Integrating WebGPU with Java: Tackling Common Challenges
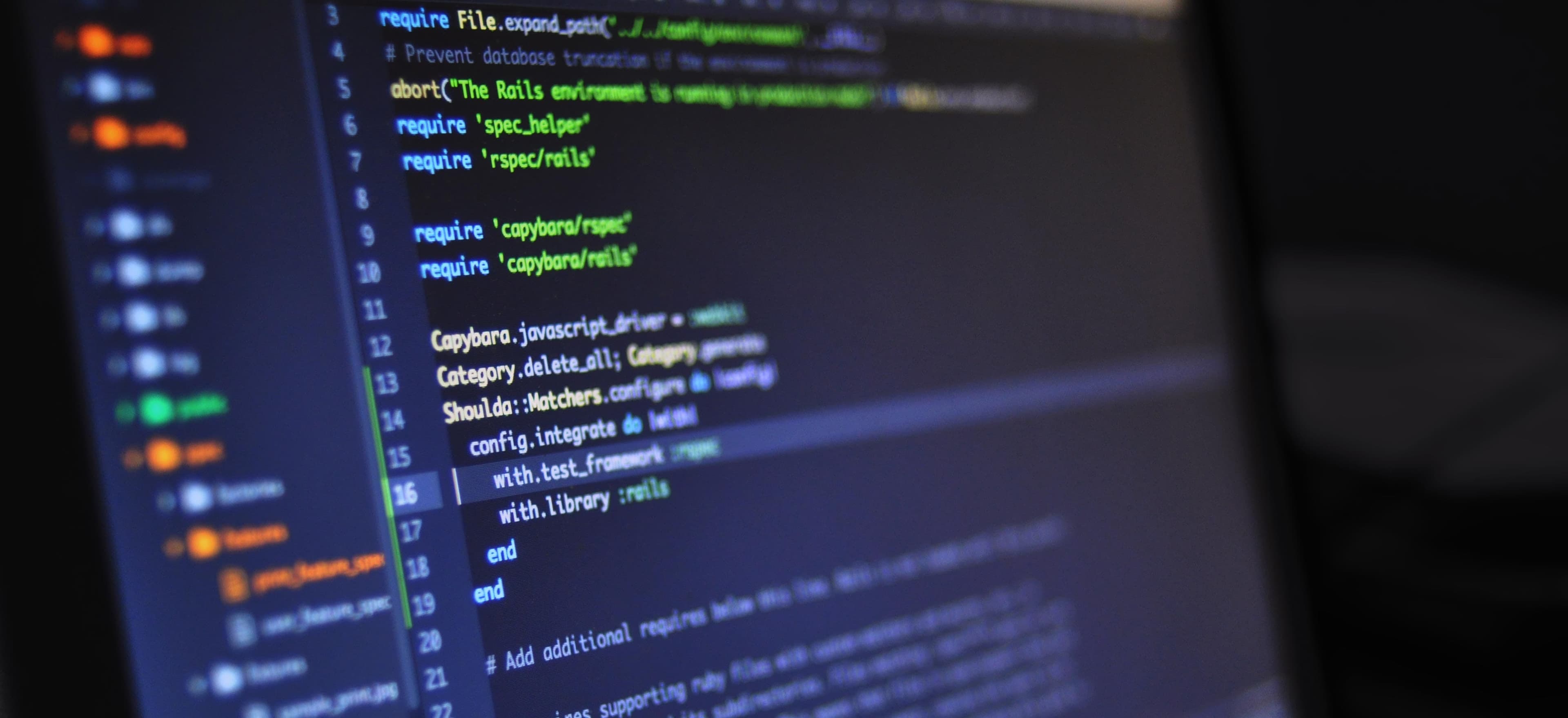
- Published on
Integrating WebGPU with Java: Tackling Common Challenges
WebGPU is a cutting-edge API designed to enable high-performance graphics and computation on the web. As Java developers venture into this revolutionary realm, they may face unique challenges. This blog post will guide you through integrating WebGPU with Java while addressing common hurdles. Let’s delve into the nuances of this integration without losing sight of the bigger picture.
Understanding WebGPU
WebGPU serves as a modern alternative to WebGL, offering a much richer set of features and capabilities. It leverages the power of low-level graphics APIs and allows developers to harness the full potential of the hardware. Sounds compelling, right?
Key Features of WebGPU
- Low-Level Access: Provides direct access to the GPU, allowing for more control and efficiency.
- Async Operations: Improves performance by allowing non-blocking GPU operations.
- Cross-Platform Support: Works on various platforms, making it versatile for web development.
Before diving into coding, it's essential to familiarize yourself with the abstractions and functions offered by WebGPU. For a more comprehensive understanding of the topic, the article titled "Overcoming WebGPU Canvas Integration Woes: A Guide" serves as an excellent resource.
Prerequisites
To embark on this journey, you'll need:
- A basic understanding of Java and web technologies.
- Familiarity with WebGPU principles.
- JavaFX installed for creating graphical user interfaces (GUIs).
Setting Up Your Environment
To make the best use of WebGPU, ensure your browser supports it. Browsers like Chrome and Firefox are leading the charge with experimental support. Here’s how to set up your JavaFX application:
- Download and Install JavaFX: JavaFX is crucial for rendering graphics in Java.
- Configure your IDE: Ensure your IDE recognizes JavaFX.
Here's how you might set up a simple JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class WebGPUExample extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 800, 600);
primaryStage.setTitle("WebGPU with Java");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary on the Code
- Application Class: Inherit from
Application
to create your JavaFX app. - Stage & Scene: These objects represent the window and the contents of your app.
- Launch Method: The
main
method launches the application.
This setup lays the groundwork for further integration with WebGPU.
Connecting Java with WebGPU
In essence, bridging Java and WebGPU requires interaction between Java's backend logic and WebGPU’s graphics capabilities. One approach to achieve this is through a JavaScript bridge, enabling communication with WebGPU APIs.
Creating a JavaScript Bridge
You can utilize the JavaFX WebView component to load JavaScript code that interacts with WebGPU. Here’s how to set up the WebView:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.web.WebEngine;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
public class WebGPUExample extends Application {
@Override
public void start(Stage primaryStage) {
WebView webView = new WebView();
WebEngine webEngine = webView.getEngine();
// Load an HTML file that interacts with WebGPU
webEngine.loadContent(getHtmlContent());
Scene scene = new Scene(webView, 800, 600);
primaryStage.setTitle("WebGPU with Java");
primaryStage.setScene(scene);
primaryStage.show();
}
private String getHtmlContent() {
return "<html><body>" +
"<script>" +
"async function initWebGPU() { /* WebGPU initialization code */ }" +
"initWebGPU();" +
"</script>" +
"</body></html>";
}
public static void main(String[] args) {
launch(args);
}
}
Commentary on the Code
- WebView Component: The
WebView
allows rendering of web content in JavaFX applications. - WebEngine: This engine executes JavaScript inside the WebView.
- HTML Content: The string returned from
getHtmlContent
contains the script for initializing WebGPU.
With the above setup, you can embed JavaScript code directly into your Java application, allowing you to use WebGPU features.
Handling Common Challenges
As you integrate WebGPU with Java, several hurdles may arise. Let’s address a few of the most common challenges developers face.
1. Asynchronous Initialization
WebGPU APIs are primarily asynchronous. Java developers often expect synchronous operations, leading to anticipation errors.
How to Overcome: Use Promises in JavaScript and callbacks to inform your Java application when the GPU is ready.
async function initWebGPU() {
const adapter = await navigator.gpu.requestAdapter();
if (!adapter) {
console.error("Failed to get GPU adapter.");
return;
}
const device = await adapter.requestDevice();
console.log("WebGPU Device initialized.");
}
2. Communication Latency
Java and JavaScript run in different environments, which can lead to communication latency.
Solution: Optimize your function calls to minimize unnecessary data transfers between the contexts. Batch your operations.
3. Debugging Issues
Debugging WebGPU code can be tricky, especially if you encounter errors during rendering.
Tip: Utilize browser developer tools for JavaScript debugging and ensure you log error messages to pinpoint issues.
device.createShaderModule({
code: '...',
}).catch(err => console.error("Shader Module Error: ", err));
Best Practices for a Smooth Integration
- Always Check Compatibility: Before deploying your application, ensure that your users' environments support WebGPU.
- Optimize Performance: Leverage GPU capabilities responsibly, avoiding unnecessary computations on the CPU.
- Regular Updates: WebGPU is a rapidly evolving API. Stay updated with changes and improvements.
Closing the Chapter
Integrating WebGPU with Java presents both exciting opportunities and unique challenges. By understanding WebGPU's capabilities and using JavaFX effectively, you can create engaging, high-performance applications.
Moreover, the reference to resources like "Overcoming WebGPU Canvas Integration Woes: A Guide" can deepen your understanding and provide further knowledge.
As you embrace this integration, remember that challenges are opportunities to learn and improve. Happy coding!
Checkout our other articles