Tackling Inactive Sessions in Java Web Applications
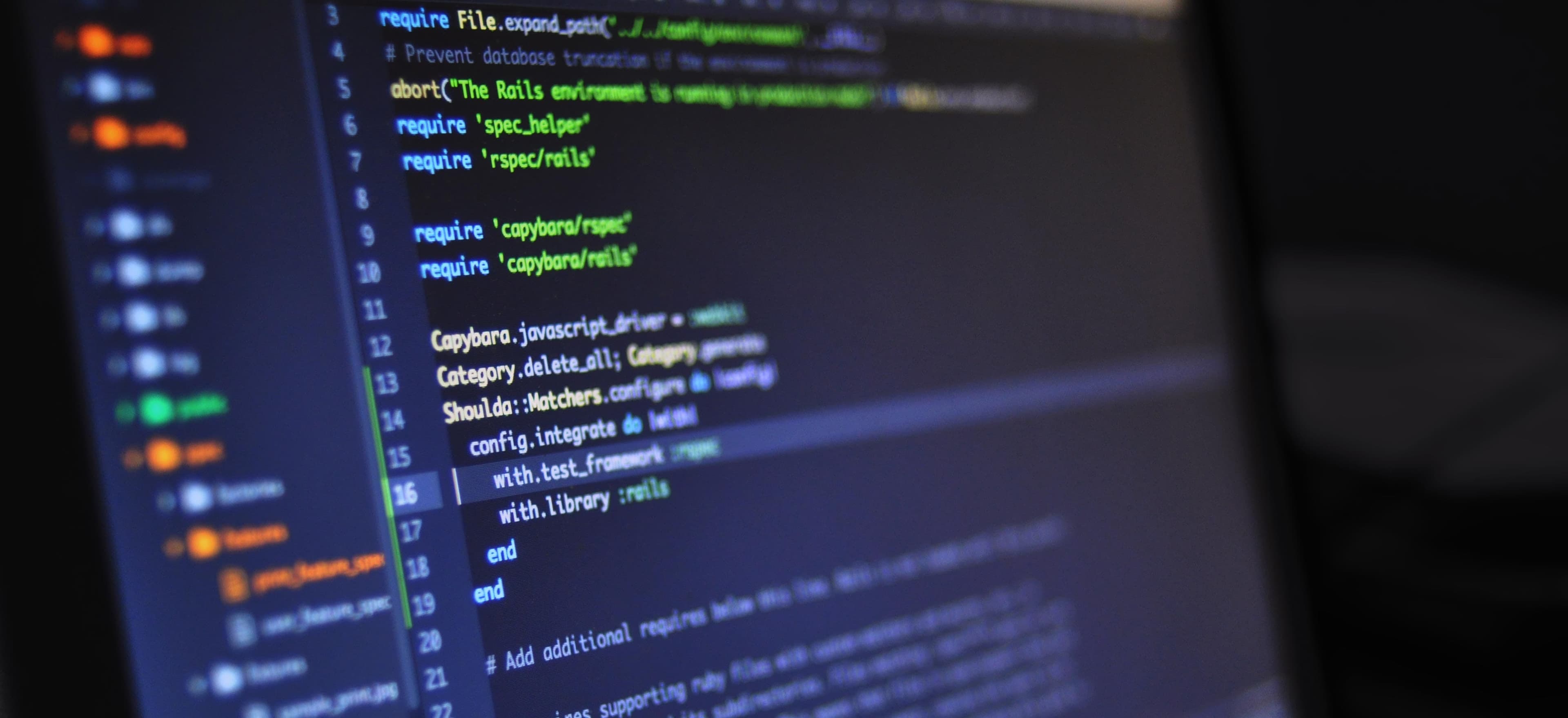
- Published on
Tackling Inactive Sessions in Java Web Applications
Managing session states is critical in any web application, especially for Java developers. Sessions help in maintaining the state across multiple requests from the same client. However, inactive sessions can lead to disruptions, affecting user experience, performance, and resource management. In this blog post, we will dive deep into the implications of inactive sessions and provide practical solutions to handle this issue effectively.
What are Inactive Sessions?
Before we delve into solutions, it's essential to understand what inactive sessions are. Inactive sessions occur when a user is logged into a web application but does not engage with it for a specified period. These sessions can cause memory leaks, resource exhaustion, and, in some cases, security vulnerabilities.
The importance of managing inactive sessions cannot be overstated. As discussed in the article Troubleshooting: Inactive Sessions Causing Disruptions, appropriately configuring session timeouts can lead to significant improvements in both performance and user experience.
Why Should We Care?
- Performance: Unmanaged inactive sessions consume server resources.
- User Experience: Continuously checking active sessions ensures that users have a speedy, responsive interface.
- Security: Active sessions can be hijacked if properly managed and cleared.
Steps to Handle Inactive Sessions
Step 1: Configure Session Timeout
Configuring session timeout is the first defense line against idle sessions. You can configure the session timeout in your web.xml file for your Java web application:
<session-config>
<session-timeout>15</session-timeout>
</session-config>
This sets the session timeout to 15 minutes. Properly setting the timeout balances between user engagement and resource management.
Why Configure Timeout?
Configuring a reasonable timeout directly impacts system performance and responsiveness. It also plays a vital role in freeing up server resources, allowing for better scalability.
Step 2: Monitor Session Activity
To actively manage inactive sessions, monitoring their activity can be invaluable. You can implement a filter to log session activity:
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
public class SessionActivityFilter implements Filter {
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletRequest req = (HttpServletRequest) request;
HttpSession session = req.getSession(false);
if (session != null) {
// Log the session activity
session.setAttribute("lastActivityTime", System.currentTimeMillis());
}
chain.doFilter(request, response);
}
}
This filter sets the last activity time for each session. You can further enhance this by adding tasks that periodically check if sessions have surpassed their timeout thresholds.
Why Monitor Session Activity?
Logging session activity allows you to handle exceptions more gracefully. If users know their sessions are about to timeout, for instance, they can be prompted to continue browsing.
Step 3: Cleanup Inactive Sessions
For active cleanup of inactive sessions, you might want to schedule a regular task. This task can check all sessions and invalidate the ones that have exceeded their timeout.
Here’s an example using a ScheduledExecutorService:
import javax.servlet.http.HttpSession;
import java.util.Iterator;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class SessionCleanupService {
private final ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
public void startCleanupTask() {
scheduler.scheduleAtFixedRate(() -> {
// This can be an actual session store to be checked
Iterator<HttpSession> sessions = getSessionIterator(); // Assume this method fetches all active sessions
while (sessions.hasNext()) {
HttpSession session = sessions.next();
Long lastActivityTime = (Long) session.getAttribute("lastActivityTime");
if (lastActivityTime != null && isSessionStale(lastActivityTime)) {
session.invalidate();
}
}
}, 0, 10, TimeUnit.MINUTES);
}
private boolean isSessionStale(Long lastActivityTime) {
return (System.currentTimeMillis() - lastActivityTime) > TimeUnit.MINUTES.toMillis(15);
}
}
In this setup, every 10 minutes, inactive sessions exceeding the defined timeout will be invalidated to free up resources.
Why Cleanup Inactive Sessions?
Regular cleanup of sessions ensures your application maintains optimal performance. It minimizes memory leaks and potential security vulnerabilities from idle sessions.
Step 4: User Notifications
Notify users before session expiration. Doing so enhances user experience by giving users the opportunity to remain active in their session.
Here’s a simple JavaScript implementation to juxtapose your Java application's session management:
<script>
let timeoutWarning;
const sessionTimeoutDuration = 15 * 60 * 1000; // 15 minutes
const warningDuration = 2 * 60 * 1000; // 2 minutes
document.onload = resetTimer();
function resetTimer() {
clearTimeout(timeoutWarning);
timeoutWarning = setTimeout(() => {
alert("Your session will expire soon. Please interact to stay logged in.");
// Optionally, here you can call a function to keep the session alive
}, sessionTimeoutDuration - warningDuration);
}
document.addEventListener("mousemove", resetTimer);
document.addEventListener("keypress", resetTimer);
</script>
Why Use User Notifications?
User notifications act as a buffer, granting users a chance to remain active instead of being abruptly logged out. This approach fosters a sense of control and improves the overall user experience.
Bringing It All Together
Inactive sessions can disrupt the functionality and performance of any Java web application. By configuring session timeouts, monitoring session activity, regularly cleaning up inactive sessions, and providing user notifications, developers can maintain a seamless experience for their users.
For further insights into managing inactive sessions, refer to the Troubleshooting: Inactive Sessions Causing Disruptions.
Managing sessions is more than just a necessity—it's a commitment to user experience and application performance. Leveraging the above techniques will lead to more responsive applications and happier users.