Optimizing Java Applications for Large SQL Dataset Access
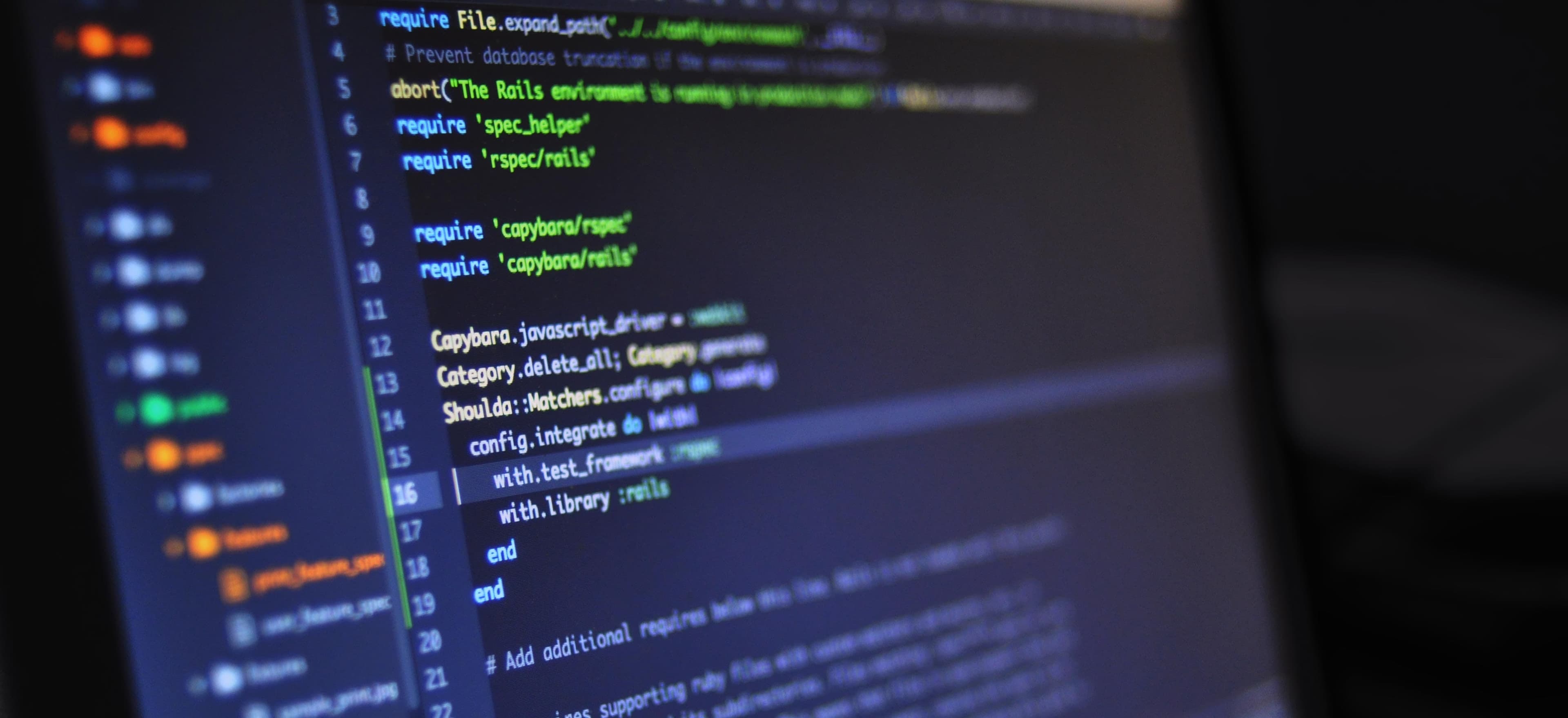
- Published on
Optimizing Java Applications for Large SQL Dataset Access
In the era of big data, applications often require efficient ways to access and manage large datasets. For Java developers, this challenge is even more pronounced given Java's interoperability with various databases. In this blog post, we will explore effective techniques to optimize Java applications for handling large SQL datasets. We will delve into connection management, lazy loading, effective query practices, and other indispensable strategies. If you find yourself working with large datasets, this guide will help you refine your implementation and improve performance.
1. Connection Management
The foundation of any database operation is establishing a reliable connection. Poor connection management can lead to issues, such as connection timeouts or excessive resource usage. Here are the key aspects to consider:
Connection Pooling
Utilizing a connection pool can significantly enhance performance by reusing existing database connections. This reduces the overhead of constantly opening and closing connections.
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("user");
config.setPassword("password");
config.setMaximumPoolSize(10); // Set maximum connections
HikariDataSource dataSource = new HikariDataSource(config);
Why this works: Connection pooling minimizes the time spent dealing with connection setups. This leads to quicker request handling, especially in high-traffic applications. For more information about connection pooling, check out the HikariCP documentation.
Closing Connections
Always ensure that connections are closed after use to avoid resource leaks.
try (Connection conn = dataSource.getConnection()) {
// Your SQL operations here
} catch (SQLException e) {
e.printStackTrace(); // Handle exceptions
}
Why this works: Using try-with-resources ensures that resources are automatically closed, making code cleaner and preventing memory leaks.
2. Efficient Query Practices
The efficiency of your SQL queries directly influences application performance. Consider these strategies:
Use SELECT
Wisely
Avoid using SELECT *
. Instead, specify only the required columns in your queries:
SELECT id, name FROM users WHERE status = 'active';
Why this works: Reducing the amount of data retrieved speeds up the query execution and processing time. Additionally, it lowers network bandwidth usage.
Pagination
When dealing with large datasets, it is crucial to implement pagination:
String sql = "SELECT id, name FROM users LIMIT ?, ?";
try (PreparedStatement ps = conn.prepareStatement(sql)) {
ps.setInt(1, offset); // offset for the current page
ps.setInt(2, limit); // number of records per page
ResultSet rs = ps.executeQuery();
while (rs.next()) {
// Process the results
}
}
Why this works: Pagination prevents loading an entire dataset into memory, which can lead to performance bottlenecks and memory overflow.
Indexing
Proper indexing in the database can drastically enhance query performance. Always create indexes on columns frequently used in WHERE clauses.
CREATE INDEX idx_status ON users(status);
Why this works: Indexing allows the database to quickly find rows that match the query conditions, resulting in faster query execution.
3. Lazy Loading
Lazy loading is a design pattern that postpones the loading of data until it is actually needed. This can significantly reduce memory consumption.
public class UserService {
public User getUserById(int id) {
// Only loads user lazily when needed
return new UserLazyLoader(id);
}
}
Why this works: By delaying data loading, applications can reduce unnecessary database calls and enhance performance, especially in scenarios where not all data is used immediately.
4. Batch Processing
In scenarios where multiple inserts or updates are required, batch processing can drastically cut down the time spent interacting with the database.
String sql = "INSERT INTO users(name) VALUES(?)";
try (PreparedStatement ps = conn.prepareStatement(sql)) {
for (String name : names) {
ps.setString(1, name);
ps.addBatch();
}
ps.executeBatch(); // Execute all at once
}
Why this works: Instead of executing each statement one by one, batched execution reduces the number of round trips to the database, leading to increased efficiency.
5. Caching
Caching can be a game changer in reducing database load and improving response times.
In-memory Caching
Using libraries like Ehcache for in-memory caching can optimize frequently accessed data retrieval.
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder().build(true);
Cache<Integer, User> cache = cacheManager.createCache("userCache", CacheConfigurationBuilder.newCacheConfiguration(Integer.class, User.class, ResourcePoolsBuilder.heap(100)));
Why this works: Cache data that is accessed frequently, which means less need for database queries—this can lead to faster response times and reduced load on your SQL server.
The Last Word
Optimizing Java applications for large SQL dataset access requires a multi-faceted approach, from effective connection management to careful query design and the implementation of caching strategies. By applying these techniques, you can significantly improve the performance of your Java applications, ensuring they are capable of handling large data efficiently.
If your project involves managing large datasets using SQL, you may find additional insights in the article Managing Large Datasets: Efficient SQL Techniques. Implementing the discussed techniques will not only enhance performance but also provide a robust framework for future scalability.
By focusing on these strategies, you are investing in the long-term performance and reliability of your applications. Remember that continuous profiling and refining your approach based on real-world usage will further enhance the effectiveness of your optimizations. Happy coding!