Enhancing Spring Data Solr: Custom Methods Simplified
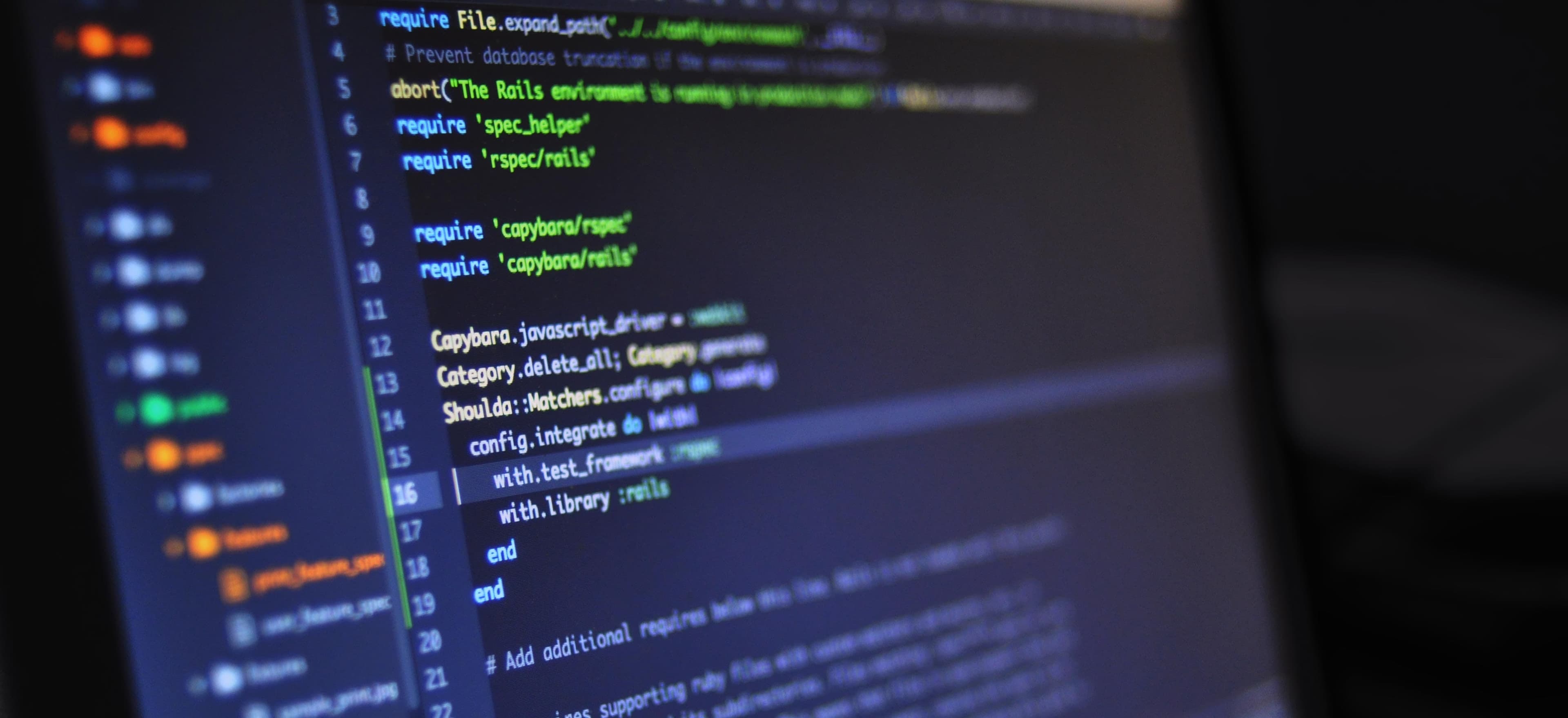
- Published on
Enhancing Spring Data Solr: Custom Methods Simplified
Spring Data Solr offers a robust solution for interacting with Apache Solr, a powerful search platform. While Spring Data Solr provides many features out of the box, creating custom methods to cater to your specific requirements can greatly enhance your application's functionality. In this blog post, we'll explore how to build custom repository methods in Spring Data Solr and why it matters.
Why Use Custom Repository Methods?
The default functionalities of Spring Data Solr include methods for CRUD operations and simple query capabilities. However, every application has unique requirements. Custom repository methods allow developers to:
- Extend the capabilities of the built-in repositories.
- Create efficient queries tailored to specific use cases.
- Improve performance by reducing unnecessary data processing.
Setting Up Your Spring Data Solr Environment
Before diving into custom methods, make sure you have a Spring Boot application configured with Spring Data Solr. If you haven't set up your project yet, follow these steps:
-
Add Dependencies: Update your
pom.xml
file to include the Spring Data Solr dependency.<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-solr</artifactId> </dependency>
-
Configure Solr: In your
application.properties
file, specify the Solr server URL and other configurations.spring.data.solr.host=http://localhost:8983/solr spring.data.solr.repositories.enabled=true
-
Create Domain Entities: Define your entity classes annotated with
@Document
.import org.springframework.data.annotation.Id; import org.springframework.data.solr.core.mapping.SolrDocument; @SolrDocument public class Product { @Id private String id; private String name; private double price; // Getters and setters }
Defining a Custom Repository Interface
Custom repository methods require defining a custom repository interface. This interface will extend the base repository and allow for additional functionality.
import org.springframework.data.solr.repository.SolrCrudRepository;
public interface CustomProductRepository extends SolrCrudRepository<Product, String> {
List<Product> findByNameAndPriceLessThan(String name, double price);
}
Implementing the Custom Repository
Now that we've defined our custom interface, let's implement it. Create an implementation class that provides the logic for the custom method.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.solr.core.SolrOperations;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class CustomProductRepositoryImpl implements CustomProductRepository {
@Autowired
private SolrOperations solrOperations;
@Override
public List<Product> findByNameAndPriceLessThan(String name, double price) {
// Create a Solr query
String queryStr = String.format("name:\"%s\" AND price:[* TO %.2f]", name, price);
return solrOperations.queryForList(queryStr, Product.class);
}
// Other methods from SolrCrudRepository can be implemented or inherited
}
Explanation of Code
In the above implementation:
- We used
SolrOperations
to interact with Solr.SolrOperations
abstracts the complexity of Solr queries and helps us retrieve data with ease. - The
findByNameAndPriceLessThan
method constructs a Solr query to find products by name and filter their prices. - The query is structured using Solr's Query Parser syntax, emphasizing why we are filtering on both
name
andprice
.
Registering the Custom Repository
Spring Data provides mechanisms to automatically detect and register custom repositories. Make sure your implementation class is in the same package or a subpackage of your Spring Boot application class.
Using the Custom Method
To utilize the custom method, simply inject your repository interface into the service layer or controller.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class ProductService {
@Autowired
private CustomProductRepository customProductRepository;
public List<Product> getAffordableProducts(String name, double maxPrice) {
return customProductRepository.findByNameAndPriceLessThan(name, maxPrice);
}
}
Testing Your Custom Method
To validate the functionality of your newly created method, write unit tests that check its behavior under various conditions.
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.data.solr.DataSolrTest;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertFalse;
@DataSolrTest
public class CustomProductRepositoryTest {
@Autowired
private CustomProductRepository customProductRepository;
@Test
public void testFindByNameAndPriceLessThan() {
List<Product> products = customProductRepository.findByNameAndPriceLessThan("Laptop", 1000);
assertFalse(products.isEmpty(), "Expected products should not be empty");
}
}
Best Practices for Custom Repository Methods
- Keep it Simple: Only add custom methods that are absolutely necessary for your application.
- Optimize Queries: Ensure your queries are optimized to prevent performance bottlenecks.
- Document Your Code: Always add comments explaining complex logic in your custom repository methods, aiding future maintainability.
The Bottom Line
Enhancing Spring Data Solr with custom repository methods can significantly improve the functionality and performance of your application. By following the steps laid out in this blog post, you can easily develop tailored solutions that meet your specific data retrieval needs.
With a little creativity and clear understanding of your requirements, you can turn Spring Data Solr into a powerful tool capable of efficiently managing complex queries. If you have further questions or would like to share your experiences, please leave a comment below!
For additional insights into Spring Data Solr or related technologies, consider visiting Spring.io and the Apache Solr documentation.
Checkout our other articles