Essential Tools Every Java Developer Must Master Today!
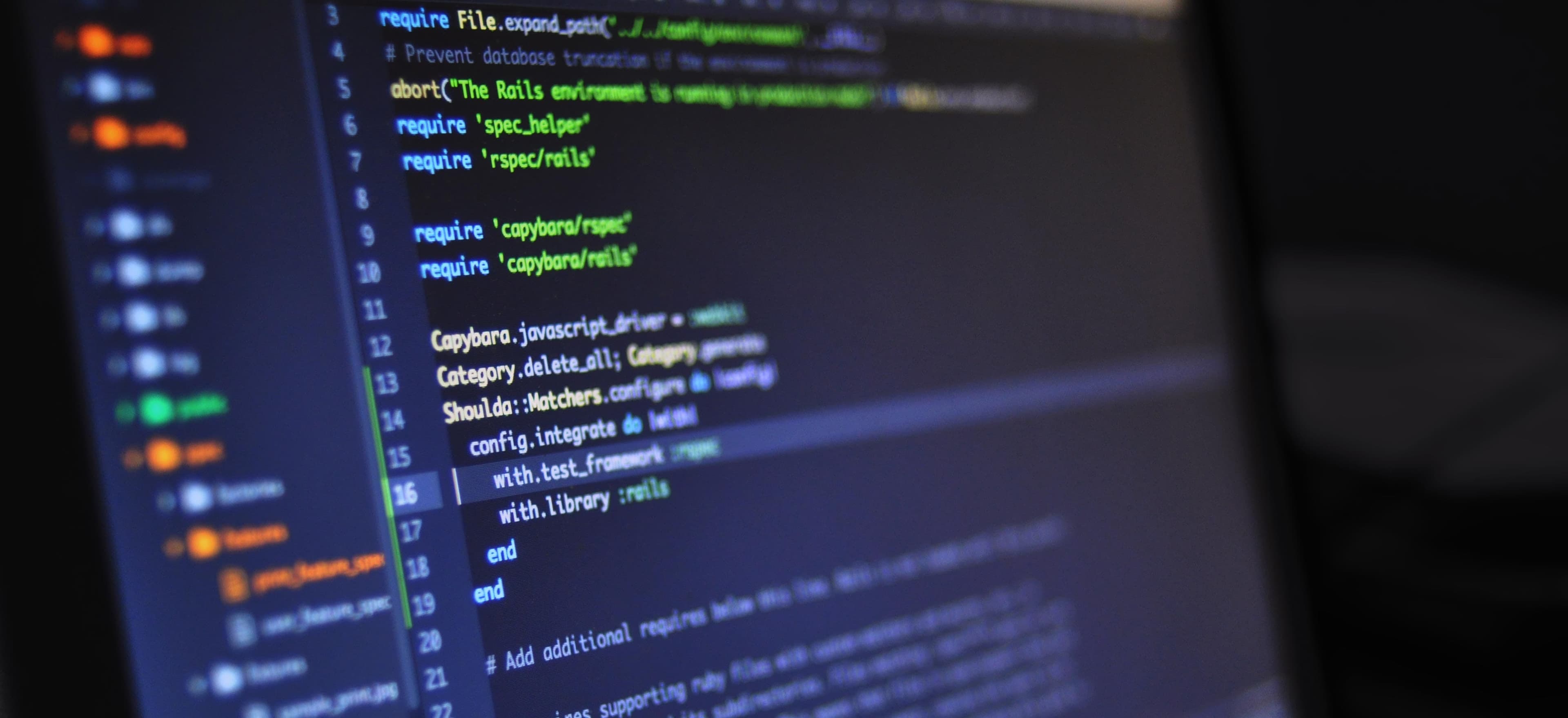
- Published on
Essential Tools Every Java Developer Must Master Today!
Java continues to be one of the most popular programming languages in the world, primarily due to its versatility, ease of use, and robust community support. Whether you're working on web applications, enterprise solutions, or mobile applications, mastering the right tools can significantly enhance your productivity. In this blog post, we’ll explore essential tools every Java developer must master today, breaking them down into categories, and providing code snippets for illustrative purposes.
Development Environments
1. Integrated Development Environment (IDE)
A powerful IDE can streamline coding, compiling, debugging, and testing processes. The most popular choices for Java development include:
- Eclipse: Offers a versatile environment with a plethora of plugins.
- IntelliJ IDEA: Known for its smart code completion and advanced refactoring features.
- NetBeans: A user-friendly option with built-in support for Maven and JavaFX.
Choosing the right IDE can improve your coding efficiency and comfort. Here’s an example of a simple Java program created in IntelliJ IDEA:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Using IntelliJ, the IntelliJ Inspector can highlight code issues, helping you correct them before running the project.
2. Build Tools
Build tools automate the creation of executable applications from source code. Two of the most widely used tools are:
-
Apache Maven: A project management tool that uses XML files (pom.xml).
Example of a simple Maven
pom.xml
file:<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>my-app</artifactId> <version>1.0-SNAPSHOT</version> </project>
-
Gradle: A modern dependency management and build tool that combines the best of Maven and Ant.
Gradle build script example:
plugins { id 'java' } group 'com.example' version '1.0-SNAPSHOT' repositories { mavenCentral() } dependencies { testImplementation 'junit:junit:4.13.2' }
Understanding these tools helps streamline your workflows and keeps project dependencies organized.
Version Control Systems
3. Git
Version control is integral to collaborative software development. Git is the most widely used system, allowing you to track changes and work with branches effectively.
Snippet for initializing a new Git repository:
git init my-java-project
cd my-java-project
With Git, you can quickly revert changes, track the history of your project, and collaborate with other developers via platforms like GitHub or GitLab.
Testing Frameworks
4. JUnit
Testing is critical for maintaining code quality. JUnit is a widely used framework allowing developers to write and run repeatable tests seamlessly.
Sample unit test with JUnit:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class MathUtilsTest {
@Test
public void testAdd() {
MathUtils mathUtils = new MathUtils();
assertEquals(5, mathUtils.add(2, 3));
}
}
JUnit allows for quick feedback on changes in your code, which is crucial for the development process. Learn more about JUnit here.
Frameworks
5. Spring Framework
The Spring Framework is essential for building Java applications, especially when it comes to developing enterprise-level solutions. Spring simplifies Java development with features such as Dependency Injection (DI), Aspect-Oriented Programming (AOP), and a robust set of modules for various needs including web applications.
Spring configuration example using Java annotations:
@Configuration
public class AppConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
Mastering Spring can greatly reduce boilerplate code and improve application modularity. For detailed resources, visit the Spring Official Website.
6. Hibernate
Hibernate is a powerful Object-Relational Mapping (ORM) tool for Java. It simplifies database operations, allowing you to work with an object-oriented paradigm while interacting with relational databases.
Basic Hibernate mapping example:
@Entity
@Table(name = "employee")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Other fields and methods...
}
Understanding Hibernate is essential when dealing with database interactions in Java applications.
Logging and Monitoring
7. Log4j and SLF4J
Logging is crucial for troubleshooting and maintaining application health. Log4j and SLF4J are two popular logging frameworks in Java.
Basic example using SLF4J:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyClass {
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public void doSomething() {
logger.info("Doing something...");
}
}
Proper logging can provide insights into your application, making issue resolution faster and easier.
Continuous Integration/Continuous Deployment (CI/CD)
8. Jenkins
Jenkins is a widely used CI/CD tool that automates the building and testing of Java applications. Integrating Jenkins into your workflow can lead to more reliable deployments and faster feedback.
Here is an example of a Jenkins pipeline in a Jenkinsfile:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
}
This allows for automated builds and tests every time you push changes to your repository. Explore Jenkins here.
My Closing Thoughts on the Matter
In this post, we covered essential tools that every Java developer should master, from IDEs to testing frameworks, logging systems, and CI/CD tools. Mastery of these tools can substantially improve your coding efficiency, collaboration accuracy, and the maintainability of your applications.
Stay current, keep learning, and always look for ways to enhance your toolset so that you can continue to thrive in the dynamic world of Java development. For additional resources, websites like Java Tutorials offer many opportunities to deepen your knowledge.
Remember, the journey of a Java developer is ongoing, and the mastery of these tools is just the beginning. Happy coding!