Common WebSocket Pitfalls in Spring 4 Development
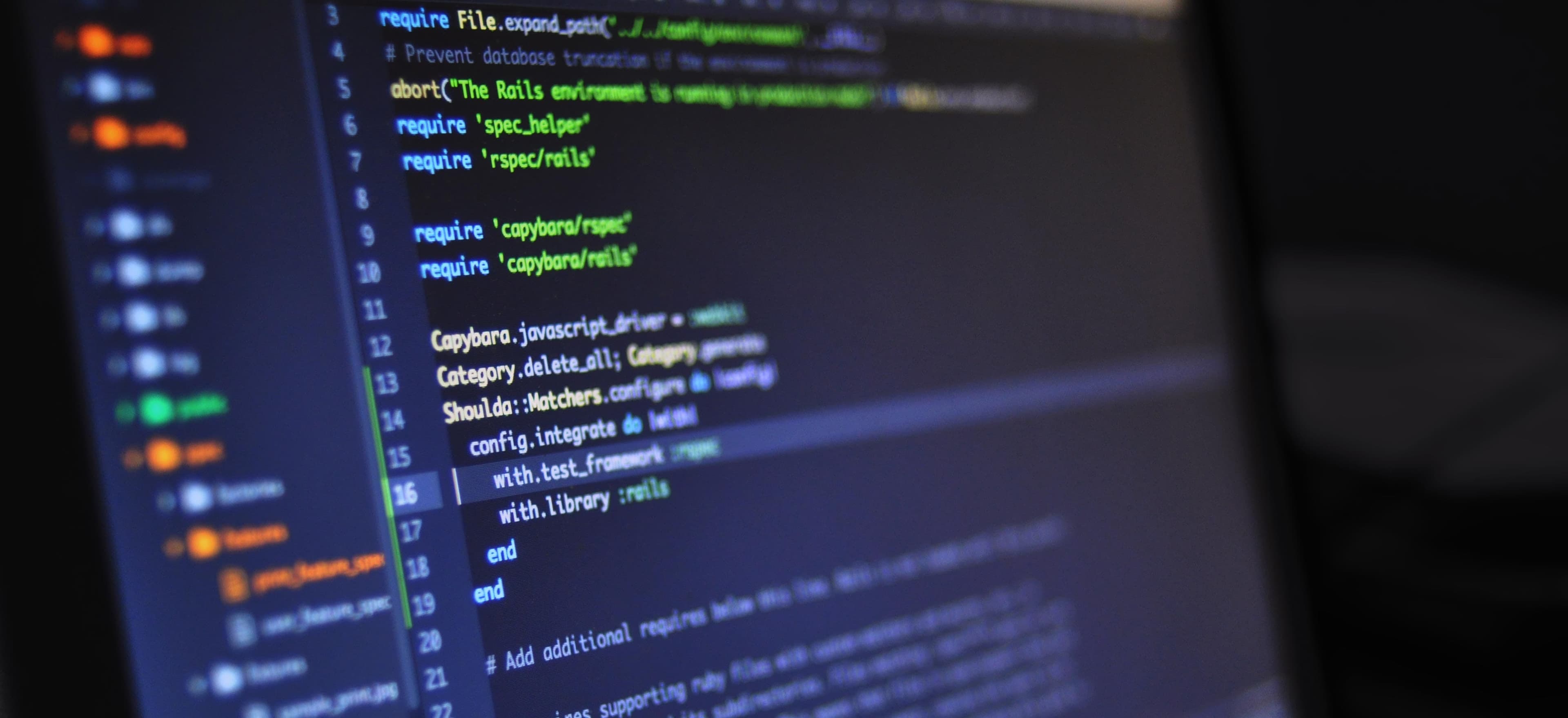
- Published on
Common WebSocket Pitfalls in Spring 4 Development
WebSocket is a powerful technology that has taken web communication to new heights. It enables real-time, two-way interaction between clients and servers, making it ideal for applications requiring constant data exchange. In the Spring Framework, particularly Spring 4, WebSocket support provides a robust foundation for building such applications. However, like any powerful tool, there are pitfalls developers often encounter. In this post, we will explore common WebSocket pitfalls in Spring 4 development and how to avoid them.
Table of Contents
- Understanding WebSocket Basics
- Common Pitfalls in Spring WebSocket Development
- 2.1 Misunderstanding WebSocket Handshake
- 2.2 Ignoring Error Handling
- 2.3 Not Implementing Security Measures
- 2.4 Failing to Handle Session Management
- 2.5 Neglecting Message Broker Configuration
- Best Practices for Spring WebSocket Development
- Conclusion
Understanding WebSocket Basics
Before diving into the pitfalls, it’s essential to understand the foundation of WebSocket. WebSocket is a standardized protocol that enables interactive communication between a web browser and a server. Unlike HTTP, which follows a request-response model, WebSocket establishes a persistent connection, allowing data to flow in both directions freely.
In a typical Spring WebSocket application, you would use annotations to configure your WebSocket endpoints. Here’s an example:
import org.springframework.web.socket.config.annotation.EnableWebSocketMessageBroker;
import org.springframework.context.annotation.Configuration;
import org.springframework.messaging.simp.config.MessageBrokerRegistry;
import org.springframework.web.socket.config.annotation.StompEndpointRegistry;
import org.springframework.web.socket.config.annotation.WebSocketMessageBrokerConfigurer;
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void configureMessageBroker(MessageBrokerRegistry config) {
config.enableSimpleBroker("/topic");
config.setApplicationDestinationPrefixes("/app");
}
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/ws").withSockJS();
}
}
Explanation:
@EnableWebSocketMessageBroker
enables WebSocket message handling.configureMessageBroker
configures the message broker used to send messages.registerStompEndpoints
registers the WebSocket endpoint and enables SockJS for compatibility with browsers that do not support WebSocket.
Now, let’s examine the common pitfalls in this context.
Common Pitfalls in Spring WebSocket Development
2.1 Misunderstanding WebSocket Handshake
WebSocket communication starts with a handshake between the client and the server. A common pitfall is not understanding or configuring this handshake correctly. Ensure your server properly handles the handshake requests. Misconfiguration can cause silent failures where WebSocket connections never establish.
Tip: Monitor handshake events in your server logs to ensure they occur without errors.
2.2 Ignoring Error Handling
Error handling is critical in any application but can be overlooked when working with WebSockets. If your WebSocket connections fail or if messages encounter issues, the lack of error handling will lead to poor user experiences or silent failures.
To manage errors in your WebSocket application, you can override the error handling methods. Here's an example:
import org.springframework.messaging.Message;
import org.springframework.messaging.MessageHandlingException;
import org.springframework.messaging.support.ErrorMessage;
import org.springframework.web.socket.messaging.SessionDisconnectEvent;
import org.springframework.context.event.EventListener;
import org.springframework.stereotype.Controller;
@Controller
public class WebSocketErrorHandler {
@EventListener
public void handleWebSocketDisconnectListener(SessionDisconnectEvent event) {
System.out.println("User Disconnected : " + event.getSessionId());
}
@MessageExceptionHandler
public void handleError(MessageHandlingException ex, Message<?> message) {
// Log the error
System.out.println("Error occurred: " + ex.getMessage());
// Additional error handling logic
}
}
Why: By managing errors, you ensure that your application can handle unexpected scenarios gracefully, providing better feedback to the user and maintaining app stability.
2.3 Not Implementing Security Measures
Security is paramount in any application exposed to the internet, including WebSocket applications. By default, WebSocket connections are not secured, making it crucial to implement proper security measures.
Spring Security can easily be integrated with WebSockets. Here’s how you might secure your WebSocket configuration:
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.builders.WebSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/ws/**").permitAll() // Adjust for your authentication
.anyRequest().authenticated()
.and()
.csrf().disable(); // CSRF protection is not needed for WebSocket
}
}
Explanation: This configuration allows you to control which users can access your WebSocket endpoints while disabling CSRF protection because WebSocket does not work with standard CSRF tokens.
2.4 Failing to Handle Session Management
Managing user sessions correctly is essential for tracking users and their connections in real-time applications. A common pitfall is not preserving user identities or mismanaging sessions, leading to unexpected behavior and loss of context.
Spring provides the SimulatedSession
class that allows you to manage user sessions effectively. Remember to keep track of connected users, especially in multiplayer or chat applications.
import org.springframework.messaging.simp.stomp.StompHeaderAccessor;
import org.springframework.messaging.simp.stomp.StompSession;
import org.springframework.stereotype.Controller;
@Controller
public class ChatController {
@MessageMapping("/chat.sendMessage")
public void sendMessage(@Payload ChatMessage chatMessage, SimpMessageHeaderAccessor headerAccessor) {
// Save the username in the session
headerAccessor.getSessionAttributes().put("username", chatMessage.getSender());
// Broadcast message to all connected clients
messagingTemplate.convertAndSend("/topic/public", chatMessage);
}
}
Why: By using session attributes, you can easily track users and maintain state information, essential for applications that require context like chat rooms.
2.5 Neglecting Message Broker Configuration
When dealing with high-load applications, neglecting the message broker configuration can lead to performance bottlenecks and message loss. In Spring, you can use either a simple message broker or implement more robust options like RabbitMQ or ActiveMQ.
By default, a simple broker is provided, but for more complex, production-grade applications, consider configuring an external message broker.
@Override
public void configureMessageBroker(MessageBrokerRegistry config) {
config.enableSimpleBroker("/topic", "/queue");
config.setApplicationDestinationPrefixes("/app");
// For RabbitMQ
config.enableStompBrokerRelay("/topic", "/queue")
.setRelayHost("localhost")
.setRelayPort(61613);
}
Why: Using an external message broker can improve scalability and reliability, especially in applications with numerous connected clients or high message throughput.
Best Practices for Spring WebSocket Development
-
Use STOMP over WebSocket: The STOMP (Streaming Text Oriented Messaging Protocol) protocol provides a better abstraction level for message handling. It allows you to define more structured messages and easily handle subscriptions.
-
Performance Monitoring: Monitor your WebSocket connections and queues to identify performance issues early. Tools like Spring Boot Actuator can help you expose operational metrics.
-
Graceful Shutdown: Implement logic to gracefully handle application shutdown, such as informing users of impending disconnections and closing WebSocket sessions properly.
-
Keep Alive Mechanism: Implement a keep-alive mechanism in your client to maintain active WebSocket connections and monitor the server’s availability.
Closing Remarks
Developing with WebSockets in Spring 4 can drastically improve user experiences through real-time communication. However, developers need to be mindful of the common pitfalls we have discussed. Understanding and addressing these issues will aid in creating a reliable, robust, and performant WebSocket application.
For more detailed guidance on Spring WebSockets, consider checking out the Spring Framework Documentation and the Spring Blog for the latest updates.
By avoiding these pitfalls and adhering to best practices, you can leverage WebSocket technology to create dynamic, responsive web applications. Happy coding!
Checkout our other articles