Mastering Redis Streams: Handling Data Loss in Java
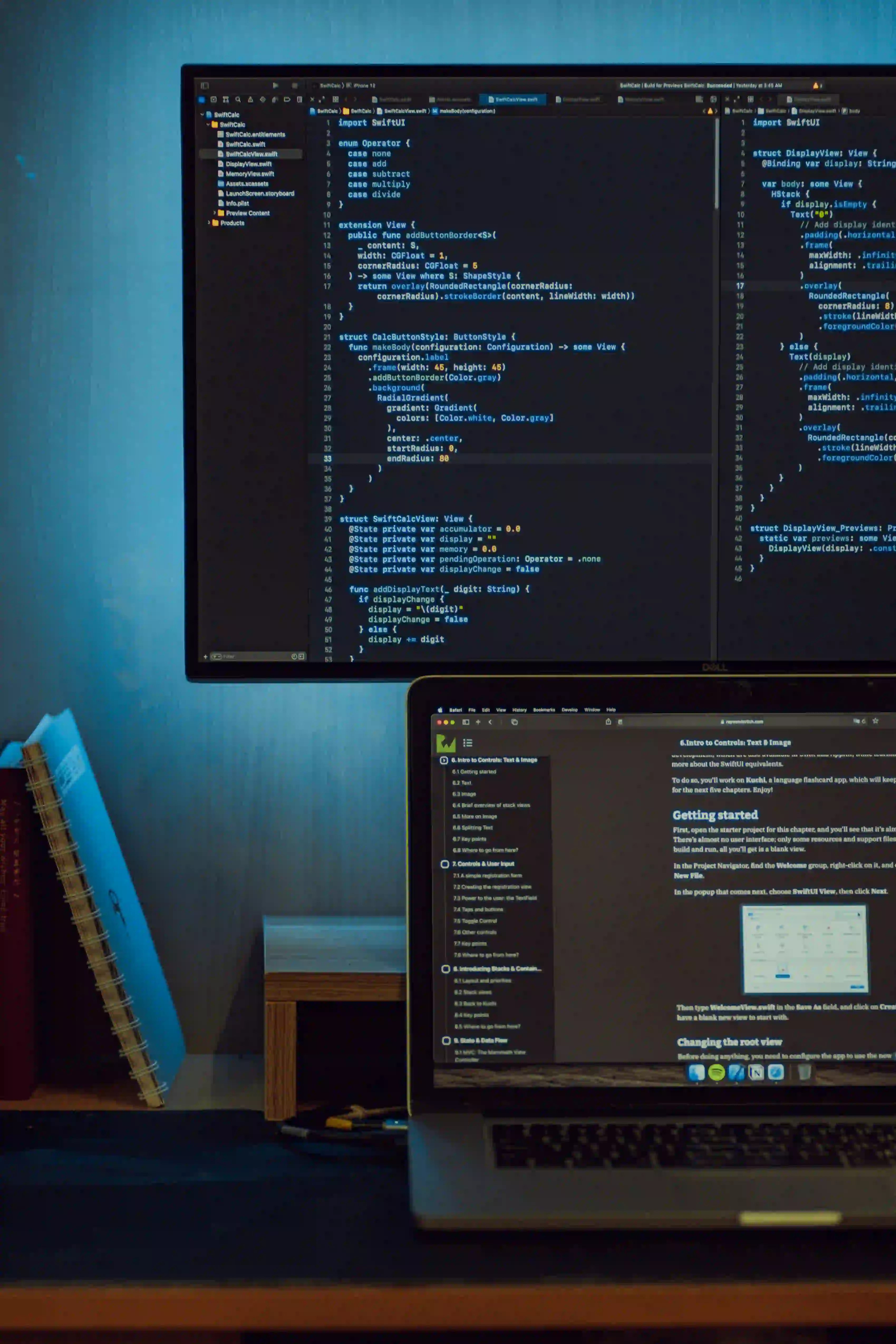
Mastering Redis Streams: Handling Data Loss in Java
In the modern era of data processing and management, Redis has emerged as a powerful tool for handling real-time data with its sophisticated data structures. One such feature, Redis Streams, revolutionizes how we deal with messages and events. However, data loss can be a significant concern when dealing with streams. This post will delve into how to effectively manage Redis Streams in Java while mitigating the risks of data loss.
What Are Redis Streams?
Redis Streams is a data structure introduced in Redis 5.0. It provides an efficient way to manage collections of messages in an ordered, time-series fashion. Streams allow data producers to append messages and consumers to read them in a manner that ensures reliable delivery.
Key Characteristics of Redis Streams
- Ordered: Messages are stored in an ordered manner.
- Time-based: Each message has a unique ID based on its insertion time.
- Consumer Groups: Multiple consumers can read the same stream without processing the same messages again.
For detailed insights on Redis Streams, refer to the Redis official documentation.
Why Should You Care About Data Loss?
Data loss is not just a technical hiccup; it can have severe implications for businesses. Logically, each message can represent critical data points such as transactions, notifications, or user events. Losing these could mean losing crucial insights or business opportunities.
When working with Redis Streams, a common challenge is ensuring that data is not lost due to network issues, application crashes, or logical flaws. Hence, understanding how to minimize these risks is pivotal.
Setting Up Redis and Java
You need a working instance of Redis to start using Redis Streams with Java. Redis can be installed via Docker for easy setup. Use the following command to get started:
docker run -d --name redis-streams -p 6379:6379 redis:latest
Next, make sure you have the required Java dependencies. Include the following in your pom.xml
if using Maven:
<dependency>
<groupId>org.redisson</groupId>
<artifactId>redisson-spring-boot-starter</artifactId>
<version>3.16.3</version>
</dependency>
If you're using Gradle, add this to your build.gradle
:
implementation 'org.redisson:redisson-spring-boot-starter:3.16.3'
Basic Operations with Redis Streams
Before diving into data loss prevention, let's look at basic stream operations in Java.
Initializing a Stream
Creating a new stream can be done using the following code:
import org.redisson.Redisson;
import org.redisson.api.RStream;
import org.redisson.api.RedissonClient;
import org.redisson.config.Config;
// Configure Redisson client
Config config = Config.fromYAML("path/to/redisson-config.yaml");
RedissonClient redisson = Redisson.create(config);
// Initialize the stream
RStream<String, String> stream = redisson.getStream("mystream");
Why?
Initializing the stream is necessary to define where your data will be stored and retrieved. It acts as the endpoint for incoming messages.
Adding Messages to a Stream
Once the stream is created, you can add messages to it using the following method:
String messageId = stream.add("Hello, Redis Streams!");
System.out.println("Message ID: " + messageId);
Why?
Adding messages to a stream allows you to capture events or transactions systematically. Each message is assigned a unique ID, enabling you to track it precisely.
Reading Messages from a Stream
To read messages from a stream, you can implement the consumer logic as follows:
Map<String, String> messages = stream.readGroup("mygroup", "myconsumer");
messages.forEach((id, message) -> {
System.out.println("ID: " + id + " Message: " + message);
});
Why?
This snippet demonstrates how you can retrieve messages while specifying a consumer group. It ensures that multiple consumers can read from the same stream in a distributed way.
Handling Data Loss in Redis Streams
Implement Error Handling
One of the best practices for minimizing data loss is to implement robust error handling. You can utilize try-catch blocks to manage potential exceptions.
try {
String messageId = stream.add("Important Event");
// Acknowledge message after successful processing
} catch (Exception e) {
System.err.println("Failed to add message: " + e.getMessage());
}
Why?
Error handling ensures you catch exceptions that can arise during data operations. Ignoring these errors could lead to silent data loss.
Message Acknowledgments
To ensure processed messages are not read again, you need to acknowledge them:
stream.ack("mygroup", messageId);
Why?
Acknowledgments signify that a message has been correctly processed. If an acknowledgment is not given, the consumer can re-process the message, leading to potential duplication errors.
Reliable Message Processing
Incorporate mechanisms such as "at-least-once" processing to ensure message integrity. This approach may involve retrying message handling and tracking the state of each message.
for (int i = 0; i < MAX_RETRIES; i++) {
try {
// Attempt to process the message
break; // if successful, break
} catch (ProcessingException e) {
System.err.println("Retrying... Attempt: " + i);
}
}
Why?
Retrying failed message processing aims to minimize data loss. However, it's essential to implement a maximum retry limit to avoid indefinite loops.
Persisting Data to Stable Storage
In scenarios where message fidelity is crucial, consider persisting your data to a more stable storage option like a database after processing.
// Assume we have a function saveMessageToDatabase
saveMessageToDatabase(messageId, message);
Why?
Persisting to an external, durable storage mechanism acts as a safety net should the Redis instance fail or become unreachable.
Best Practices
- Use Consumer Groups: This will allow you to manage parallel processing and balance workloads effectively.
- Message ID Tracking: Store a record of processed message IDs in a database to avoid reprocessing.
- Scale Redis: If dealing with a high volume of messages, consider scaling your Redis installation to handle the load efficiently.
- Monitor Performance: Keep an eye on your Redis performance metrics to identify potential issues before they lead to data loss.
Closing Remarks
Mastering Redis Streams in Java is a journey that pays off significantly when implemented with data integrity in mind. By understanding how to handle potential data loss, applying robust processing patterns, and utilizing Redis's features to their fullest, you contribute to building resilient applications.
With the right practices in place, Redis Streams can serve as both a powerful messaging system and a safe reservoir of valuable data. Take the time to get acquainted with Redis Streams, and you'll find it to be an invaluable asset in your software engineering arsenal.
For more information on Redis and its features, check out these useful resources:
Happy coding!