. By using Spring's built-in eventing mechanism, we can effectively handle changes without cluttering the application's business logic.
2. Handling State Changes with @Scheduled
Sometimes, state changes happen in the background and should be pushed to the UI. In such cases, Spring's @Scheduled
annotation can become handy.
Example Code Snippet
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
class StateChangeScheduler {
private String state = "Initial State";
@Scheduled(fixedRate = 5000)
public void checkAndUpdateState() {
// Simulate a background state change
state = "Updated State - " + System.currentTimeMillis();
System.out.println("State changed: " + state);
}
}
Why this code matters: This snippet shows how the @Scheduled
annotation can be employed to manipulate state based on predefined intervals. Such periodic checks can keep the application state in sync with backend services or user actions.
Working with JavaFX
JavaFX, a powerful framework for building desktop applications, also has change detection mechanisms, particularly through property binding.
1. Property Binding
JavaFX uses a property-based system which allows for automatic updates to UI components. This can simplify the change detection process significantly.
Example Code Snippet
import javafx.application.Application;
import javafx.beans.property.SimpleStringProperty;
import javafx.beans.property.StringProperty;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ChangeDetectionDemo extends Application {
private final StringProperty userInput = new SimpleStringProperty();
@Override
public void start(Stage primaryStage) {
Label label = new Label();
TextField textField = new TextField();
// Bind label text to user input
label.textProperty().bind(userInput);
// Update user input as the text field changes
textField.textProperty().addListener((observable, oldValue, newValue) -> {
userInput.set(newValue);
});
VBox vbox = new VBox(textField, label);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why this code matters: This example illustrates how easy it is to manage state changes using JavaFX’s property binding. The UI automatically updates when the underlying data changes, thanks to the listener on the TextField
.
2. Observable Collections
JavaFX also supports observable collections, which notify listeners about any modifications.
Example Code Snippet
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.collections.ListChangeListener;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ListView;
import javafx.scene控制控制.controls.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ObservableListDemo extends Application {
@Override
public void start(Stage primaryStage) {
ObservableList<String> items = FXCollections.observableArrayList();
ListView<String> listView = new ListView<>(items);
Button button = new Button("Add Item");
button.setOnAction(e -> {
items.add("Item " + (items.size() + 1));
});
items.addListener((ListChangeListener<String>) c -> {
while (c.next()) {
if (c.wasAdded()) {
System.out.println("Added: " + c.getAddedSubList());
}
}
});
VBox vbox = new VBox(listView, button);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why this code matters: By using ObservableLists
, changes are tracked, and developers can respond to modifications. This is crucial when multiple components need to respond to the same data changes in the UI.
Final Thoughts
Navigating change detection within Java frameworks like Spring and JavaFX may initially seem daunting, but understanding the tools and techniques available can simplify these challenges. As illustrated through the examples, reactive programming principles and event-driven architectures can be integral to managing state effectively.
For further insights, you may also want to explore Demystifying Angular: Solving Change Detection Mysteries as it offers a different but equally relevant take on handling change detection—a concept that is universally important across various programming frameworks.
In mastering Java frameworks, remember that effective change detection not only streamlines your development process but also enriches the user experience significantly. Happy coding!
Checkout our other articles