Solving Connectivity Issues with Android Things Nearby
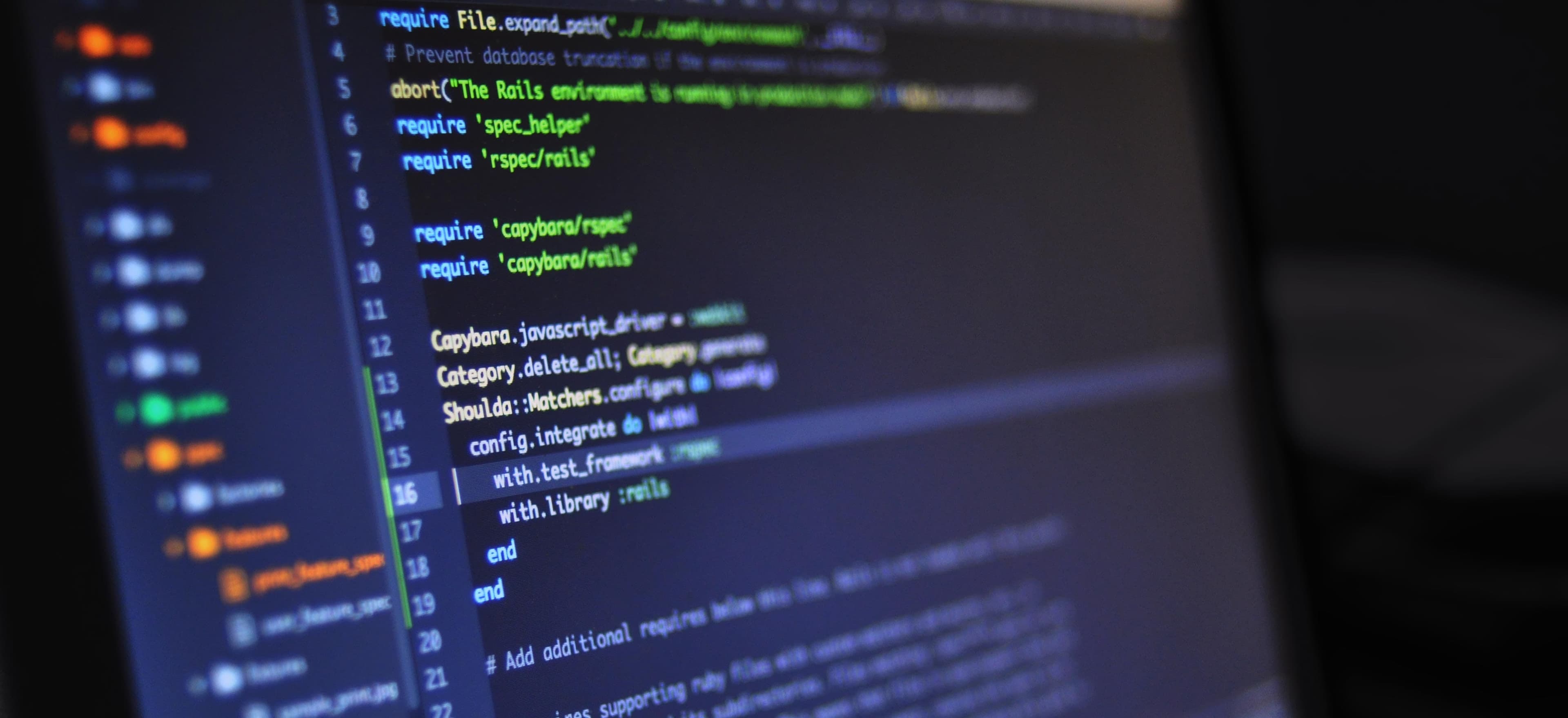
- Published on
Solving Connectivity Issues with Android Things Nearby
In today's increasingly connected world, creating seamless experiences between devices is more crucial than ever. With Android Things, Google aimed to simplify the development of Internet of Things (IoT) applications. One of the features that stand out in this ecosystem is the Nearby Connections API. If you're finding connectivity issues when using this API, you're not alone. It's a common challenge for developers dealing with low-energy Bluetooth connections, Wi-Fi direct, and other forms of data exchange.
What is Android Things and Nearby Connections API?
Android Things is a version of Android designed specifically for IoT devices. It allows developers to build and manage smart devices efficiently, often remotely. The Nearby Connections API is a crucial subset of Android Things, facilitating easy discovery and communication between devices in close proximity. This is significant for developers creating applications that require interaction not only between local device clusters but also across varying platforms.
The Importance of Connectivity
Before diving into resolving connectivity issues, let's explore why robust connectivity matters. In the context of IoT:
- User Experience: Users expect devices to connect seamlessly and quickly without complicated setup processes.
- Data Accuracy: Consistent connections facilitate real-time data acquisition and sharing, boosting operational efficiency.
- Interoperability: Devices often need to communicate with varying platforms, making strong, reliable connectivity a must.
With these factors in mind, let's tackle common challenges and how to resolve them.
Common Connectivity Issues with Nearby API
- Device Discovery Failing
- Data Transfer Slowdown
- Connection Timeouts
- Inconsistent Device Behavior
Each of these issues can arise from several underlying problems—poor configurations, Bluetooth interference, or even incorrect implementations. Let’s dive deeper into each issue and explore solutions.
1. Device Discovery Failing
Problem: One of the most common problems developers face is that devices may not detect each other during discovery.
Solution: Ensure the proper permissions are set and that your application is registered correctly.
Example Code Snippet
public class NearbyConnection {
private ConnectionsClient connectionsClient;
public NearbyConnection(Context context) {
connectionsClient = Nearby.getConnectionsClient(context);
}
public void startDiscovery(String serviceId) {
connectionsClient.startDiscovery(
serviceId,
endpointDiscoveryCallback,
new DiscoveryOptions.Builder().setStrategy(Strategy.P2P_CLUSTER).build());
}
}
Why This Works: The startDiscovery
method, combined with appropriate strategies, like P2P_CLUSTER
, allows for better scope in discovering devices. Always ensure you check for relevant permissions, especially for Bluetooth functionalities in your manifest.
2. Data Transfer Slowdown
Problem: Sometimes, when devices seem to connect, the data transfer is sluggish.
Solution:
- Optimize data payloads.
- Use the right strategies.
Example Code Snippet
public void sendData(String endpointId, byte[] payload) {
connectionsClient.sendPayload(endpointId, Payload.fromBytes(payload));
}
Why This Works: Sending smaller payloads can lead to improvements in transfer speed. While Payload.fromBytes
can handle large arrays, segmenting bigger payloads into smaller bits often resolves throughput problems.
3. Connection Timeouts
Problem: Another issue many developers face is connection timeouts, which can be frustrating to debug.
Solution: Increase timeout settings where applicable and provide user feedback.
Example Code Snippet
connectionsClient.requestConnection(
endpointId,
localEndpointName,
connectionLifecycleCallback,
new ConnectionOptions.Builder()
.setTimeoutMillis(10000) // Increased timeout
.setStrategy(Strategy.P2P_POINT_TO_POINT)
.build()
);
Why This Works: The connection timeout can be tweaked to allow for more extended periods of trying to connect. In environments with connectivity challenges, this small adjustment can often make a huge difference.
4. Inconsistent Device Behavior
Problem: Devices may behave inconsistently owing to diverse factors such as device capabilities, power management, and environmental factors.
Solution: Monitor device states and adjust dynamically.
Example Code Snippet
// Lifecycle Callbacks
private final ConnectionLifecycleCallback connectionLifecycleCallback = new ConnectionLifecycleCallback() {
@Override
public void onConnectionInitiated(String endpointId, ConnectionInfo connectionInfo) {
// Auto-accept connection
connectionsClient.acceptConnection(endpointId, payloadCallback);
}
@Override
public void onConnectionResult(String endpointId, ConnectionResolution connectionResolution) {
if (connectionResolution.getStatus().isSuccess()) {
// Handle successful connection state
}
}
@Override
public void onDisconnected(String endpointId) {
// Re-attempt connections or notify user
}
};
Why This Works: Using lifecycle callbacks helps manage the state of each connection actively. You can programmatically verify the success or failure of connections, allowing your app to respond appropriately.
Best Practices to Minimize Connectivity Issues
- Assess Environment: Be conscious of surrounding devices and networks that may interfere.
- Robust Error Handling: Implement comprehensive error-handling strategies to address connectivity problems dynamically.
- User Feedback: Always provide visual feedback to users during discovery or transfers, enhancing the experience.
- Frequent Testing: Regularly test your application in various conditions and on different devices, focusing on connectivity.
In Conclusion, Here is What Matters
Connectivity issues with Android Things' Nearby Connections API can be a significant hurdle, but understanding how to tackle them can lead to smoother user experiences and enhanced application performance. By applying the methods and best practices discussed in this post, you can prepare to create robust IoT applications that function seamlessly in interconnected environments.
For more in-depth explorations of Android Things and IoT applications, be sure to check the official documentation here for updates and additional resources.
As always, developing a keen UX awareness and rigorous testing practices will ensure your applications transcend connectivity challenges, propelling you into a new era of smart, connected living. Happy coding!