Fixing UI Inconsistencies in Java Web Applications
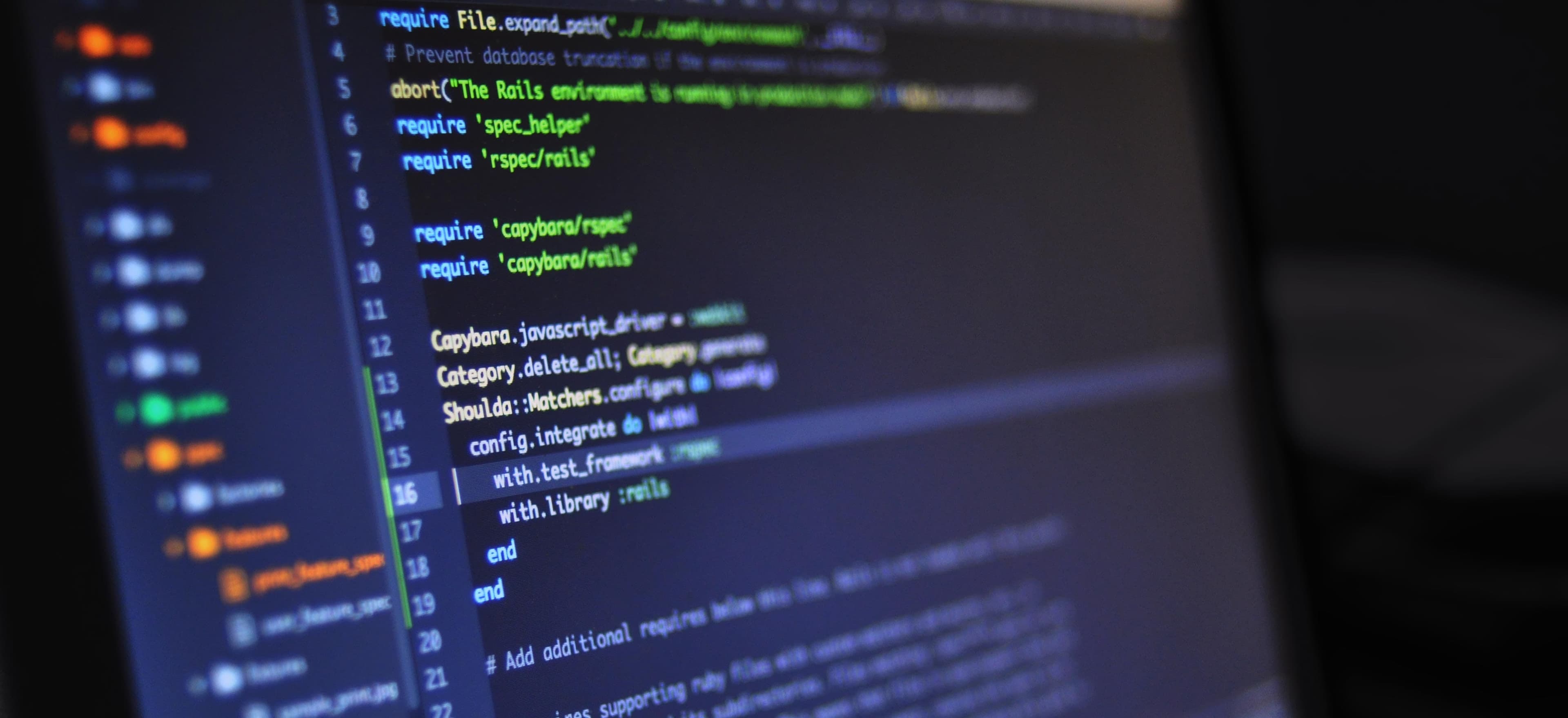
- Published on
Fixing UI Inconsistencies in Java Web Applications
In today's digital world, user interface (UI) design plays an essential role in the success of web applications. For developers, ensuring a consistent look and feel across different browsers and devices can be challenging. This post will explore common UI inconsistencies in Java web applications and provide actionable solutions to help you create a more seamless user experience.
Understanding UI Inconsistencies
UI inconsistencies manifest in various ways, including differences in spacing, font sizes, colors, and button sizes. Such discrepancies can stem from differences in browser rendering engines and CSS interpretations. A notable example includes the Inconsistent Search Input and Button Sizes in IE7 and Firefox, which illustrates how browsers can render the same code differently. You can read about this issue here.
Why UI Consistency Matters
Maintaining a consistent UI is crucial for several reasons:
- User Experience: A consistent interface fosters familiarity, allowing users to navigate with confidence.
- Credibility: Consistency enhances perceived reliability and trustworthiness in your brand.
- Reducing Errors: An inconsistent UI can confuse users, leading to mistakes in navigation and interaction.
Now, let’s dive into the strategies and tips to fix these inconsistencies.
CSS Fundamentals for Consistency
Resetting CSS Styles
One of the first steps in achieving UI consistency is to reset CSS styles to ensure a neutral baseline across all browsers. Many CSS frameworks come with built-in styles that reduce inconsistencies. A simple CSS reset can look like this:
/* CSS Reset */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
line-height: 1.6;
}
This code snippet eliminates default browser styling, making it easier to implement your desired design. The box-sizing: border-box;
property simplifies width calculations, making layout design straightforward.
Using Global CSS Variables
CSS variables help enforce consistent spacing, colors, and font sizes across your application. Below is an example of how to declare and utilize CSS variables:
:root {
--primary-color: #4CAF50;
--secondary-color: #ffffff;
--font-size: 16px;
--spacing-unit: 8px;
}
body {
background-color: var(--secondary-color);
color: var(--primary-color);
font-size: var(--font-size);
padding: var(--spacing-unit);
}
By defining these variables, you can easily change your design's core aspects without rewriting multiple lines of CSS code. This method greatly enhances maintainability.
Responsive Web Design
Media Queries for Flexibility
With users accessing web applications on a myriad of devices, applying responsive design techniques is vital. Media queries enable you to adapt your layout based on device characteristics:
@media (max-width: 600px) {
body {
padding: var(--spacing-unit);
font-size: calc(var(--font-size) * 0.9);
}
}
In this example, the font size decreases on smaller screens, ensuring that text remains legible and well-proportioned.
Using Flexbox and Grid
Leveraging CSS Flexbox or CSS Grid can help neatly handle layout issues. Consider a basic Flexbox setup for aligning buttons and input fields uniformly:
.container {
display: flex;
flex-wrap: wrap;
gap: var(--spacing-unit);
}
.button, .input-field {
flex: 1 1 calc(50% - var(--spacing-unit));
}
This ensures that buttons and inputs are consistently sized relative to one another in different viewport sizes, thus promoting visual harmony.
Java and JSP: Best Practices
When working with Java web applications and JSP (JavaServer Pages), following certain best practices will aid in maintaining UI consistency.
Use Tag Libraries
JSP tag libraries can help standardize UI components. Using custom tags reduces clutter and improves readability. Here's an example of a custom button tag:
<%@ tag language="java" pageEncoding="UTF-8"%>
<%@ attribute name="label" required="true" %>
<button class="custom-button"><%= label %></button>
This single tag can be called multiple times with different labels throughout your application, creating consistency without repetitively writing button code.
Integrating Frameworks
Incorporate a front-end framework like Bootstrap or Materialize CSS to leverage their built-in styling. For instance, integrating Bootstrap in a Java application involves including their CDN link in your JSP file:
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
Using these frameworks provides a consistent design and significantly reduces the time spent on CSS styling.
Testing for Cross-Browser Compatibility
Automated Testing Tools
To ensure your web application behaves consistently across browsers, it is essential to implement automated testing. Tools like Selenium WebDriver can help you create test scripts that mimic user interactions, allowing you to identify UI inconsistencies proactively.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class UITest {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("http://yourwebapp.com");
// Check for button size consistency
String buttonSize = driver.findElement(By.id("submitButton")).getCssValue("width");
System.out.println("Button width: " + buttonSize);
driver.quit();
}
}
In this snippet, we launch a web app and check the button size. This practice ensures you catch UI discrepancies early during the testing phase.
User Testing Feedback
Gathering feedback from users can provide insight into how the UI is perceived in real-world situations. Consider conducting usability tests or surveys to identify specific areas that may need attention.
The Closing Argument
UI consistency is a crucial aspect of developing Java web applications. By implementing best practices for CSS styling, leveraging frameworks, and utilizing testing tools, you can create a more coherent user experience. Be sure to read the article on Inconsistent Search Input and Button Sizes in IE7 and Firefox here for additional insights on specific UI issues.
Building a beautiful and responsive UI is a continuous process, but with these strategies and techniques, you will be well on your way to ensuring a smooth and consistent user experience across various browsers and devices.
Embrace the challenge, stay updated with best practices, and let your Java web applications shine!