Boosting JVM Security with Enhanced Entropy Generation
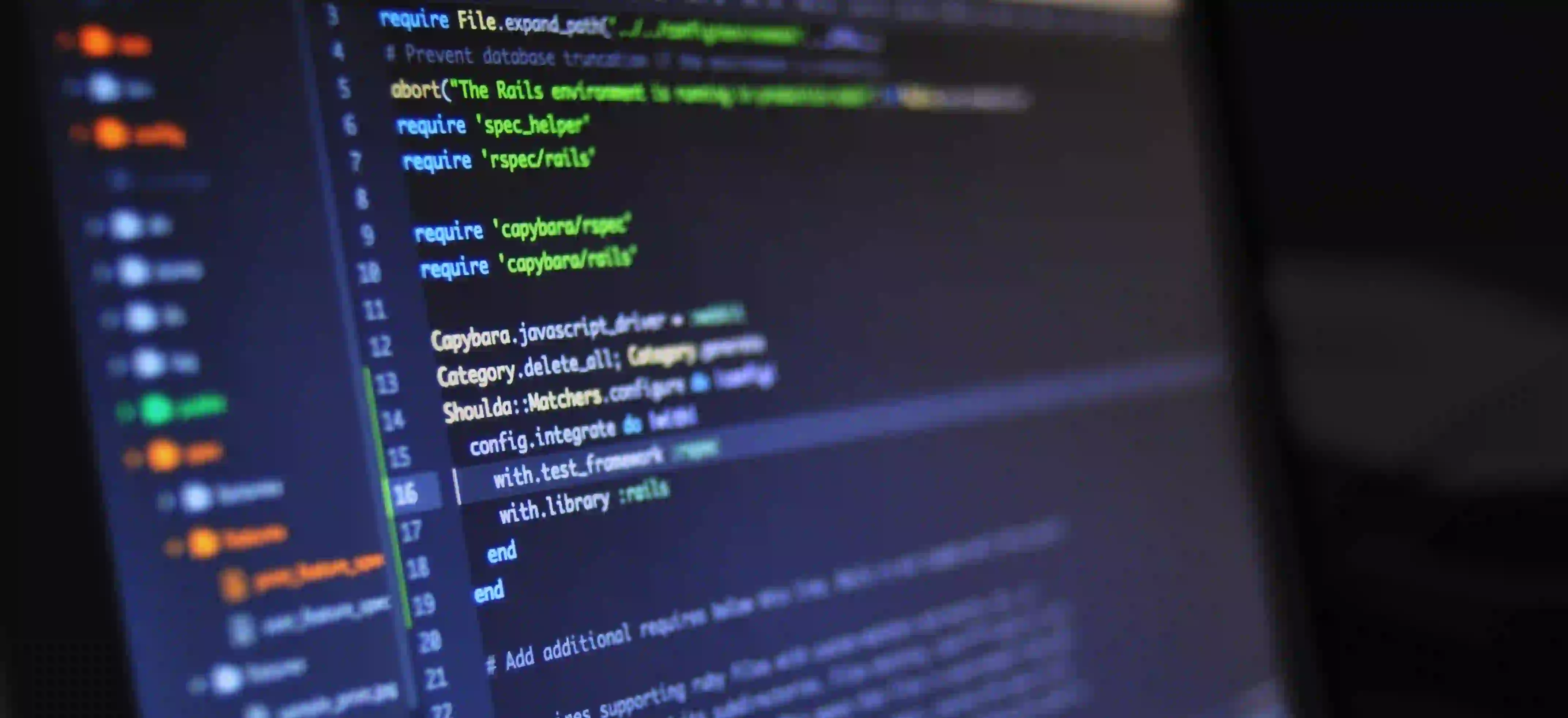
Boosting JVM Security with Enhanced Entropy Generation
In the age of increasing cybersecurity threats, ensuring the security of Java applications running on the Java Virtual Machine (JVM) has never been more vital. One area that's often overlooked is entropy generation. In this blog post, we will dive into the concept of entropy, its significance in JVM security, and how to enhance it for better protection against potential attacks.
Understanding Entropy
What is Entropy?
In the context of computing, entropy is a measure of randomness collected by an operating system or a program. It is critical for cryptographic operations, such as generating secure keys and tokens. The more unpredictable the input, the better the security of cryptographic algorithms, making high entropy essential.
Why is Entropy Important?
Entropy plays a significant role in various areas:
- Key Generation: Cryptographic keys must be unique and unpredictable.
- Session Tokens: Secure generation of tokens prevents attackers from guessing valid sessions.
- Random Numbers: Many security protocols rely on high-quality random numbers to maintain integrity.
Inadequate entropy can lead to predictable keys, making systems susceptible to cyberattacks. As such, Java developers must address entropy issues when building applications.
The State of Entropy Generation in the JVM
The Java platform provides several built-in mechanisms for random number generation. The most common is java.util.Random
and java.security.SecureRandom
.
import java.security.SecureRandom;
public class EntropyExample {
public static void main(String[] args) {
SecureRandom secureRandom = new SecureRandom();
byte[] randomBytes = new byte[16]; // Generate 16 random bytes for secure use
secureRandom.nextBytes(randomBytes);
System.out.println("Generated random bytes: " + bytesToHex(randomBytes));
}
// Utility to convert bytes to hex representation
public static String bytesToHex(byte[] bytes) {
StringBuilder hexString = new StringBuilder();
for (byte b : bytes) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) hexString.append('0');
hexString.append(hex);
}
return hexString.toString();
}
}
Commentary on the Code
- SecureRandom Initialization: By using
SecureRandom
, you leverage a cryptographically strong random number generator (RNG). This provides a far superior level of randomness compared tojava.util.Random
, which is based on linear congruential generators (LCGs) and can be predictable. - Random Byte Generation: The
nextBytes()
method fills the byte array with random data. These random bytes can be used where high-security random data is essential.
While the built-in classes offer decent randomness, real-world applications often require enhanced entropy sources, primarily to guard against various attacks influenced by entropy weaknesses.
Enhancing Entropy Generation
1. Using Operating System Entropy Sources
One effective way to enhance the entropy pool is to utilize the operating system's randomness sources. Operating systems like Linux offer /dev/random
and /dev/urandom
. Java can read from these files to gather additional entropy.
Here’s how you can implement this:
import java.io.FileInputStream;
import java.io.IOException;
public class OSRandomEntropy {
public static byte[] generateOSRandomBytes(int numBytes) throws IOException {
byte[] randomBytes = new byte[numBytes];
try (FileInputStream fis = new FileInputStream("/dev/urandom")) {
fis.read(randomBytes);
}
return randomBytes;
}
public static void main(String[] args) {
try {
byte[] osRandomBytes = generateOSRandomBytes(16);
System.out.println("Generated OS random bytes: " + bytesToHex(osRandomBytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary on the Code
- FileInputStream Usage: Reading from
/dev/urandom
allows us to tap into the OS's entropy pool. Unlike/dev/random
, which may block until enough entropy is collected,/dev/urandom
is non-blocking, making it more suitable for modern applications. - Cross-Platform Considerations: Be aware that this method is platform-dependent; while it works on UNIX-like systems, a different approach is required for Windows platforms.
2. Mixing Additional Entropy Sources
You can also combine entropy from various sources to further enhance randomness. This includes user-generated events such as mouse movements, keyboard presses, or even environmental randomness obtained through external APIs.
Utilizing a ThreadLocal
store is a powerful way to aggregate entropy:
import java.security.SecureRandom;
public class MixedEntropyGenerator {
private static final ThreadLocal<SecureRandom> secureRandomThreadLocal = ThreadLocal.withInitial(SecureRandom::new);
public static byte[] generateMixedEntropy(int numBytes) {
SecureRandom secureRandom = secureRandomThreadLocal.get();
byte[] randomBytes = new byte[numBytes];
secureRandom.nextBytes(randomBytes);
// Additional entropy can be mixed here
return randomBytes;
}
public static void main(String[] args) {
byte[] mixedEntropyBytes = generateMixedEntropy(16);
System.out.println("Generated mixed entropy bytes: " + bytesToHex(mixedEntropyBytes));
}
}
Commentary on the Code
- ThreadLocal Storage: Maintaining a separate instance of
SecureRandom
per thread avoids contention and improves performance in multithreaded environments. - Flexible Design: By modifying the
generateMixedEntropy()
method, developers can easily integrate additional entropy sources as needed.
The Closing Argument
Boosting JVM security requires a proactive approach to entropy generation. By implementing enhanced entropy strategies, Java developers can greatly improve the security of their cryptographic operations.
- SecureRandom: Always prefer
SecureRandom
overjava.util.Random
. - OS Entropy Sources: Incorporate operating system entropy when possible and mix additional sources to maximize randomness.
- Stay Informed: Regularly update your knowledge and leverage suitable libraries and frameworks that improve security.
For more details on security practices within the Java ecosystem, consider checking out Oracle's Security Documentation or OWASP's Java Security Cheat Sheet.
By adding these layers of entropy, we are not merely coding to create; we are also coding to protect, ensuring a resilient Java application that stands firm against potential threats.