Boost Your Java Projects with Effective Dependency Injection
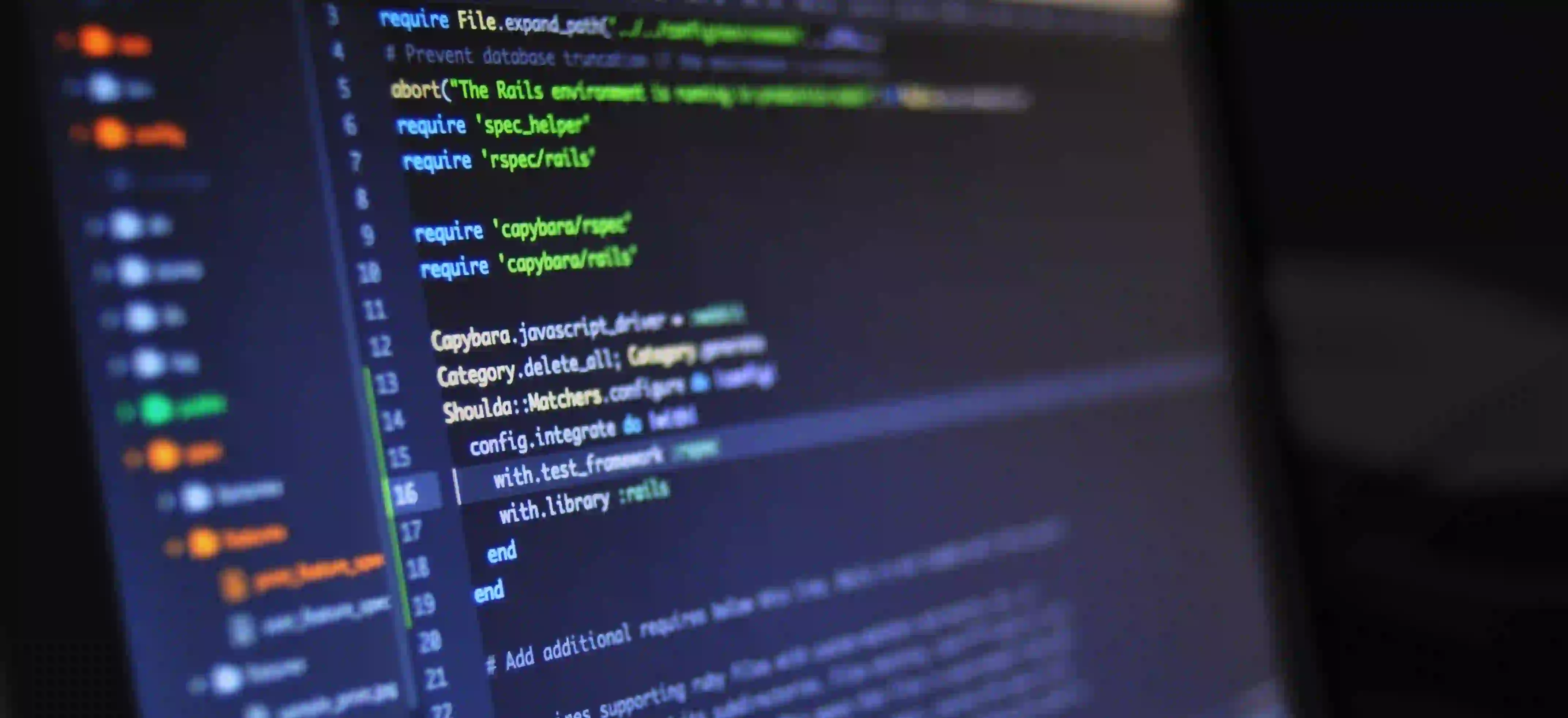
Boost Your Java Projects with Effective Dependency Injection
Java is a powerful programming language widely used for building robust applications. One of the critical practices that can enhance the modularity and testability of your Java projects is Dependency Injection (DI). Understanding how to implement DI effectively can significantly streamline your coding process. In this post, we will explore the fundamentals of Dependency Injection, its advantages, and practical examples that you can use in your own Java projects.
What is Dependency Injection?
Dependency Injection is a design pattern that allows a programmer to remove hard-coded dependencies and instead rely on external sources for object creation. In simpler terms, it enables you to write code that is less tightly coupled and more maintainable.
Types of Dependency Injection
There are three main types of Dependency Injection:
- Constructor Injection: Dependencies are provided through a class constructor.
- Setter Injection: Dependencies are set through public setter methods after the object is created.
- Interface Injection: The dependency provides an injector method that will inject the dependency into any client that passes the injector.
As a Java developer, you will often find yourself using Constructor and Setter Injection, as these are the most common approaches.
Why Use Dependency Injection?
Adopting Dependency Injection in your projects has multiple advantages:
- Increased Testability: As components are easier to isolate, unit testing becomes more straightforward.
- Improved Code Maintainability: Changes to dependencies require less reworking of the dependent classes.
- Enhanced Modularity: Code becomes more modular, leading to better organization and reduced complexity.
Implementation of Dependency Injection in Java
Let’s take a closer look at how you can implement Dependency Injection in your Java projects with some practical examples.
1. Constructor Injection Example
Constructor Injection is typically the preferred method, as it ensures that once a class is created, its dependencies are already injected.
public class Service {
private final Repository repository;
// Constructor Injection
public Service(Repository repository) {
this.repository = repository;
}
public void execute() {
repository.performAction();
}
}
public class Repository {
public void performAction() {
System.out.println("Action performed.");
}
}
public class Main {
public static void main(String[] args) {
Repository repository = new Repository();
Service service = new Service(repository);
service.execute();
}
}
Why Constructor Injection?
- It enforces immutability: the repository reference cannot change after the
Service
is constructed. - It makes it clear what dependencies the class requires.
2. Setter Injection Example
Setter Injection offers flexibility, allowing you to change dependencies after the object construction.
public class Service {
private Repository repository;
// Setter Injection
public void setRepository(Repository repository) {
this.repository = repository;
}
public void execute() {
if (repository != null) {
repository.performAction();
} else {
System.out.println("Repository not initialized.");
}
}
}
public class Repository {
public void performAction() {
System.out.println("Action performed.");
}
}
public class Main {
public static void main(String[] args) {
Service service = new Service();
Repository repository = new Repository();
service.setRepository(repository);
service.execute();
}
}
Why Setter Injection?
- It provides the ability to change the dependency at runtime.
- It can be useful when dependencies are optional.
3. Using Frameworks for Dependency Injection
While you can implement DI manually as shown above, it is often more convenient to use existing frameworks. One popular framework for Dependency Injection in Java is Spring. Spring can handle the boilerplate code and complex configuration, letting you focus on writing your application logic.
To understand how you can leverage Spring for Dependency Injection, let's take a quick look:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="repository" class="com.example.Repository"/>
<bean id="service" class="com.example.Service">
<constructor-arg ref="repository"/>
</bean>
</beans>
In a Spring application context, you can define beans and their dependencies in XML or using Java annotations. This practice allows for cleaner configurations and easier scalability of your applications.
Best Practices for Dependency Injection
- Favor Constructor Injection: Use constructor injection whenever possible to promote immutability and clarity.
- Be Cautious with Setter Injection: While it offers flexibility, too much reliance on it can make your classes harder to understand.
- Utilize Dependency Injection Frameworks: Frameworks like Spring, Guice, and Dagger can simplify your DI management, eliminating the need for boilerplate code.
- Keep Dependencies to a Minimum: Aim to limit the number of dependencies in any class to reduce complexity.
Additional Resources
If you're looking to dive deeper into Dependency Injection and improve your coding practices, check out this article titled Streamline Your Code: Mastering Dependency Injection with StructureMap. This resource provides additional insight into StructureMap, a DI framework designed for .NET but useful for understanding core DI principles that are applicable across languages.
Final Considerations
Effectively using Dependency Injection can lead to more manageable, scalable, and testable code in your Java projects. By adopting this pattern, you can significantly boost your productivity and the quality of your applications.
By integrating Dependency Injection through either manual methods or frameworks, you empower your code to evolve and change easily, ultimately enhancing collaboration, maintainability, and adaptability in your software projects. Keep these practices in mind when developing your next Java application, and watch your code transform into a more streamlined and maintainable structure.
Now that you have a foundational understanding of Dependency Injection in Java, it’s time to implement these concepts into your own projects. Don’t hesitate to experiment and find the best practices that work for you!