Simplifying Java Applications with Dependency Injection
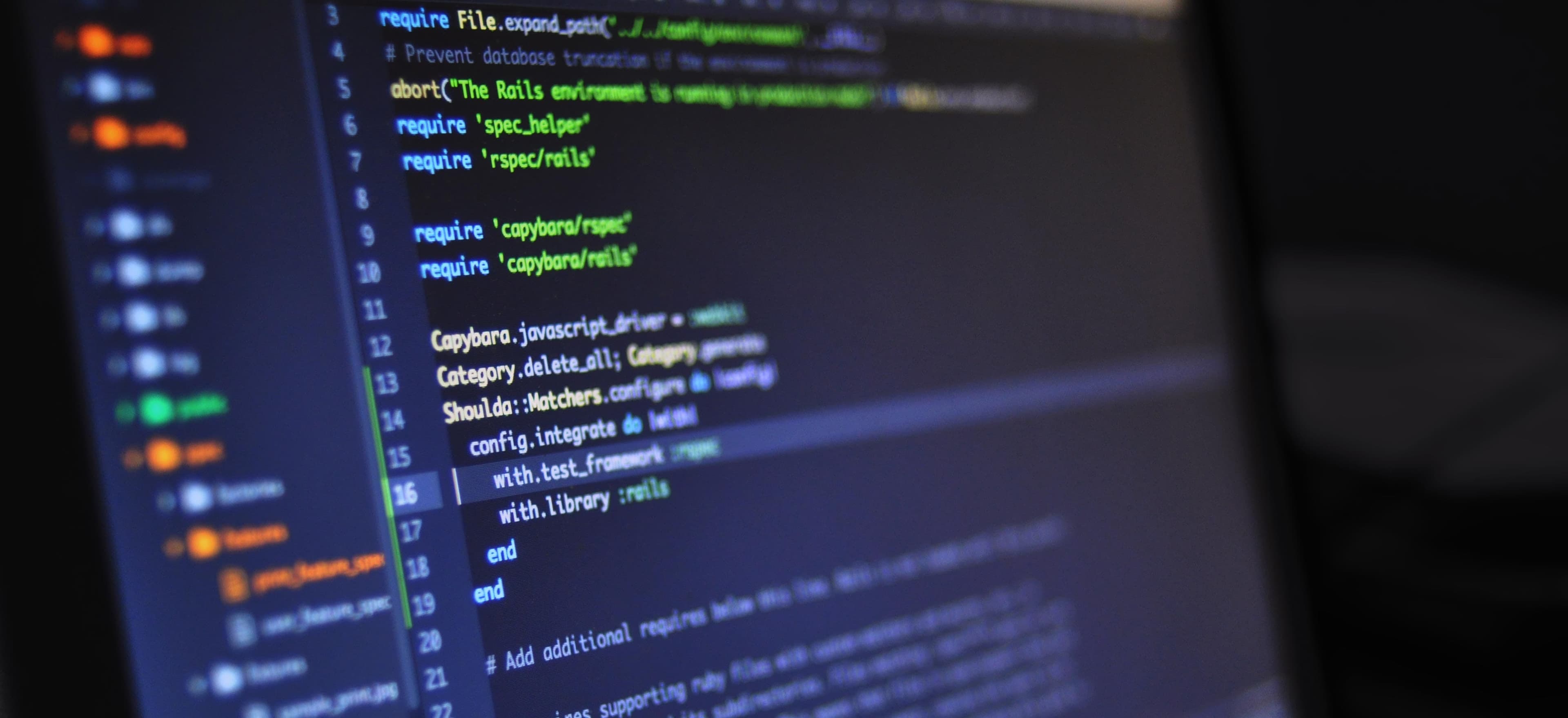
- Published on
Simplifying Java Applications with Dependency Injection
In today's rapidly evolving software development landscape, building scalable and maintainable applications is paramount. One architectural principle that has gained significant traction is Dependency Injection (DI). In this blog post, we'll explore how you can simplify your Java applications using DI, its benefits, and a practical approach to implementing it.
What is Dependency Injection?
At its core, Dependency Injection is a design pattern that allows for the decoupling of components within an application. Instead of a class creating its dependencies, they are passed to it. This promotes loose coupling and enhances testability. In a typical setup, a class relies on an interface or an abstract class, while the actual implementation is injected at runtime.
Why Use Dependency Injection?
-
Enhanced Testability: DI makes unit testing easier. By injecting mocks or stubs, you can isolate tests for specific components.
-
Loose Coupling: Components interact through interfaces, reducing the interdependencies among classes.
-
Code Reusability: When classes are independently constructed, they can be reused across different applications.
-
Improved Maintainability: Changes in one module won’t necessitate changes in another, as long as the interface remains consistent.
Common DI Frameworks in Java
While manual DI can be implemented, leveraging frameworks can simplify the process significantly. Some popular DI frameworks used in Java applications include:
- Spring Framework: Arguably the most popular DI framework used in enterprise applications.
- Guice: A lightweight DI framework developed by Google.
- Dagger: A compile-time DI framework that eliminates runtime performance overhead.
For the purpose of this blog post, we will focus on Spring and demonstrate its DI capabilities.
Getting Started with Spring
To introduce DI in your Java application using Spring, you first need to set up your development environment. Here’s a simple way to get started:
- Create a new Maven project.
- Add the necessary dependencies for Spring in your
pom.xml
:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.10</version>
</dependency>
Creating Your Components
Consider a simple application where you manage user notifications. We will create a NotificationService
that depends on a MessageService
.
Here are the steps to implement DI with explanations.
Step 1: Define the Interface
First, define a MessageService
interface:
public interface MessageService {
void sendMessage(String message);
}
Here, the MessageService
interface establishes a contract for message sending. The primary function, sendMessage
, will be implemented in concrete classes.
Step 2: Create Offspring of the Interface
Next, implement the MessageService
interface:
public class EmailService implements MessageService {
@Override
public void sendMessage(String message) {
System.out.println("Email message sent: " + message);
}
}
public class SMSService implements MessageService {
@Override
public void sendMessage(String message) {
System.out.println("SMS message sent: " + message);
}
}
Both EmailService
and SMSService
classes provide specific implementations for sending messages. This approach allows you to switch message types without changing the core business logic.
Step 3: Create the NotificationService
Now let’s create the NotificationService
, which depends on MessageService
.
public class NotificationService {
private MessageService messageService;
// Constructor Injection
public NotificationService(MessageService messageService) {
this.messageService = messageService;
}
public void notifyUser(String message) {
messageService.sendMessage(message);
}
}
In this example, notice how we use constructor injection to provide the MessageService
implementation. This emphasizes that NotificationService
does not own the MessageService
; it merely receives it, thus promoting loose coupling.
Step 4: Configure Spring
Next, let’s configure Spring to manage our dependencies. Create an XML configuration file named applicationContext.xml
:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="emailService" class="EmailService"/>
<bean id="notificationService" class="NotificationService">
<constructor-arg ref="emailService"/>
</bean>
</beans>
In this configuration, we define our beans. Spring will understand that NotificationService
requires an instance of EmailService
and will inject it automatically.
Step 5: Running the Application
Finally, let's run the application:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class App {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
NotificationService notificationService = context.getBean(NotificationService.class);
notificationService.notifyUser("Hello DI!");
}
}
Explanation
-
ApplicationContext: It is the center of the Spring framework. It reads the configuration from
applicationContext.xml
and creates the required beans. -
notifyUser: This method in
NotificationService
invokessendMessage
on the injectedMessageService
. -
Loose Coupling and Testability: If you wanted to switch to
SMSService
, you simply modify the XML configuration without alteringNotificationService
orMessageService
.
The Closing Argument
Dependency Injection is a powerful mechanism that can significantly improve the structure and maintainability of your Java applications. By decoupling your components, relying on interfaces, and using a DI framework like Spring, you can achieve a higher degree of flexibility and testability.
For further reading on how DI can transform your code, check out the existing article titled "Streamline Your Code: Mastering Dependency Injection with StructureMap." This resource delves deeper into the principles of DI and its implementing frameworks.
By adopting Dependency Injection principles in your development process, you can not only streamline your workflow but also enhance collaboration and code quality. Are you ready to implement DI in your Java application? Happy coding!