Common Pitfalls in Android Main Activity Setup
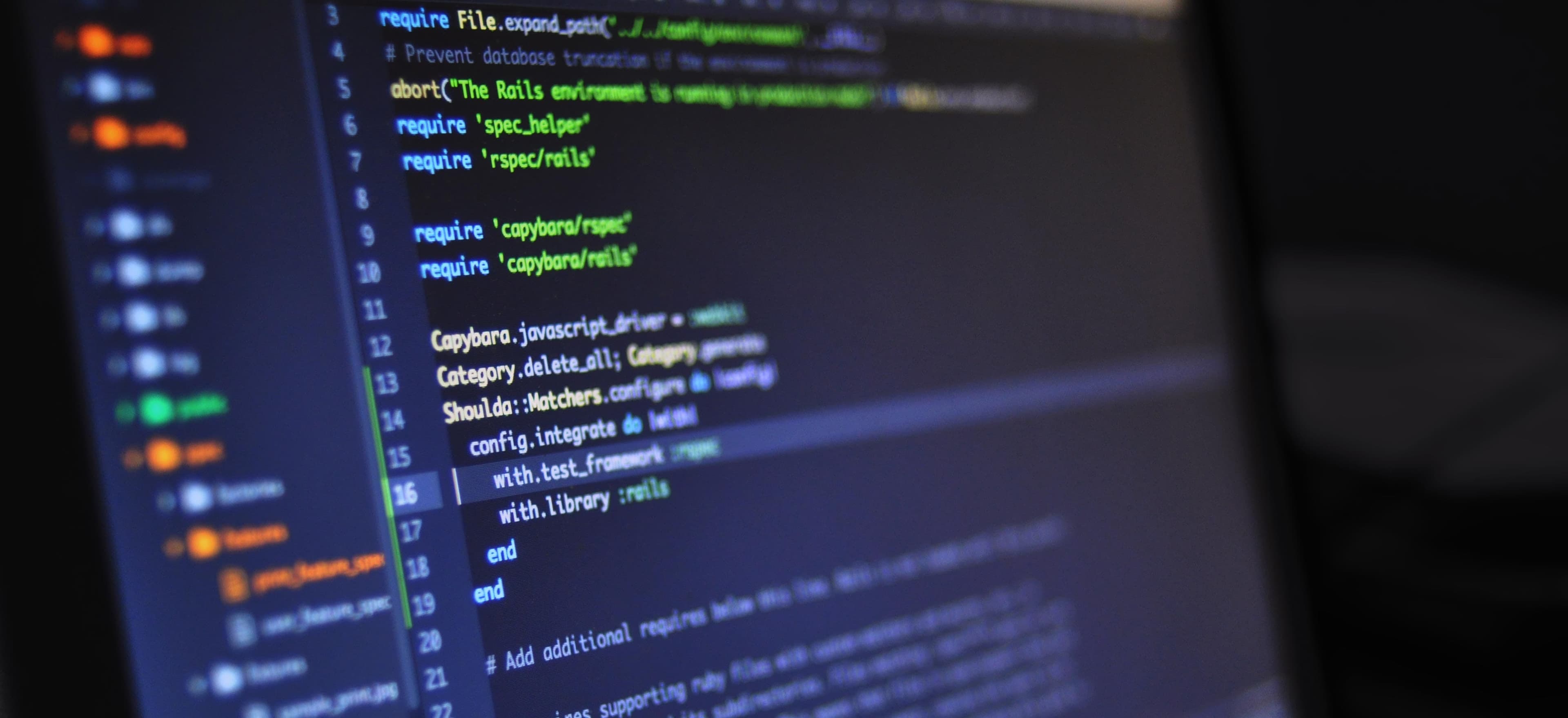
- Published on
Common Pitfalls in Android Main Activity Setup
When developing Android applications, the main activity serves as the entry point for users. Mistakes made in setting it up can lead to poor user experiences, crashes, or unexpected behavior. In this blog post, we'll explore common pitfalls that developers encounter when establishing the main activity in an Android app, provide sample code snippets for clarity, and highlight the 'why' behind the best practices to follow.
Table of Contents
- Understanding the Main Activity
- Common Pitfalls
- Incorrect Manifest Configuration
- Layout Issues
- Lifecycle Mismanagement
- Resource Management
- Intents and Navigation
- Best Practices
- Conclusion
Understanding the Main Activity
The main activity is crucial because it initializes the user interface and starts the application's flow. It involves configuring both the AndroidManifest.xml file and the activity class itself. Getting these right enhances user experience and minimizes issues.
Common Pitfalls
1. Incorrect Manifest Configuration
One of the first hurdles is improperly declaring the main activity in the AndroidManifest.xml
.
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
Why?
The intent-filter
marks the activity as the launch point of the app. Missing this declaration can lead to the app crashing on startup, as Android will not know what activity to present first.
2. Layout Issues
Another common pitfall is not properly referencing the layout file in the main activity:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main); // Ensure this layout exists
}
}
Why?
If the layout file mentioned in setContentView
does not exist or is misnamed, the app will throw a Resources.NotFoundException
. It's crucial to double-check the resource IDs.
3. Lifecycle Mismanagement
Misunderstanding the lifecycle methods of an Android activity can lead to unexpected behavior. Let's look at the activity lifecycle:
@Override
protected void onStart() {
super.onStart();
// Code to execute when the activity becomes visible
}
@Override
protected void onPause() {
super.onPause();
// Code to execute when the user leaves the activity
}
Why? Failing to override lifecycle methods properly can lead to resource leaks, data loss, or unexpected UI states. Understanding when each method is called helps manage resource states and user interaction more effectively.
4. Resource Management
Within the main activity, proper management of resources is vital; this includes handling views and listeners.
Button myButton = findViewById(R.id.my_button);
myButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// Handle button click
}
});
Why? Not managing resources can lead to memory leaks. Always unregister listeners or avoid static references to the activity in inner classes that can outlive the activity lifecycle.
5. Intents and Navigation
Navigation is a key aspect. Failing to pass data correctly through intents can lead to null pointer exceptions or incorrect input.
Intent intent = new Intent(this, NextActivity.class);
intent.putExtra("key", value);
startActivity(intent);
Why?
This data needs to be retrieved in the NextActivity
. A missing or mismatched key can lead to null values or application crashes. When passing data between activities, ensure that you are maintaining a consistent key naming scheme.
Best Practices
To avoid the common pitfalls, follow these best practices:
-
Manifest Verification: Always double-check your AndroidManifest.xml to ensure that your main activity is properly configured with the correct filters.
-
Consistent Naming: Use meaningful resource names and adhere to consistent naming conventions for layouts and identifiers. It avoids confusion and aids maintainability.
-
Lifecycle Awareness: Familiarize yourself with the Android activity lifecycle. Always override lifecycle methods where necessary and ensure resources are appropriately managed.
-
Memory Management: Take care of your views, listeners, and other resources. Use
WeakReference
where necessary to prevent memory leaks. -
Thorough Testing: Implement rigorous testing for your activity to catch potential issues early. Use tools such as Android Profiler to monitor memory usage.
Final Considerations
The main activity of an Android application plays a pivotal role in the user experience. By steering clear of common pitfalls like improper manifest configuration, layout issues, lifecycle mismanagement, resource mismanagement, and poor intent handling, developers can create robust applications that perform well.
By following best practices and being cautious, you can ensure a smooth experience for users right from the first launch. For further reading on best practices, consider checking the Android Developer Documentation.
By understanding these common pitfalls and their surrounding best practices, you can drastically improve the stability and usability of your Android applications. Happy coding!