Balancing Cost and Quality in DevOps Testing Automation
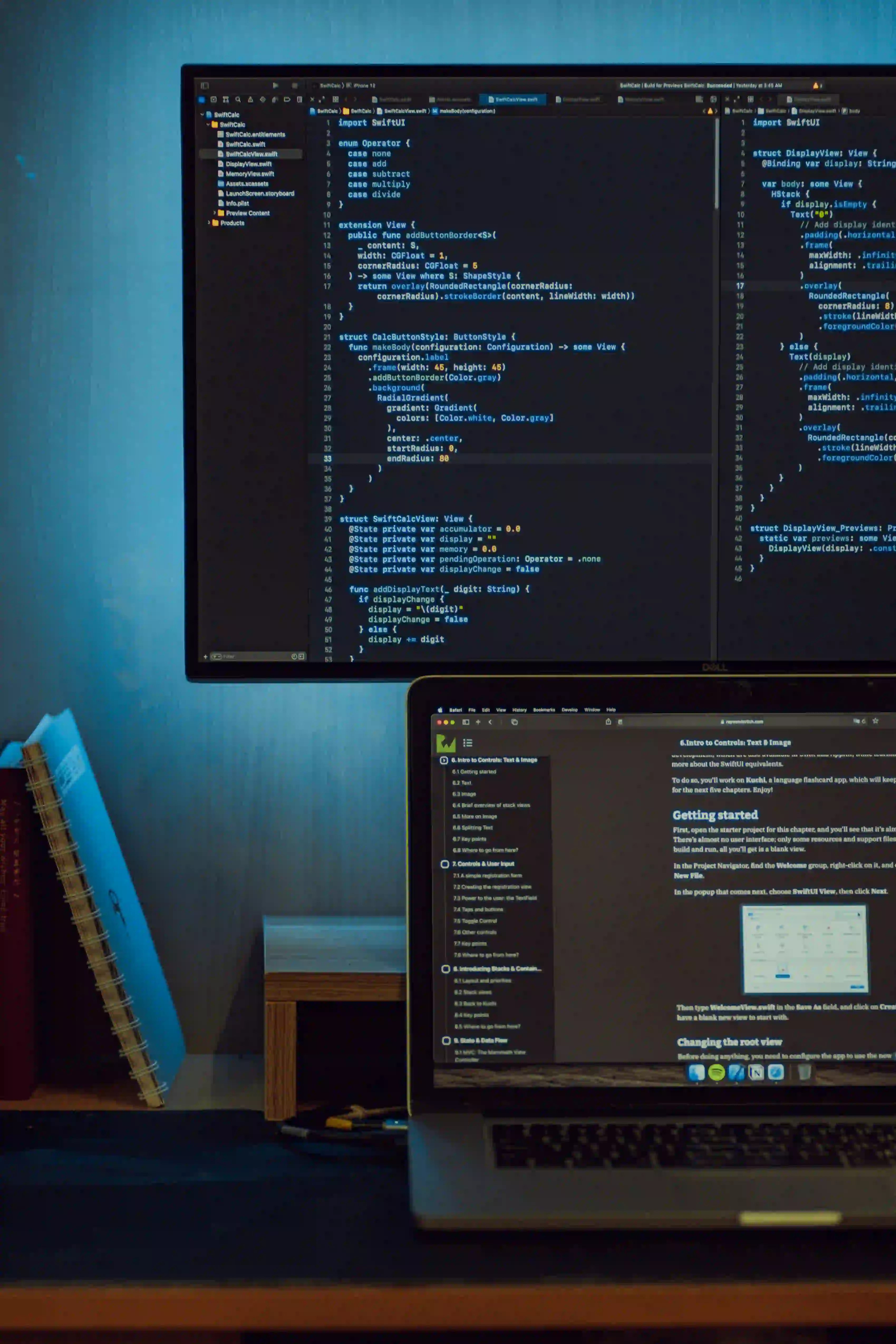
Balancing Cost and Quality in DevOps Testing Automation
In today's fast-paced software development environment, organizations are increasingly adopting DevOps practices to enhance collaboration between developers and operations teams. One of the key areas of focus in DevOps is testing automation. However, as companies look to automate their testing processes, they often find themselves grappling with a critical challenge: how to balance cost and quality. In this blog post, we will explore the intricacies of this balance and how Java can be utilized to achieve effective DevOps testing automation.
Understanding the Importance of Testing Automation
Before delving into the specifics, it's crucial to understand why testing automation is important. Testing automation allows teams to:
-
Accelerate Release Cycles: Automated tests can be run quickly and repeatedly, reducing the time it takes to validate code changes. This is vital in a DevOps environment where continuous delivery is the goal.
-
Improve Accuracy and Reliability: Manual testing is prone to human error. Automated tests provide consistent results, enhancing the reliability of software.
-
Reduce Costs in the Long Run: Although the initial investment in automated testing tools can be significant, the long-term savings from reduced manual effort and faster time-to-market can be substantial.
-
Enhance Test Coverage: Automation allows for a broader range of tests to be executed, improving the overall quality of the software.
However, achieving the perfect balance between cost and quality in testing automation requires strategic decision-making.
The Cost-Quality Dilemma
Testing automation can be expensive, particularly when it involves complex test scenarios and the adoption of various testing tools. Investing too heavily in automation might inflate costs, while under-investing can compromise the quality of testing.
Factors Influencing Cost
-
Tooling: The choice of testing framework or tools can significantly impact the overall budget. Some tools may have high licensing fees, while others are open-source.
-
Skillset: The expertise of the team conducting the automation is crucial. High-skilled developers may demand higher salaries, which can increase costs.
-
Test Maintenance: Automated tests must be continuously maintained. Frequent changes in codebases can lead to increased costs if these tests require ongoing updates.
Striking a Balance Between Cost and Quality
Here are some strategies to balance cost and quality in your DevOps testing automation efforts:
-
Choose the Right Tools: Selecting the right automation tools is paramount. Open-source solutions like Selenium or JUnit for Java can reduce costs while still providing robust functionality.
-
Focus on High-Impact Tests: Not all tests need to be automated. Prioritize tests that provide the most value, such as regression tests or tests for critical functionalities.
-
Adopt a Test Automation Pyramid: This concept emphasizes having a larger number of unit tests (which are cheaper to run) compared to integration and end-to-end tests. This layered approach helps reduce costs while maintaining quality.
-
Continuous Monitoring: Monitor the effectiveness of your automated tests. Are they catching bugs? Are they maintained? Regular review ensures that automation doesn’t become an unnecessary cost burden.
Practical Implementation in Java
To provide a clearer picture, let’s implement a simple automated testing scenario in Java using JUnit, a widely-used testing framework. This example focuses on the cost-effective approach by utilizing a unit test.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class MathUtilsTest {
@Test
public void testAdd() {
MathUtils mathUtils = new MathUtils();
assertEquals(5, mathUtils.add(2, 3), "2 + 3 should equal 5");
}
}
Code Explanation
-
JUnit Framework: We have imported JUnit, which is an essential framework for unit testing in Java. By using JUnit, we can easily implement and manage our automated tests.
-
Test Method: The
@Test
annotation denotes that the methodtestAdd
is a test case. This allows JUnit to recognize and execute it as part of the testing framework. -
Assertions: The
assertEquals
method checks whether the result of ouradd
method matches the expected value. The error message specified provides context if the test fails.
Why This Matters
By automating this basic test, you're ensuring that any changes to the add
method in MathUtils
won't inadvertently break its core functionality. The cost associated with running this unit test is negligible compared to the potential costs of fixing bugs in production.
Scaling Automation with CI/CD
Incorporating Continuous Integration and Continuous Deployment (CI/CD) enhances the automated testing process. Automating tests as part of the CI/CD pipeline means that every code change triggers automated tests, ensuring immediate feedback.
Here’s how you can integrate JUnit tests into a CI/CD pipeline using tools like Jenkins:
-
Commit: Developers commit their changes to a shared repository.
-
Trigger Tests: Jenkins detects changes and triggers JUnit tests automatically.
-
Report Results: Jenkins can report test results through its dashboard, creating visibility on test success and failure rates.
-
Deploy: If tests pass, changes can be deployed to production automatically.
The CI/CD process combined with automated testing not only saves time but also creates a safety net to ensure that quality remains high while keeping costs in check.
Emphasizing Continuous Learning and Adaptation
Finally, balancing cost and quality is not a one-time effort. It requires continuous learning and adaptation. Keep an eye on emerging tools, methodologies, and best practices in testing automation. Join communities, attend webinars, and read current articles to stay abreast of innovations.
For further insight into the importance of testing in DevOps, check out DevOps Testing Strategies and Benefits of Test Automation.
Final Thoughts
Balancing cost and quality in DevOps testing automation is essential for thriving in a competitive software landscape. By choosing the right tools, focusing on high-impact tests, and integrating automation into your CI/CD pipeline, you can effectively manage both aspects. With Java's powerful frameworks and solid practices, you can streamline your testing processes, reduce costs, and ensure high-quality software delivery.
Remember, the ideal scenario is not simply about automating tests; it's about intelligently automating them to derive real value. As you implement these strategies, keep iterating on your approach, and you'll find the right equilibrium for your unique situation.