Common Pitfalls in Java URL Validation Techniques
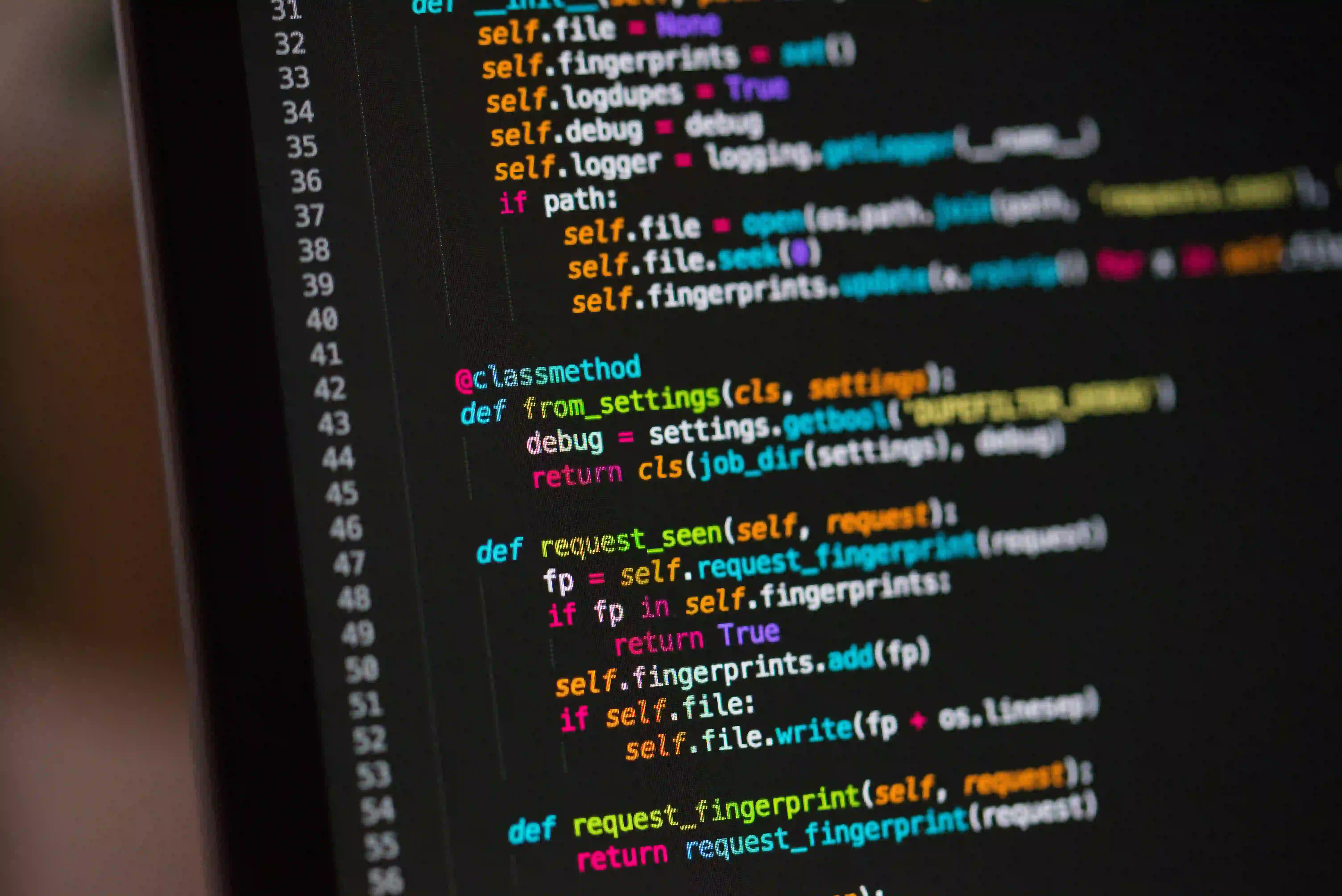
Common Pitfalls in Java URL Validation Techniques
Validating URLs is an essential practice in web development. Whether you're dealing with user input, API requests, or configuration settings, ensuring that URLs are correct is critical for your application's functionality and security. In this blog post, we will explore common pitfalls in Java URL validation techniques and provide effective strategies to avoid them.
Why Validate URLs?
Before we dive into the pitfalls, it is important to understand the reasons for URL validation:
- Security: Malicious URLs can lead to security vulnerabilities, such as XSS attacks.
- User Experience: Proper validation can prevent users from submitting incorrect URLs.
- Data Integrity: Avoid incorrect or broken URLs that may cause runtime exceptions or API failures.
The Basics of URL Validation in Java
Java provides various classes and methods to facilitate URL validation. For instance, the java.net.URL
class can help construct and validate URLs.
Here's a simple example:
import java.net.MalformedURLException;
import java.net.URL;
public class URLValidator {
public boolean isValidURL(String urlString) {
try {
URL url = new URL(urlString);
url.toURI(); // Check if it's a valid URI
return true; // URL is valid
} catch (MalformedURLException | IllegalArgumentException e) {
return false; // URL is invalid
}
}
}
In this code snippet, we attempt to create a URL from a string. If the string is not valid, a MalformedURLException
will be thrown, and we catch it to return false.
Common Pitfalls
While the above method seems straightforward, there are several pitfalls that developers encounter when validating URLs in Java. Let's take a closer look at these:
1. Ignoring the "http" or "https" Scheme
One common mistake is not enforcing the HTTP or HTTPS scheme. URLs without these schemes are technically not valid and can lead to security issues.
public boolean isValidHTTPUrl(String urlString) {
if (!urlString.startsWith("http://") && !urlString.startsWith("https://")) {
return false; // Not secure
}
return isValidURL(urlString);
}
In this enhanced method, we ensure the URL begins with either HTTP or HTTPS.
2. Overlooking IPv6 Address Support
As the internet evolves, IPv6 addresses have become more common. Java's URL
class does support these addresses, but it’s crucial to handle them properly.
public boolean isValidIPv6Url(String urlString) {
if (urlString.startsWith("http://") || urlString.startsWith("https://[")) {
// Check if the URL is an IPv6 address
try {
new URI(urlString);
return true; // Valid IPv6 URL
} catch (URISyntaxException e) {
return false; // Invalid IPv6 URL
}
}
return isValidURL(urlString);
}
In this case, we ensure the proper handling of IPv6 by explicitly checking for the correct syntax.
3. Not Handling Malicious Input
Another critical pitfall is failing to sanitize user input. Even if a string appears to be a valid URL, it could still be crafted to cause harm, such as redirecting users to malicious sites.
Always sanitize input before using it in any context, and consider using libraries like OWASP's [ESAPI for safer data handling.
4. Overreliance on Regular Expressions
Regular expressions may seem like an attractive solution for URL validation. However, they can easily become complicated and fail to capture all valid cases or incorrectly validate certain patterns.
Here's a simple regex that validates URLs, but use it cautiously:
public boolean isValidWithRegex(String urlString) {
String regex = "^(http://|https://|ftp://)?(www\\.)?[a-zA-Z0-9-]+\\.[a-zA-Z]{2,}(/.*)?$";
return urlString.matches(regex);
}
While regex can work, it often struggles with edge cases. For validation, rely on the in-built mechanisms offered by Java, like URL
and URI
.
5. Failing to Handle Redirects
Some URLs may appear valid but redirect to different domains. It’s important to handle these cases, especially if the user expects to be taken directly to a specific page.
You can use the HttpURLConnection
class to check for redirects:
import java.net.HttpURLConnection;
import java.net.URL;
public boolean isRedirectingUrl(String urlString) {
try {
HttpURLConnection connection = (HttpURLConnection) new URL(urlString).openConnection();
connection.setInstanceFollowRedirects(false);
connection.connect();
int responseCode = connection.getResponseCode();
return responseCode >= 300 && responseCode < 400; // Check if it’s a redirect
} catch (IOException e) {
return false;
}
}
6. Neglecting Port Numbers
URL validation must also consider port numbers, which can be present in URLs. For instance, http://example.com:8080
is a valid URL.
Always account for port numbers when validating:
public boolean isValidPortedUrl(String urlString) {
try {
URL url = new URL(urlString);
int port = url.getPort();
return (port >= 1 && port <= 65535); // Valid port range
} catch (MalformedURLException e) {
return false;
}
}
Wrapping Up
Validating URLs in Java requires careful consideration of various factors. By being aware of common pitfalls, such as neglecting schemes, overlooking IPv6 support, failing to sanitize input, overreliance on regex, ignoring redirects, and not accounting for ports, developers can create robust validation mechanisms.
If you want to delve deeper into URL validation and security practices, consider exploring the OWASP Top Ten security vulnerabilities and guidelines. Always prioritize security and user experience in your applications.
By keeping these strategies in mind, you can improve your URL validation techniques and enhance the overall quality and security of your Java applications. If you would like to discuss more about URL validation techniques or share your experiences, feel free to leave a comment below!