Mastering Dependency Injection in JavaFX with Dagger
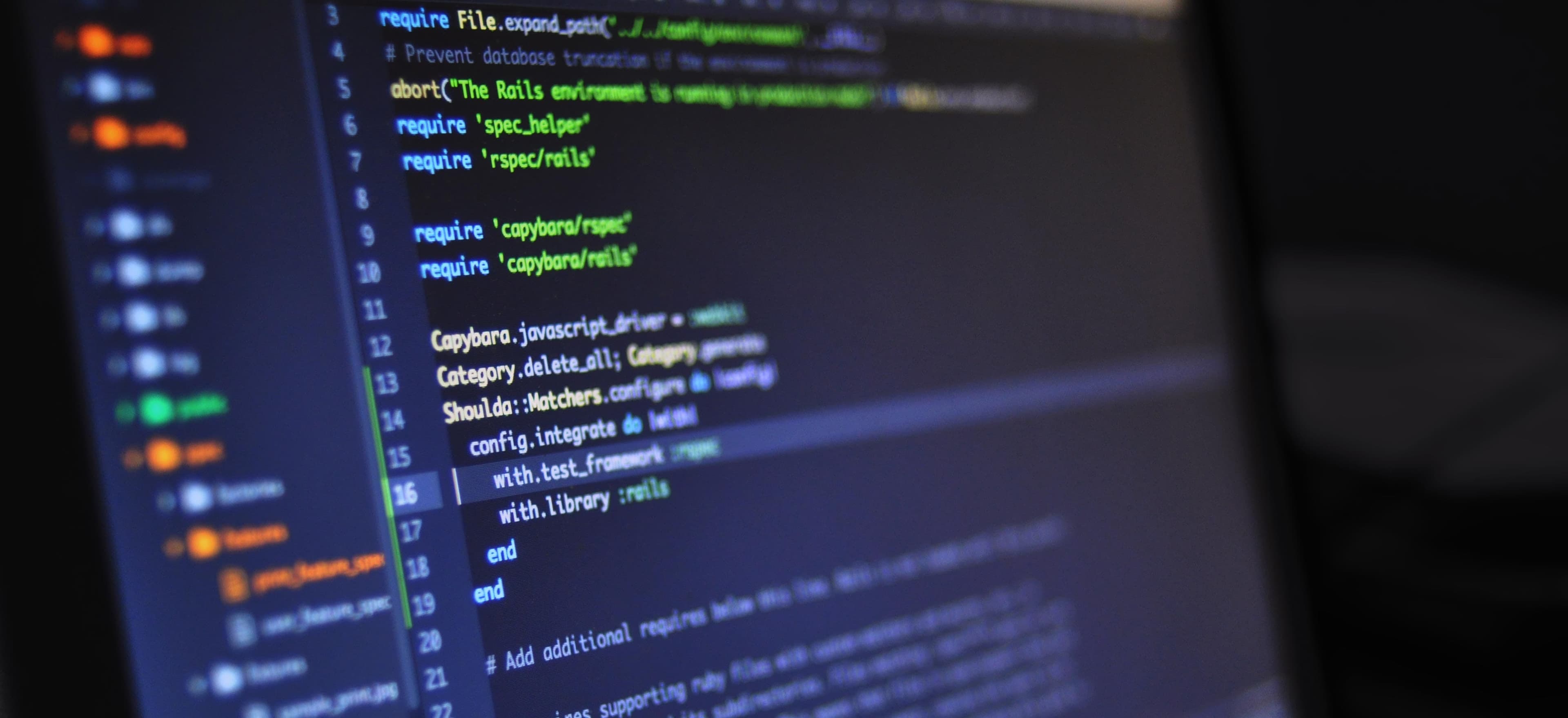
- Published on
Mastering Dependency Injection in JavaFX with Dagger
JavaFX is a wonderful toolkit for creating rich client applications in Java. However, as applications grow in complexity, managing their components becomes challenging. Enter Dependency Injection (DI), a design pattern fundamentally changing how you instantiate and manage your object dependencies. In this blog post, we will delve into mastering Dependency Injection in JavaFX using the Dagger framework.
What is Dependency Injection?
Dependency Injection is a software design pattern that implements Inversion of Control, allowing a class to receive its dependencies rather than creating them internally. This approach promotes loose coupling, easier testing, and enhances code maintainability.
In JavaFX, the DI pattern can help manage complex UI components and business logic, making your application more modular and testable.
Why Use Dagger for Dependency Injection?
Dagger is a popular DI framework for Java and Android applications. Here are a few reasons why you should consider using Dagger in your JavaFX application:
-
Compile-Time Validation: Dagger generates code to ensure that dependencies are provided or injected at compile time, reducing runtime errors.
-
Performance: With Dagger, you can avoid reflection, leading to better performance.
-
Simplicity: Dagger is straightforward to set up and use, with an easy learning curve.
Setting Up Dagger in a JavaFX Application
Before we dive into the code, let’s set up Dagger in our JavaFX application.
Step 1: Add Dagger Dependencies
If you're using Maven, add the following dependencies to your pom.xml
:
<dependency>
<groupId>com.google.dagger</groupId>
<artifactId>dagger</artifactId>
<version>2.40.5</version>
</dependency>
<dependency>
<groupId>com.google.dagger</groupId>
<artifactId>dagger-compiler</artifactId>
<version>2.40.5</version>
<scope>provided</scope>
</dependency>
If you are using Gradle, include these lines in your build.gradle
file:
implementation 'com.google.dagger:dagger:2.40.5'
annotationProcessor 'com.google.dagger:dagger-compiler:2.40.5'
Step 2: Create a Simple JavaFX Application
Here's an initial setup for a JavaFX application that uses a simple controller and service.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class MainApp extends Application {
@Override
public void start(Stage primaryStage) {
Label label = new Label("Hello, Dependency Injection with Dagger!");
StackPane root = new StackPane();
root.getChildren().add(label);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Dagger in JavaFX");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Step 3: Create Service and Controller Classes
Now let’s create a service that simulates business logic.
public class GreetingService {
public String greet() {
return "Welcome to the world of Dependency Injection!";
}
}
Next, we create a controller to handle the UI interactions.
public class GreetingController {
private final GreetingService greetingService;
public GreetingController(GreetingService greetingService) {
this.greetingService = greetingService;
}
public String getGreeting() {
return greetingService.greet();
}
}
Step 4: Set Up Dagger Modules and Components
To let Dagger know how to provide instances of GreetingService
, we will create a module.
import dagger.Module;
import dagger.Provides;
@Module
public class GreetingModule {
@Provides
GreetingService provideGreetingService() {
return new GreetingService();
}
}
Now we need a component interface to connect our module and the classes that require the dependencies.
import dagger.Component;
@Component(modules = GreetingModule.class)
public interface AppComponent {
void inject(MainApp mainApp);
}
Step 5: Integrate Dagger into Your Application
Now that we have everything set up, let’s integrate Dagger into the mainApp
class.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javax.inject.Inject;
public class MainApp extends Application {
@Inject
GreetingController greetingController;
@Override
public void init() throws Exception {
super.init();
AppComponent appComponent = DaggerAppComponent.create();
appComponent.inject(this);
}
@Override
public void start(Stage primaryStage) {
Label label = new Label(greetingController.getGreeting());
StackPane root = new StackPane();
root.getChildren().add(label);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Dagger in JavaFX");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Here’s What’s Happening in the Code:
-
@Inject: This annotation tells Dagger to inject the
GreetingController
dependency into theMainApp
class. -
init() method: This method is overridden to establish the connection between Dagger components and our application.
-
DaggerAppComponent.create(): This line creates an instance of our component, allowing Dagger to wire up the dependencies.
Testing Your Application
Once you've implemented these steps, run your application. You should see a JavaFX window displaying the greeting message:
Welcome to the world of Dependency Injection!
Bringing It All Together
In this blog post, we explored the intricacies of Dependency Injection in JavaFX with Dagger. We discussed the benefits of using DI and went through a hands-on example, building a simple JavaFX application using Dagger to manage dependencies.
Using DI frameworks like Dagger can streamline your JavaFX application's architecture. It promotes cleaner code, easier testing, and better scalability.
For more reading, check out the official Dagger documentation.
By mastering Dependency Injection, you are well on your way to becoming a more proficient JavaFX developer. Happy coding!