Understanding the Misconceptions of Abstract Classes vs Interfaces
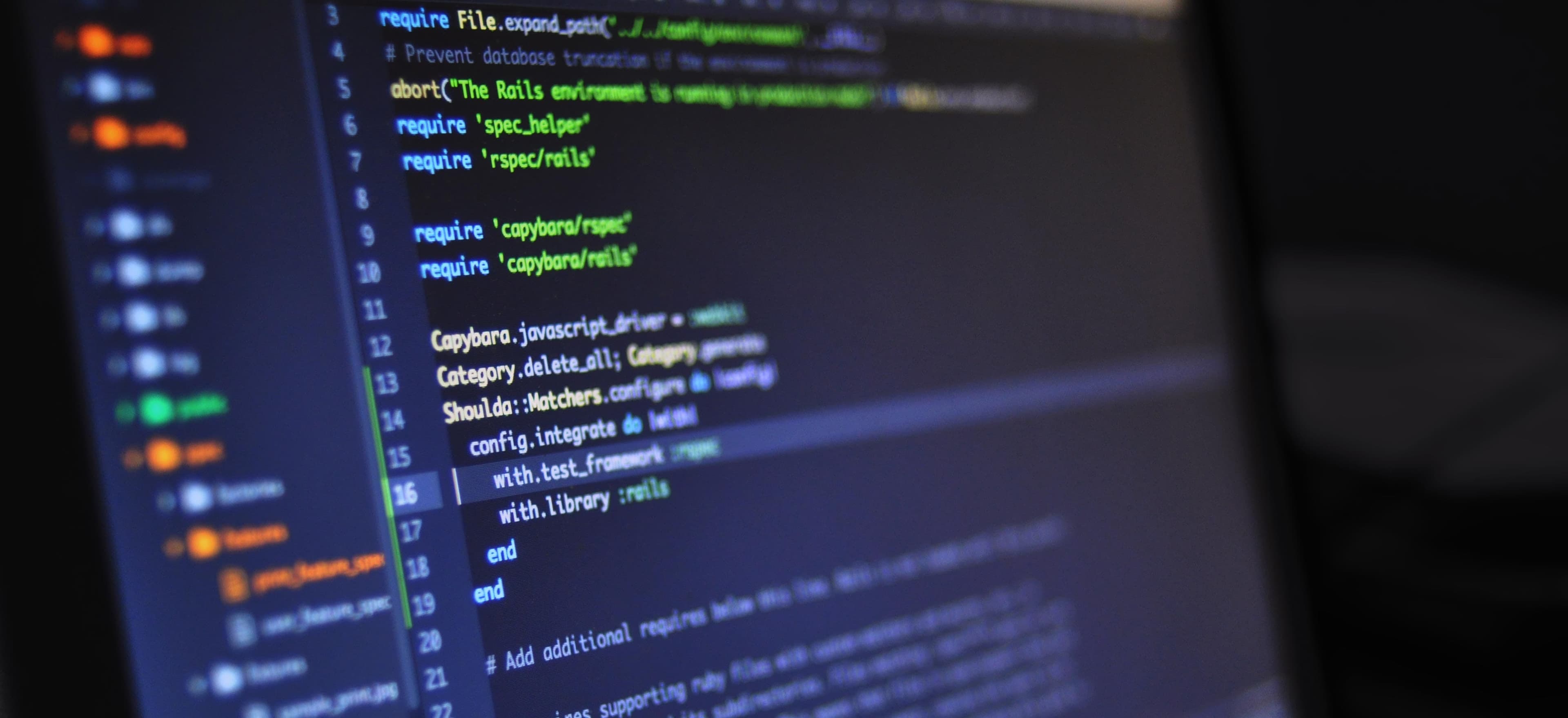
- Published on
Understanding the Misconceptions of Abstract Classes vs Interfaces in Java
Java is a powerful object-oriented programming language that is widely used in various applications. As part of its design, Java offers two important concepts: abstract classes and interfaces. While many developers are familiar with these concepts, misconceptions often arise regarding their differences, use cases, and implementation. In this blog post, we will clarify these misconceptions and highlight when to use each structure effectively.
What is an Abstract Class?
An abstract class in Java is a class that cannot be instantiated on its own. Instead, it serves as a blueprint for other classes. Abstract classes can contain both abstract methods (methods without implementation) and concrete methods (methods with implementation). This versatility allows an abstract class to provide some common functionality while enforcing specific behaviors in its subclasses.
Example of an Abstract Class
Here is an example to illustrate an abstract class:
abstract class Animal {
String name;
Animal(String name) {
this.name = name;
}
abstract void sound(); // Abstract method
void info() {
System.out.println("This is " + name);
}
}
Why Use an Abstract Class?
-
Shared Code: If there are methods that should be shared across multiple subclasses, an abstract class allows you to define those methods once, promoting code reuse.
-
Partial Implementation: You can provide some default behavior through concrete methods, which can be overridden by subclasses if necessary.
-
Control Over Subclass: Abstract classes can control how subclasses are implemented, enforcing certain methods to be present in all subclasses.
What is an Interface?
An interface in Java, on the other hand, is a contract that classes must adhere to. It can only contain abstract methods (although since Java 8, it can also contain default and static methods). Interfaces cannot hold any state (variables) other than constants. Classes that implement an interface must provide implementations for all its abstract methods, unless they are abstract themselves.
Example of an Interface
Consider the following example:
interface Pet {
void play();
void feed();
}
Why Use an Interface?
-
Multiple Inheritance: A class can implement multiple interfaces, which allows for a form of multiple inheritance.
-
Loose Coupling: Interfaces allow for flexibility and loose coupling. Classes can depend upon interfaces rather than concrete implementations, enhancing maintainability and testing.
-
API Evolution: Interfaces can evolve over time (e.g., adding new default methods) without breaking existing implementations.
Key Differences Between Abstract Classes and Interfaces
To further clarify the distinction, let’s summarize the main differences:
| Feature | Abstract Class | Interface | |-------------------------------|-------------------------------------|------------------------------------| | Instantiation | Cannot be instantiated | Cannot be instantiated | | Methods | Can have both abstract and concrete methods | Only abstract methods (default/statically allowed since Java 8) | | State | Can have instance variables | Cannot have state (only constants) | | Constructors | Can have constructors | Cannot have constructors | | Inheritance | Single inheritance | Multiple inheritance is possible | | Accessibility | Access modifiers can be used | All methods are public by default |
Understanding these differences is crucial for making informed decisions in designing your Java applications.
Common Misconceptions
1. Interfaces are just like abstract classes
This misconception can lead to confusion. While both interfaces and abstract classes define a contract for subclasses, the way they are utilized is different. Interfaces focus on defining capabilities, while abstract classes can encapsulate shared code logic.
2. You must choose one over the other
Many developers feel compelled to use abstract classes or interfaces exclusively. In reality, both can be used simultaneously in a project. For instance, a class can implement an interface and also extend an abstract class.
abstract class Bird {
abstract void fly();
}
interface Domesticated {
void feed();
}
class Parrot extends Bird implements Domesticated {
@Override
void fly() {
System.out.println("Parrot is flying.");
}
@Override
public void feed() {
System.out.println("Feeding the parrot.");
}
}
3. Abstract classes are always the better choice
While it may seem tempting to use abstract classes as they can have implemented methods, you lose flexibility due to single inheritance. Interfaces are often the better choice when you want to define a shared behavior across disparate classes.
4. You cannot define behavior in an interface
With the introduction of default methods in Java 8, interfaces can now provide default behavior. This means that interfaces can have methods that offer some level of implementation, breaking the misconception that they can only declare behavior.
interface Appliance {
void turnOn();
default void turnOff() {
System.out.println("Turning off the appliance.");
}
}
Choosing Between Abstract Classes and Interfaces
When to Use Abstract Classes
- When you have a base class that should share some implementation details with its subclasses.
- When you want to provide a common interface that can include some default behavior.
- When you plan to evolve the class hierarchy and require a common base for future extensions.
When to Use Interfaces
- When you want to define capabilities that can be used by any class, regardless of its location in the class hierarchy.
- When you require multiple inheritance of type, allowing a class to implement multiple interfaces.
- When you want to provide a contract that various implementations can follow, enhancing code flexibility.
Lessons Learned
In conclusion, understanding the distinctions and applications of abstract classes and interfaces in Java is essential for effective software design. While both serve unique purposes, they can complement each other in your projects. Proper utilization of these features will lead to cleaner, more maintainable code. To deepen your understanding, consider exploring the Java Tutorials offered by Oracle, which covers these concepts in greater detail.
By clarifying the misconceptions surrounding abstract classes and interfaces, we can better harness their strengths in our Java development endeavors. Happy coding!