Enhancing Java Applications with Feature Flags for A/B Testing
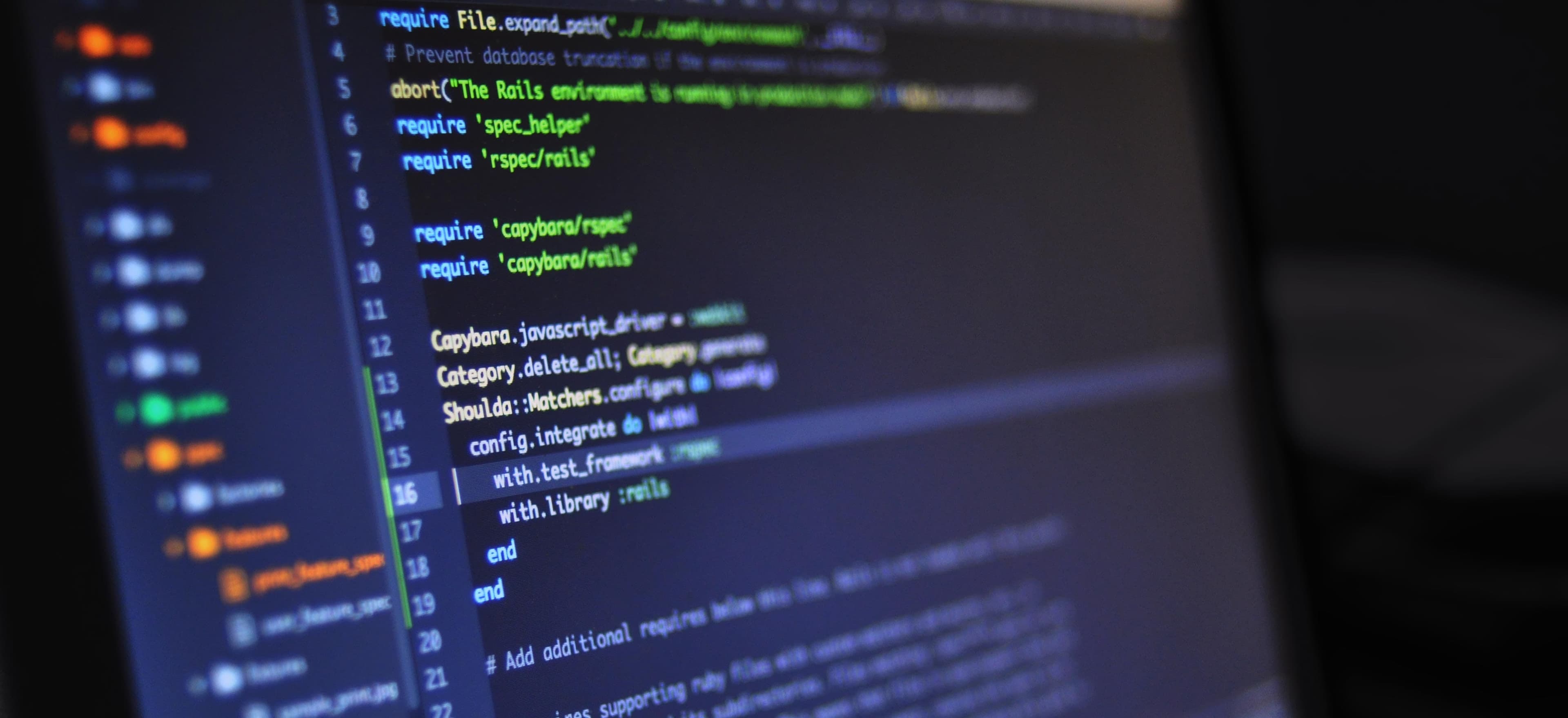
- Published on
Enhancing Java Applications with Feature Flags for A/B Testing
In the continuously evolving landscape of software development, the need for robust and flexible applications is more pertinent than ever. One effective method that has gained traction among developers is the use of feature flags (or feature toggles). This technique allows for the incremental rollout of features and is particularly advantageous when performing A/B testing. In this blog post, we will delve into the essentials of implementing feature flags in Java applications, their benefits, and how they pair beautifully with A/B testing.
What Are Feature Flags?
Feature flags are essentially conditional statements that enable you to turn features on or off in your application without deploying new code. This means you can experiment with new features on a subset of users or even among internal team members. By utilizing feature flags, development teams can mitigate risk, gather feedback, and iterate based on real-time data. This approach closely aligns with agile development methodologies, which prioritize adaptability and responsiveness.
Why Use Feature Flags in A/B Testing?
A/B testing is a powerful methodology used to compare two versions of a web page or app against each other to determine which performs better. When coupled with feature flags, A/B testing empowers developers to:
- Test features in production with real users.
- Roll back features quickly if something goes wrong.
- Analyze results based on defined metrics, making data-driven decisions.
One insightful article titled Optimizing A/B Testing with Feature Flag Configuration outlines strategies for efficiently managing feature flags and A/B testing. This blog will build upon those principles while providing practical examples relevant to Java developers.
How to Implement Feature Flags in Java
Below, we’ll take a closer look at implementing feature flags in a Java application, utilizing a simple example.
Basic Structure of a Feature Flag
Here is a straightforward example of a feature flag implementation in Java:
public class FeatureFlag {
private final boolean isEnabled;
public FeatureFlag(boolean isEnabled) {
this.isEnabled = isEnabled;
}
public boolean isEnabled() {
return isEnabled;
}
}
Usage in a Java Application
In a practical scenario, you might want to apply a feature flag to control access to a new feature within your application, such as a "New User Dashboard". Here's how you could implement that:
public class UserDashboard {
private final FeatureFlag newDashboardFlag;
public UserDashboard(FeatureFlag newDashboardFlag) {
this.newDashboardFlag = newDashboardFlag;
}
public void display() {
if (newDashboardFlag.isEnabled()) {
displayNewDashboard();
} else {
displayOldDashboard();
}
}
private void displayNewDashboard() {
// Implementation of the new dashboard
System.out.println("Displaying the new user dashboard.");
}
private void displayOldDashboard() {
// Implementation of the old dashboard
System.out.println("Displaying the old user dashboard.");
}
}
Explanation of the Code
-
FeatureFlag Class: This class encapsulates the state of a feature flag using a boolean variable (
isEnabled
). This modular approach enhances readability and maintainability. -
UserDashboard Class: This is where the feature flag is put into action. By checking if the
newDashboardFlag
is enabled, we can control the flow of the application seamlessly. If the new dashboard is enabled, users see the updated version; otherwise, they revert to the classic layout.
Initializing Feature Flags
To initialize and use the feature flags, you would do something like this:
public class Application {
public static void main(String[] args) {
FeatureFlag newDashboard = new FeatureFlag(true); // Toggle to 'false' to disable
UserDashboard userDashboard = new UserDashboard(newDashboard);
userDashboard.display();
}
}
Benefits of This Implementation
- Simplicity: The
FeatureFlag
class makes it simple to manage the status of features. - Maintainability: Adding new feature flags becomes straightforward, helping teams manage multiple toggles without clutter.
- Performance: Since feature flags operate at runtime, you can adjust features without the need to redeploy your application.
Managing Feature Flags in a Production Environment
While feature flags offer flexibility, managing them effectively is essential as applications grow. Here are a few best practices:
- Centralized Configuration: Store feature flags in a centralized source, such as a database or a configuration server. This allows for easier adjustments in a production environment.
- Limit Flags' Lifespan: Avoid bloat by ensuring that feature flags are temporary. Once a feature is fully rolled out, remove the associated flag to maintain clarity in the codebase.
- Analytics: When conducting A/B tests, gather analytics on user interactions with each version. This data is essential for assessing the performance of the tested features accurately.
Integrating A/B Testing with Feature Flags
When combined with A/B testing, feature flags enable you to serve different versions of a feature to different user segments. Here’s a simplified way to implement A/B testing along with feature flags:
public class ABTest {
private FeatureFlag versionA;
private FeatureFlag versionB;
public ABTest(FeatureFlag versionA, FeatureFlag versionB) {
this.versionA = versionA;
this.versionB = versionB;
}
public void runTest() {
if (Math.random() < 0.5) {
if (versionA.isEnabled()) {
System.out.println("Showing version A.");
}
} else {
if (versionB.isEnabled()) {
System.out.println("Showing version B.");
}
}
}
}
Explanation of the A/B Testing Code
- Here,
Math.random()
is used to randomly select which feature (version A or version B) is displayed to the user. This random selection mimics a typical A/B testing scenario. - The
runTest
method checks if the selected version is enabled before displaying it, ensuring users only see available features.
Final Thoughts
Incorporating feature flags in Java applications significantly enhances the A/B testing process. By allowing developers to toggle features dynamically, you can gather data, make informed decisions, and ultimately deliver a better user experience. As explored in this blog post, the practical implementation of feature flags can be simple but powerful.
For a deeper understanding of how to effectively use feature flags to optimize A/B testing, consider reading the article on Optimizing A/B Testing with Feature Flag Configuration.
Employing these strategies can help streamline your development process and create a more resilient application. Let us know how you implement feature flags and what challenges you’ve encountered along the way!