Why Overusing Synchronization Can Slow Down Your Code
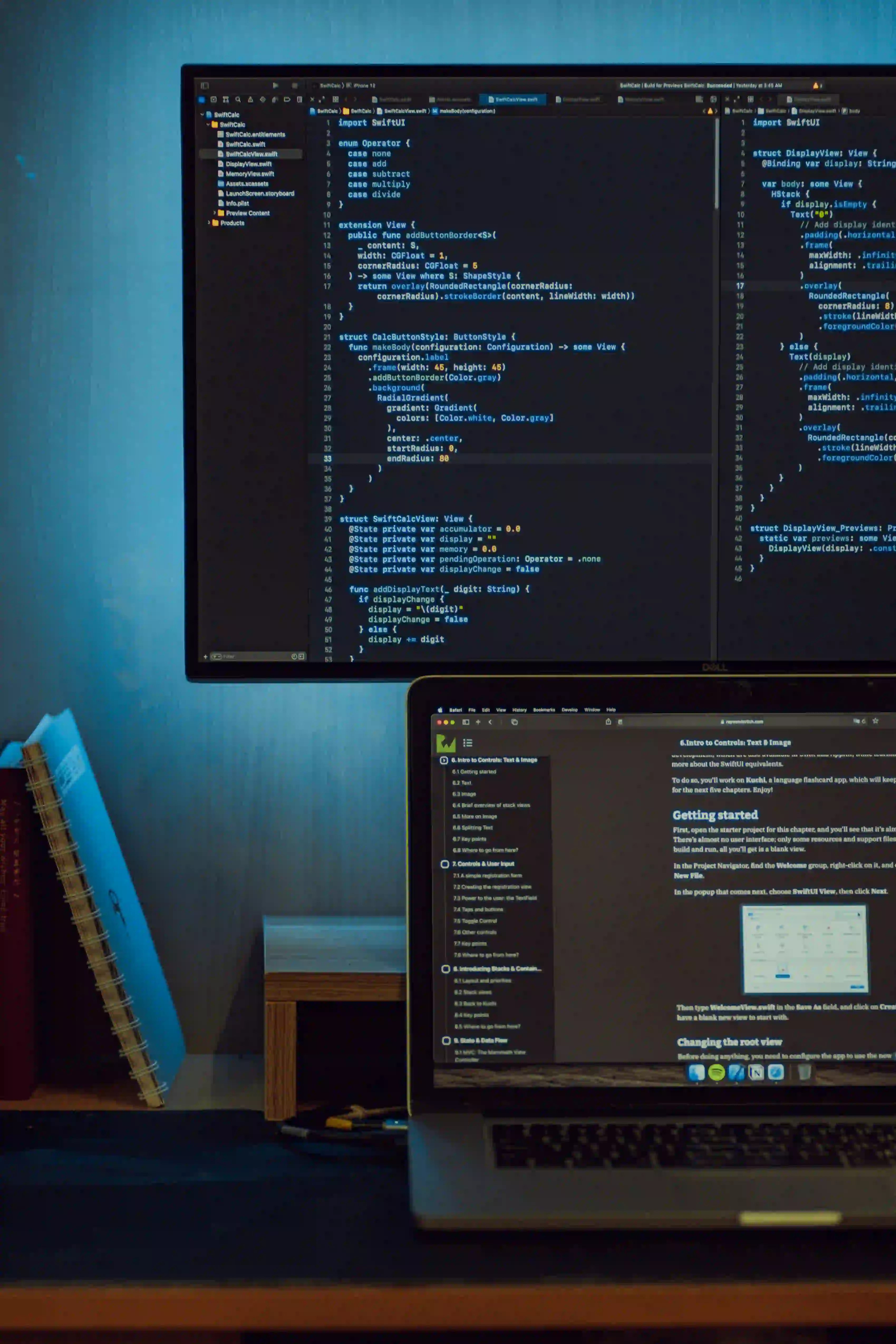
Why Overusing Synchronization Can Slow Down Your Code
In the era of multi-core processors, concurrent programming has become a necessity. With the ability to run multiple threads simultaneously, Java developers can create applications that are faster, more responsive, and more efficient. However, with the rise of thread management comes the challenge of ensuring that shared resources are handled correctly. This is where synchronization comes into play.
While synchronization is vital for maintaining data integrity in concurrent environments, overusing it can severely degrade performance. In this blog post, we will explore why overusing synchronization can slow down your Java code and provide best practices to strike the right balance.
Understanding Synchronization
Synchronization in Java is a mechanism that restricts access to shared resources by multiple threads. It prevents a scenario known as a race condition, where two threads attempt to modify a shared variable at the same time, leading to unpredictable results.
How It Works
In Java, you can synchronize methods or blocks of code using the synchronized
keyword. Here's a basic example:
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
In the above code:
- The
increment
method is synchronized, meaning only one thread can execute it at a time. - This approach ensures that the value of
count
is updated safely.
The Cost of Synchronization
The simplicity that synchronization provides can also be its downfall. When a thread acquires a lock to enter a synchronized block, other threads that are trying to acquire the same lock must wait. This waiting introduces latency and can cause performance bottlenecks.
Context Switching
When threads are blocked waiting for access to a synchronized method, the underlying operating system may need to perform context switching. Context switching is the process where the CPU switches from one process or thread to another, saving the state of the current thread and loading the state of the next thread. This procedure is expensive and time-consuming.
Example of Bottleneck
Consider the following code snippet:
public class PrinterQueue {
public synchronized void printJob(String document) {
System.out.println("Printing: " + document);
// Simulating a job that takes time
try {
Thread.sleep(2000); // Sleep for two seconds
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
If multiple threads attempt to call printJob()
, they will be forced to wait for one another to finish. This is inefficient and can introduce significant delays in applications that require rapid queued processing.
Impact on Throughput
Throughput, the number of tasks completed in a given time frame, is negatively affected by overusing synchronization. As threads wait for locks, fewer tasks can be processed, reducing the overall throughput of your application.
According to a Java performance tuning guide, the objective is to reduce the time spent in synchronized blocks while keeping data integrity intact.
Best Practices to Minimize Synchronization Overhead
1. Favor the Use of Concurrency Utilities
Java provides built-in high-level concurrency utilities in the java.util.concurrent
package. These utilities, like ConcurrentHashMap
and CountDownLatch
, are designed to reduce the need for explicit synchronized blocks:
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentCache {
private ConcurrentHashMap<String, String> cache = new ConcurrentHashMap<>();
public void addItem(String key, String value) {
cache.put(key, value);
}
public String getItem(String key) {
return cache.get(key);
}
}
This approach allows concurrent read and write access without the overhead of locking around each method.
2. Limit the Scope of Synchronization
Instead of synchronizing entire methods, you can localize synchronization to only the parts of the code that need it, using synchronized blocks:
public class SharedResource {
private final Object lock = new Object();
private int sharedVariable;
public void safeIncrement() {
synchronized (lock) {
sharedVariable++;
}
}
}
This not only reduces the time spent in a synchronized state but also minimizes contention.
3. Use Immutable Objects
Immutable objects are inherently thread-safe as they can’t be modified after they are created. They eliminate the need for synchronization altogether.
public final class ImmutablePoint {
private final int x;
private final int y;
public ImmutablePoint(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
As a best practice, prefer to return immutable objects from methods, reducing the risk of unintended side effects.
4. Track Performance
Often overlooked, tracking the performance of synchronized sections of code can provide insights into bottlenecks. Tools like Java VisualVM can help you analyze thread states and identify excessive blocking issues.
The Last Word
In multi-threaded programming, it is crucial to understand the trade-offs between synchronization and performance. While ensuring data integrity is essential, overusing synchronization can lead to poor performance, bottlenecks, and high latency.
By adopting high-level concurrency utilities, limiting the scope of synchronization, using immutable objects, and monitoring performance, Java developers can create efficient applications that maintain both speed and reliability.
In summary, strive to use synchronization judiciously. Only apply it where absolutely necessary, and always look for alternative designs that minimize its usage. For more on Java concurrency and synchronization, consider reading the Java Concurrency in Practice.
Implement these practices, and you will find a noticeable improvement in your application's performance. Happy coding!