Mastering SQL Window Functions: Avoiding Common Mistakes
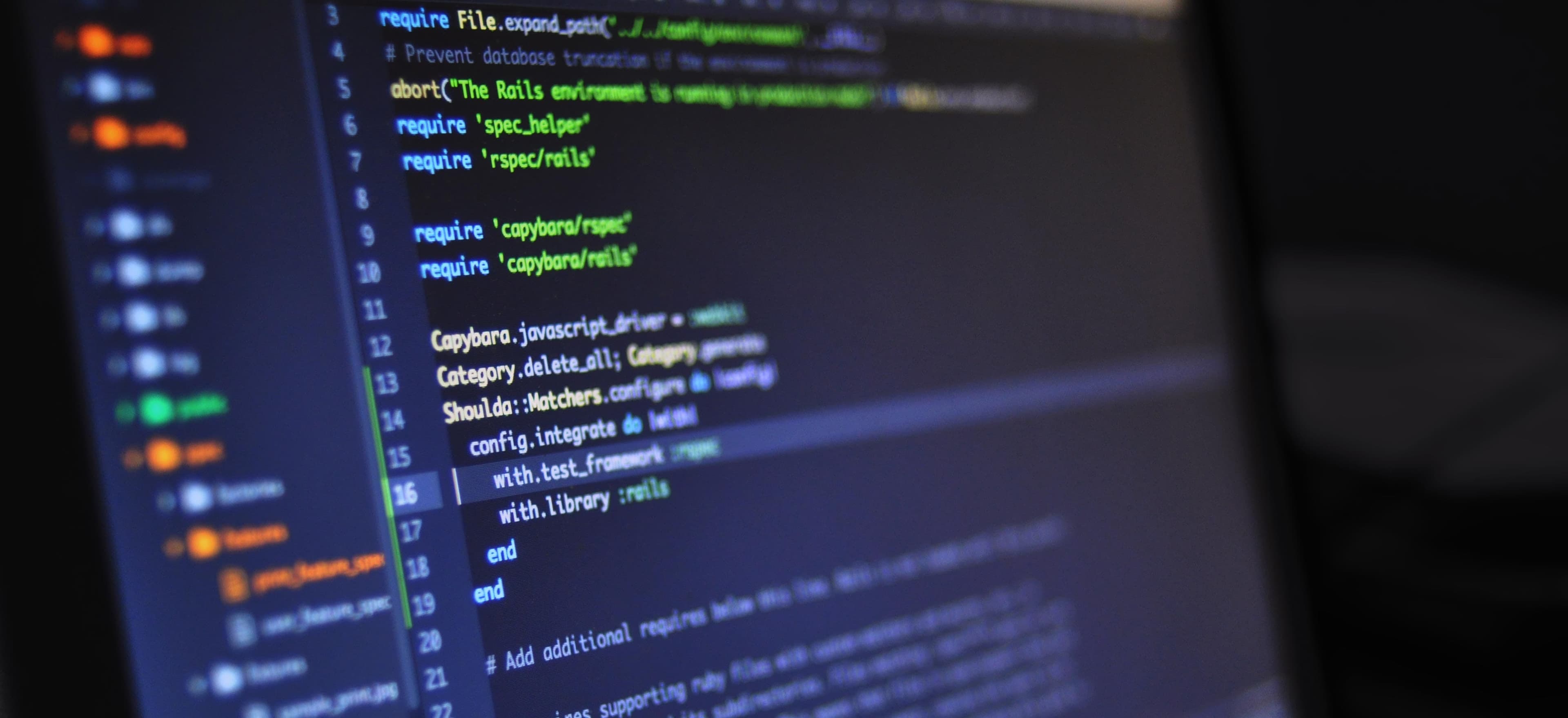
- Published on
Mastering SQL Window Functions: Avoiding Common Mistakes
SQL window functions are an essential yet oft-misunderstood component of SQL. They allow users to perform calculations across a set of rows related to the current row, without collapsing the result set. This capability makes them incredibly powerful for analytical tasks. However, many developers encounter pitfalls that can lead to inefficient queries or unexpected results. In this blog post, we will explore common mistakes with SQL window functions, guide you towards mastering their use, and provide illustrative code snippets to reinforce your understanding.
What Are SQL Window Functions?
Before diving into the common pitfalls, let’s clarify what window functions are. Unlike aggregate functions that summarize data (e.g., SUM()
, AVG()
), window functions, such as ROW_NUMBER()
, RANK()
, and LEAD()
, allow calculations across a set of rows while retaining the individual rows in the output.
Example of a Window Function
Here’s a basic example that uses the ROW_NUMBER()
window function:
SELECT
employee_id,
employee_name,
department_id,
ROW_NUMBER() OVER (PARTITION BY department_id ORDER BY salary DESC) AS salary_rank
FROM
employees;
Why this matters: This query ranks employees within each department based on their salary, giving us insights into the top earners while still listing all employees.
Common Mistakes in SQL Window Functions
Now that we have a baseline understanding of window functions, let’s explore some frequent pitfalls developers encounter.
Mistake 1: Forgetting the OVER() Clause
The most fundamental mistake is omitting the OVER()
clause when defining a window function.
Example of the Mistake
SELECT
employee_id,
employee_name,
SUM(salary) AS total_salary
FROM
employees;
What’s Wrong: This code snippet is trying to use an aggregate function without a grouping, so it results in an error.
Correct Usage
SELECT
employee_id,
employee_name,
SUM(salary) OVER () AS total_salary
FROM
employees;
Why this matters: Including OVER()
allows the SQL engine to know how to partition or order the window for calculations.
Mistake 2: Improper Partitioning
Partitioning is vital for defining how to group rows for window functions. Often, developers forget to partition by the right column.
Example of the Mistake
SELECT
employee_id,
employee_name,
DENSE_RANK() OVER (ORDER BY salary DESC) AS salary_rank
FROM
employees;
What’s Wrong: This query ranks salaries globally rather than within departments.
Correct Usage
SELECT
employee_id,
employee_name,
DENSE_RANK() OVER (PARTITION BY department_id ORDER BY salary DESC) AS salary_rank
FROM
employees;
Why this matters: Correctly partitioning ensures that the analysis is relevant to the desired context (in this case, by department).
Mistake 3: Ignoring NULL Values
Many developers overlook how NULL values affect analytical calculations within window functions.
Example of the Mistake
SELECT
employee_id,
employee_name,
LEAD(salary, 1) OVER (ORDER BY salary) AS next_salary
FROM
employees;
What’s Wrong: If there are NULL values in salary, it could lead to misleading results regarding subsequent salaries.
Correct Usage
SELECT
employee_id,
employee_name,
LEAD(salary, 1, 0) OVER (ORDER BY salary) AS next_salary
FROM
employees;
Why this matters: Using the default value in the LEAD function helps ensure that NULLs don’t skew your results.
Mistake 4: Not Using Frames Properly
Frames are a way to specify subsets of rows within the defined partition. Developers sometimes do not optimize this aspect.
Example of the Mistake
SELECT
employee_id,
SALARY,
AVG(salary) OVER (PARTITION BY department_id) AS avg_salary
FROM
employees;
What’s Wrong: This averages the entire department’s salary with multiple rows, instead of rolling averages which can be insightful.
Correct Usage
SELECT
employee_id,
salary,
AVG(salary) OVER (PARTITION BY department_id ORDER BY hire_date ROWS BETWEEN UNBOUNDED PRECEDING AND CURRENT ROW) AS rolling_avg_salary
FROM
employees;
Why this matters: This frame definition allows for progressive averages that can inform decision-making processes.
Mistake 5: Overly Complex Queries
SQL window functions can combine various calculations. However, writing overly complex queries may lead to performance issues.
Example of the Mistake
SELECT
employee_id,
department_id,
ROW_NUMBER() OVER (PARTITION BY department_id ORDER BY salary) AS salary_rank,
SUM(salary) OVER (PARTITION BY department_id) AS total_salary
FROM
employees;
What’s Wrong: Loading multiple aggregations can lead to inefficiencies.
Correct Usage
Instead of combining them in a single query, separate the analyses:
WITH ranked_employees AS (
SELECT
employee_id,
department_id,
ROW_NUMBER() OVER (PARTITION BY department_id ORDER BY salary) AS salary_rank
FROM
employees
),
total_salaries AS (
SELECT
department_id,
SUM(salary) AS total_salary
FROM
employees
GROUP BY
department_id
)
SELECT
re.employee_id,
re.salary_rank,
ts.total_salary
FROM
ranked_employees re
JOIN
total_salaries ts ON re.department_id = ts.department_id;
Why this matters: Breaking complex queries into CTEs (Common Table Expressions) can improve readability and performance.
Final Thoughts
Mastering SQL window functions can significantly enhance your ability to perform complex data analyses efficiently. However, avoiding common mistakes is essential for leveraging their full potential. From the importance of the OVER()
clause to understanding how partitioning, NULL values, and frames work, each element plays a vital role.
Want to dive deeper into SQL best practices? Consider checking SQL Window Function Documentation and explore more about analytical functions, which can further broaden your SQL skills.
Always remember: practice is key! By applying what you read in real-world scenarios, you will solidify your understanding and become proficient in SQL window functions. Happy querying!