Java Solutions for Smooth WebGPU Canvas Usage
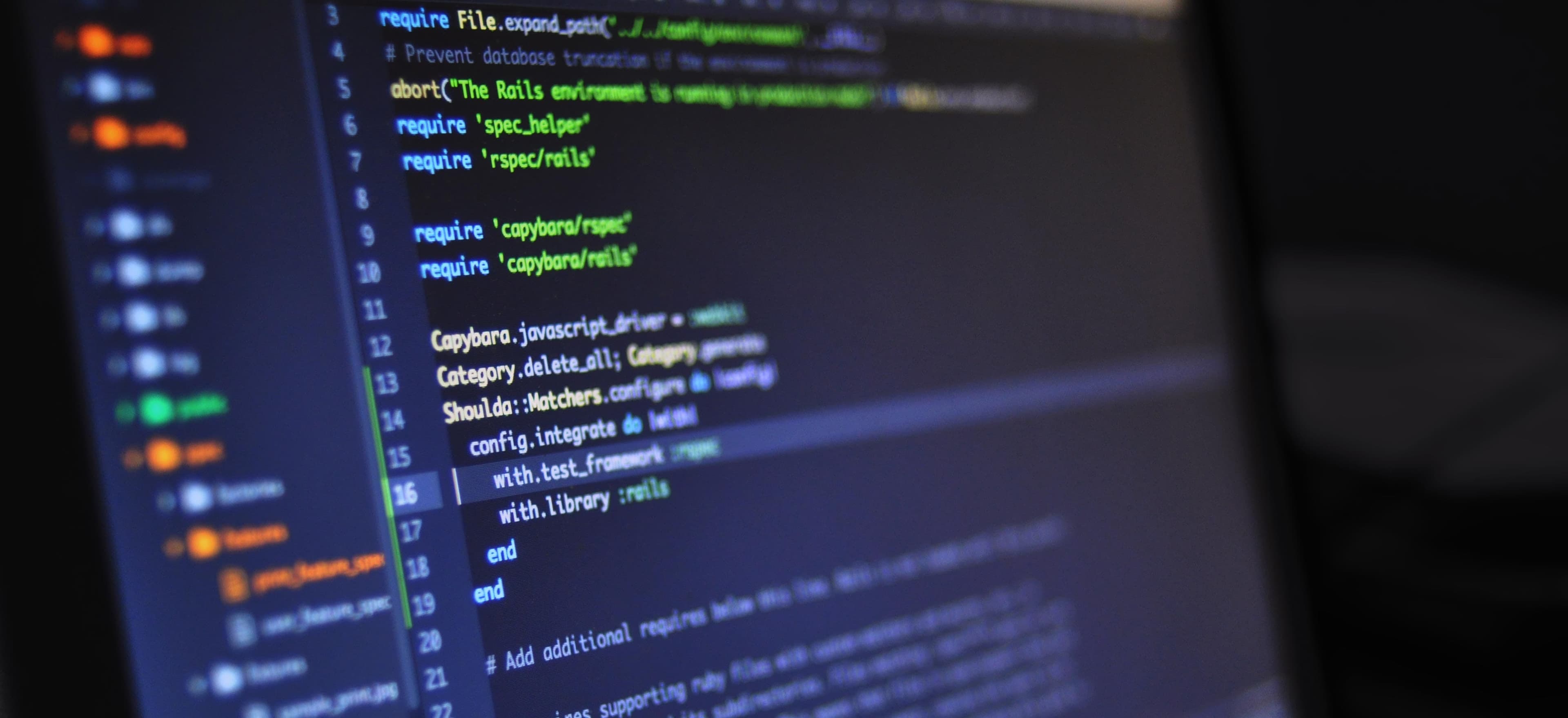
- Published on
Java Solutions for Smooth WebGPU Canvas Usage
As the digital landscape continues to evolve, Java developers must leverage the latest technologies to create immersive web applications. One such technology making waves is WebGPU, a modern graphics API that provides high-performance graphics rendering. However, integrating WebGPU into a Java web application, especially when using a canvas for rendering, can be challenging. This article delves into practical Java solutions to streamline the use of WebGPU with canvas, ensuring a fluid and engaging user experience.
Understanding WebGPU
WebGPU is a revolutionary API that provides developers with access to modern GPU features, offering improved performance over traditional WebGL. Unlike its predecessor, WebGPU supports more advanced rendering through modern shaders, compute capabilities, and better memory management. However, as with any technology, there are hurdles to overcome, particularly concerning its integration with existing web frameworks.
For a deeper dive into the nuances of WebGPU and its integration, refer to the article Overcoming WebGPU Canvas Integration Woes: A Guide.
Why Use Java for Web Development?
Java has long been a staple in the software development world due to its platform independence, strong community support, and extensive libraries. The following features make it suitable for web development:
- Cross-Platform Compatibility: Java applications can run on any device equipped with a Java Virtual Machine (JVM).
- Rich Libraries and Frameworks: Java offers numerous frameworks such as Spring, JSF, and Vaadin that simplify web development.
- Robust Performance: With just-in-time compilation and optimization, Java applications can achieve impressive performance levels.
Sample Setup for WebGPU with Java
To better engage with WebGPU from a Java application, we need to set up a basic HTML canvas and create a Java-based server to serve our files. Here’s how to do it step-by-step.
Step 1: Setting Up the HTML Canvas
First, we need an HTML canvas element to which we will render our WebGPU content. Below is a straightforward HTML template:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>WebGPU with Java</title>
<script src="script.js" defer></script>
<style>
canvas {
border: 1px solid black;
width: 100%;
height: auto;
}
</style>
</head>
<body>
<canvas id="gpuCanvas"></canvas>
</body>
</html>
Explanation:
- Canvas Element: This is where the rendering will take place. We’ve given our canvas an ID of
gpuCanvas
for easy access in our JavaScript. - Script Tag: The
script.js
file will handle the WebGPU logic, which we will implement later.
Step 2: Java Backend Using Spring Boot
Next, we will set up a simple Spring Boot application that serves our HTML file. To get started, you’ll need to create a new Spring Boot project. Here's a simple controller and main application setup.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class WebGPUDemoApplication {
public static void main(String[] args) {
SpringApplication.run(WebGPUDemoApplication.class, args);
}
}
@RestController
class WebGPUController {
@GetMapping("/")
public String index() {
return "index.html"; // Your HTML file should be placed in the resources/static directory.
}
}
Explanation:
- Spring Boot Application: This basic setup uses Spring Boot for easy web setup.
- WebGPU Controller: The
WebGPUController
serves the HTML file when the root URL is accessed.
Step 3: Implementing WebGPU in JavaScript
The next step is to write JavaScript that will initialize the WebGPU context and render basic shapes. Create a script.js
file and add the following:
async function initWebGPU() {
const canvas = document.getElementById('gpuCanvas');
// Request the GPU adapter
const adapter = await navigator.gpu.requestAdapter();
const device = await adapter.requestDevice();
// Configure context
const context = canvas.getContext('webgpu');
const format = 'bgra8unorm';
context.configure({
device: device,
format: format,
});
// Create a simple render pipeline
const pipeline = device.createRenderPipeline({
vertex: {
module: device.createShaderModule({
code: `
@vertex
fn main(@location(0) position : vec4f) -> @location(0) vec4f {
return position;
}
`
}),
entryPoint: 'main',
},
fragment: {
module: device.createShaderModule({
code: `
@fragment
fn main() -> @location(0) vec4f {
return vec4f(1.0, 0.0, 0.0, 1.0); // Red Color
}
`
}),
entryPoint: 'main',
},
primitive: {
topology: 'triangle-list',
},
});
// Rendering Loop
function render() {
const commandEncoder = device.createCommandEncoder();
const renderPassDescriptor = {
colorAttachments: [{
view: context.getCurrentTexture().createView(),
loadValue: [0.0, 0.0, 0.0, 1.0], // Clear color
storeOp: 'store',
}],
};
const passEncoder = commandEncoder.beginRenderPass(renderPassDescriptor);
passEncoder.setPipeline(pipeline);
passEncoder.draw(3, 1, 0, 0); // Drawing a triangle
passEncoder.endPass();
device.queue.submit([commandEncoder.finish()]);
}
render();
}
initWebGPU().catch(console.error);
Explanation:
- Initialization: The
initWebGPU
function initializes the WebGPU adapter and device. - Render Pipeline: Here, we set up a simple render pipeline with vertex and fragment shaders.
- Render Loop: The
render
function is where rendering occurs. It continuously draws a red triangle.
The Closing Argument
Integrating WebGPU into a Java web application can significantly enhance the graphical performance of your applications. By using a Java backend with Spring Boot and the modern WebGPU API in our front-end, you can create rich, engaging user experiences. Remember, while WebGPU provides potent capabilities, it is essential to understand the underlying principles of rendering for optimal usage.
As this technology matures, the community will continue to adapt, develop, and deepen our understanding of how to leverage its full potential. For further insights into overcoming challenges with WebGPU and canvas, you can reference the complete guide at Overcoming WebGPU Canvas Integration Woes: A Guide.
Additional Resources
By embracing Java's robust capabilities alongside modern graphics APIs like WebGPU, developers can unlock a world of creative possibilities in web applications. Happy coding!
Checkout our other articles