Mastering Java Frameworks for Smooth WebGPU Integration
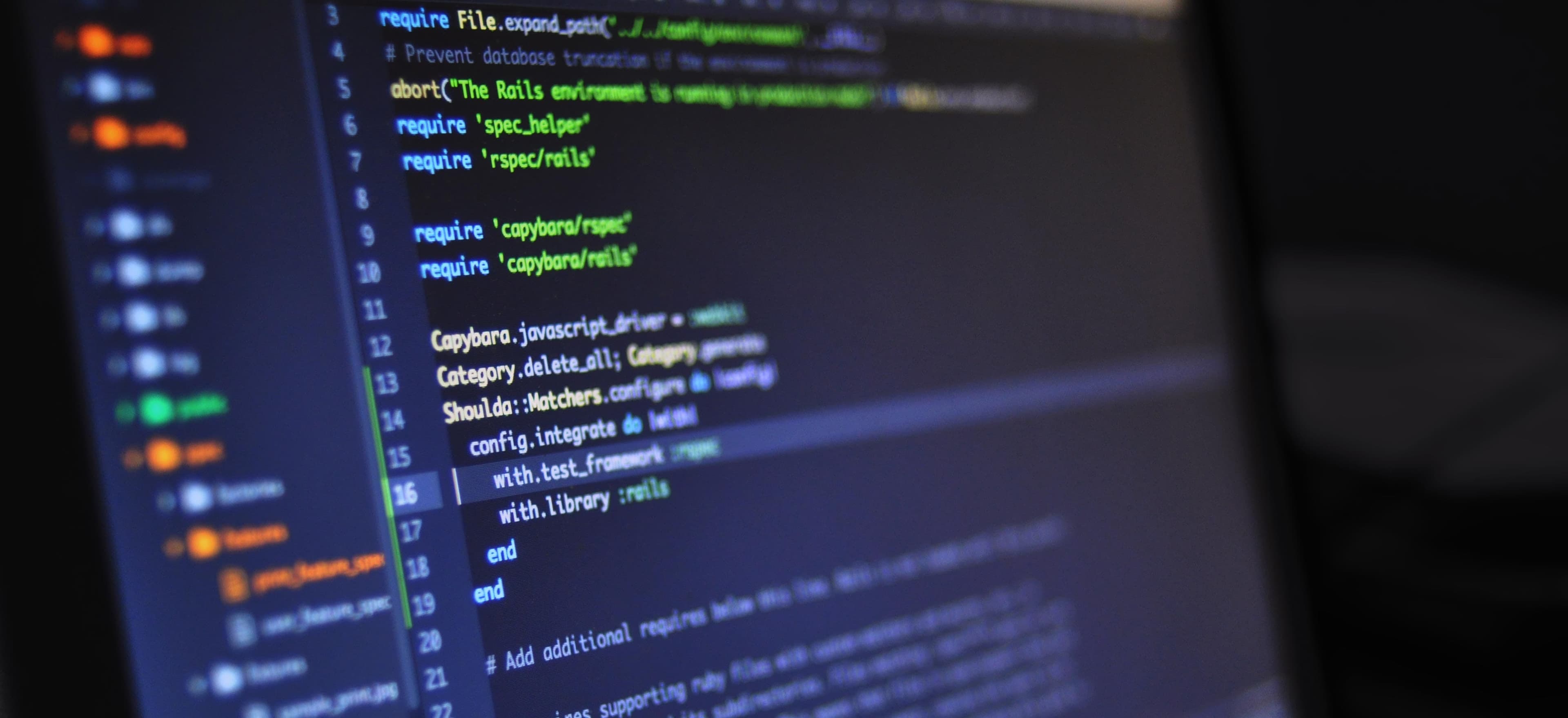
- Published on
Mastering Java Frameworks for Smooth WebGPU Integration
The world of web graphics has shifted dramatically from traditional rendering techniques to GPU-accelerated technologies. One key player in this transformation is WebGPU - a modern graphics API that provides high-performance graphics and computation capabilities for the web. However, integrating WebGPU into Java frameworks can present unique challenges. In this blog post, we'll dive into the best practices for achieving a smooth integration of WebGPU in your Java applications, while mastering some of the popular Java frameworks like Spring and JavaFX.
Understanding WebGPU: A Brief Overview
Before we explore how to integrate WebGPU with Java, let’s quickly recap what WebGPU is. Developed as a web standard, WebGPU allows developers to leverage the GPU for complex computations directly within web applications. Its low-level access to the GPU enables efficient processing and rendering.
For a detailed guide to addressing common issues with WebGPU integration, check out Overcoming WebGPU Canvas Integration Woes: A Guide.
Why Use Java for WebGPU Integration?
Java is a powerful, versatile programming language that is widely used in enterprise applications. Its stability and rich ecosystem make it an excellent choice for backend development. Furthermore, Java has frameworks that can efficiently manage complicated tasks, allowing you to focus on integrating WebGPU features with ease.
Key Java Frameworks for WebGPU Integration
In the context of WebGPU, a few Java frameworks stand out:
-
Spring Boot: This is a popular framework for creating stand-alone, production-ready applications. It simplifies configuration and deployment, making it ideal for complex web GPU applications.
-
JavaFX: Ideal for rich client applications, JavaFX can be seamlessly integrated with WebGPU for rendering and visualization.
Setting Up a Java Project with Gradle
Before diving into the integration, let's set up a basic Java project using Gradle. Gradle not only simplifies dependency management but also provides a smooth build process.
build.gradle:
plugins {
id 'application'
}
mainClassName = 'com.example.WebGPUApp'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.openjfx:javafx-base:19'
implementation 'org.openjfx:javafx-controls:19'
implementation 'org.openjfx:javafx-fxml:19'
// Add more dependencies as needed
}
Commentary
This configuration file specifies the JavaFX dependencies which we'll use later for our application. The mainClassName
indicates where the execution will start.
Implementing JavaFX for WebGPU Rendering
JavaFX provides a rich framework for building graphical user interfaces. Here’s an example of how to set up a simple JavaFX application that includes a canvas for rendering WebGPU graphics.
WebGPUApp.java:
package com.example;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.stage.Stage;
public class WebGPUApp extends Application {
@Override
public void start(Stage stage) {
stage.setTitle("WebGPU Integration with JavaFX");
// Create a canvas for rendering
Canvas canvas = new Canvas(800, 600);
GraphicsContext gc = canvas.getGraphicsContext2D();
setupCanvas(gc);
Group root = new Group();
root.getChildren().add(canvas);
stage.setScene(new Scene(root));
stage.show();
}
private void setupCanvas(GraphicsContext gc) {
// Perform initial rendering setup here
gc.setFill(Color.WHITE);
gc.fillRect(0, 0, 800, 600);
}
public static void main(String[] args) {
launch(args);
}
}
Commentary
In this JavaFX setup, we create a Canvas
where our WebGPU content will be rendered. The setupCanvas
method initializes the canvas with a white background. It's important to establish a clear visual foundation before any actual WebGPU rendering takes place.
Integrating a WebGPU Context
For rendering using WebGPU, you’ll need to create a web canvas element that is GPU-compatible. This process typically involves using Java’s interactiveness with JavaScript. Entering the realm of browser-based operations, we move into using Java’s ability to leverage JS interops via libraries like JxBrowser or similar.
Example using JxBrowser
Ensure you have added JxBrowser as a dependency:
dependencies {
implementation 'com.teamdev.jxbrowser:jxbrowser:7.19'
}
Embedding WebGPU in JavaFX:
package com.example;
import com.teamdev.jxbrowser.browser.Browser;
import com.teamdev.jxbrowser.browser.BrowserFactory;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class WebGPUAppWithJxBrowser extends Application {
private Browser browser;
@Override
public void start(Stage stage) {
stage.setTitle("WebGPU with JxBrowser");
browser = BrowserFactory.create();
browser.loadHtml(getHtmlForWebGPU());
Group root = new Group();
Scene scene = new Scene(root);
root.getChildren().add(browser.getView());
stage.setScene(scene);
stage.show();
}
private String getHtmlForWebGPU() {
return "<!DOCTYPE html><html>" +
"<body><canvas id='gpuCanvas'></canvas>" +
"<script src='path/to/webgpu.js'></script>" +
"<script>" +
"const canvas = document.getElementById('gpuCanvas');" +
"const context = canvas.getContext('webgpu');" +
" // Additional WebGPU rendering code goes here" +
"</script>" +
"</body></html>";
}
public static void main(String[] args) {
launch(args);
}
}
Commentary
This example integrates a web-based canvas with WebGPU functionality directly into a JavaFX application using JxBrowser. Here, we load HTML that contains the WebGPU code. This showcases the powerful capability of bridging Java and web technologies.
Creating a Seamless User Experience
When integrating WebGPU with Java, it’s paramount to ensure a seamless user experience. Here are some best practices to enhance your application:
-
Use Asynchronous Programming: Since WebGPU operations can be intensive, employing asynchronous programming helps keep the UI responsive.
-
Error Handling: Ensure your application gracefully handles errors, especially those arising from WebGPU API calls.
-
Performance Monitoring: Profile the rendering performance to optimize your WebGPU code and ensure smooth graphics.
A Final Look
Mastering the integration of WebGPU with Java frameworks opens up exciting possibilities for web-based graphics applications. By leveraging JavaFX for rich user interfaces and using JxBrowser or similar JS interop libraries, you can create stunning visual applications.
WebGPU represents a shift in how we think about rendering on the web. As you implement these strategies, keep exploring the robust capabilities of Java ecosystems and how they can enhance your graphical applications.
For more insights and straightforward solutions on common WebGPU challenges, don't forget to check the article: Overcoming WebGPU Canvas Integration Woes: A Guide.
While this is only the beginning of your journey in integrating WebGPU with Java frameworks, consider building on this knowledge to create diverse applications that tap into the power of the GPU.
Happy coding!
Checkout our other articles