Bridging Angular with Java: Tackling Change Detection Issues
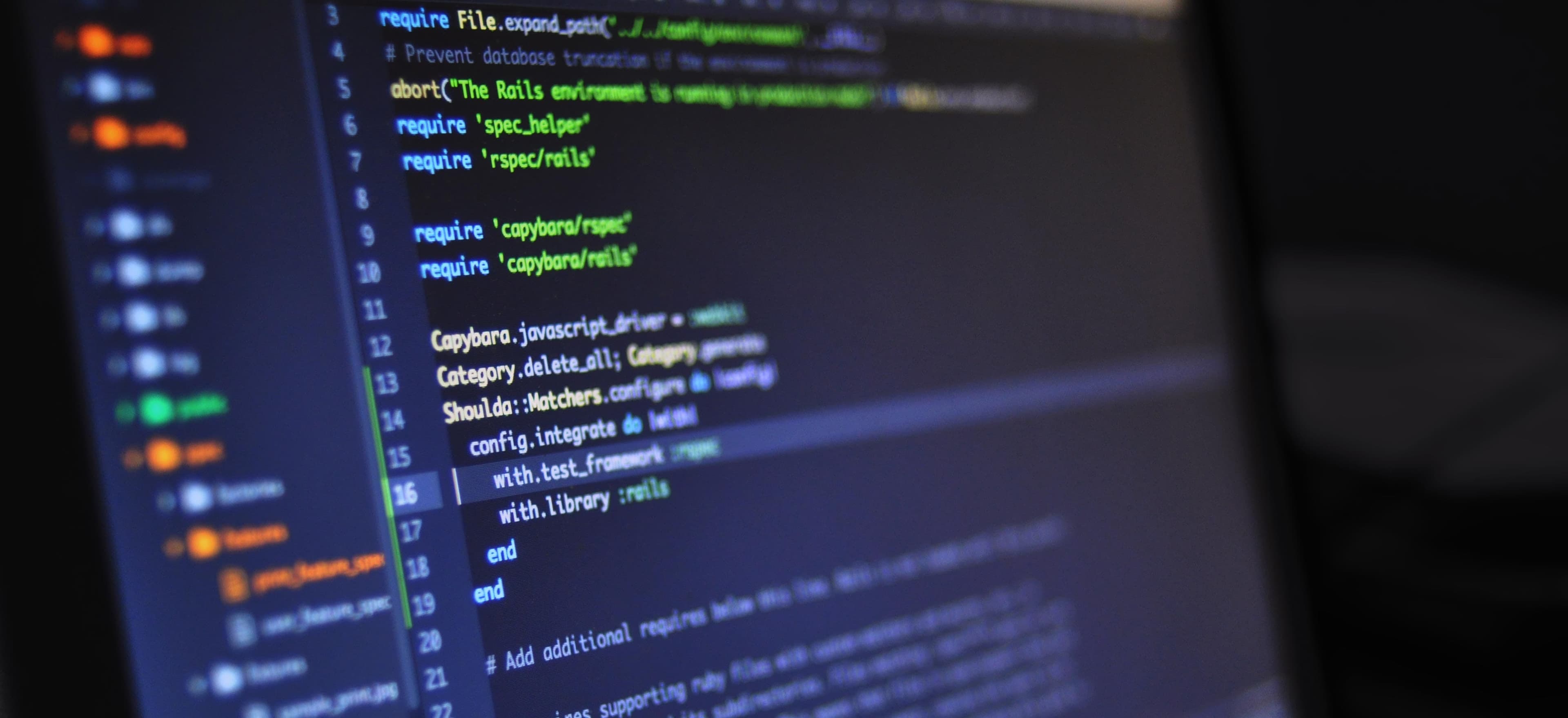
- Published on
Bridging Angular with Java: Tackling Change Detection Issues
In the landscape of modern web development, two powerful technologies—Angular and Java—often find themselves working together to create robust applications. This synergy, while potent, poses unique challenges, particularly in areas like change detection. In this blog post, we will explore how Angular's change detection mechanism works, its implications in terms of performance, and how Java can interface with Angular to optimize these processes.
Understanding Angular Change Detection
What is Change Detection?
Change detection is a process that Angular employs to monitor changes in data-bound properties and then modify the view accordingly. This allows Angular applications to present dynamic content as they respond to user interactions, HTTP requests, and other events.
Angular relies on a digest cycle where it checks the model (data) against the view. If there is a discrepancy, it triggers a view update. This can be an efficient process; however, for complex applications, it can lead to performance bottlenecks. For a deep dive into this topic, refer to the article "Demystifying Angular: Solving Change Detection Mysteries" which thoroughly unpacks how change detection works in Angular (URL: infinitejs.com/posts/demystifying-angular-change-detection-mysteries).
Issues with Change Detection
-
Performance Overhead: In large applications, Angular may trigger excessive change detection cycles, especially when there are many bindings that need monitoring.
-
Zone.js: Angular uses Zone.js—an implementation of the Observer pattern. While it simplifies asynchronous operations, it may not always be the most efficient path, especially in CPU-intensive operations.
-
Scalability Concerns: As the application grows in complexity, the default change detection strategy (which is quite aggressive) might become a hindrance.
Bridging Angular and Java for Optimal Performance
To bridge the gap between Angular and Java properly, one must consider using Java as a backend service that effectively communicates changes to the frontend. This means the backend should only send relevant updates to reduce unnecessary change detection in the Angular application. Below, we will illustrate how you can produce such a connection.
Setting Up a Java Rest API
Java's Spring Boot framework provides a seamless way to create REST APIs that Angular can interact with.
Example: Creating a Simple REST API
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ItemController {
@GetMapping("/api/items")
public List<Item> getItems() {
// Simulate fetching items from a data source
return fetchItemsFromDatabase();
}
private List<Item> fetchItemsFromDatabase() {
// Imagine this method fetching items from a database
return Arrays.asList(new Item("Item 1"), new Item("Item 2"));
}
}
Why Spring Boot? Spring Boot simplifies the Java backend development, allowing developers to focus more on core application logic rather than boilerplate.
Making API Calls in Angular
Once you have your Java REST API set up, the next step is to make API calls using Angular’s HttpClientModule.
Example: Fetching Data in Angular
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-item-list',
template: `
<ul>
<li *ngFor="let item of items">{{ item.name }}</li>
</ul>
`
})
export class ItemListComponent implements OnInit {
items: Array<{ name: string }> = [];
constructor(private http: HttpClient) { }
ngOnInit() {
this.http.get<{ name: string }[]>('/api/items')
.subscribe(data => this.items = data);
}
}
Why Use HttpClient? The HttpClient module simplifies HTTP requests and returns typed observables, providing a cleaner, more robust approach to working with asynchronous data in Angular.
Optimizing Change Detection
OnPush Strategy
By default, Angular uses the Default Change Detection Strategy. However, for performance reasons in complex applications, consider switching to the OnPush Change Detection Strategy.
Example: Implementing OnPush Strategy
import { Component, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-item-list',
changeDetection: ChangeDetectionStrategy.OnPush,
template: `
<ul>
<li *ngFor="let item of items">{{ item.name }}</li>
</ul>
`
})
export class ItemListComponent {
items = [];
}
Why OnPush? This strategy reduces the number of checks Angular makes during change detection. With OnPush, Angular will only check the component if its inputs change or if an event is emitted from the component.
Leveraging Observables
Incorporating observables in your Angular app ensures that you are responding to data streams effectively. Observables provide significant benefits for change detection because they are inherently asynchronous and emit values over time.
Example: Using Observables with Angular
import { Component, OnInit } from '@angular/core';
import { ItemService } from './item.service';
@Component({
selector: 'app-item-list',
template: `
<ul>
<li *ngFor="let item of items | async">{{ item.name }}</li>
</ul>
`
})
export class ItemListComponent implements OnInit {
items$ = this.itemService.getItems();
constructor(private itemService: ItemService) { }
}
@Injectable({ providedIn: 'root' })
export class ItemService {
constructor(private http: HttpClient) { }
getItems(): Observable<Item[]> {
return this.http.get<Item[]>('/api/items');
}
}
Why Use Observables? Using an observable in the template with the async pipe automatically subscribes and unsubscribes, preventing potential memory leaks and ensuring a clean, concise syntax.
My Closing Thoughts on the Matter
The integration of Angular with Java can lead to powerful, maintainable applications, but understanding the intricacies of Angular's change detection is crucial for performance optimization. By implementing strategies such as switching to the OnPush strategy and leveraging observables, developers can significantly enhance efficiency.
As the web continues to evolve, so too will the relationships between frameworks and languages. Ensuring seamless communication between Angular and Java not only improves the performance of applications but also enhances the developer experience.
For further understanding of Angular’s change detection and how to maneuver through its challenges, check out "Demystifying Angular: Solving Change Detection Mysteries" at infinitejs.com/posts/demystifying-angular-change-detection-mysteries.
Happy coding!
This blog post is designed to be both educational and engaging, providing clear examples and an in-depth look at Angular and Java's interplay while adhering to SEO best practices. Feel free to dive deeper into each topic to craft your application seamlessly!
Checkout our other articles