Streamlining Git-Flow: Overcoming Jenkins Integration Hurdles
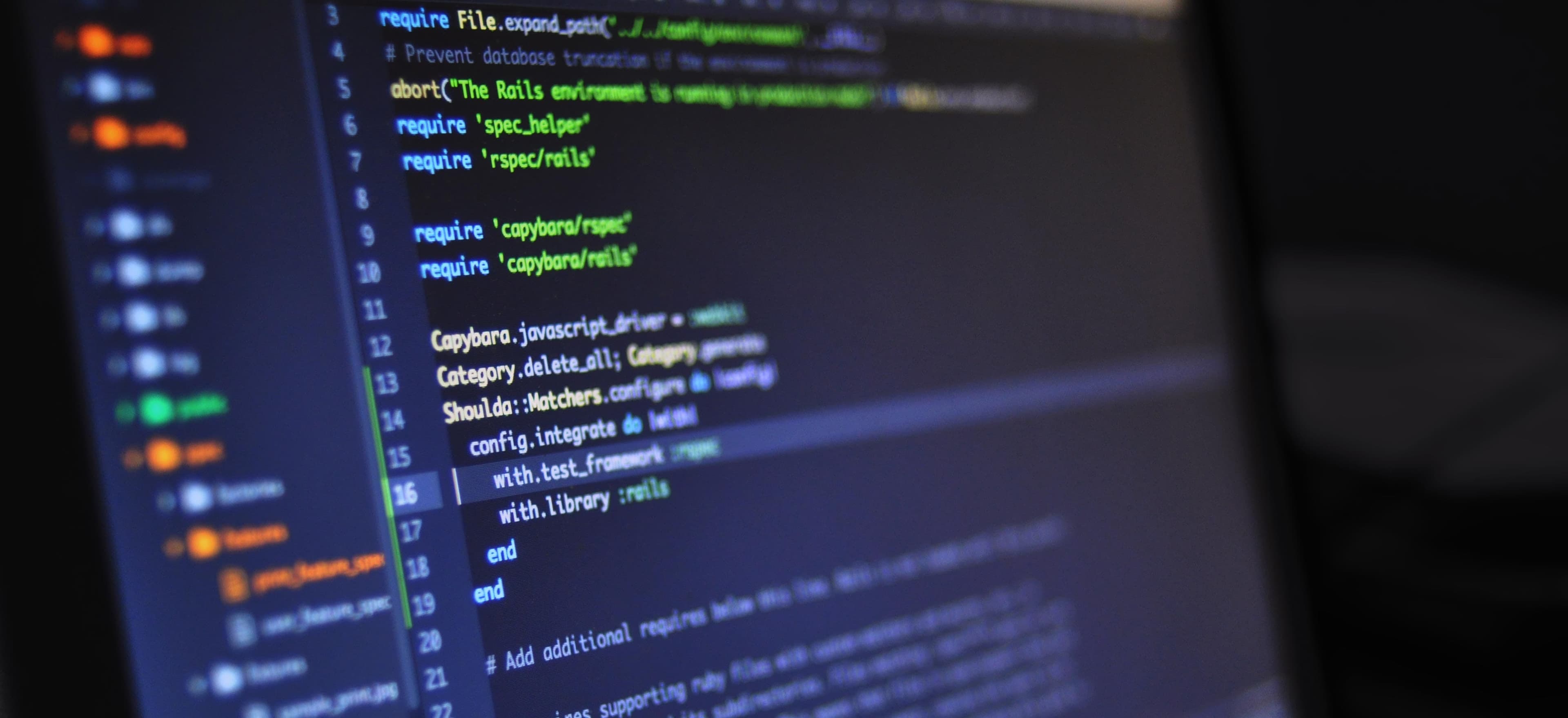
- Published on
Streamlining Git-Flow: Overcoming Jenkins Integration Hurdles
In today's robust software development landscapes, efficient workflow management is paramount. One popular methodology for source control management is Git-Flow, which provides a clear branching model for collaborative development. However, integrating Git-Flow with Continuous Integration/Continuous Delivery (CI/CD) tools like Jenkins can sometimes be challenging. In this post, we’ll explore these hurdles and provide actionable solutions to streamline your development process.
Understanding Git-Flow
Before diving into integration strategies, let's briefly cover Git-Flow. Git-Flow is an extension of Git that introduces a set of branch naming conventions and rules. Essentially, it involves maintaining the following branches:
- master: The production-ready state.
- develop: The integration branch for features.
- feature/*: New feature development branches.
- release/*: Branches for preparing the production release.
- hotfix/*: Quick fixes to production.
For more in-depth information, refer to Atlassian's Git-Flow documentation.
Jenkins: An Overview
Jenkins is one of the most popular automation servers that facilitates CI/CD. It helps automate the building, testing, and deployment processes, ultimately speeding up software delivery. However, integrating Jenkins with Git-Flow requires careful planning and configuration.
Common Integration Hurdles
1. Branch Management Complexity
Managing multiple branches can lead to confusion when configuring Jenkins. When particular builds should trigger based on specific branch activity, it becomes crucial to articulate these rules clearly.
Solution: Utilize Jenkins features like MultiBranch Pipelines. This allows Jenkins to automatically discover branches in your repository and manage their builds accordingly.
2. Merge Conflicts
Working collaboratively often leads to code merge conflicts, especially in larger teams. If multiple developers are pushing changes simultaneously, integration becomes a challenge.
Solution: Implement a Merge Strategy in your CI/CD process. Encourage frequent commits to the develop
branch, and utilize features like Git's rebase
command to maintain a clean history.
Here’s a quick code snippet on how to use rebase
:
# First, fetch the latest changes
git fetch origin
# Rebase your feature branch with the latest 'develop'
git checkout feature/my-feature
git rebase origin/develop
By keeping your feature branches up-to-date, you minimize the likelihood of conflicts during merges.
3. Environment Consistency
Inconsistencies in the development environment can lead to failed builds. Different developers might have different configurations or dependencies.
Solution: Use a Docker-based environment alongside Jenkins to ensure consistent app behavior. This can further streamline setup and provisioning. Create a Dockerfile for your application as shown below.
# Use the official Java image from the Docker Hub
FROM openjdk:11-jdk-slim
# Set the working directory
WORKDIR /app
# Copy the build artifacts
COPY target/my-app.jar /app/my-app.jar
# Run the application
ENTRYPOINT ["java", "-jar", "my-app.jar"]
Docker ensures that every developer’s environment is identical, reducing the issues introduced by discrepancies.
4. Build Triggers
Determining when to trigger builds can get complicated with multiple branches. For example, developers might want different behaviors for the develop
branch versus release
or hotfix
branches.
Solution: Configure Jenkins to use Webhooks for triggering builds seamlessly on events. GitHub and Bitbucket support webhooks, which can trigger Jenkins jobs on various events such as push and pull requests.
Here's how a webhook can be integrated in a Jenkins pipeline:
pipeline {
agent any
triggers {
githubPush() // Trigger on GitHub push events
}
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'docker run my-app:latest'
}
}
}
}
5. Monitoring and Reporting
After the deployment stage, it is vital to monitor the application and provide reports on the build status. Lack of visibility can obscure potential issues.
Solution: Jenkins offers various plugins such as the Monitoring Plugin and integration with tools like Prometheus for better resource monitoring.
My Closing Thoughts on the Matter
By focusing on these specific challenges and adopting the suggested solutions, you can significantly smooth the integration of Git-Flow with Jenkins in your DevOps framework.
Key Takeaways:
- Branch Management: Use MultiBranch Pipelines to simplify build triggers.
- Minimize Merge Conflicts: Frequent commits and rebasing practices help maintain a clean history.
- Consistency with Docker: Establish a uniform development environment for all team members.
- Efficient Build Triggers: Use webhooks for dynamic build triggers.
- Enhance Monitoring: Leverage Jenkins monitoring plugins to maintain visibility on builds and deployments.
As technology progresses, it's vital to stay updated with best practices and enhancements in tooling. Regularly revisit your workflows to adapt to these changes effectively and ensure your setup remains optimal.
For more insights on CI/CD and Jenkins best practices, check out Jenkins' Official Documentation. Happy coding!