Mastering Feature Flags for Effective Java A/B Testing
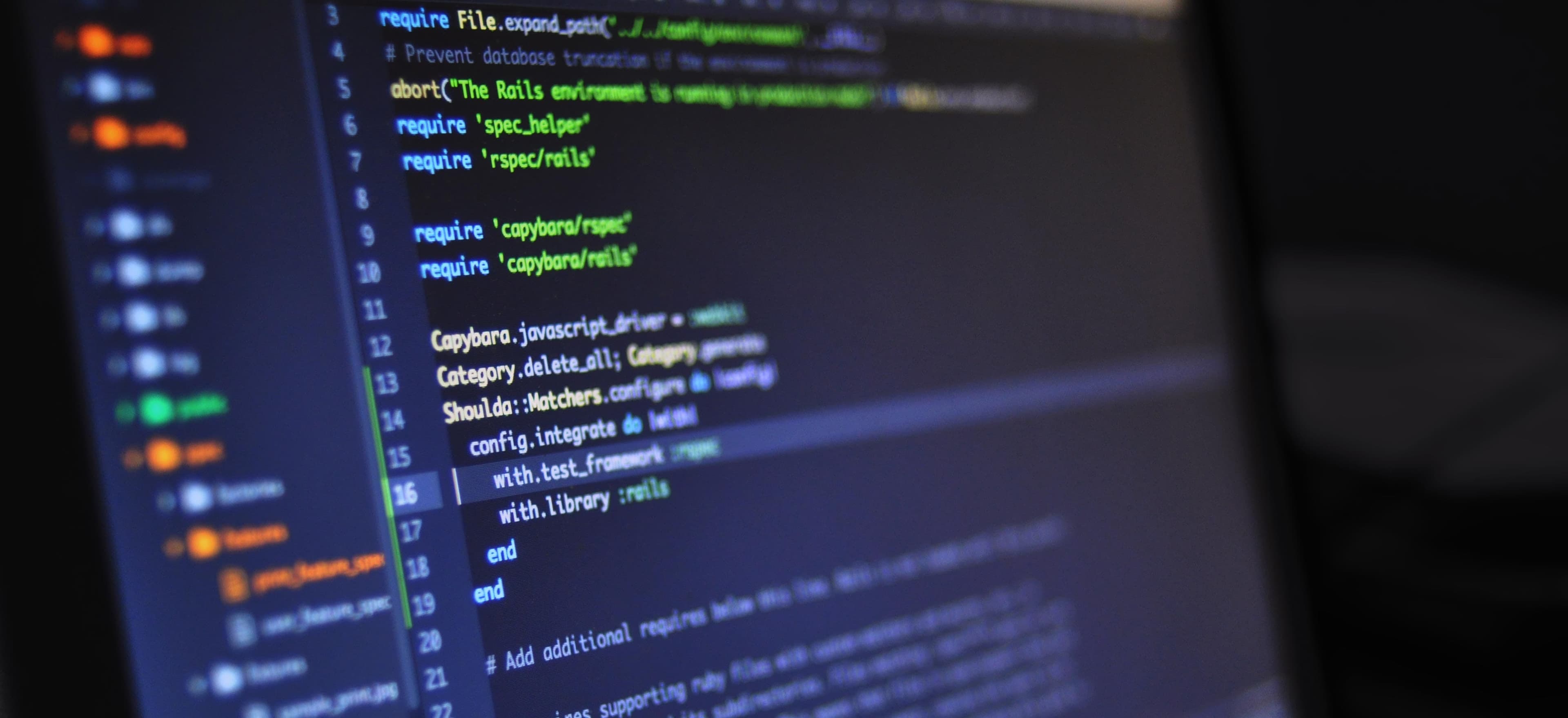
- Published on
Mastering Feature Flags for Effective Java A/B Testing
In the ever-evolving landscape of software development, A/B testing has emerged as a pivotal technique for optimizing user experience. The crux of successful A/B testing lies in its implementation, and this is where feature flags shine. In this article, we'll explore how to leverage feature flags to enhance your A/B testing strategies in Java applications, while referencing insights from our previous article, Optimizing A/B Testing with Feature Flag Configuration.
What Are Feature Flags?
Feature flags, also known as feature toggles, are a technique that allows you to enable or disable functionality within your application without deploying new code. They serve as a control mechanism that allows stakeholders to experiment with different versions of a feature seamlessly.
Benefits of Feature Flags in A/B Testing
- Granular Control: With feature flags, you can enable a feature for a subset of users, allowing you to measure its impact effectively.
- Rapid Iteration: Developers can deploy features quicker and roll back if necessary without going through a lengthy deployment process.
- Risk Mitigation: Feature flags allow for staged rollouts, minimizing the risk of negatively impacting the entire user base.
Setting Up Feature Flags in Java
Step 1: Choose a Feature Flag Library
Selecting the right feature flag library is the first step in your journey. There are several libraries available for Java, allowing for different levels of complexity and integration. One of the popular libraries is FF4J, which is open-source and easy to set up.
Step 2: Basic Implementation
Here's a simple example of how to implement feature flags using FF4J.
import org.ff4j.FF4j;
import org.ff4j.core.Feature;
public class FeatureFlagExample {
public static void main(String[] args) {
// Initialize FF4J instance
FF4j ff4j = new FF4j("ff4j.xml");
// Check if a feature is enabled
if (ff4j.check("newFeature")) {
System.out.println("New feature is enabled.");
// Executing new feature logic
runNewFeature();
} else {
System.out.println("Running default feature logic.");
runDefaultFeature();
}
}
public static void runNewFeature() {
// Logic for the new feature goes here
System.out.println("Executing new feature...");
}
public static void runDefaultFeature() {
// Logic for the default feature goes here
System.out.println("Executing default feature...");
}
}
Commentary
- Initialization: We initialize the
FF4j
instance using a configuration file (ff4j.xml), where our feature flags are defined. - Feature Check: Using
ff4j.check
, we can determine if a specific feature is enabled, allowing us to control which logic executes based on the feature state.
Step 3: Configuring Feature Flags
In the ff4j.xml
file, you could configure your feature flags like this:
<ff4j>
<feature uid="newFeature" enable="true" />
</ff4j>
Commentary
- Each feature is configured with a unique identifier (
uid
). Theenable
attribute determines whether the feature is activated. This configurability allows for quick changes without code alterations.
Integrating A/B Testing with Feature Flags
Step 1: Create Distinct User Groups
To conduct effective A/B testing, you need to create distinct user groups. For example, you might want to enable a new UI for 50% of your users while keeping 50% on the existing interface.
Step 2: Use Feature Flags for Group Assignment
Here's how to implement a simple A/B testing mechanism using feature flags:
import org.ff4j.FF4j;
import java.util.Random;
public class ABTestingWithFeatureFlags {
public static void main(String[] args) {
FF4j ff4j = new FF4j("ff4j.xml");
// Simulate user assignment to control or variant group
Random random = new Random();
boolean isVariantGroup = random.nextBoolean();
// Set feature flag based on user group
if (isVariantGroup) {
ff4j.enable("newUI");
} else {
ff4j.disable("newUI");
}
// Display the feature based on the flag status
if (ff4j.check("newUI")) {
System.out.println("Displaying new user interface...");
displayNewUI();
} else {
System.out.println("Displaying old user interface...");
displayOldUI();
}
}
public static void displayNewUI() {
// Logic for new UI goes here
System.out.println("Welcome to the new UI!");
}
public static void displayOldUI() {
// Logic for old UI goes here
System.out.println("Welcome to the old UI!");
}
}
Commentary
- Random User Assignment: Using a random boolean, we simulate the assignment of users to different groups.
- Dynamic Flag Setting: The feature flag (
newUI
) is enabled or disabled based on the group assignment, allowing for real-time A/B testing.
Monitoring and Analyzing A/B Test Results
Once your test is running, it's crucial to gather metrics to analyze the performance of each variant.
- Use analytics tools like Google Analytics or Mixpanel to track performance.
- Focus on key performance indicators (KPIs) such as conversion rates, bounce rates, and user engagement.
Lessons Learned
Feature flags are a powerful tool for Java developers engaging in A/B testing. They provide a level of control that allows for rapid experimentation and ultimately leads to improved user experiences.
With the setup outlined in this article, you can begin integrating feature flags into your A/B testing framework. Remember, the goal is to iterate quickly and analyze results efficiently. As you refine your testing process, consider revisiting our article on Optimizing A/B Testing with Feature Flag Configuration, where we delve deeper into advanced configurations and best practices.
By mastering feature flags, you can ensure your Java applications are agile, adaptable, and always aligned with the needs of your users. Happy coding!