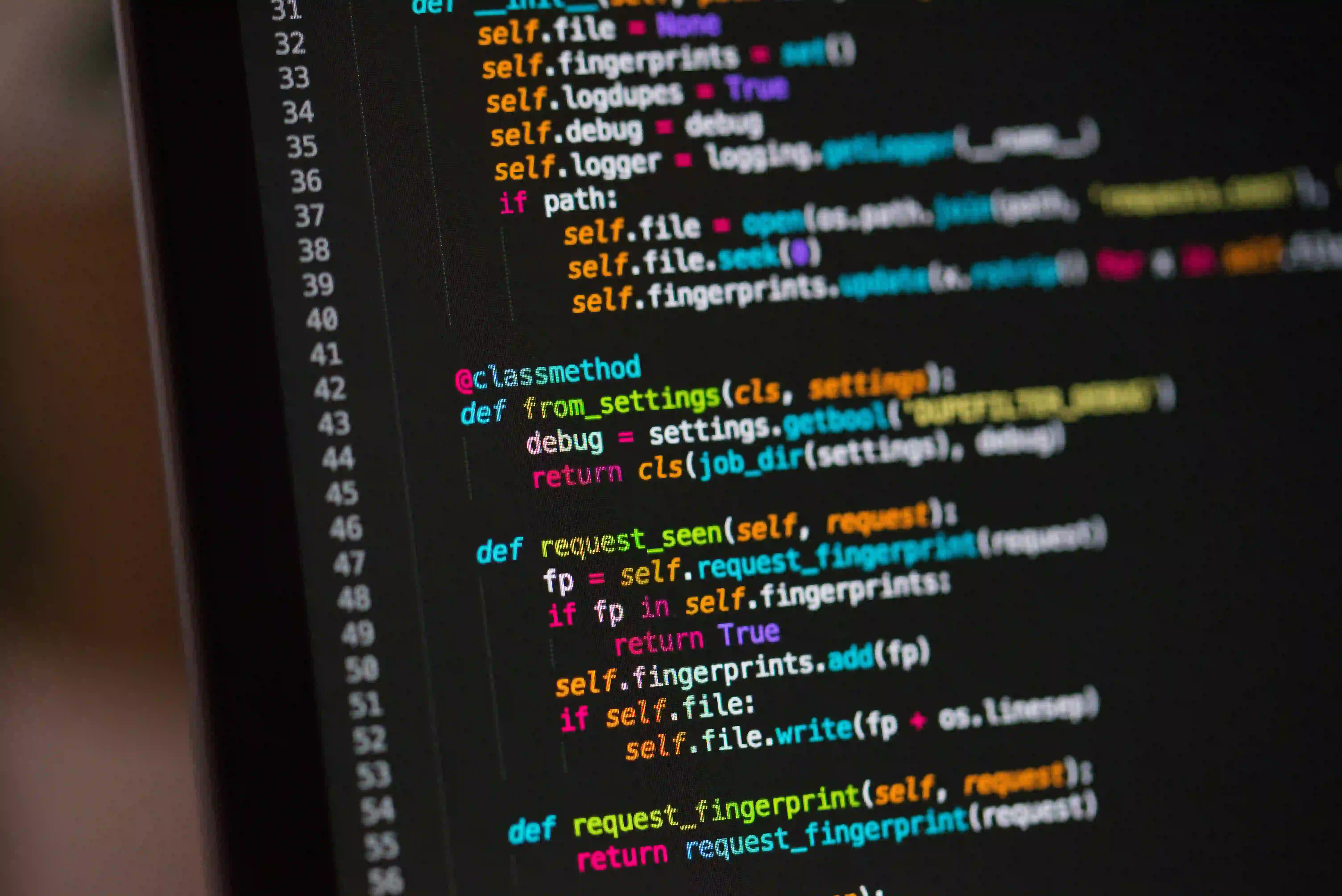
Common Spring Security Pitfalls and How to Avoid Them
Spring Security is powerful and incredibly useful for securing Java applications. However, its flexibility can lead developers into common pitfalls. In this blog post, we'll explore these pitfalls and provide best practices to avoid them.
Understanding the Basics of Spring Security
Before diving into pitfalls, it’s crucial to understand what Spring Security is. It's a powerful and customizable authentication and access-control framework for Java applications, widely used to secure web applications. It integrates seamlessly with Spring, ensuring you can secure your application with minimal effort.
To get started with Spring Security, be sure to visit the official Spring Security documentation.
Pitfall 1: Inadequate Configuration of Authentication Providers
Issue
Many developers focus solely on configuring the authentication provider for user credentials without considering the implications of different types of authentication (like OAuth, LDAP, etc.). Failing to properly configure the chosen authentication method can lead to potential security vulnerabilities.
Solution
Whenever you set up an authentication provider, ensure there’s a clear understanding of it within your application. For example, if you're using in-memory authentication for testing, it's crucial to switch to a more secure method (like JDBC or LDAP) for production.
Example Code
Here's a simple example of how to configure in-memory user authentication:
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER")
.and()
.withUser("admin").password(passwordEncoder().encode("admin")).roles("ADMIN");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
Why This Matters: In this code snippet, we use BCrypt for password encoding, which protects user credentials from being stored in plain text. Weak or missing password encoding is a common oversight that can lead to security breaches.
Pitfall 2: Ignoring CSRF Protection
Issue
Cross-Site Request Forgery (CSRF) can lead to unauthorized commands being transmitted from a user. Despite Spring Security enabling CSRF protection by default, developers sometimes disable this feature, either by misunderstanding its importance or for ease of development.
Solution
Always keep CSRF protection enabled and utilize tokens in your web forms. This ensures that requests are coming from legitimate sources only.
Example Code
To ensure CSRF protection is in place, you can check your WebSecurityConfigurerAdapter
configuration as follows:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().and() // CSRF protection enabled
.authorizeRequests()
.anyRequest().authenticated();
}
Why This Matters: The csrf()
method in the configuration ensures that CSRF tokens are required for form submissions. Neglecting this can expose your application to malicious requests.
Pitfall 3: Misconfigured URL Access Control
Issue
Another common mistake arises from improperly configured URL access restrictions. It's tempting to broadly allow access and only restrict specific endpoints, which can expose sensitive data or functions to unauthorized users.
Solution
Be explicit with your access control policies. Configure secure endpoints clearly and limit access only to users who should have it.
Example Code
Here's how to specifically block access to a URL:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN") // Only users with ADMIN role can access
.anyRequest().authenticated(); // All other requests must be authenticated
}
Why This Matters: In the above code, access is strictly handled. Identifying roles and ensuring that only the right users have access to certain URLs prevents unauthorized access to sensitive features.
Pitfall 4: Relying on Default Settings
Issue
Developers often deploy applications with default Spring Security settings. While defaults can be convenient during development, they may not be secure in production environments.
Solution
Always review and customize defaults based on the specific requirements of your application.
Example Approach
-
Enable HTTPS: Ensure that your application communicates over HTTPS to protect data in transit.
-
Custom Error Pages: Override the default error pages to prevent revealing sensitive information about your application's internal structure.
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.requiresChannel()
.anyRequest().requiresSecure() // Redirect to HTTPS
.and()
.exceptionHandling()
.accessDeniedPage("/403") // Custom error page
.authenticationEntryPoint(new LoginUrlAuthenticationEntryPoint("/login"));
}
Why This Matters: Customizing error and access handling can obscure your application's structure from potential attackers, adding another layer of security.
Pitfall 5: Not Utilizing Security Context
Issue
Developers sometimes neglect the importance of the Security Context in their code, which is essential for checking the currently authenticated user's roles and authorities.
Solution
Always use the Security Context to retrieve the authenticated user's information when necessary. This helps with role-based authorization checks within your application logic.
Example Code
Here’s how you can extract user details from the Security Context:
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
String username = authentication.getName(); // Current Username
boolean isAdmin = authentication.getAuthorities().stream()
.anyMatch(auth -> auth.getAuthority().equals("ROLE_ADMIN"));
if (isAdmin) {
// Perform admin-specific logic
}
Why This Matters: This practice allows you to enforce permissions programmatically within your business logic, utilizing a secure and robust approach to user roles.
Key Takeaways
Spring Security is an essential framework for securing Java applications, but the associated pitfalls can lead to significant security vulnerabilities. By understanding these common issues and their solutions, you can make informed decisions that enhance the security of your applications.
By focusing on proper authentication configuration, ensuring CSRF protection, meticulously managing access controls, avoiding default settings, and utilizing the Security Context, developers can fortify their applications against threats.
For more information and resources, you may explore Baeldung's comprehensive guide on Spring Security, which offers in-depth tutorials and examples.
With vigilance and adherence to best practices, you can harness the power of Spring Security effectively while safeguarding your applications against potential exploits. Remember, security is a continuous process that requires ongoing education and adaptation to new threats.