Navigating the Balance: Abstraction vs. Detail in Design
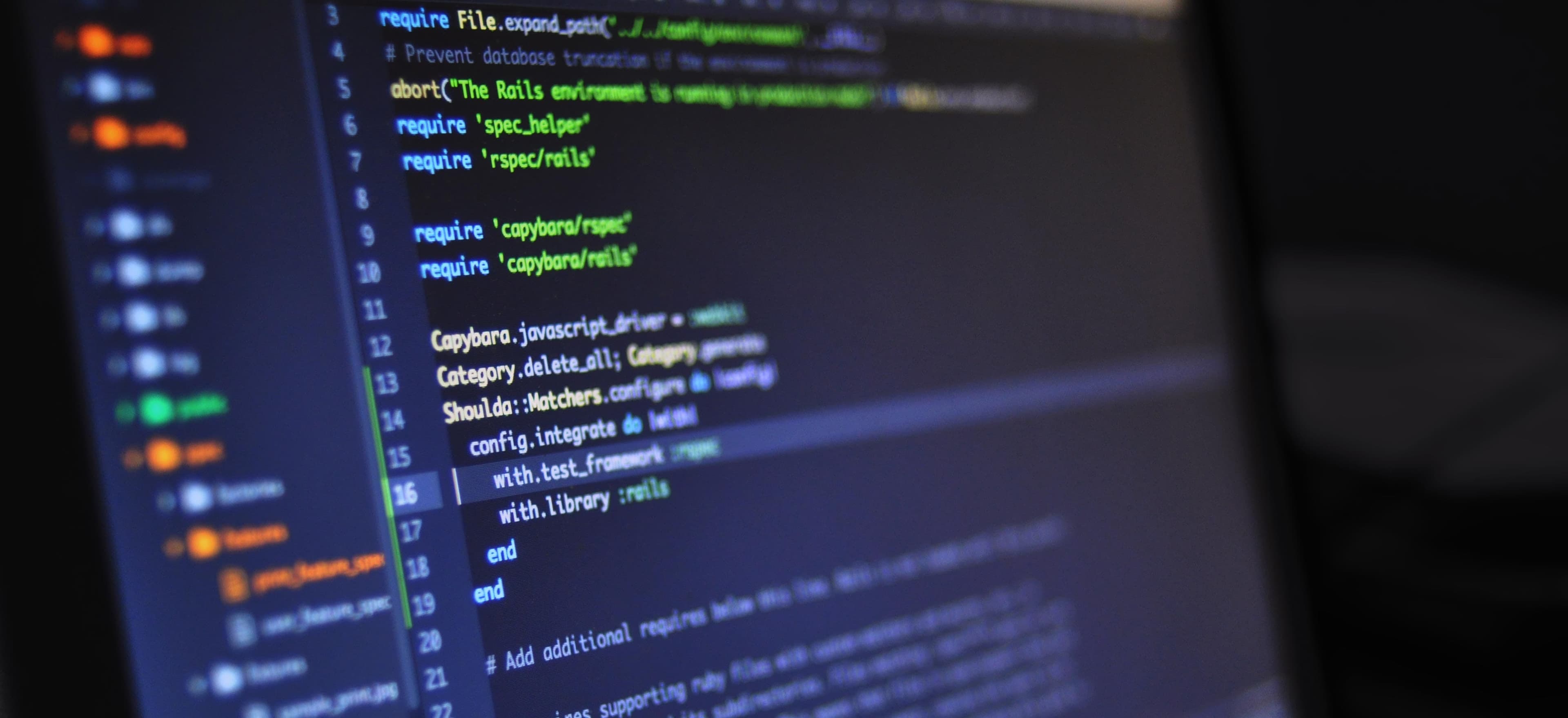
- Published on
Navigating the Balance: Abstraction vs. Detail in Java Design
In software development, particularly using Java, the balance between abstraction and detail can be a decisive factor in creating efficient, maintainable, and scalable systems. It’s essential to understand the importance of both concepts and how they can influence your application's architecture. In this post, we'll explore these ideas, providing insights and examples that highlight best practices in Java design.
Understanding Abstraction and Detail
What is Abstraction?
Abstraction in software design is the practice of simplifying complex systems by breaking them down into manageable parts. This process hides the intricate realities of the system while exposing only the necessary features to the users. In Java, abstraction can be achieved through concepts such as interfaces, abstract classes, and design patterns.
Why is Abstraction Important?
Abstraction serves several purposes:
- Reduces Complexity: By exposing only the necessary components, it minimizes the cognitive load on developers.
- Promotes Reusability: Abstract layers can be reused across different implementations, saving time and effort.
- Increases Flexibility: Changes in one part of the system won't necessarily affect unrelated parts.
What is Detail?
Detail refers to the comprehensive representation of the components within a system, emphasizing their specific functionalities and characteristics. While abstraction simplifies, detail encompasses the intricacies that allow parts to perform their tasks effectively.
Why is Detail Important?
Detail plays a crucial role in:
- Functionality: Accurate details ensure that a system works as intended.
- Debugging: When problems arise, having a detailed understanding of components helps in quicker troubleshooting.
- Optimization: Detailed designs allow for fine-tuning and performance enhancements.
Striking the Right Balance
Achieving a balance between abstraction and detail can be tricky. Too much abstraction can lead to confusion, making it hard for developers to understand how components interact. Conversely, focusing too much on details can overwhelm the user or create tight coupling among components, making maintenance difficult.
Best Practices for Balancing Abstraction and Detail in Java
Utilize Interfaces and Abstract Classes
Java inherently supports abstraction through interfaces and abstract classes. Interfaces define a contract that classes can implement, while abstract classes provide a base of common functionality.
// Interface defining the payment method
public interface PaymentMethod {
void pay(double amount);
}
// Implementation of CreditCard payment
public class CreditCard implements PaymentMethod {
@Override
public void pay(double amount) {
System.out.println("Paid " + amount + " using Credit Card.");
}
}
Commentary: Using an interface here abstracts payment methods from their implementations. This allows for different payment options to be easily implemented without changing the existing codebase.
Leverage Design Patterns
Design patterns provide well-established solutions to recurring design problems, promoting both abstraction and detailed implementation strategies.
Example: Strategy Pattern
The Strategy Pattern is a behavioral design pattern that defines a family of algorithms, encapsulates each one, and makes them interchangeable. This pattern allows a client to choose which algorithm to use during runtime.
// Strategy interface
public interface SortingStrategy {
void sort(int[] array);
}
// Concrete strategy implementing Bubble Sort
public class BubbleSort implements SortingStrategy {
@Override
public void sort(int[] array) {
// Implement bubble sort here
for (int i = 0; i < array.length - 1; i++) {
for (int j = 0; j < array.length - 1 - i; j++) {
if (array[j] > array[j + 1]) {
int temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
}
}
Commentary: In this example, the sorting algorithm is abstracted away from the client. The client can now sort an array without needing to know how bubble sort works internally, enhancing clarity while maintaining the required detail in sorting logic.
Refactor for Clarity
Regularly refactoring code can significantly improve both abstraction and detail management. Refactoring allows you to simplify complex sections by clarifying the responsibilities of classes or methods.
// Before refactoring - Long method with unclear responsibilities
public void handleOrder(Order order) {
// Handle validation
// Process payment
// Update inventory
// Notify user
}
// After refactoring - Clear responsibilities
public void handleOrder(Order order) {
validateOrder(order);
processPayment(order.getPaymentMethod());
updateInventory(order.getItems());
notifyUser(order);
}
Commentary: By breaking down the handleOrder
method into separate functions with concrete responsibilities, we enhance clarity while keeping the intricate details neatly encapsulated.
Embrace Dependency Injection
Dependency Injection (DI) is a software design pattern that implements Inversion of Control (IoC), allowing for better management of dependencies between classes, promoting loose coupling.
public class OrderService {
private final PaymentService paymentService;
// Constructor injection
public OrderService(PaymentService paymentService) {
this.paymentService = paymentService;
}
public void completeOrder(Order order) {
paymentService.processPayment(order.getPaymentMethod());
// Additional order completion logic
}
}
Commentary: Here, the OrderService
depends on the PaymentService
through constructor injection. This enhances testability and reduces the complexity of the class by clearly defining its dependencies.
The Bottom Line
Balancing abstraction and detail in Java design is pivotal for building efficient and maintainable software. By implementing concepts such as interfaces, design patterns, refactoring, and dependency injection, you can gracefully navigate the tension between simplicity and complexity.
Remember, the goal is not to eliminate detail or abstraction, but to use them harmoniously to forge clear and robust architectures. By adopting these principles, you can create Java applications that stand the test of time, both in performance and in readability.
For a deeper dive into design patterns, consider exploring Refactoring.Guru or further your understanding with Java Design Patterns. Happy coding!
Checkout our other articles